Boosting Java Performance: Caching with useMemo and useCallback
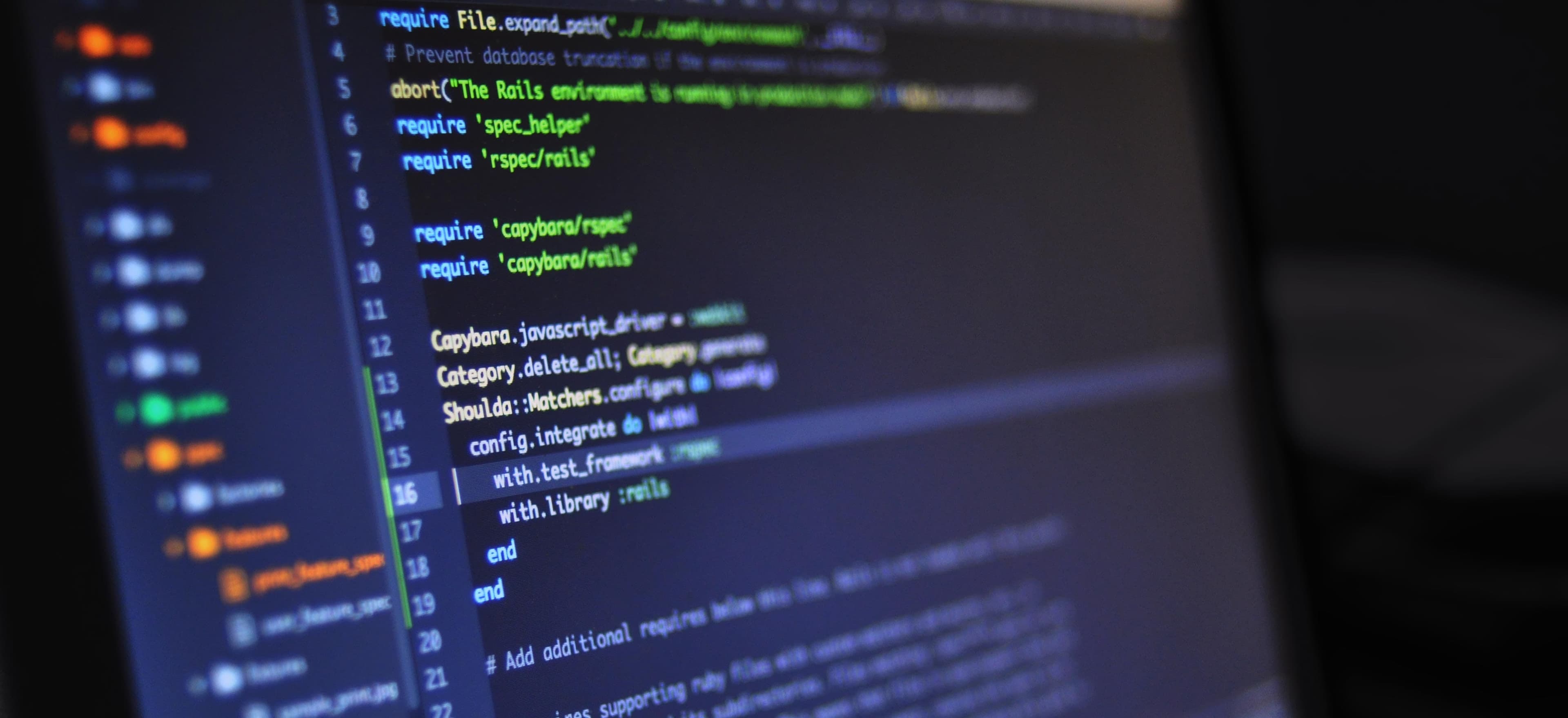
- Published on
Boosting Java Performance: Caching with useMemo and useCallback
When it comes to developing Java applications, performance is often a critical consideration. In the realm of web development with frameworks like React, caching becomes essential—especially in optimizing performance and user experience. This blog post will explore how to leverage caching in Java applications and draw parallels with the concepts of useMemo
and useCallback
in React, which are extensively discussed in an article titled Mastering useMemo and useCallback for Efficient React Caching.
Understanding Caching in Java
What is Caching?
Caching is the process of storing copies of files or data in a temporary storage location for quick access. By keeping frequently accessed data in memory, applications can reduce the time spent retrieving data from slower sources, such as databases or APIs.
Benefits of Caching
- Performance Improvement: Faster data access reduces the load time of applications.
- Reduced Database Load: Less frequent queries lower strain on the database, allowing for more scalability.
- Cost Efficiency: By minimizing data retrieval requests, caching can help reduce operational costs.
Types of Caching
In Java development, caching can take many forms, including:
- In-memory Caching: Data is stored temporarily in the application’s memory. Popular libraries include Ehcache, Caffeine, and Guava.
- Distributed Caching: Data is shared across multiple servers, useful for large scale applications. Options include Redis and Hazelcast.
- Page Caching: Storing entire responses for repeated requests, especially useful in web applications.
Implementing In-Memory Caching in Java
Let's demonstrate how to implement simple in-memory caching using Caffeine, which is a high-performance caching library for Java. it provides a lightweight solution with minimal setup.
Maven Dependency
First, you need to add the Caffeine dependency in your pom.xml
:
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.2</version>
</dependency>
Caching Example
Here is an example where we cache the results of a computation-intensive function.
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class ExpensiveComputation {
private final Cache<Integer, String> cache;
public ExpensiveComputation() {
// Configuring the cache with an expiry time of 10 minutes
this.cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build();
}
public String compute(int input) {
// Using caching to avoid recomputation
return cache.get(input, this::performExpensiveComputation);
}
private String performExpensiveComputation(int input) {
// Simulate a heavy computation
try {
Thread.sleep(1000); // simulates time-consuming process
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Result of " + input;
}
}
Explanation of the Code
-
Cache Initialization: A new
Cache
is created with a maximum size of 100 entries that expire after 10 minutes. Such settings help manage memory effectively while keeping frequently accessed data. -
Computing with Cache: The
compute
method checks if the result is in the cache. If it is not present, it invokesperformExpensiveComputation
to fetch and cache the result. -
Heavy Computation Simulation: The
performExpensiveComputation
simulates a resource-intensive process. This method should ideally contain the logic you want to cache.
When to Use In-Memory Caching
Using in-memory caching is appropriate when:
- Data retrieval from an external source is costly.
- The estimated size of the dataset fits comfortably in the available memory.
- You need low-latency access to cached data.
Drawing Parallels with useMemo and useCallback in React
In React, caching efficiencies are brought to life with hooks like useMemo
and useCallback
.
useMemo
The useMemo
hook allows developers to optimize performance by caching the result of a computation between renders. The function inside useMemo
will only re-run when its dependencies change.
Example:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
In the above code, the computeExpensiveValue
function is executed only when a
or b
change, enhancing performance.
useCallback
On the other hand, useCallback
is used for memoizing functions. It helps prevent unnecessary re-renders of child components by ensuring that function references remain consistent between renders.
Example:
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
This ensures that the doSomething
function retains the same reference unless either a
or b
changes.
Why useMemo and useCallback?
Both hooks are essential for optimizing React applications, particularly in complex state management. They ensure that your application only recalculates values or recreates functions when necessary.
Performance Considerations
-
Balance Complexity with Performance: While caching can boost performance, it also adds complexity. Assess whether the improvement justifies the added complexity.
-
Cache Size Management: Ensure that you have appropriate size limits. A cache that grows indefinitely can lead to memory exhaustion.
-
Cache Invalidation: Develop strategies for cache invalidation. Cached data can become stale, which may lead to inconsistencies in your application.
Lessons Learned
Caching is a powerful strategy to enhance performance in Java applications, much like how useMemo
and useCallback
provide optimization in React. By utilizing tools like Caffeine for in-memory caching, developers can significantly reduce the computational overhead associated with repeated data-fetching tasks.
By embracing these techniques, you not only boost performance but also elevate the user experience in your applications. Interested in reading more about performance optimization in web frameworks? Check out the Mastering useMemo and useCallback for Efficient React Caching article to dive deeper into caching in the React ecosystem.
Further Reading
- Caffeine GitHub Repository
- Java Caching Best Practices
This comprehensive approach to caching will bring your Java applications to the next level, paving the way for more responsive and faster systems. Happy coding!
Checkout our other articles