Enhancing Java UI Development with Sketch App Plugins
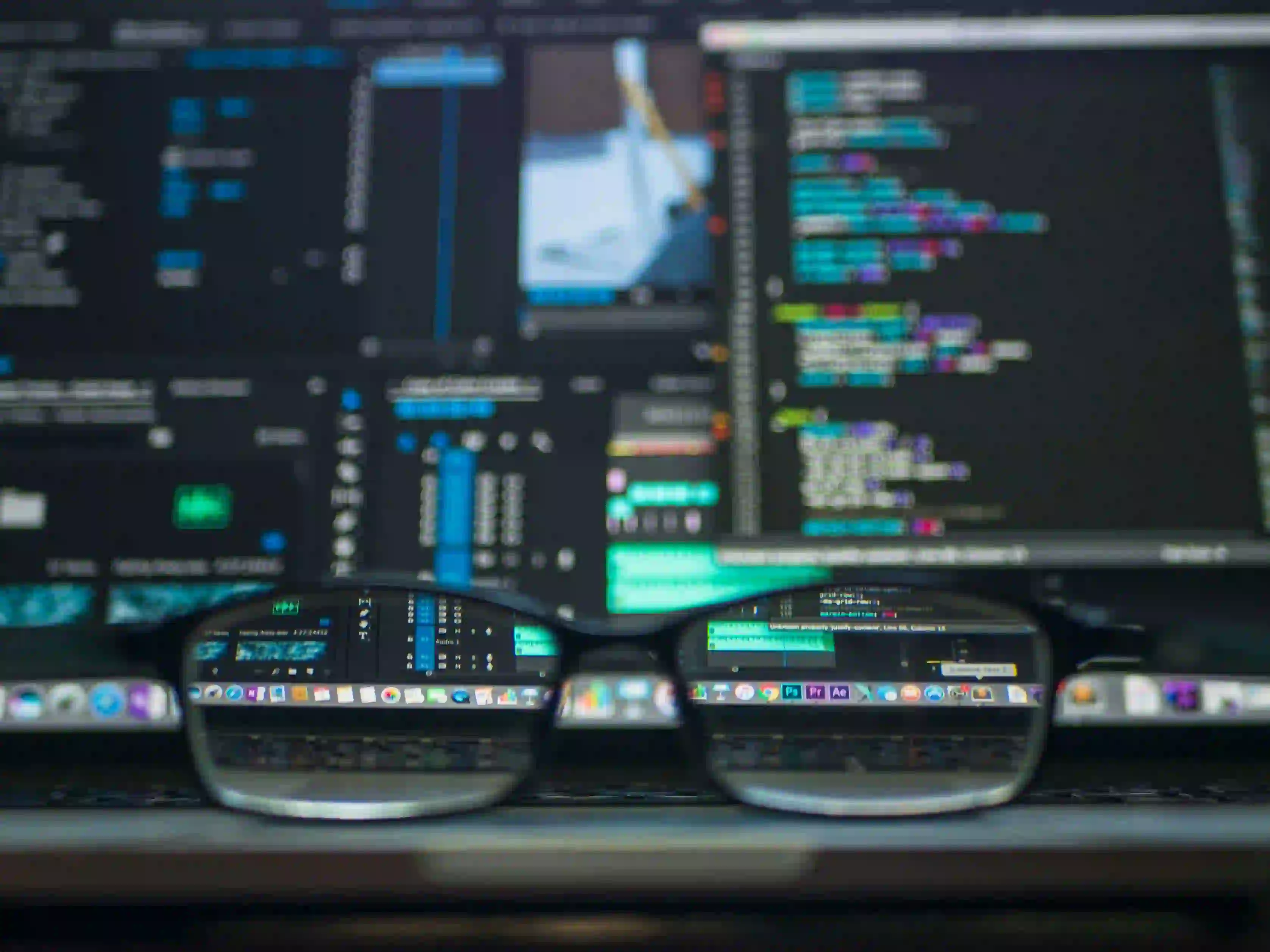
Enhancing Java UI Development with Sketch App Plugins
In the realm of software development, particularly in Java UI development, efficient design and prototyping are pivotal to creating a seamless user experience. One might not immediately associate a design tool like Sketch with Java programming, but the integration of Sketch App plugins can significantly elevate your workflow. This blog post will unveil how these plugins can be harnessed to enhance your Java UI development pipeline, ultimately leading to more efficient project delivery and improved user interfaces.
What is Sketch?
Sketch is a powerful vector-based design tool that has become a staple for UI and UX designers. Its intuitive interface, coupled with a plethora of plugins and assets, enables designers to craft beautiful and functional UI elements quickly. The flexibility of Sketch makes it an essential tool when designing user interfaces for Java applications.
Why Use Sketch Plugins?
With a rich ecosystem of plugins, Sketch automates repetitive tasks, enhances collaboration, and provides unique features that can transform your design workflow. For Java developers, using Sketch plugins can:
- Streamline Design Processes: By automating habitual tasks, you can reduce the time spent on routine design work.
- Enhance Collaboration: Plugins that facilitate design handoff to developers make it easier to translate designs into code.
- Improve Prototyping: Rapid prototyping with interactive components allows for testing design fidelity early in the development cycle.
For in-depth understanding and real-world applications, the article How Sketch App Plugins Can Boost Your Workflow digs deeper into these advantages.
Integrating Sketch in Java UI Development
Integrating Sketch into your Java UI development process provides a unique advantage. Here’s how:
-
Design-in Code Collaboration: Use plugins that enable designers and developers to communicate effectively. For example, “Craft” allows real-time collaboration, enabling developers to access design specifications directly from Sketch.
-
Design Systems: Many Java applications depend on UI consistency. By using plugins like “Symbol Organizer”, designers can create a design system that can be reused in multiple projects, ensuring aesthetic coherence.
-
Responsive Design Tools: Plugins such as “Sketch Responsively” allow designers to create dynamic layouts that adapt to different screen sizes, a common requirement in Java application development, particularly for mobile and web applications.
Example: Using Craft to Import Designs
Let’s delve into a practical example using the Craft plugin for Sketch. Craft allows you to pull design elements directly into your Java projects, which means you can focus more on coding your application rather than fiddling with design translations.
Here is a basic code structure for a Java Swing application that caters to a design created in Sketch:
import javax.swing.*;
public class BasicApp {
public static void main(String[] args) {
// Create frame
JFrame frame = new JFrame("My Sketch Inspired App");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
// Design element from Sketch
JPanel panel = new JPanel();
JButton button = new JButton("Click Me");
// Adding action listener to button
button.addActionListener(e -> {
JOptionPane.showMessageDialog(frame, "Button Clicked!");
});
// Layout
panel.add(button);
frame.add(panel);
frame.setVisible(true);
}
}
Why This Code Works
-
Clear Framework: This code snippet utilizes Java Swing, a robust framework for creating graphical user interfaces (GUI). Swing is simple yet powerful for UI development as it is part of the Java Foundation Classes.
-
Basic Components: It includes a basic JFrame and JPanel set up, akin to what you would be designing in Sketch. The JButton represents an interactive element you may design in Sketch before importing it.
-
Event Handling: The inclusion of
ActionListener
demonstrates interactivity, a crucial element in modern applications.
Best Practices for Integrating Sketch Plugins
Maintain Consistency
Maintaining design consistency is critical in development. Use plugins like “Style Organizer” to ensure that your colors, typography, and other stylistic elements are uniform throughout your design.
Optimize for Performance
When exporting assets from Sketch to use in your Java application, ensure that they are optimized for performance. Use the plugin “ImageOptim” for illustration assets. Compressed images not only reduce loading times but improve the overall UI performance.
Documentation is Key
When working collaboratively, documentation can bridge the gap between design and development. Ensure that your design files are well-documented and that any alterations made in Sketch are logged. Tools like “Data Populator” can help fill designs with real data, making your documentation meaningful.
A Final Look
Incorporating Sketch App plugins into your Java UI development process can profoundly improve your workflow. By harnessing the capabilities of Sketch, developers can spend less time translating designs into code and more time writing efficient and effective applications.
This integration not only enhances productivity but ensures that the final product is visually appealing and user-friendly. For further details on how Sketch plugins can enhance your work processes, refer to the article How Sketch App Plugins Can Boost Your Workflow.
Additional Resources
By adapting your design workflow with Java development through Sketch and its plugins, you are setting a precedent for future projects, making design a structured and less chaotic part of your coding journey. Happy coding!