JavaFX and CSS: Solving Your Button Styling Challenges
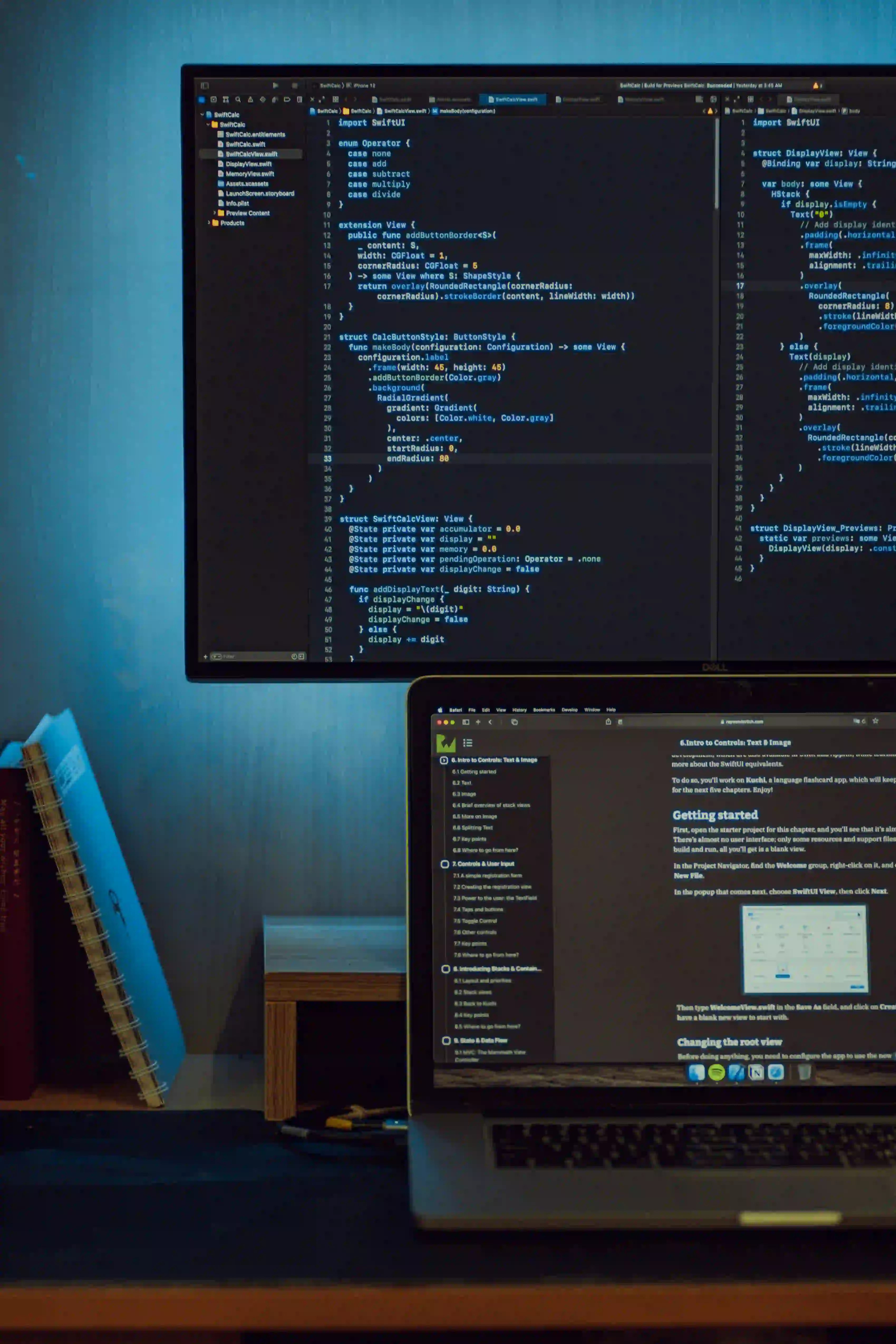
JavaFX and CSS: Solving Your Button Styling Challenges
In the world of JavaFX, designing a visually appealing user interface is crucial for delivering a great user experience. With the power of CSS, you can enhance your JavaFX applications remarkably, especially when it comes to styling UI elements like buttons. This blog post aims to bridge the gap between JavaFX and CSS, empowering you to tackle button styling challenges effectively.
Why JavaFX?
JavaFX is a versatile framework designed for building rich client applications. It provides a powerful set of tools to create dynamic and visually stunning UIs. As more developers turn to JavaFX for modern applications, mastering the art of styling becomes essential. A polished button can go a long way in improving usability and aesthetics.
The Role of CSS in JavaFX
CSS (Cascading Style Sheets) is not just limited to web design; it finds its place in JavaFX too. By implementing CSS in JavaFX, you can separate the design from the code, leading to cleaner, more maintainable applications.
Key Benefits of Using CSS with JavaFX
-
Separation of Concerns: CSS allows you to keep your styling rules separate from your Java code. This leads to better organization and maintainability.
-
Reusable Styles: With CSS, you can define styles once and reuse them across different components. This promotes consistency in your UI.
-
Dynamic Styling: CSS enables you to change appearance dynamically based on user interactions or state changes.
The Basics of CSS Styling in JavaFX
Before we dive into advanced styling techniques, let's cover the basics. Here's how to apply a simple CSS style to a JavaFX button.
Example 1: Basic CSS for a Button
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ButtonStylingExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
button.setId("my-button"); // Assign an ID for CSS styling
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 250);
scene.getStylesheets().add(getClass().getResource("style.css").toExternalForm()); // Linking CSS
primaryStage.setTitle("JavaFX Button Styling");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example:
- We create a basic JavaFX application with a single button.
- The
setId
method is used to assign an ID to the button, enabling us to style it uniquely in our CSS file. - The
getStylesheets().add
method links the external CSS file namedstyle.css
.
corresponding CSS (style.css)
#my-button {
-fx-font-size: 16px; /* Set font size */
-fx-background-color: lightblue; /* Set background color */
-fx-text-fill: darkblue; /* Set text color */
-fx-padding: 10px; /* Padding around the text */
-fx-border-radius: 5px; /* Rounded corners */
-fx-background-radius: 5px; /* Rounded corners on background */
}
Why This Matters
You may be wondering why it's essential to specify properties like padding
, border-radius
, and background-color
. These properties contribute to a button's overall feel and usability. A button that looks well-designed is more inviting to users, enticing them to interact with your application.
Solving Common Button Styling Challenges
1. Hover Effects
A hover effect can improve the interactivity and responsiveness of your button. Let's revisit our CSS file to add hover effects.
CSS (style.css)
#my-button:hover {
-fx-background-color: deepskyblue; /* Change background on hover */
-fx-text-fill: white; /* Change text color on hover */
}
Why Hover Effects?
Hover effects signal to users that the button is clickable. It enhances the user experience by providing visual feedback.
2. Active State Feedback
Showing users that their click is being acknowledged is vital. Let’s add styles for when the button is pressed.
CSS (style.css)
#my-button:pressed {
-fx-background-color: dodgerblue; /* Darken background on press */
-fx-text-fill: white; /* Keep text color for contrast */
}
Why Active States Matter?
Active states provide immediate feedback that an action is being performed. This alleviates uncertainty for users who may be waiting for a response.
Advanced Styling: Styling Multiple Buttons
Suppose you have multiple buttons and want to apply the same styles. Using .class
selectors ensures consistency across your UI elements.
Example: Java Code with Multiple Buttons
Button button1 = new Button("Button 1");
button1.getStyleClass().add("my-button");
Button button2 = new Button("Button 2");
button2.getStyleClass().add("my-button");
Corresponding CSS (style.css)
.my-button {
-fx-font-size: 14px;
-fx-padding: 12px;
-fx-background-color: #66ccff;
-fx-text-fill: white;
-fx-border-radius: 8px;
-fx-background-radius: 8px;
}
.my-button:hover {
-fx-background-color: #3399ff; /* Lighter blue on hover */
}
Why Use Class Selectors?
Class selectors allow you to style multiple elements consistently. This minimizes redundancy and ensures uniformity across your application.
Incorporating Media Queries for Responsive Design
It’s vital to adapt your UI for different screen sizes, especially with JavaFX applications that run on various platforms. You can achieve this via media queries in your CSS.
Example (style.css)
@media screen and (max-width: 600px) {
.my-button {
-fx-font-size: 12px; /* Decrease font size on small screens */
-fx-padding: 8px; /* Adjust padding */
}
}
Why Media Queries?
Incorporating media queries ensures that your application maintains usability across different devices, enhancing user experience for all users.
Key Takeaways
JavaFX, when paired with CSS, provides developers with robust tools to create attractive and user-friendly interfaces. Button styling, although a small aspect, is essential in enhancing the overall user experience.
To delve deeper into button styling, consider referring to the article titled "Styling Woes: Mastering CSS for Perfect Buttons." This article provides additional insights that can complement your JavaFX projects beautifully.
By mastering CSS for your buttons, you’ll create a polished, professional user interface that engages users effectively. So, start experimenting with styles and make your JavaFX applications shine!
Ready to dive deeper? Explore more on JavaFX and its advanced styling capabilities in your projects, and let your creativity run wild!