Java vs. JavaScript: Copy-Paste Errors Unpacked
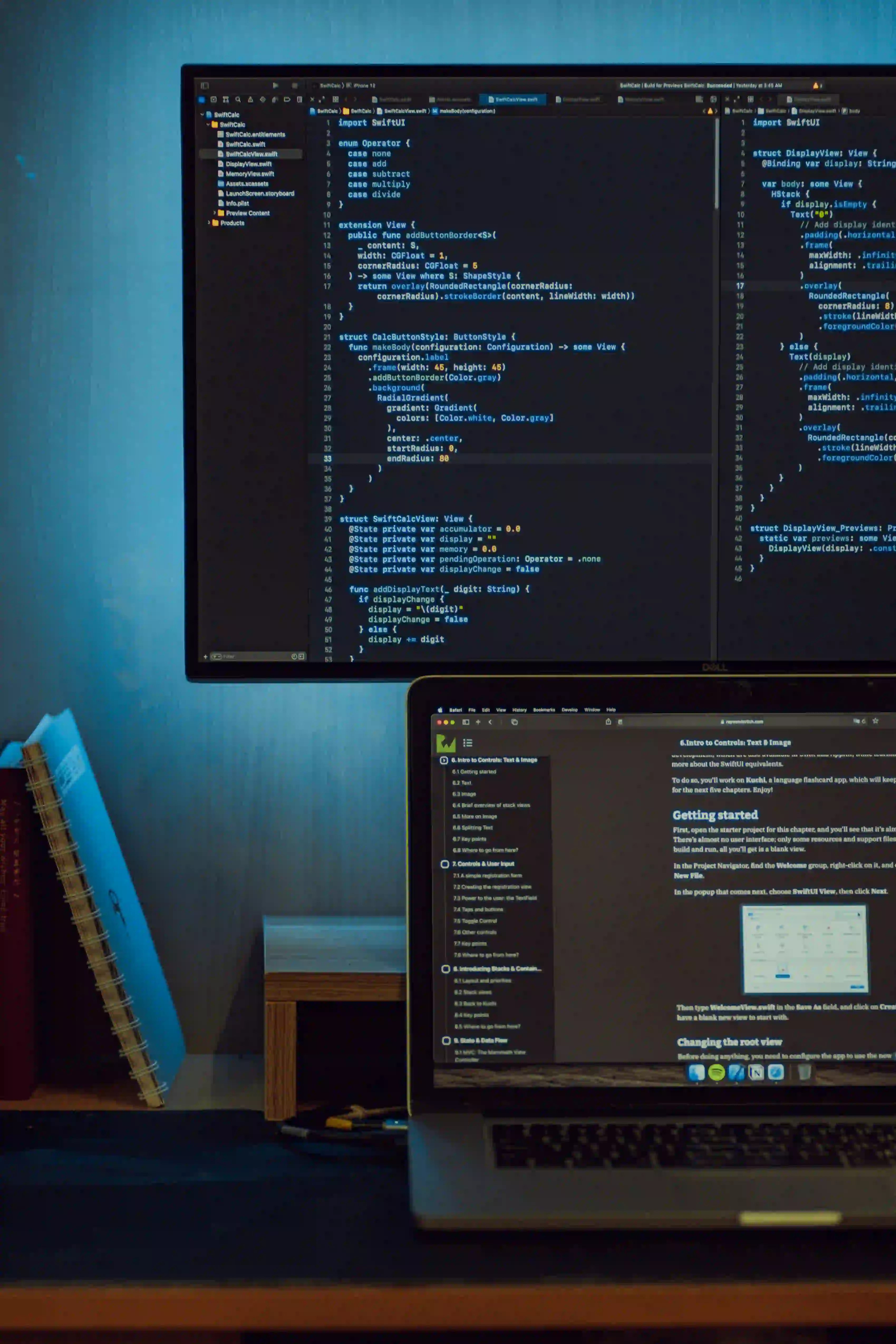
Java vs. JavaScript: Copy-Paste Errors Unpacked
When developers engage with programming languages, they often encounter peculiarities and intricacies that can lead to unexpected challenges. Among these challenges, copy-paste errors are often at the forefront. Specifically, Java and JavaScript can present unique issues when code is replicated across projects. But what are these challenges and how do they differ between the two languages?
This article will delve into the various copy-paste pitfalls you might encounter when working with Java and JavaScript. We will explore why these errors occur, how to mitigate them, and gain a clearer understanding of their different ecosystems. Additionally, we will touch upon insights from the existing article, Challenges Faced in JavaScript Copy-Pasting.
Understanding the Basics: Java and JavaScript
Before diving into copy-paste issues, let’s lay the groundwork by briefly discussing both languages.
Java
Java is a statically typed, object-oriented programming language widely used for building platform-independent applications. It runs on the Java Virtual Machine (JVM), which allows developers to write code once and run it anywhere. This makes it a robust choice for enterprise-level applications.
JavaScript
JavaScript, on the other hand, is a dynamic, prototype-based scripting language primarily used for web development. It runs in the browser, allowing developers to create interactive and dynamic web pages. Unlike Java, it is loosely typed, which leads to a different experience when it comes to code reuse and adaptability.
The Copy-Paste Culture
Copying and pasting code has become a staple practice among developers seeking quick solutions or trying to replicate successes. However, this method often leads to errors, especially if the context of the code is not regarded.
Copy-Paste Errors in Java
Java developers face unique challenges when copying and pasting code. Here are a few frequent issues:
1. Type Safety
Java's static typing system means that type checking is done at compile-time. This can lead to errors if different sections of your copied code utilize incompatible types.
public class Example {
public static void main(String[] args) {
int number = 10;
String str = "This is number: " + number; // Implicitly converts 'number' to String
System.out.println(str);
// Example of a potential copy-paste error:
String invalidOperation = str + 10; // No compile-time error, but can lead to confusion
}
}
Why is this important? Understanding type safety can help prevent logical errors during the execution of your program. Knowing when and where to cast types explicitly helps maintain code clarity and stability.
2. Scope and Context
Java has a strict scope and context management for its variables, which can be problematic when snippets of code are copied without fully understanding their scope.
public class ScopeExample {
public void display() {
String message = "Hello, Java!";
// Using a nested loop example
for (int i = 0; i < 5; i++) {
// Copy-pasting this snippet might result in an error if 'message'
// does not exist within the new context where it's copied
System.out.println(message);
}
}
}
In Context: When reusing code, always ensure that the requisite variables and methods are present in the new context. Always test copied snippets extensively.
3. Exception Handling
Java requires detailed error handling through try-catch blocks. When copying code, if exception handling is overlooked, it may lead to uncatchable run-time exceptions.
public class ExceptionExample {
public static void main(String[] args) {
String str = "Not a number";
// This will generate a run-time error if left unhandled
try {
int number = Integer.parseInt(str);
} catch (NumberFormatException e) {
System.out.println("Invalid number format: " + e.getMessage());
}
}
}
Key Point: Always scrutinize exception handling when copying code. Unhandled exceptions can lead to program crashes and costly downtime.
Copy-Paste Errors in JavaScript
Now let’s shift our focus to JavaScript, where the dynamics are a bit more fluid.
1. Dynamic Typing
JavaScript’s loosely typed nature can lead to unexpected results when copying and pasting variables.
let num = 5;
// Copy-pasting this variable might lead to unexpected pitfalls
let result = num + " is a number";
console.log(result); // Output: "5 is a number"
// If later changed to parseInt
result = parseInt(result);
console.log(result); // NaN, leading to confusion
Why Watch Out? JavaScript can implicitly convert types, resulting in unexpected behavior. Always confirm variable types, especially when copying code.
2. Function Context
JavaScript functions have their own execution context, leading to scoping issues if code is copied from one context to another, particularly in the use of this
.
const person = {
name: 'John',
greet: function () {
console.log(`Hello, I am ${this.name}`);
}
};
const greetCopy = person.greet;
// The context is lost when called like this
greetCopy(); // Output: Hello, I am undefined
The Takeaway: Always consider function context when copying methods. Use .bind()
, .call()
, or .apply()
to set correct execution contexts.
3. Asynchronous Behavior
In JavaScript, asynchronous operations can be mismanaged when copying callback functions, leading to unpredictable behavior.
setTimeout(() => {
console.log("Executed after 1 second");
}, 1000);
// Copying this into another context without properly managing timing can lead to logical flaws.
Important Note: Ensure that async handling and dependencies are understood when copying asynchronous code. Always document and thoroughly test copied snippets.
Best Practices for Avoiding Copy-Paste Errors
Whether you are working in Java or JavaScript, avoiding copy-paste errors can save you significant time and headaches. Here are some best practices:
-
Understand the Code: Before copying any code, take time to understand how it works and the context surrounding it.
-
Refactoring: Rewrite or refactor code instead of copy-pasting. This helps reinforce understanding.
-
Use Version Control: Tools like Git can help keep track of changes, making it easier to manage code snippets.
-
Comment and Document: Leave comprehensive comments when copying code. This will help you or others grasp why certain decisions were made.
-
Leverage Unit Tests: Writing unit tests can help catch issues stemming from copied code before deployment.
The Closing Argument
Copy-pasting code—from Java to JavaScript—can save time but also introduces a host of potential errors. By understanding the intricacies of each language and applying best practices, you can significantly reduce the risk of encountering copy-paste errors.
For additional insights into the challenges faced by JavaScript developers when copy-pasting code, consult the article Challenges Faced in JavaScript Copy-Pasting.
By being mindful of the differences and similarities between Java and JavaScript, and applying clear frameworks for writing quality code, you can ensure a smoother development experience. Whether you're building enterprise applications in Java or interactive web pages with JavaScript, informed coding practices will pay dividends in the long run.