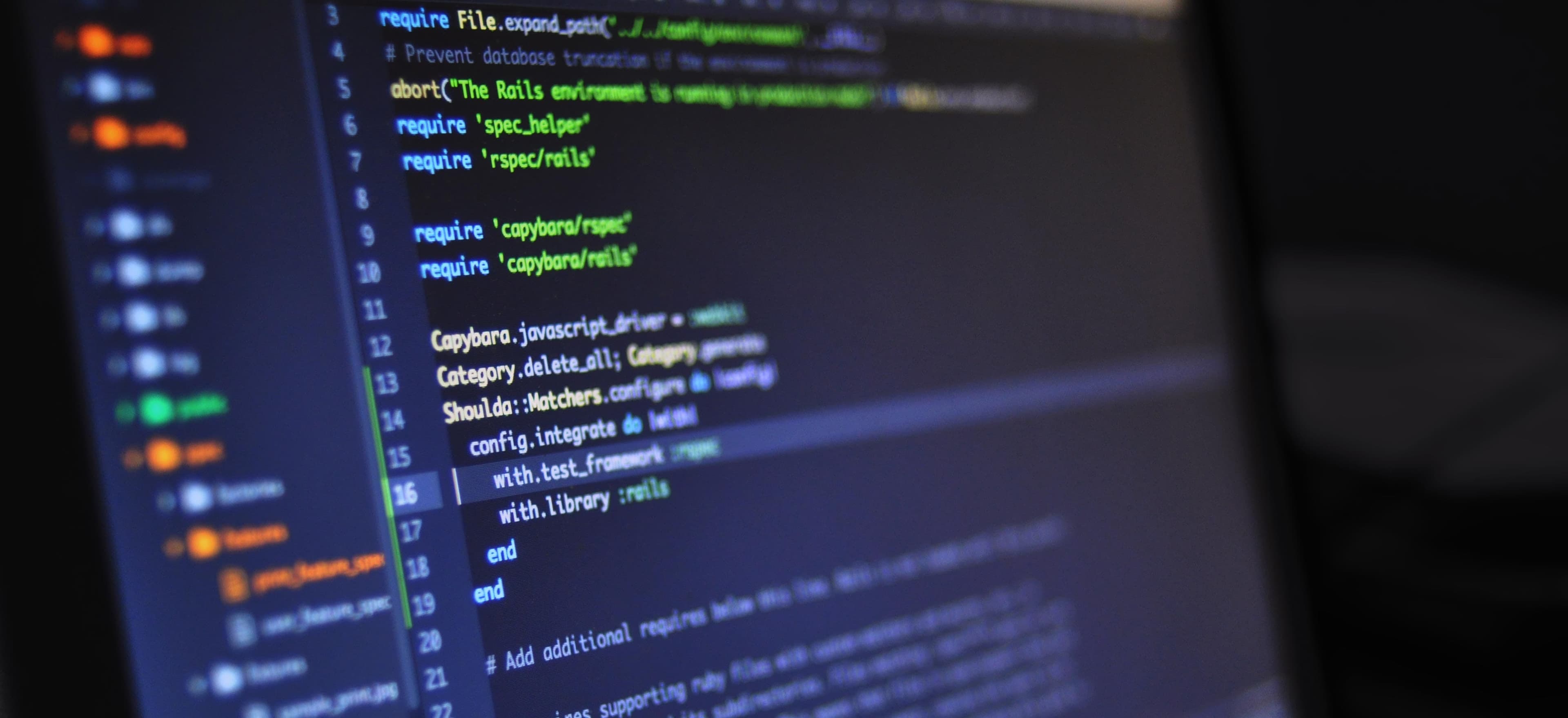
- Published on
Managing Session State in Java Web Apps with Cookies
In the world of web development, managing session state is crucial for creating interactive and user-friendly applications. This is especially true for web applications built using Java technologies. While there are several ways to manage session state, cookies remain one of the simplest and most effective solutions. In this blog post, we'll explore how to manage session state in Java web apps with cookies, providing you with practical code snippets and insights along the way.
What are Cookies?
Cookies are small pieces of data that a server sends to the user's web browser. The browser may store these cookies and send them back with subsequent requests to the same server. Cookies can be used for various purposes, including session management, personalization, and tracking.
Cookies are particularly useful in managing session state because they allow the server to store user information in a persistent manner. This way, web applications can remember user preferences, authentication details, and more, even after the user has navigated away or closed their browser.
Why Use Cookies for Session State?
Using cookies for session management in Java web apps has several advantages:
- Persistence: Cookies can have an expiration date, allowing them to persist across sessions.
- Client-side storage: Cookies are stored on the client’s machine, reducing server-side storage overhead.
- Simplicity: Implementing cookies is straightforward and PHP developers can easily manipulate them.
However, it’s essential to balance cookie use with security, as cookies can be manipulated by users if not handled properly.
Setting Up Cookies in Java
Java provides the javax.servlet.http.Cookie
class to work with cookies easily. Below is a step-by-step guide to managing cookies for session state in Java web applications.
Step 1: Creating a Cookie
When a user logs in, we can create a cookie to store their session ID or relevant information. An example code snippet is:
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
// Function to create a login cookie
public void createLoginCookie(HttpServletRequest request, HttpServletResponse response, String sessionId) {
Cookie cookie = new Cookie("sessionId", sessionId); // Create a cookie with the name 'sessionId'
cookie.setMaxAge(60 * 60); // Set to expire in 1 hour
cookie.setPath("/"); // Accessible on the whole application
response.addCookie(cookie); // Add the cookie to the response
}
Explanation of the Code
- sessionId: This represents a unique identifier for the user's session.
- setMaxAge: This method specifies how long the cookie should live. In this case, it's set for 1 hour (3600 seconds).
- setPath: By setting the path to "/", the cookie becomes accessible throughout the entire application.
Step 2: Retrieving a Cookie
To retrieve the session cookie when a user makes subsequent requests, you can use the following code snippet:
// Function to retrieve a session cookie
public String getLoginCookie(HttpServletRequest request) {
Cookie[] cookies = request.getCookies(); // Retrieve all cookies
if (cookies != null) {
for (Cookie cookie : cookies) {
if ("sessionId".equals(cookie.getName())) { // Check for the specific cookie
return cookie.getValue(); // Return the value of the sessionId cookie
}
}
}
return null; // Return null if not found
}
Explanation of the Code
- request.getCookies(): This retrieves all cookies sent with the current request.
- Checking for the sessionId: By iterating over the cookies, we can find our desired cookie by name.
Step 3: Validating and Using the Cookie
After retrieving the session ID, it is crucial to validate it before using it. Validation typically involves checking against a database or session management system.
Example Validation Code
// Function to validate the session ID
public boolean isSessionValid(String sessionId) {
// Assuming you have a method to check session validity
return sessionIdDatabase.contains(sessionId); // Validate against database
}
Explanation of the Code
- sessionIdDatabase: This represents a collection that stores active session IDs. You would typically check against a database or in-memory data structure.
Best Practices for Using Cookies
-
Use Secure and HttpOnly Flags:
- Secure cookies are only transmitted over HTTPS.
- HttpOnly cookies are not accessible via JavaScript, reducing the risk of XSS attacks.
Here's how to set these flags:
cookie.setSecure(true); cookie.setHttpOnly(true);
-
Minimize Cookie Size: Cookies should not be too large. A good rule of thumb is to keep them under 4KB.
-
Manage Expiration Correctly: Ensure cookies have a reasonable expiration time to enhance security while still providing a good user experience.
-
Regularly Rotate Session IDs: This practice limits the risk of session hijacking.
Integrate Cookie Management with Java Applications
Java web frameworks such as Spring and JavaServer Faces (JSF) provide additional support for managing cookies if you're looking for a more streamlined approach. For instance, Spring's @CookieValue
annotation simplifies cookie retrieval.
My Closing Thoughts on the Matter
Cookies are an essential part of managing session state in Java web applications. By implementing session management through cookies, you can provide a more personalized experience for your users while maintaining performance and security.
For a more detailed exploration of cookies, refer to the article Handling Cookie Persistence in jQuery UI Tabs.
By following best practices and implementing the techniques shared in this article, you can ensure robust session state management in your Java applications.
Feel free to share your thoughts and experiences in managing session states using cookies in the comments below!
Checkout our other articles