Tackling Java Compatibility Issues with Legacy Browsers
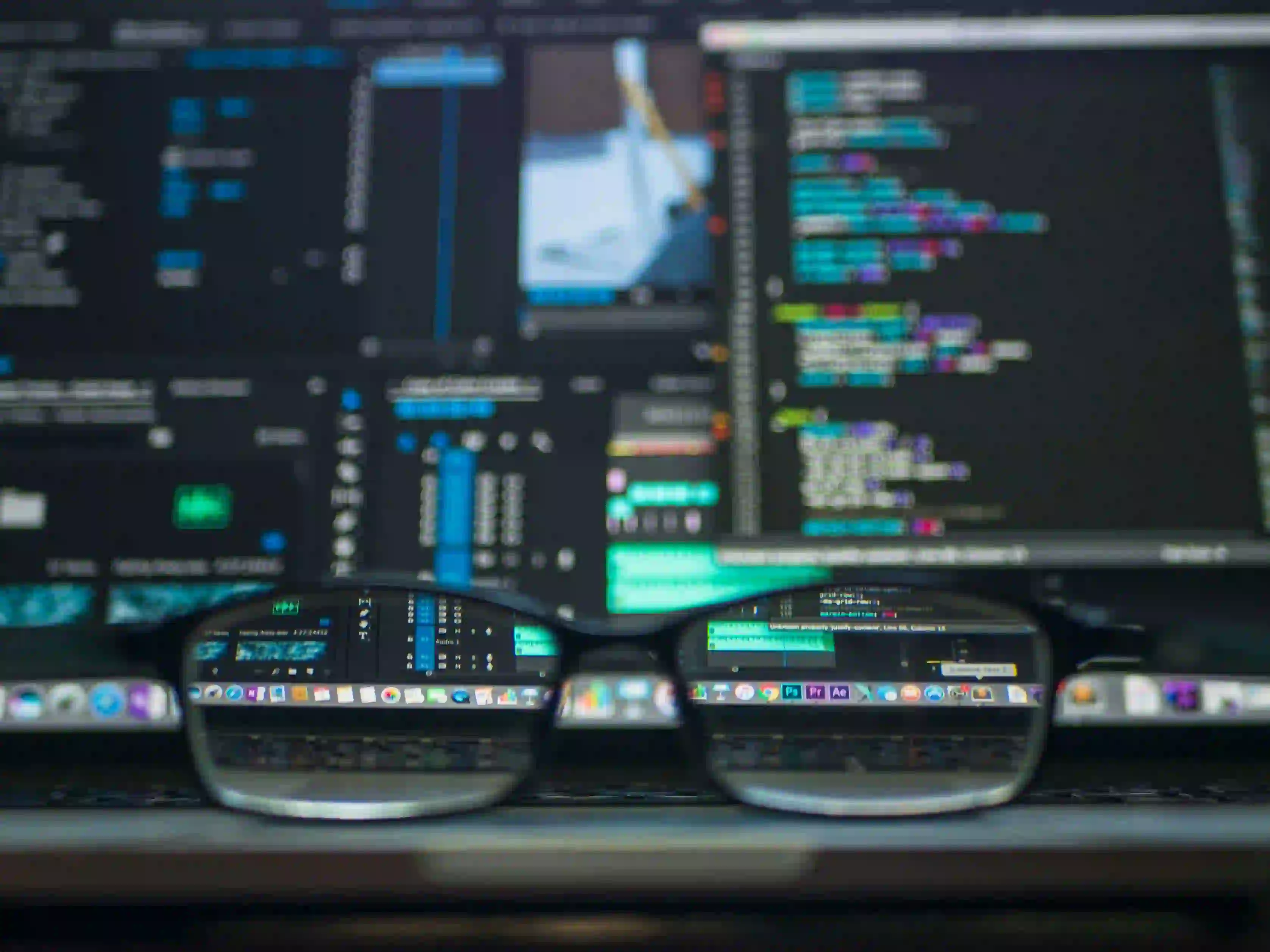
Tackling Java Compatibility Issues with Legacy Browsers
In today’s web-driven world, ensuring seamless functionality across various platforms is essential. However, developers frequently encounter compatibility issues, especially when dealing with legacy browsers. These browsers often struggle with modern technologies, leading to frustrating user experiences. A common example is the continued presence of bugs in older versions of Internet Explorer. For a deeper dive into that specific issue, refer to the article Why Some Versions of Internet Explorer Still Have Bugs.
Understanding the Landscape of Legacy Browsers
Legacy browsers, particularly older versions of Internet Explorer, present unique challenges. Support for modern web standards—such as HTML5, CSS3, and JavaScript ES6—can be abysmal. This lack of support can affect Java applets, JavaScript, and other web technologies.
Why Legacy Support Matters
Supporting legacy browsers is vital for several reasons:
-
User Base: Many users still rely on older browsers due to organizational policies or hardware limitations. Ignoring these can alienate a significant segment of your audience.
-
Business Implications: Companies that have invested in legacy systems may not update due to budget constraints. Their users, and consequently your potential users, might still be using outdated technology.
-
Security Concerns: Older browsers may lack critical security updates, making it risky for users to interact with modern web applications.
Considering these factors makes it clear that compatibility is more than just a technical issue—it's a user experience and business consideration.
Common Java Compatibility Issues
Developers often face specific challenges when working with Java in conjunction with legacy browsers:
-
Java Applet Support: Many legacy browsers have either limited or such poor compatibility with Java applets that they will not run correctly or at all.
-
JavaScript Dependencies: Java programs often rely on JavaScript for interactivity. If the JavaScript execution fails in a legacy browser, the entire application may also fail.
-
CSS Styling: Differences in CSS support can lead to design and layout discrepancies, making applications look broken.
To address these issues effectively, a mix of coding creativity and strategic planning is required.
Strategies for Tackling Compatibility Issues
1. Conditional Comments and Polyfills
Using conditional comments and polyfills allows developers to provide fallbacks or alternative scripts for legacy browsers.
<!--[if lt IE 9]>
<script src="https://code.polyfill.io/v3/polyfill.min.js"></script>
<![endif]-->
This snippet will load a polyfill for Internet Explorer versions lower than 9. Utilizing polyfills can help bridge the gap between modern functionality and legacy support, allowing users to experience a more seamless interaction with your Java applications.
2. Graceful Degradation
Graceful degradation entails designing your application to work on the latest browsers while still providing a basic experience in older versions.
For example, if you rely on advanced CSS features, ensure that your app can still function with basic styles. Here’s a small CSS example:
/* Modern CSS */
.box {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
/* Fallback for legacy browsers */
.box {
display: block;
}
In this CSS snippet, the application still works with basic display properties, ensuring compatibility without sacrificing too much modern functionality.
3. Server-Side Rendering (SSR)
Using server-side rendering can reduce the burden on legacy browsers by sending pre-rendered content. Java frameworks such as Spring allow developers to render views on the server side:
@Controller
public class HomeController {
@GetMapping("/home")
public String home(Model model) {
model.addAttribute("message", "Welcome to the legacy-compatible application!");
return "home"; // Returns home.html
}
}
This controller sets up a basic route that returns a view populated with data directly rendered from the server. Legacy browsers can then load this pre-rendered view without struggling with JavaScript.
4. Avoid Java Applets
Java applets are typically discouraged in modern web development due to their compatibility issues with browsers. In most cases, it is far more effective to rewrite the applet application as a Java Web Start application or, even better, convert the functionality directly into a web-based solution using JavaScript or WebSockets.
5. Feature Detection
Instead of relying on user-agent strings, use feature detection to check if a browser supports specific functionalities. Libraries like Modernizr can make this easier:
if (Modernizr.canvas) {
// Supported
// Your canvas-related code
} else {
// Unsupported
// An alternative
}
Feature detection helps ensure that the code only runs in compatible environments, thereby preventing incompatible applications from running in the first place.
Final Thoughts
Legacy browsers may seem like artifacts of the past, but their continued use demands that developers adapt to deliver a high-quality experience to all users. By implementing strategies such as polyfills, graceful degradation, SSR, avoiding applets, and feature detection, you can build Java applications that work effectively across different platforms. Doing so not only widens your user base but also enhances user satisfaction regardless of their browser choice.
Addressing Java compatibility issues is more than just code; it’s about understanding user needs and delivering solutions that keep pace with technological evolution.
For further reading on issues related to browser bugs and their implications in the broader web landscape, check out the article Why Some Versions of Internet Explorer Still Have Bugs.
By embracing these practices and remaining proactive about compatibility, you can ensure your applications thrive in any environment. Happy coding!