Debugging Common Issues in Eclipse RCP with Spring Boot
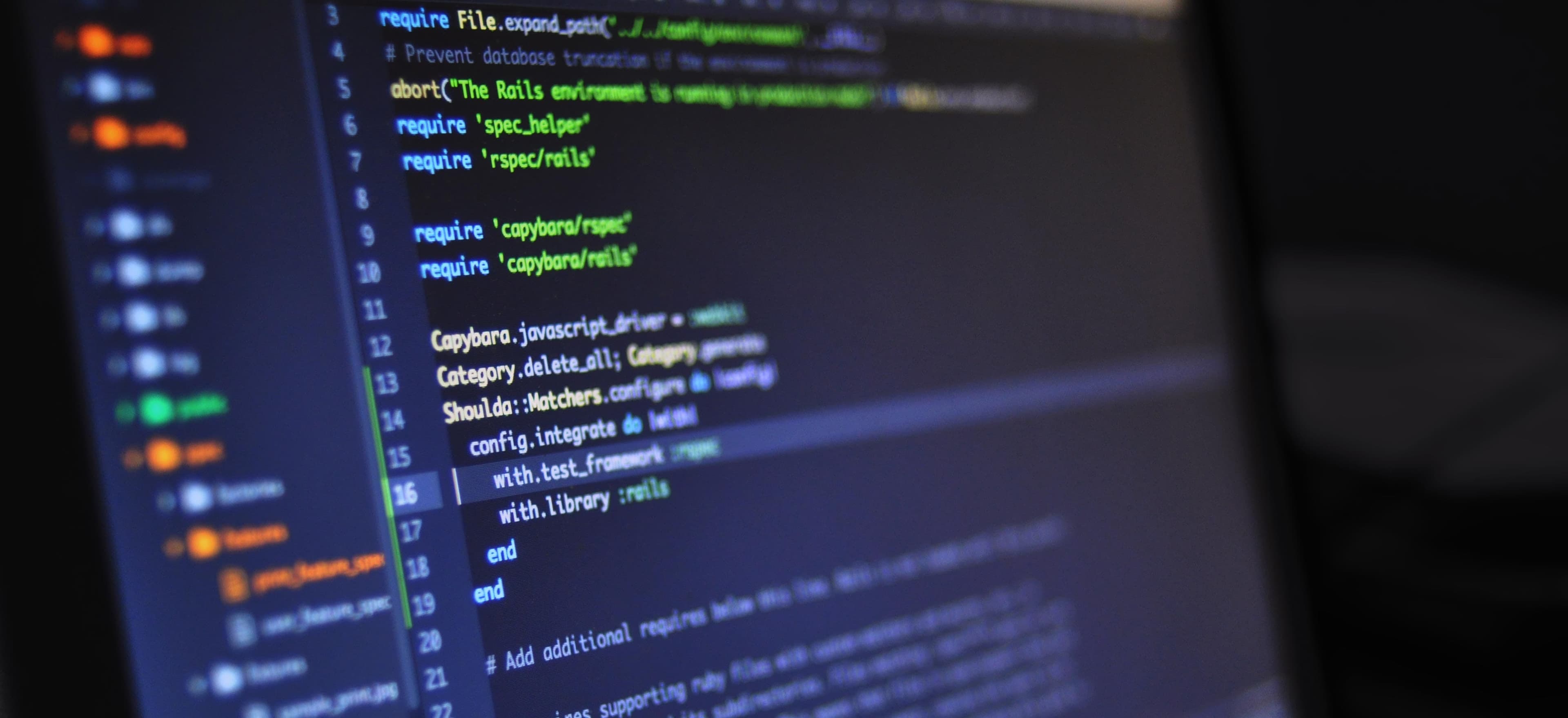
- Published on
Debugging Common Issues in Eclipse RCP with Spring Boot
Java Development is a powerful journey, and it becomes even more rewarding when you integrate the strengths of Eclipse Rich Client Platform (RCP) with Spring Boot. However, like any sophisticated technology stack, this combination can come with its own set of challenges. This blog post will guide you through common issues faced in Eclipse RCP when integrating with Spring Boot and how to debug these effectively.
The Roadmap to Eclipse RCP and Spring Boot
Before delving into debugging strategies, it's essential to understand the frameworks at hand. Eclipse RCP enables developers to create rich, desktop-based applications using the Eclipse platform. Meanwhile, Spring Boot simplifies the creation of stand-alone, production-grade Spring applications, minimizing configuration complexities.
Why Use Eclipse RCP with Spring Boot?
- Modularity: Eclipse RCP allows you to break your application into plug-ins. This structure can be cleaner than traditional Java applications.
- Embedded Server: With Spring Boot, you can run a servlet container directly from your application, unfurling the capability of creating desktop applications that require server-side logic without external servers.
Common Issues and Solutions
Let's explore some frequent issues developers encounter when integrating these two platforms and how to tackle them.
Issue 1: Dependency Injection Not Working
Spring Boot relies heavily on dependency injection. If your beans aren't being injected in the Eclipse RCP application, it may lead to NullPointerExceptions.
Solution
Ensure that you have properly set up the Spring Application Context. Use the @Configurable
annotation in your classes that need Spring-managed components.
@Component
public class MyResource {
public void execute() {
System.out.println("Executing Resource");
}
}
@Configurable
public class MyApplication {
@Autowired
private MyResource myResource;
public void start() {
myResource.execute(); // Ensure this is not null
}
}
Commentary
Here, the @Configurable
annotation helps you bridge Eclipse's own environment with Spring's management capabilities. If you're experiencing injection failures, always check if Spring component scanning is correctly set up.
Issue 2: Console Output Not Appearing
Sometimes, console outputs may not appear in the Eclipse Console when running your Spring Boot application from RCP. This can make debugging difficult.
Solution
Make sure you're correctly fetching the output stream from the Java process. Set your ApplicationWorkbenchWindowAdvisor
to capture this output.
@Override
public void preWindowOpen() {
IDE.setActiveWorkbenchWindowHandler(new MyConsoleHandler());
}
Commentary
Capturing console output is crucial for debugging. In this case, extending the workbench behavior will ensure critical logs are displayed directly in the Eclipse environment.
Issue 3: Bean Creation Errors
Spring Boot applications rely on the auto-configuration feature to create beans. Occasionally, a classpath issue might prevent Spring from creating necessary beans.
Solution
Inspect your dependencies and ensure all required libraries are included in your pom.xml
.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Commentary
Keeping your pom.xml
or build.gradle
in check is essential with Spring Boot. The classpath must include the necessary dependencies so that Spring can handle the instantiation.
Issue 4: Conflicting Versions of Libraries
Using different versions of libraries in your Eclipse project can lead to conflicts that hinder your application's functionality.
Solution
Using Spring's Dependency Management Plugin can help maintain consistent versions.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Commentary
This snippet ensures you are using versions recommended by Spring Boot, alleviating many compatibility headaches.
Debugging Tips
Apart from resolving common issues, here's how to enhance your debugging skills:
Enable Detailed Logging
Spring Boot allows you to set logging levels in application.properties
. For debugging:
logging.level.org.springframework=DEBUG
Use Eclipse's Debugger
Leverage the built-in debugger in Eclipse to step through your code. This feature is invaluable for identifying the precise location of errors.
- Set breakpoints.
- Run your application in debug mode.
- Inspect variable states at runtime.
Analyze Stack Traces
When exceptions occur, carefully analyze stack traces in the console. They provide information about where the problem originated.
Wrapping Up
Debugging an application that integrates Eclipse RCP with Spring Boot isn't without its challenges. However, understanding the common pitfalls and learning to troubleshoot them will significantly enhance your development experience.
For further insights on integrating Spring with other frameworks, consider visiting the Spring Official Documentation and Eclipse RCP Documentation.
Your feedback on this content is essential—share your experiences and issues you've encountered while working with these technologies. Happy coding!
Checkout our other articles