Java UI Libraries: Solve Your CSS Button Styling Issues
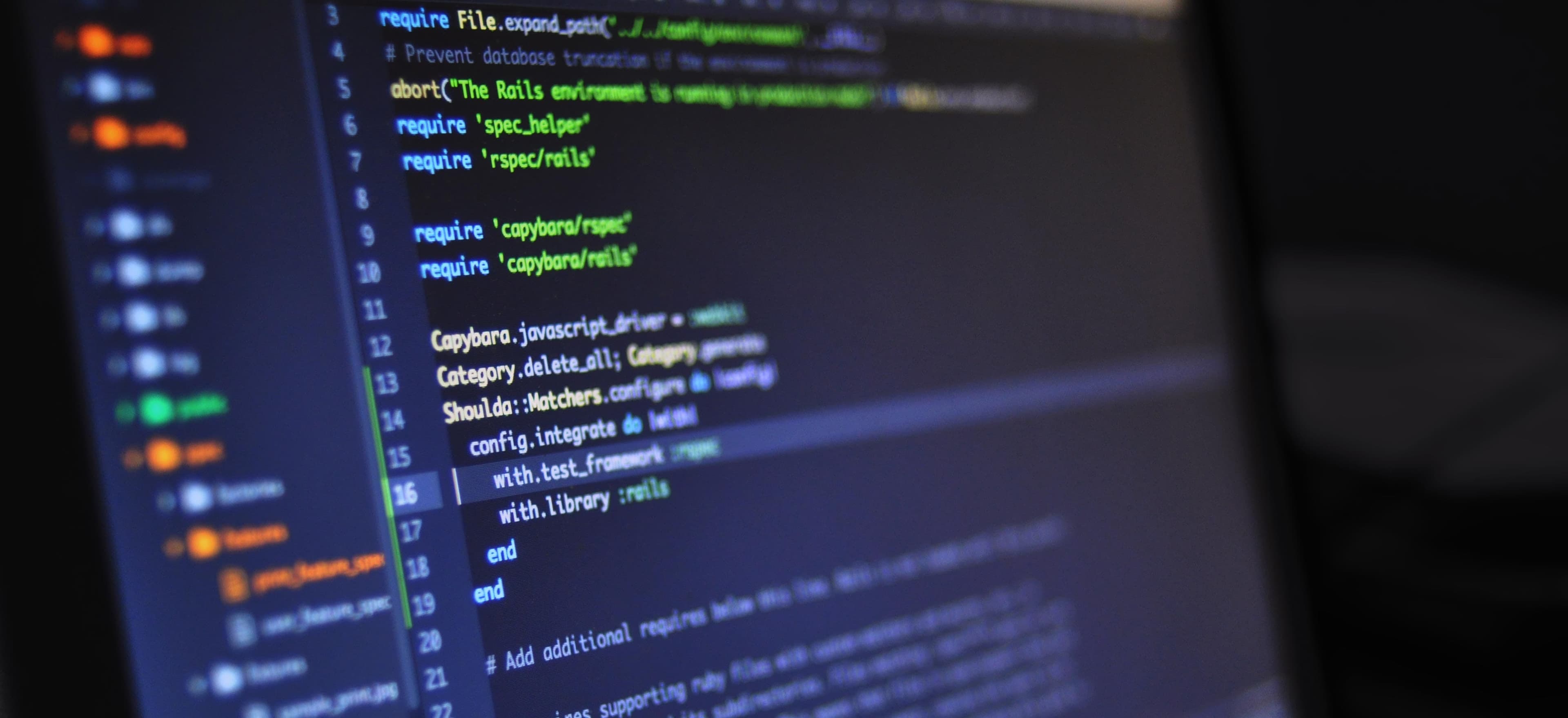
- Published on
Java UI Libraries: Solve Your CSS Button Styling Issues
Creating effective user interfaces in Java can be challenging, particularly when it comes to styling elements such as buttons. Many developers rely on CSS to achieve the perfect look, yet integration between Java UI libraries and CSS styling can sometimes lead to unexpected frustrations. This blog post aims to address common challenges associated with styling buttons in Java applications, and offer concrete solutions with the aid of popular Java UI libraries.
Understanding Java UI Libraries
Java offers several UI libraries to aid developers in building sophisticated user interfaces. Here are a few notable options:
- JavaFX: A modern UI toolkit providing rich graphical user interface capabilities. It allows for CSS styling similar to web applications.
- Swing: An older, yet still widely-used framework that provides a vast array of components and is suitable for building desktop applications.
- JIDE: A commercial UI toolkit that extends Swing with additional components and enhanced features for enterprise applications.
Before diving into styling solutions, let’s take a moment to understand the importance of a well-styled button in user interfaces. A button is not just a functional element; it serves as a medium for communication. Its appearance can influence user experience (UX) significantly.
Common Styling Issues
Before addressing styling problems, let's identify some common challenges that developers face when styling buttons in Java applications:
- Inconsistent Appearance: The default styles of buttons may vary from one platform to another.
- Lack of CSS Support: While Swing does not natively support CSS styling, JavaFX does, making it crucial to choose the right library based on your needs.
- Complicated Theme Implementation: Theming can become cumbersome, especially with legacy applications built using Swing.
Why Use CSS for Button Styling?
Using CSS for button styling can significantly enhance the appearance of your buttons. CSS provides a more flexible syntax for defining styling rules, enabling developers to easily create sophisticated designs without diving deep into Java's native methods for component styling. Additionally, CSS allows for rapid prototyping and easy updates to styles.
For more in-depth guidance on styling buttons using CSS, consider reading Styling Woes: Mastering CSS for Perfect Buttons.
Using JavaFX for CSS Button Styling
If you're using JavaFX, you can take full advantage of CSS styling. Here’s how you can create a styled button.
Example: JavaFX Button with CSS
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class StyledButtonExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
button.setId("my-button");
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 200);
scene.getStylesheets().add(getClass().getResource("style.css").toExternalForm());
primaryStage.setTitle("JavaFX Button Styling Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
- Setting an ID: We assign a unique ID to the button with
button.setId("my-button");
. This ID serves as a reference in CSS, allowing for targeted styles. - Loading the CSS: The CSS file is added to the scene using
scene.getStylesheets().add(...)
. Ensure thatstyle.css
is in the correct path relative to your class file.
Next, let’s take a look at what the style.css
might contain.
Example: style.css
#my-button {
-fx-background-color: #4CAF50; /* Green background */
-fx-text-fill: white; /* White text */
-fx-padding: 10px 20px; /* Spacing around the text */
-fx-font-size: 16px; /* Font size increase */
-fx-border-radius: 5px; /* Rounded corners */
-fx-background-radius: 5px; /* Rounded corners for background */
}
#my-button:hover {
-fx-background-color: #45a049; /* Darker green when hovered */
}
Key Features of this CSS
- Customization: Each CSS property directly impacts the button's appearance, making it easy to customize without altering Java code.
- Hover Effect: The button changes color on hover, improving user feedback and interaction.
- Rounded Corners: Consistent styling promotes modern UI design practices.
Solving Swing Button Styling Issues
When working with Swing, styling buttons requires a bit more effort. Swing's component customization is typically done using the setUI
and setBorder
methods. Here’s a simple example of how to customize a JButton.
Example: Styled JButton in Swing
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
public class SwingStyledButton {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Swing Button Styling Example");
JButton button = createStyledButton("Click Me");
frame.setLayout(new FlowLayout());
frame.add(button);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
});
}
private static JButton createStyledButton(String text) {
JButton button = new JButton(text);
button.setBackground(Color.GREEN);
button.setForeground(Color.WHITE);
button.setBorder(new EmptyBorder(10, 20, 10, 20));
button.setFont(new Font("Arial", Font.BOLD, 16));
button.addActionListener(e -> System.out.println("Button Clicked!"));
return button;
}
}
Commentary on the Code
- Color Customization: The
setBackground
andsetForeground
methods are used to define the button's background and text color. - Borders: The
setBorder(new EmptyBorder(...))
method allows for padding around the button to make it look neat. - Font Styles: By defining a custom font using
setFont(...)
, the button visually stands out.
Closing Remarks
Styling buttons in Java applications can be a tricky endeavor, but the right approach can drastically simplify the process. Whether you choose JavaFX for its seamless CSS integration or Swing with strategic customization, understanding each library's strengths is key to achieving the desired results.
Incorporating CSS into your JavaFX applications not only helps maintain a modern look, but it allows easier updates and adjustments down the line. Should you run into complex styling issues, remember to consult articles like Styling Woes: Mastering CSS for Perfect Buttons for advanced techniques that can refine your UI design.
As you continue your programming journey, embrace these solutions to create polished, professional buttons that enhance your user experience. Keep experimenting with Java's robust libraries and take full advantage of the graphical capabilities they offer!
Checkout our other articles