Java Strategies for Breaking Creative Blocks in Game Design
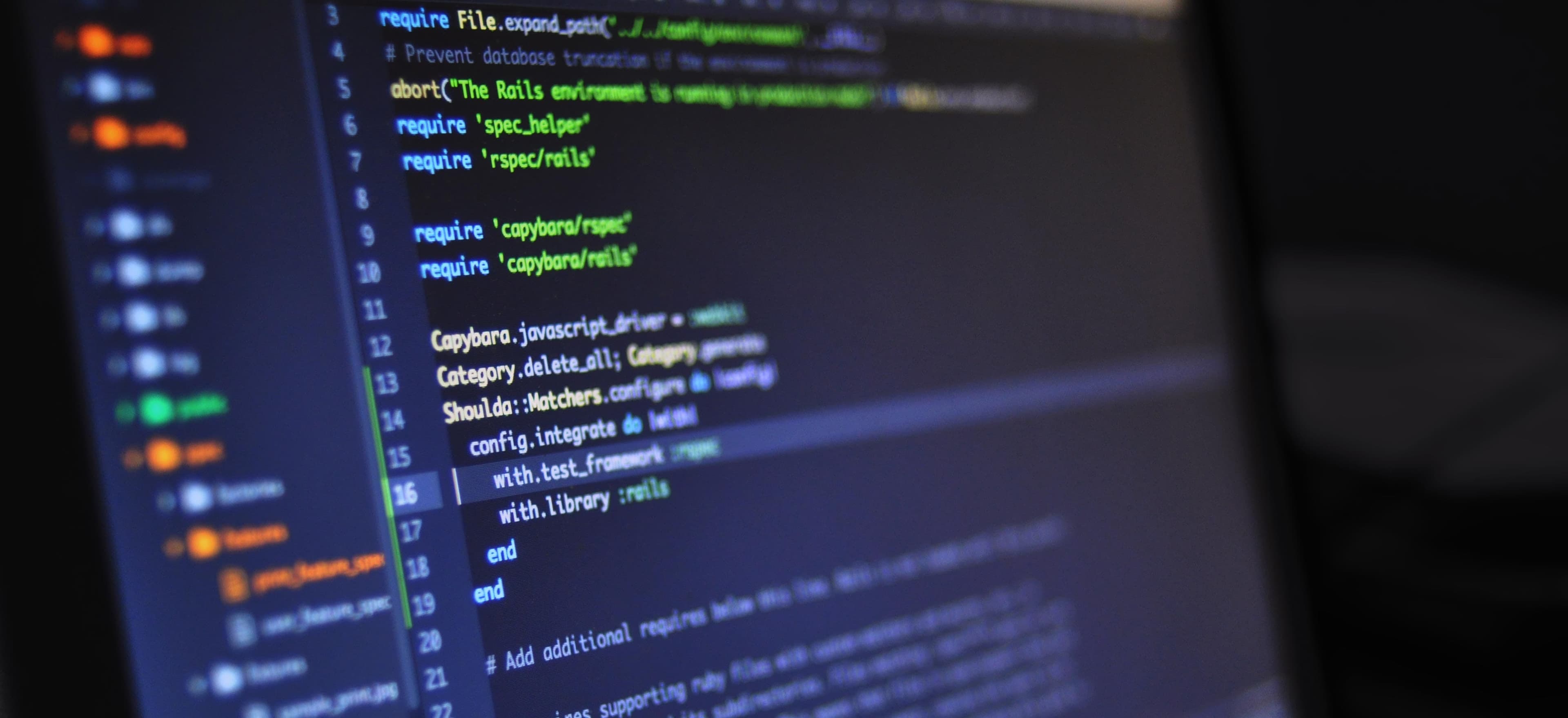
- Published on
Java Strategies for Breaking Creative Blocks in Game Design
Developing a game can be a fulfilling yet challenging endeavor. Often, developers encounter creative blocks that hinder their progress. This blog post will explore practical and effective strategies to overcome these blocks using Java, allowing you to maintain a steady workflow in your game development projects.
For those interested in a broader approach to managing creative challenges, I recommend checking out the article on How to Overcome Creative Block in Small Game Development. In this article, we will focus specifically on Java and how it can be leveraged in your game design process.
Understanding Creative Blocks
Before diving into Java-specific strategies, it's important to understand what creative blocks are. They can manifest as a lack of ideas, motivation, or direction in your projects. Identifying these blocks is the first step in breaking through them.
Common Causes of Creative Blocks
- Overthinking: Analyzing every detail can paralyze your creativity.
- Fear of Failure: Worrying about the outcome may prevent you from starting.
- Burnout: Prolonged periods of work can sap your enthusiasm.
- Lack of Inspiration: Without new ideas, it's hard to motivate the design process.
Java Strategies to Overcome Creative Blocks
1. Utilize Prototyping with Java
Prototyping allows you to quickly test ideas without committing to a full design. Java's object-oriented structure makes it easy to create simple prototypes.
class GamePrototype {
private String name;
public GamePrototype(String name) {
this.name = name;
}
public void play() {
System.out.println("Playing " + name);
}
public static void main(String[] args) {
GamePrototype breakout = new GamePrototype("Breakout");
breakout.play();
}
}
Why this works: Creating prototypes lowers the stakes, allowing you to play with mechanics and narratives freely. With Java's classes and methods, you can structure your prototypes effectively.
2. Build a Toolchain
Having a set of tools to automate your tasks can save time, reduce stress, and allow you to focus on creativity. Consider leveraging Java frameworks such as LibGDX or Unity for Java.
# Example to set up a LibGDX project
gradle setup
# Build the project
gradle build
Why this works: A streamlined toolchain means fewer distractions. By using well-supported frameworks, you spend less time on boilerplate code, directing your energy towards innovative ideas.
3. Take a Problem-Solving Approach
Identify specific challenges in your design and tackle them one by one using Java. Problem-solving not only provides clarity but also allows small victories.
public class Puzzle {
private int[] solution;
public Puzzle() {
// Simulate a complex puzzle
this.solution = new int[]{1, 2, 3, 4};
}
public boolean checkSolution(int[] userSolution) {
return Arrays.equals(solution, userSolution);
}
}
Why this works: By breaking down your project, you make it manageable. Using Java’s array manipulation features and methods, you can test your puzzles and iterate on them easily.
4. Incorporate Gameplay Mechanics from Other Games
Take inspiration from various game mechanics. Consider implementing a twist on an existing idea.
public class GameMechanic {
public void applyGravity(Entity entity) {
// Simplified representation of gravity
entity.setY(entity.getY() - 1);
}
}
Why this works: Experimenting with different mechanics might inspire new creativity. Java's extensive libraries make it easy to implement various behaviors and test their effectiveness.
5. Daily Coding Challenges
Engage in daily coding challenges to sharpen your skills and spark creativity. Websites like LeetCode or Codewars offer numerous challenges that you can solve using Java.
// Sample challenge: Reverse a string
public class StringReverser {
public String reverse(String input) {
return new StringBuilder(input).reverse().toString();
}
}
Why this works: Coding challenges push you out of your comfort zone, refine problem-solving skills, and often lead to revelations and fresh ideas applicable to your projects.
6. Collaborative Brainstorming
Working with other developers can introduce you to new perspectives. Use Java in sharing and building upon each other’s ideas.
public class TeamGame {
private List<String> ideas;
public TeamGame() {
ideas = new ArrayList<>();
}
public void addIdea(String idea) {
ideas.add(idea);
System.out.println("New idea added: " + idea);
}
}
Why this works: Collaboration fosters a creative environment. Java's collection framework allows for easy management of ideas, enabling you to focus on the content rather than the structure.
7. Break it Down to Fundamentals
Sometimes, it’s necessary to return to basics. Create small games that focus on fundamental concepts, like physics or mechanics.
public class SimpleGame {
public void run() {
// Basic game loop
while (true) {
// Game logic here
System.out.println("Executing game loop...");
}
}
}
Why this works: By simplifying your focus, you can reignite passion in game development. This approach often leads to finding new angles on more complex projects.
8. Set Time Limits for Creative Work
Establish strict time frames to brainstorm or code. Time constraints can energize your creative process.
public class Timer {
public void startTimer(long seconds) {
try {
Thread.sleep(seconds * 1000);
System.out.println("Time's up!");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Why this works: Imposing time limits can create urgency in your process, enhancing focus and productivity—not to mention breaking down the daunting task of game design into achievable steps.
Key Takeaways
Breaking through creative blocks in game design takes strategy, persistence, and sometimes, a fresh perspective. By leveraging Java's capabilities and combining it with effective practices, you can maintain momentum in your game development journey.
Utilize prototyping, problem-solving, collaboration, and the other strategies listed above to stimulate your creativity. Remember, every block is an opportunity for growth. If you are interested in more general techniques for overcoming creative challenges, consider reading the insightful article on How to Overcome Creative Block in Small Game Development.
Happy coding, and may your next game development adventure be fueled with creativity!
Checkout our other articles