Unlocking Java Creativity: Overcoming Developer's Block
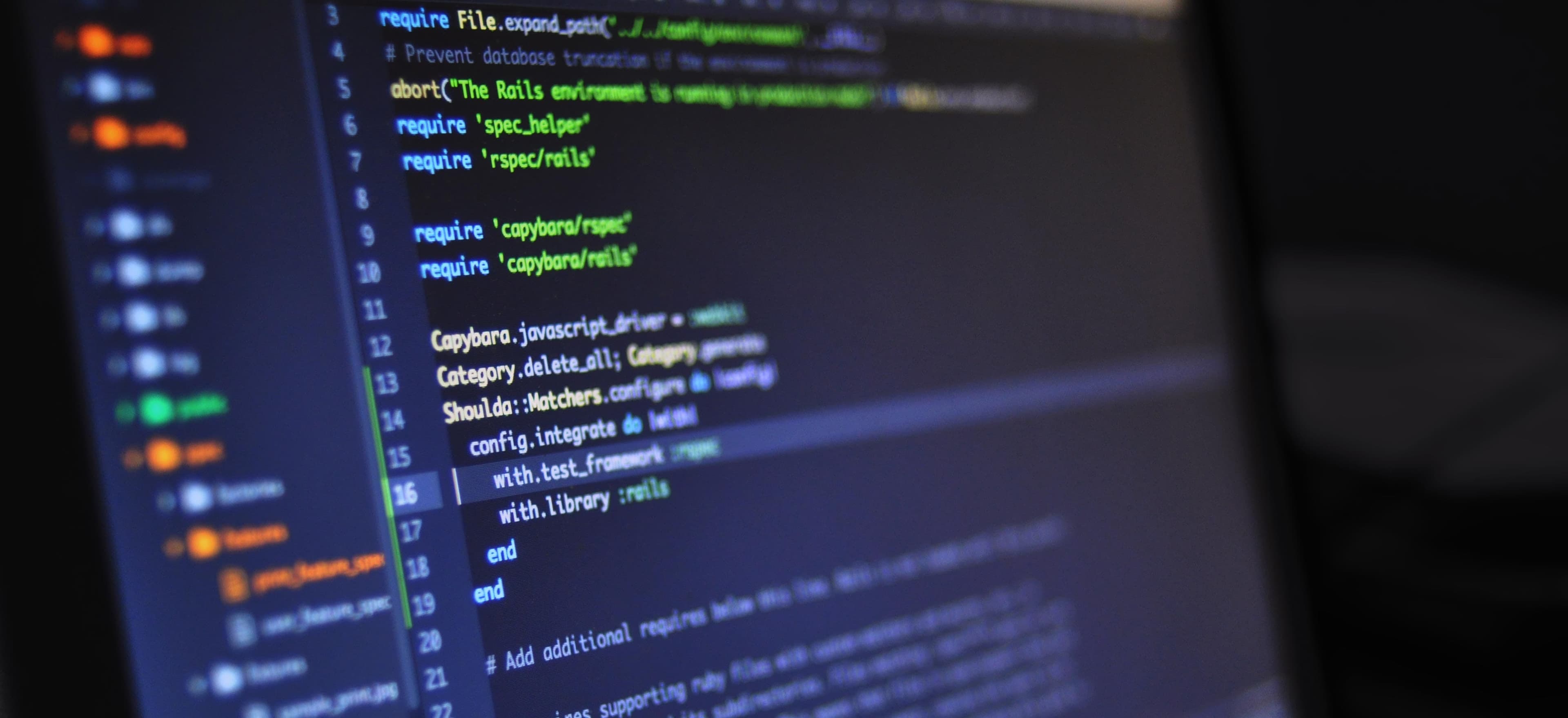
- Published on
Unlocking Java Creativity: Overcoming Developer's Block
As developers, we often find ourselves facing a familiar foe: the dreaded creative block. This phenomenon can be particularly challenging within the realm of Java programming, where the breadth of possibilities can simultaneously inspire and paralyze us. If you're seeking a way to reignite your creative spark, you’re not alone.
In this blog post, we will explore effective strategies to overcome creative block in Java development, drawing insights from broader creative processes. We will also unravel some Java concepts and provide practical code snippets that reflect innovative solutions.
Understanding Developer's Block
Developer's block can manifest in various ways: blank screens, helplessness, or overwhelming indecision. As discussed in the article "How to Overcome Creative Block in Small Game Development", creative blocks can stem from perfectionism, overloaded schedules, or simply a lack of inspiration. In programming, this can lead to stalled projects, frustration, and a stunted learning curve.
Identifying Your Block
Before you can tackle a creative block, it is crucial to identify the root cause. Is it technical incompetence, fear of failure, or perhaps a lack of direction? Once you pinpoint your block, you can implement effective strategies to overcome it.
Strategy 1: Build Small Projects
The easiest way to regain your creative juices is by building small projects. Small, manageable tasks prevent you from feeling overwhelmed.
Example: A Simple Java Calculator
Let’s illustrate this with a simple calculator project.
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter first number: ");
double firstNumber = scanner.nextDouble();
System.out.println("Enter second number: ");
double secondNumber = scanner.nextDouble();
System.out.println("Choose an operation (+, -, *, /): ");
char operation = scanner.next().charAt(0);
double result;
switch (operation) {
case '+':
result = firstNumber + secondNumber;
break;
case '-':
result = firstNumber - secondNumber;
break;
case '*':
result = firstNumber * secondNumber;
break;
case '/':
if (secondNumber != 0) {
result = firstNumber / secondNumber;
} else {
System.out.println("Cannot divide by zero");
return;
}
break;
default:
System.out.println("Invalid operation");
return;
}
System.out.println("Result: " + result);
}
}
Why This Exercise Works
This calculator project is straightforward, allowing you to relive the joy of coding without an immense time commitment. Completing small tasks gives you a sense of achievement and helps rebuild your confidence.
Strategy 2: Explore New Libraries and Frameworks
Java has a rich ecosystem, and harnessing new libraries can inspire fresh concepts. Discover new frameworks that complement Java, such as Spring or Hibernate, or even dive into libraries like JUnit for testing.
Example: Using JUnit for Testing
Using JUnit to test our simple calculator can enhance our coding proficiency and serve as an inspirational springboard.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class SimpleCalculatorTest {
@Test
public void testAddition() {
assertEquals(5, SimpleCalculator.add(2, 3), "2 + 3 should equal 5");
}
@Test
public void testSubtraction() {
assertEquals(1, SimpleCalculator.subtract(3, 2), "3 - 2 should equal 1");
}
}
Why Testing Enhances Creativity
Engaging with testing frameworks fosters a structured approach to programming that can liberate creativity. It raises confidence in code quality, allowing developers to pursue more ambitious ideas without fear of failure.
Strategy 3: Collaborate and Share Ideas
As passionate developers, collaboration with peers can spark innovative thoughts. Engage with your team or join online communities like Stack Overflow, GitHub, or Reddit. Not only can you gain feedback, but sharing your experiences can also inspire others.
Creating a Project Collaboration on GitHub
This can be as simple as starting a Java project repository:
- Create a new repository on GitHub.
- Write a README file explaining the project’s goals.
- Invite collaborators.
By working together, new ideas and perspectives can emerge, leading to novel solutions.
Strategy 4: Set Realistic Goals
Setting unrealistic deadlines can stifle creativity and lead to anxiety. Break projects into smaller, achievable goals and set firm, realistic deadlines.
Example of Dividing a Project Into Smaller Goals
Instead of aiming to build a comprehensive Java application in one go, define incremental targets such as:
- Week 1: Set up the project structure and basic functionalities.
- Week 2: Implement core features.
- Week 3: Optimize and test.
Strategy 5: Take Breaks and Reflect
Sometimes, stepping away is the best way to gain clarity. Engaging in other creative activities—like writing, drawing, or playing games—can rejuvenate your mind.
When you do return to your Java project, you may find fresh ideas surfacing. As highlighted in the article referenced earlier, creative blocks can be alleviated through self-care and intentional reflection.
Mind Mapping for Reflection
Using mind maps can help visualize project ideas, connect concepts, and brainstorm without limitations. Tools like XMind or simple pen and paper are excellent for this exercise.
Final Considerations
Every developer faces creative blocks from time to time. Whether through building manageable projects, collaborating with others, or implementing testing practices, there are myriad ways to overcome these barriers.
Keep in mind that creativity is as much about experimentation as it is about process. Embrace new libraries and frameworks as potential catalysts for inspiration.
Should you feel stumped, refer back to the techniques and strategies discussed in the article "How to Overcome Creative Block in Small Game Development" or reach out to your fellow developers for support.
Let your creativity flow freely, and you will find innovative solutions in your Java programming journey. Happy coding!
Checkout our other articles