Mastering Safe Code Transfer: Java’s Take on Copy-Pasting Issues
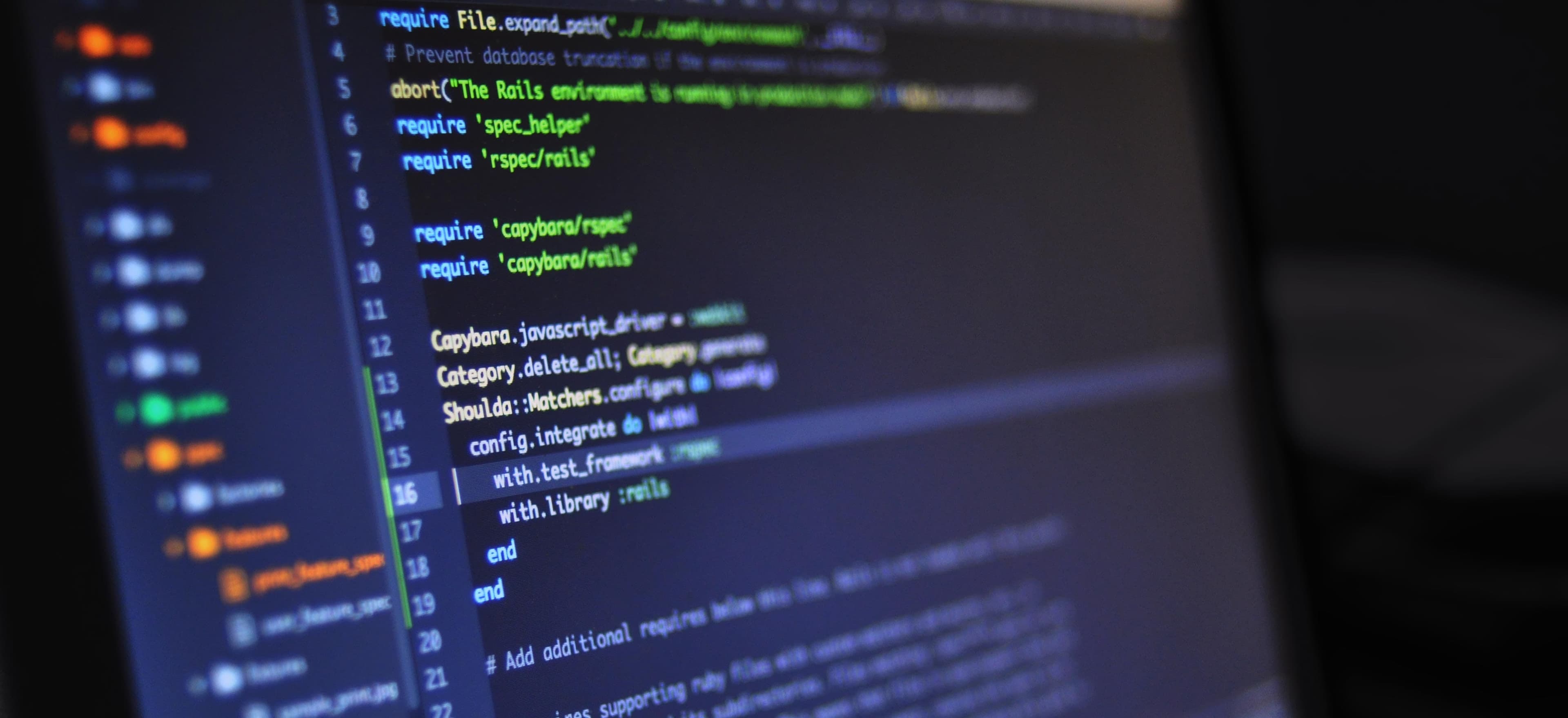
- Published on
Mastering Safe Code Transfer: Java’s Take on Copy-Pasting Issues
In today's fast-paced development environments, code reuse has become an essential aspect of software development. Java, being one of the most popular programming languages, is no exception. But while copy-pasting code can save time and enhance productivity, it often introduces a minefield of errors and bugs. In this blog post, we will delve into the challenges of code transfer and how Java developers can mitigate these issues.
Understanding Copy-Pasting in Programming
Copy-pasting code is often seen as a double-edged sword. On one hand, it allows developers to quickly use existing solutions to common problems. On the other hand, it can lead to significant pitfalls:
- Code Smells: Repeated blocks of code can indicate a need for refactoring.
- Unintended Consequences: Copying code without understanding it may introduce bugs in functionality.
- Loss of Context: Transferred code may rely on variables, configurations, or dependencies in the original context that aren't present in the new environment.
The Java Perspective
When it comes to Java, developers must be mindful of several factors before copy-pasting code. This includes understanding class structures, method signatures, and even package dependencies. The aim should always be to reuse code safely and effectively.
Code Transfer Challenges in Java
1. Dependency Management
Java relies heavily on external libraries and packages. When you copy-paste code, you may inadvertently miss necessary imports or external dependencies.
Example
Let’s say you coped this block of code from another class:
import java.util.List;
public void printNames(List<String> names) {
for (String name : names) {
System.out.println(name);
}
}
If the destination file doesn’t already import List
, it will lead to a compilation error. Always check the dependencies before moving the code.
Why It Matters: Ensuring that necessary imports are present keeps your code cleaner and prevents errors.
2. Incorrect Object References
Related to dependencies, copying code can lead to incorrect object references. Suppose the following piece of code is transferred:
public void displayUser() {
System.out.println(user.getName());
}
In your new class, if user
is not defined, you will face a runtime exception. Consistently ensure all variables are properly declared or passed into methods.
Why It Matters: Understanding variable scope and ensuring they are present in your current context can save significant debugging time.
3. Type Compatibility
Java's strong type system can often be overlooked when transferring code snippets. Copying a piece of code that expects specific data types can lead to compatibility issues.
Example
If the original code is:
public void processInt(int number) {
// Processing...
}
If you copy it into a method that attempts to input a String, you'll encounter a compile-time error.
Why It Matters: Type safety is a hallmark of Java. Being cautious about types helps maintain stability in your applications.
Best Practices for Safe Code Transfer
Understanding the above challenges is only part of the solution. Here are some effective practices for mitigating risks while copy-pasting code in Java:
1. Refactor Instead of Copy-Paste
Whenever possible, consider creating reusable methods or classes to encapsulate the code you're tempted to copy. By creating a utility class or incorporating common functionality in an existing class, you can avoid redundancy.
public class StringUtils {
public static String capitalize(String input) {
if (input == null || input.isEmpty()) {
return input;
}
return input.substring(0, 1).toUpperCase() + input.substring(1);
}
}
Why It Matters: Refactoring promotes better organization and reduces the risk of copy-paste mistakes.
2. Use Version Control Effectively
Version control systems like Git are vital for tracking changes in your code. If a copy-pasted section introduces a bug or raises issues, you can revert to previous states easily.
Why It Matters: Version control keeps your development history intact, allowing you to test the code's originality before incorporating it fully.
3. Encapsulate and Document Code
When transferring code, particularly if it's complex, always comment and document it clearly. Provide context for the code's purpose, inputs, and expected outputs.
/**
* This method retrieves user data from the database
* and prints their names.
*
* @param users List of users to print
*/
public void printUserNames(List<User> users) {
for (User user : users) {
System.out.println(user.getName());
}
}
Why It Matters: Clear documentation helps future developers (or even yourself) understand the logic and context, mitigating misunderstanding.
4. Test After Transfer
Once code has been copied and pasted, it's vital that extensive testing occurs. Can the method execute correctly in its new environment? Automated tests can be invaluable for ensuring that existing functionality remains intact.
Why It Matters: Testing helps you catch potential bugs that may arise from context loss or unapproved dependencies.
My Closing Thoughts on the Matter
In the intricate world of Java development, the temptation to copy-paste code can lead to unforeseen complications. Understanding the risks and challenges associated with code transfer allows you to take measures for safer practices. By refactoring, utilizing version control, documenting clearly, and testing your code effectively, you will build a more robust Java application.
For a broader insight into related issues, especially in the realm of JavaScript, check out Challenges Faced in JavaScript Copy-Pasting. Embrace the power of safe code transfer, and elevate the quality of your Java projects! Happy coding!
Checkout our other articles