Java vs JavaScript: Understanding Key Differences
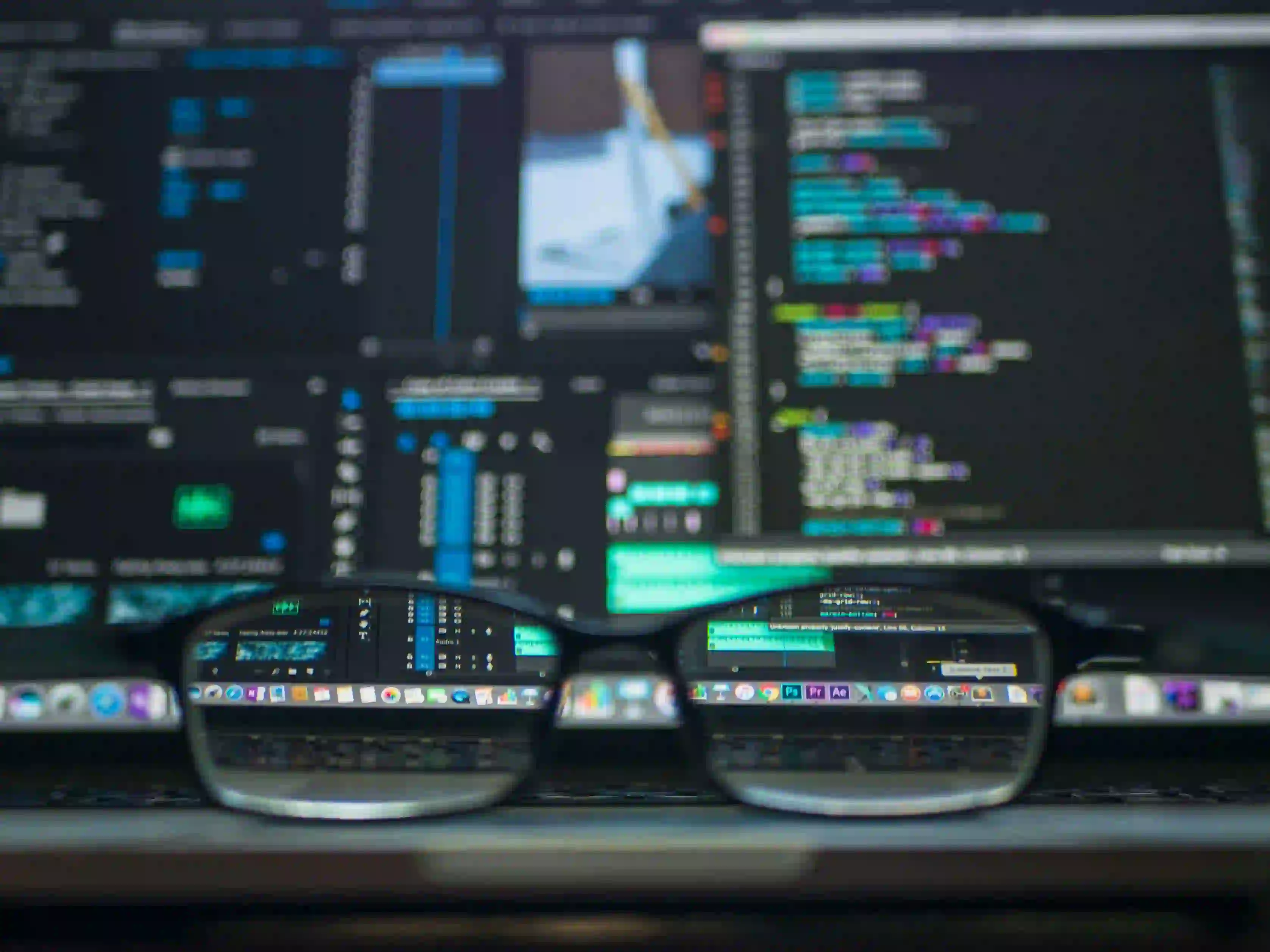
Java vs JavaScript: Understanding Key Differences
Java and JavaScript are two of the most widely-used programming languages today. Despite their similar names, they serve fundamentally different purposes and have distinct characteristics. Understanding these differences is essential for developers, businesses, and anyone interested in technology. In this blog post, we will explore the key differences between Java and JavaScript, their use cases, strengths, weaknesses, and much more.
What is Java?
Java is a versatile, object-oriented programming language designed for platform independence. Created by Sun Microsystems in 1995 and now owned by Oracle Corporation, it has remained popular due to its robust architecture, efficiency, and widespread use in large-scale applications.
Key Features of Java
- Object-Oriented: Java is fundamentally object-oriented, allowing for encapsulation, inheritance, and polymorphism.
- Platform Independence: Thanks to the Java Virtual Machine (JVM), Java applications can run on any device that has a compatible JVM.
- Strongly Typed: Java is statically typed, meaning type checking is performed at compile-time, thus reducing runtime errors.
- Multithreaded: Java supports multithreading, allowing concurrent execution of two or more threads.
Java Code Example
Here’s a simple Java code snippet that demonstrates a basic object-oriented program:
class Animal {
String name;
Animal(String name) {
this.name = name;
}
void makeSound() {
System.out.println(name + " makes a sound");
}
}
class Dog extends Animal {
Dog(String name) {
super(name);
}
@Override
void makeSound() {
System.out.println(name + " barks");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("Rex");
dog.makeSound(); // Output: Rex barks
}
}
Why This Code Matters
This code snippet introduces the concept of inheritance and method overriding, foundational aspects of object-oriented programming. By using these principles, developers can create reusable and maintainable code structures.
What is JavaScript?
JavaScript, developed by Netscape in 1995, is a scripting language primarily used for interactive web development. Unlike Java, JavaScript operates within the browser and is an essential component of front-end technologies like HTML and CSS.
Key Features of JavaScript
- Client-Side Scripting: JavaScript runs in the browser, allowing developers to create responsive and dynamic web pages.
- Prototype-Based: Instead of classes, JavaScript relies on prototypes, which allows for flexible object creation.
- Dynamic: JavaScript is dynamically typed, meaning variable types can change at runtime.
- Event-Driven: JavaScript excels in handling user interactions like clicks, hovers, and form submissions.
JavaScript Code Example
Here is a basic example of JavaScript that demonstrates the dynamic nature of the language:
function Animal(name) {
this.name = name;
}
Animal.prototype.makeSound = function() {
console.log(this.name + " makes a sound");
};
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.makeSound = function() {
console.log(this.name + " barks");
};
let dog = new Dog("Rex");
dog.makeSound(); // Output: Rex barks
Why This Code Matters
This code snippet illustrates prototype-based inheritance in JavaScript. While similar in concept to Java’s classes, it provides unique flexibility, allowing developers to define behavior on-the-fly, which is useful in web interactions.
Key Differences Between Java and JavaScript
1. Syntax and Structure
Both languages have a C-like syntax, but they differ significantly in approach. Java enforces strict OOP paradigms, whereas JavaScript supports multiple paradigms, including functional programming.
2. Typing
- Java: Statically typed; variables need explicit declarations. This reduces errors but requires more upfront planning.
- JavaScript: Dynamically typed; variable types can change, allowing for flexibility but increasing the potential for runtime errors.
3. Usage
- Java: Commonly used for backend development, mobile applications (Android), and enterprise solutions.
- JavaScript: Primarily used in front-end development, but with frameworks like Node.js, it is increasingly used on the server-side as well.
4. Performance
Java typically outperforms JavaScript due to its compiled nature and the optimization offered by the JVM. Java's performance makes it a preferred choice for heavy-duty applications, while JavaScript performs admirably for web applications due to its event-driven architecture.
5. Ecosystem and Libraries
The ecosystem surrounding both languages is vast:
- Java: Rich with frameworks like Spring, Hibernate, and tools like Maven for building enterprise solutions.
- JavaScript: Notable libraries and frameworks include React, Angular, and Vue.js for front-end development, while Node.js serves the backend needs.
Lessons Learned
Java and JavaScript are both powerful tools in a developer's toolkit, but they serve different aspirations and applications. Understanding their unique features, strengths, and weaknesses empowers developers to make informed decisions and leverage the right technology for their projects.
Whether you're building robust enterprise applications with Java or creating a fluid user interface with JavaScript, both languages hold immense potential. For further reading, check out the Java documentation and the JavaScript guide. Embracing the diverging paths of Java and JavaScript will enhance your skill set and keep you relevant in a fast-evolving tech landscape.
Final Thoughts
When deciding between Java and JavaScript for a project, consider the nature of the application, required performance, and your team's expertise. By understanding the fundamental differences between these two languages, you will be better prepared to make the most informed and effective choice for your development needs. Happy coding!