Mastering Java: Avoiding Copy-Paste Pitfalls in JavaScript
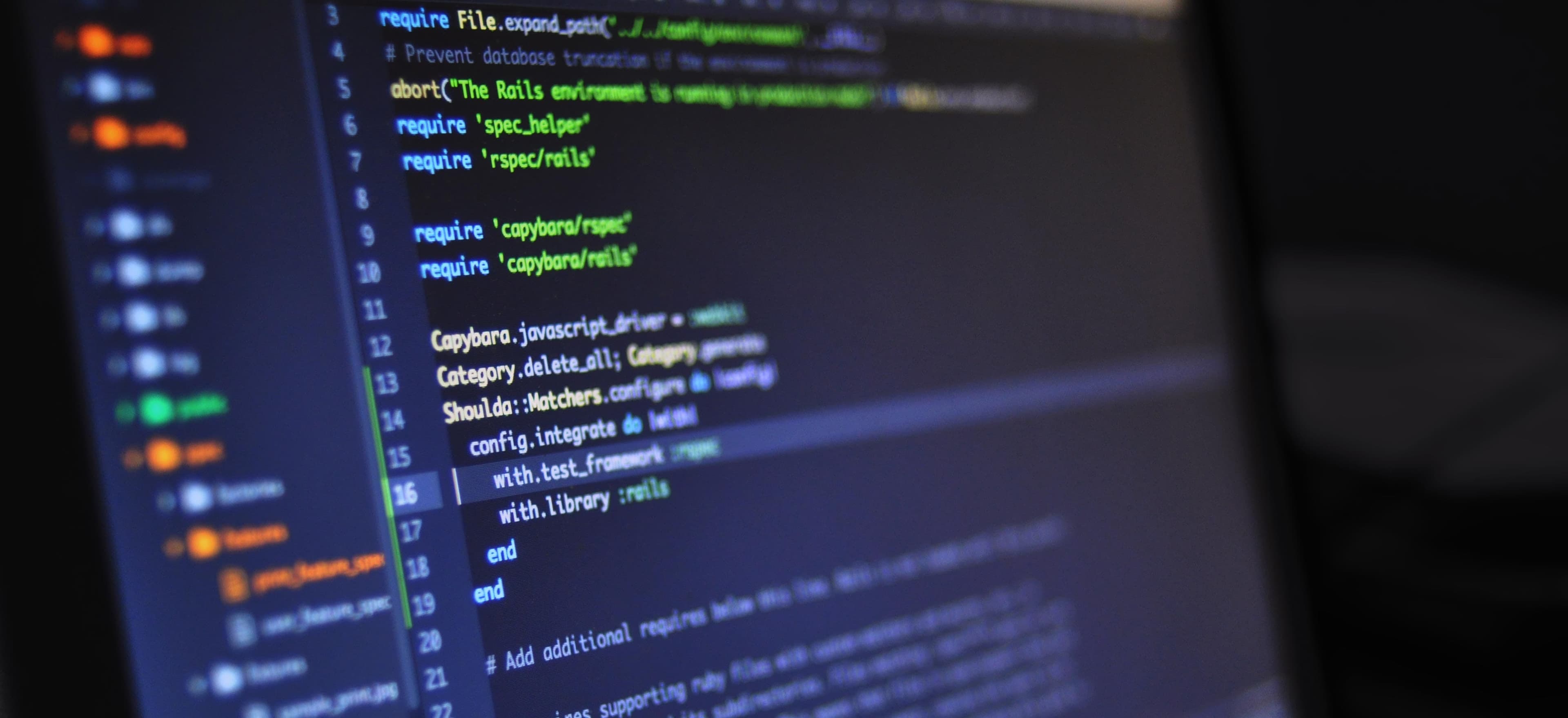
- Published on
Mastering Java: Avoiding Copy-Paste Pitfalls in JavaScript
In the vibrant world of coding, efficiency often tempts us to take shortcuts. While copy-pasting is a common practice among developers, it can lead to numerous challenges and pitfalls. This is especially true in JavaScript, as highlighted in the article "Challenges Faced in JavaScript Copy-Pasting". In this blog post, we will explore how the lessons learned from JavaScript copy-pasting can help us write better, more maintainable Java code.
The Copy-Paste Dilemma
Copy-pasting code is a double-edged sword. Developers may see it as a quick way to get things done, but the long-term consequences can be detrimental:
-
Code Duplication: When the same code is repeated across different parts of a program, it becomes cumbersome to maintain. If a bug is found or a feature needs adjusting, you might have to change it in multiple places.
-
Reduced Readability: Duplication can obfuscate the logic of your code. Other developers (or even future you) may find it challenging to understand why specific sections of code are similar or even identical.
-
Increased Testing Burden: Each instance of the duplicated code needs to be tested independently, leading to higher chances of overlooking bugs.
This is where the principles of clean coding come into play, showing us how to avoid the pitfalls of copy-pasting—lessons that can be translated directly from JavaScript to Java.
Understanding Java's Perspective
Java is a strongly typed language that promotes principles like DRY (Don't Repeat Yourself) and KISS (Keep It Simple, Stupid). Let’s learn how to leverage these principles while coding in Java.
1. Use Functions to Promote Reusability
In Java, functions (or methods) encourage you to encapsulate behavior, making it reusable. By avoiding duplication, your code becomes efficient and easier to manage. Consider the following example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
// Usage
public class Main {
public static void main(String[] args) {
int sum = MathUtils.add(5, 10);
System.out.println("Sum: " + sum);
}
}
Why this works: The MathUtils
class provides a clear, reusable method for adding integers. If you need to change the addition logic, you only update it once.
2. Leverage Inheritance
Object-oriented programming is one of Java's strong suits. By utilizing inheritance, you can create a structure where common functionality is inherited from a parent class.
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
// Usage
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat(); // Inherited method
dog.bark(); // Dog's specific behavior
}
}
Why this works: In this code, the Dog
class inherits the behavior of the Animal
class, promoting code reuse without duplicating logic.
3. Use Interfaces for Flexibility
Interfaces can enable you to define a contract that different classes can implement. This method helps in avoiding duplication while allowing flexibility.
interface Vehicle {
void start();
}
class Car implements Vehicle {
public void start() {
System.out.println("Car starting...");
}
}
class Bike implements Vehicle {
public void start() {
System.out.println("Bike starting...");
}
}
// Usage
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.start(); // Car starting...
Vehicle myBike = new Bike();
myBike.start(); // Bike starting...
}
}
Why this works: Here, Vehicle
defines a common functionality, and both Car
and Bike
provide their specific implementations. This allows for seamless interchangeability without duplicating the start
method logic.
The Importance of Code Reviews
Before you hit the save button, consider implementing a code review process. Peer reviews can catch instances of potential copy-pasting and help maintain a high coding standard.
- Fresh Eyes: A second pair of eyes can see redundancies you might miss.
- Best Practices: Reviews can ensure adherence to the coding standards and principles of DRY and KISS.
The Role of Testing
Automated tests are invaluable against duplication. They ensure that even if the same logic appears in multiple spots, bugs can be quickly identified and patched. When writing tests, consider the following practices:
- Unit Tests: Target individual parts of your code to ensure they work as intended.
- Integration Tests: Check how different parts of your application work together.
Using a popular testing library like JUnit can make your life easier:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class MathUtilsTest {
@Test
void testAdd() {
assertEquals(15, MathUtils.add(5, 10));
}
}
Why this works: The test not only validates the functionality of your method but allows you to refactor confidently. If changes introduce bugs later, the tests will quickly reveal them.
The Closing Argument
Copy-pasting may be tempting in the fast-paced world of software development. However, the practices learned from the challenges of copy-pasting in JavaScript help reinforce how to write efficient and maintainable code in Java. By encapsulating functionality within methods, utilizing inheritance, implementing interfaces, conducting code reviews, and writing comprehensive tests, developers can embrace the principles of clean coding.
For a deeper look into the pitfalls encountered during code copy-pasting and methods to mitigate those issues, refer to the article "Challenges Faced in JavaScript Copy-Pasting".
Embrace best practices and become a master of Java. Happy coding!
Checkout our other articles