Troubleshooting JavaFX Layout Issues: Anomaly Detection Guide
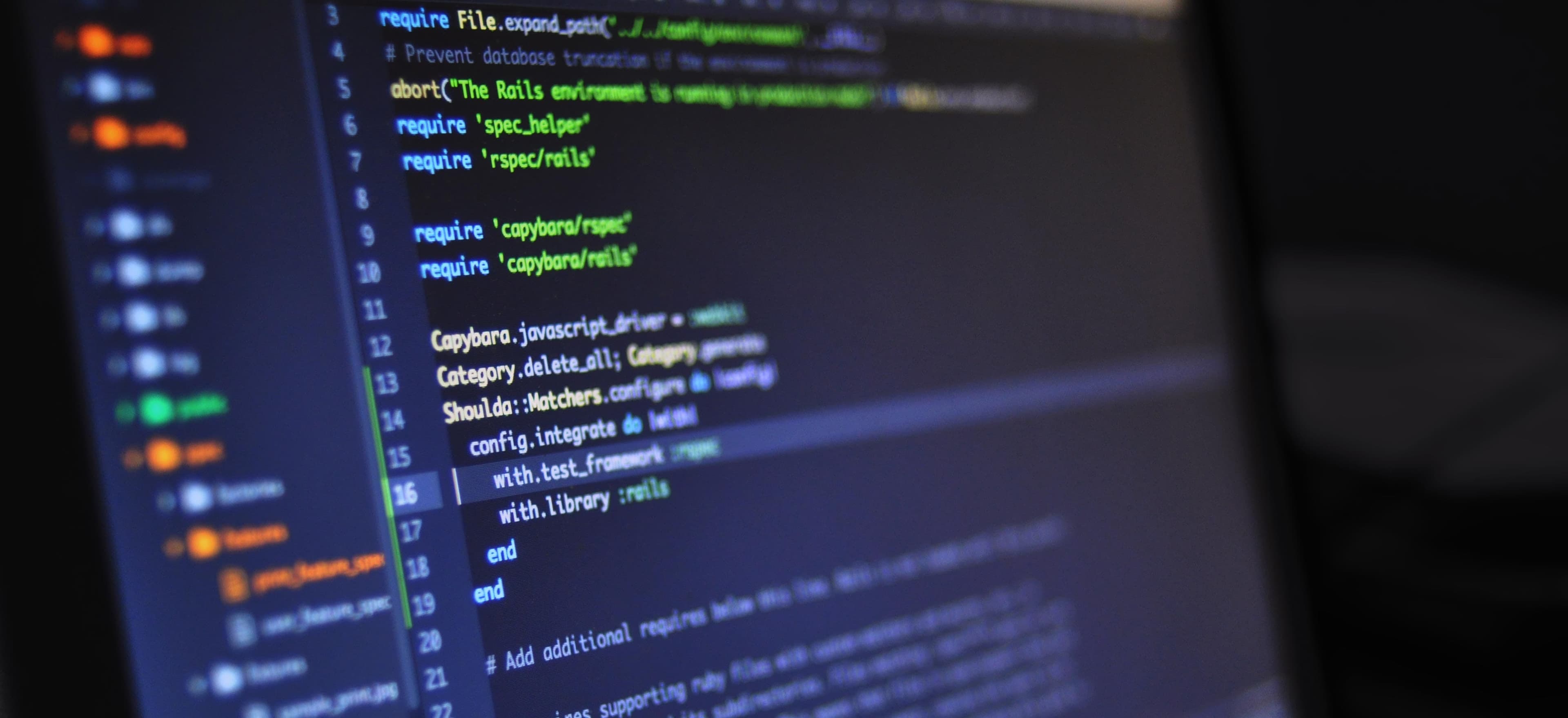
- Published on
Troubleshooting JavaFX Layout Issues: Anomaly Detection Guide
JavaFX is a powerful and versatile framework for building rich desktop applications in Java. While it offers many capabilities for creating aesthetically pleasing UIs, it is not without its challenges. Particularly, developers often encounter layout issues that can be frustrating and time-consuming to resolve. In this guide, we will explore how to troubleshoot common layout problems in JavaFX, incorporating techniques for anomaly detection and best practices that can help you build better applications.
Understanding JavaFX Layouts
JavaFX provides a variety of layout containers designed to manage the placement and sizing of UI components. These containers include:
- VBox: Arranges its children in a vertical column.
- HBox: Arranges its children in a horizontal row.
- BorderPane: Divides the layout into five regions (top, bottom, left, right, and center).
- GridPane: Allows for a flexible grid layout.
Example: Using VBox and HBox
VBox vbox = new VBox();
HBox hbox = new HBox();
// Adding buttons to the containers
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
hbox.getChildren().addAll(button1, button2);
Label label = new Label("This is a VBox");
vbox.getChildren().addAll(label, hbox);
// Setting properties
vbox.setSpacing(10);
hbox.setSpacing(5);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
In this example, we create a vertical box (VBox
) containing a label and a horizontal box (HBox
). The setSpacing
method is used to add space between elements. This ability to control spacing is one of the reasons why JavaFX layouts are flexible and powerful.
Common Layout Anomalies in JavaFX
Despite the robust features of JavaFX, layout anomalies can arise due to various reasons. Here are some common issues:
-
Overlapping UI Elements: Often occurs when the layout manager does not allocate sufficient space for components.
-
Unintended Size Behavior: Components may not resize as expected, either resulting in truncated content or excessive whitespace.
-
Invisible Elements: Elements can sometimes appear missing when not added to the scene graph properly or due to layout constraints.
-
Responsive Design Problems: With varying screen sizes, components may not adapt correctly to larger or smaller displays.
Steps for Anomaly Detection
To effectively troubleshoot layout issues, consider the following steps:
Step 1: Inspect the Scene Graph
The scene graph is the internal representation of your UI components. Use the JavaFX Scene Builder or logging to inspect your components.
System.out.println(getChildren());
This will help you identify any elements that might be missing or misconfigured.
Step 2: Check Layout Properties
Each layout pane has unique properties. Check for correct settings like padding, spacing, and alignment.
VBox vbox = new VBox();
vbox.setPadding(new Insets(10)); // Ensure proper padding
Using appropriate padding ensures that components are visually separated from each other and the edges of the layout container.
Step 3: Debugging Component Sizes
Pay attention to how you're using size properties such as minWidth
, maxWidth
, and prefWidth
.
button.setPrefWidth(200);
Setting preferred sizes can mitigate unexpected behavior, especially when combined with other layout properties.
Step 4: Responsive Design Considerations
For responsive layouts, consider using percentage-based widths or even CSS styles to handle varying screen sizes.
.button {
-fx-pref-width: 50%;
}
Using CSS allows for cleaner and more maintainable code.
Highlighting Best Practices
To avoid layout issues from the start, adhere to the following best practices:
-
Use FXML: Consider defining your UI layout in FXML, which can simplify maintenance and enable separation of concerns.
-
Leverage CSS: Apply styling through CSS to avoid embedded styling in your Java code.
-
Thorough Testing: Test your application on different screen resolutions and sizes to identify potential layout issues early.
-
Modular Components: Break your UI into smaller, reusable components to facilitate easier debugging and reusability.
Use Case: Analyzing a Layout Issue
Let’s say you're facing overlapping buttons in a VBox
. The text you see in the application differs from what you expect. Here’s how to approach it:
-
Review the
VBox
layout and ensure there are no conflicting styles or elements added that would affect spacing. -
Inspect the properties of the buttons to ensure each has defined preferred or minimum sizes.
-
Test with different layouts to see if the issue persists across different containers.
Here’s an example code snippet that highlights potential spacing errors:
VBox vbox = new VBox();
Button overlapButton1 = new Button("Overlapping 1");
Button overlapButton2 = new Button("Overlapping 2");
vbox.getChildren().addAll(overlapButton1, overlapButton2);
// If the buttons overlap, adjust spacing
vbox.setSpacing(20); // Improving the spacing can remedy this effect
In this scenario, correctly setting the spacing can resolve overlap issues.
In Conclusion, Here is What Matters
Troubleshooting layout anomalies in JavaFX can be challenging, but armed with the right knowledge and techniques, you can effectively manage and resolve these issues. By following the steps outlined in this guide, implementing best practices, and understanding the behaviors of JavaFX layout containers, you can significantly improve the quality and usability of your UI.
For those further interested in related topics, check out my other article titled Spotting Anomalies: Fixing Layout Elements Gone Rogue for additional insights on managing visual issues in your applications. Happy coding!
Checkout our other articles