Maximize Java Performance: Tackle API Gateway Caching Issues
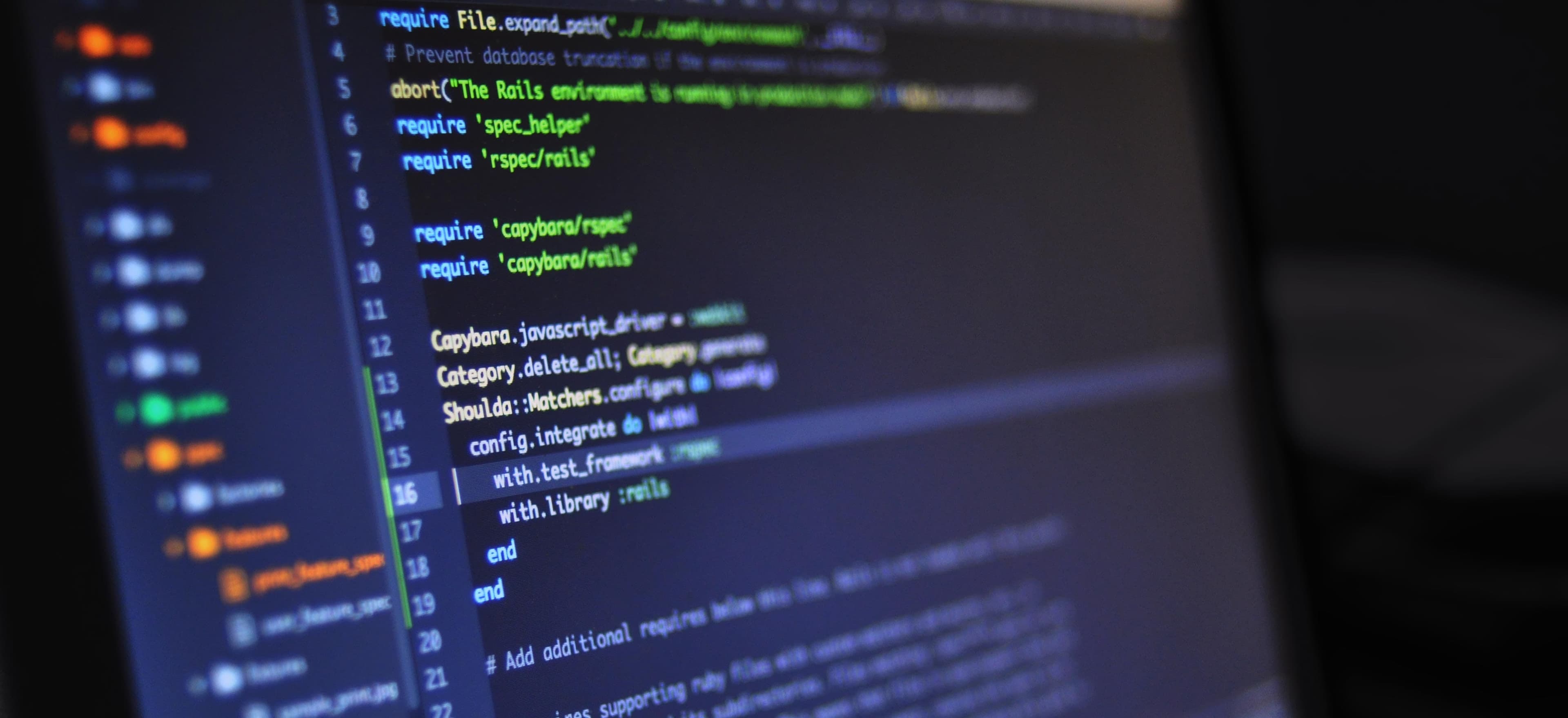
- Published on
Maximize Java Performance: Tackle API Gateway Caching Issues
As developers, we are often challenged by the intricate balance of performance and efficiency. One of the most complex areas to navigate is API gateway caching, especially when working within a Java ecosystem. In this article, we will not only shore up our understanding of API gateway caching but also explore practical solutions to help maximize your Java applications' performance.
Caching, when implemented correctly, can drastically reduce the load on your servers, decrease response times, and provide a smoother experience for your users. However, when it goes wrong, it can lead to inconsistencies, stale data, or even bottlenecking your application.
Understanding API Gateway Caching
API gateway caching allows a gateway to store responses from your services. This reduces the need to make repeated calls to backend services for the same data. The gateway can deliver cached responses much faster than fetching fresh data, thereby enhancing user experience.
Why Cache?
-
Speed: Cached data can be served much faster than a round trip to a database or another service.
-
Reduced Server Load: By caching frequent requests, you lessen the number of calls made to your backend servers.
-
Scalability: Effective caching makes it easier to support a growing number of requests without the corresponding increase in infrastructure.
Drawbacks of Incorrect Caching
-
Stale Data: If the cache isn't invalidated or updated properly, users can receive outdated information.
-
Bottlenecks: Over-reliance on caching can lead to issues if the cache storage is not optimized.
-
Complexity: Introducing caching adds another layer of complexity that can lead to bugs if not managed correctly.
Recommended Caching Strategies
Before diving into code snippets and technical setups, it's critical to choose an appropriate caching strategy. Here are three common approaches:
-
In-Memory Caching: This involves storing data temporarily in memory for fast retrieval, often using libraries like Caffeine or Ehcache in Java.
-
Distributed Caching: For larger applications, using a distributed cache like Redis or Hazelcast can be invaluable for scalability.
-
HTTP Caching: Utilizing caching headers can offer a simpler method of caching HTTP responses.
Example: In-Memory Caching with Caffeine
Using the Caffeine library, we can efficiently cache responses with a simple configuration. Here’s a basic example to illustrate this concept:
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Cache;
public class CaffeineCacheExample {
private Cache<String, String> cache;
public CaffeineCacheExample() {
// Configure the cache: maximum size and expire after write
this.cache = Caffeine.newBuilder()
.maximumSize(10_000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
}
public String getData(String key) {
// Attempt to retrieve from cache
return cache.get(key, k -> fetchDataFromService(k));
}
private String fetchDataFromService(String key) {
// Simulate fetching data from an external service
return "Data for " + key;
}
}
Why Use Caffeine?
Caffeine improves concurrency and serves high-performance caches by using techniques like adaptive eviction and removal of stale entries. The example above creates a cache that holds a maximum of 10,000 entries, expiring entries after 10 minutes.
Integrating Caching with API Gateway
When integrating caching within an API gateway like AWS API Gateway or Kong, you need to consider how the cache will interact with your backend services.
For instance, with AWS API Gateway you can set caching on methods, with a TTL that fits your data association needs.
Example: Configuring AWS API Gateway for Caching
Navigate to your API in the AWS Management Console and follow these steps:
- Select a method (GET, POST, etc.).
- In the Method Request section, enable caching.
- Specify a caching TTL that represents how fresh you want the data.
- You can also leverage cache invalidation strategies for better results.
This provides better control over what is cached and how long it stays in the cache. In addition, HTTP headers can help manage caching behaviors when responses must not be cached or should be forcibly updated.
Monitoring and Performance Tuning
When implementing caching, it is essential to monitor performance impacts. Key metrics to track include:
- Cache Hit Ratio: Indicates how often the cache is used effectively.
- Latency: Measure how caching reduces response times.
- Server Load: Monitor for resource spikes that could indicate issues with your caching strategy.
You can use tools like Prometheus for monitoring alongside Grafana to visualize your data.
Example of Monitoring Cache Metrics
If you're using Spring Boot, you can easily expose metrics using Spring Boot Actuator:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
In application.properties
, you can expose necessary metrics:
management.endpoints.web.exposure.include=*
This will expose various metrics related to your service, including cache performance. By regularly reviewing these metrics, you can identify areas for improvement or potential new caching strategies.
Closing the Chapter
Caching can dramatically enhance your applications' performance, especially when dealing with API gateways. However, it requires a thoughtful approach to avoid pitfalls such as stale data and unnecessary complexity.
Remember, the right caching strategy depends on the specifics of your application, including traffic patterns and data freshness requirements. For deeper insights and excellent solutions to caching-related issues, check out this article.
Implement caching wisely, monitor performance diligently, and you’re well on your way to maximizing Java application performance!
Additional Resources
By leveraging the right tools and techniques, you can ensure that your Java applications perform at their best, providing users with a fast and efficient experience. Happy coding!
Checkout our other articles