Optimize Your Java Apps with Effective API Gateway Caching
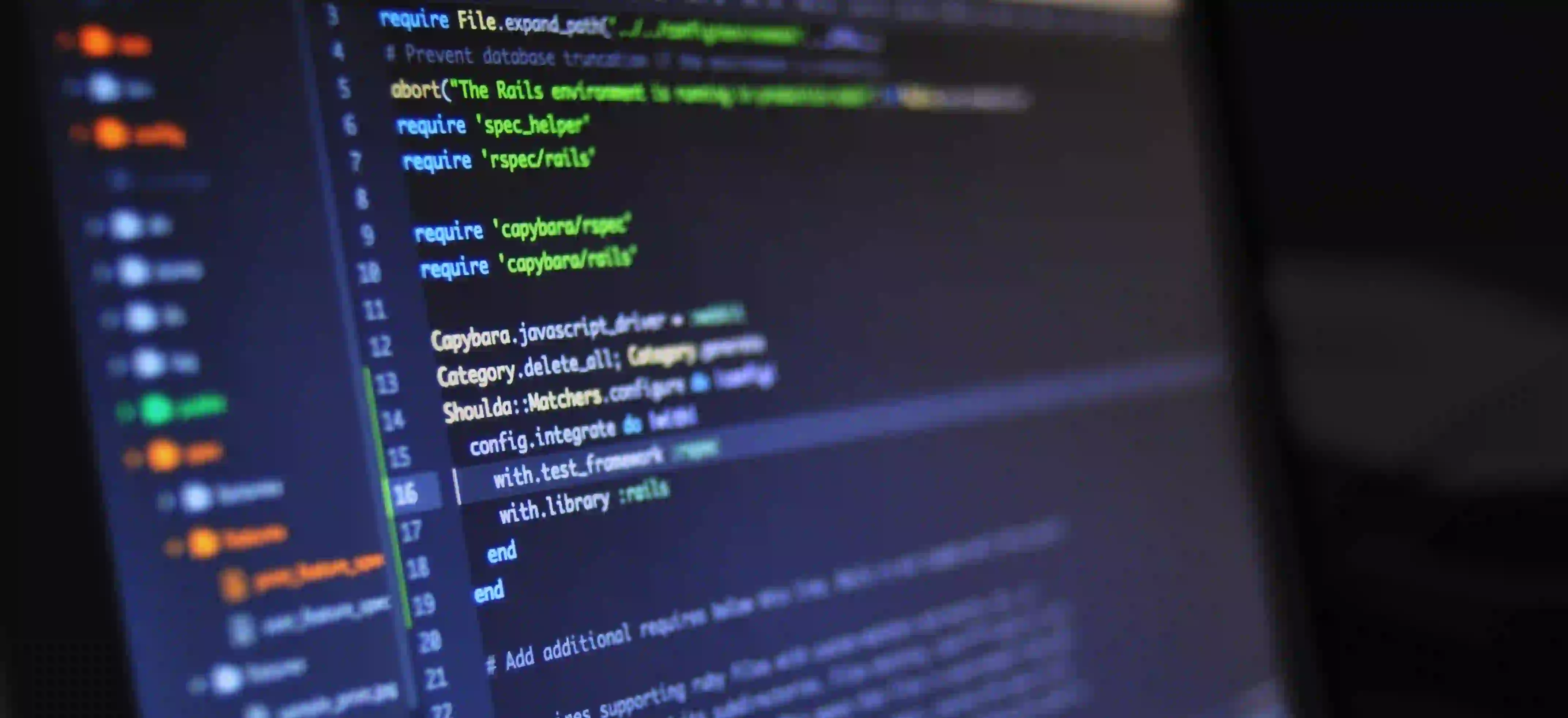
Optimize Your Java Apps with Effective API Gateway Caching
In today's fast-paced digital landscape, performance optimization is important for enhancing user experience. If you're developing Java applications that interact with APIs, you might have encountered performance bottlenecks. One effective strategy to alleviate these issues is API Gateway Caching.
This blog post will delve into what API Gateway Caching is, why it’s crucial for Java applications, and how to implement it effectively. We’ll also reference a previous article titled Struggling with API Gateway Caching? Here's Your Fix!, which provides additional context for those looking for in-depth strategies.
Understanding API Gateway Caching
API Gateway Caching refers to the technique of storing responses from API requests temporarily. By caching responses, applications can bypass repetitive requests to the backend systems, resulting in reduced latency and improved performance. This practice is especially beneficial for reducing the load on APIs, which can frequently be overwhelmed by multiple identical requests.
Why Cache?
-
Improved Performance: By caching frequently accessed data, you can significantly reduce response times and enhance user experience. Quick responses are vital for retaining users and ensuring satisfaction.
-
Reduced Load on Servers: Caching minimizes unnecessary calls to backend services. This reduction in traffic can lower operational costs and prevent outages due to high traffic.
-
Cost Savings: Reducing the number of requests made to your backend APIs can lead to notable cost savings, especially if you are operating on cloud services where API usage is billed.
How to Implement API Gateway Caching in Java
Implementing API Gateway Caching in your Java application involves several steps. Let's break it down with clear examples.
Step 1: Choose the Right API Gateway
Select a suitable API Gateway that supports caching. Some popular options include:
- AWS API Gateway: Provides built-in caching capabilities.
- Spring Cloud Gateway: Useful for applications using Spring Boot.
- Kong: An open-source gateway for cloud-native applications.
Step 2: Configure Caching
Using AWS API Gateway as an example, we'll look at how to enable caching.
Example: Enabling Caching in AWS API Gateway
import com.amazonaws.services.apigateway.AmazonApiGateway;
import com.amazonaws.services.apigateway.AmazonApiGatewayClientBuilder;
import com.amazonaws.services.apigateway.model.UpdateStageRequest;
public class ApiGatewayCaching {
public static void enableCaching(String apiId, String stageName) {
AmazonApiGateway apiGateway = AmazonApiGatewayClientBuilder.defaultClient();
// Configure caching
UpdateStageRequest updateStageRequest = new UpdateStageRequest()
.withRestApiId(apiId)
.withStageName(stageName)
.addMethodSettingsEntry(new MethodSetting()
.withHttpMethod("GET")
.withResourceId("yourResourceId")
.withCachingEnabled(true) // Enable caching for this method
);
// Update the stage
apiGateway.updateStage(updateStageRequest);
}
}
Commentary: In this code snippet, we create an instance of the AmazonApiGateway
and configure the method settings to enable caching for a GET request. This approach ensures that repeated calls to the specified API method return cached responses.
Step 3: Define Cache Settings
Each API Gateway allows granular control over cache settings. Here are key settings you can customize:
- Cache TTL (Time to Live): Sets how long responses are cached.
- Cache Key: Customize the key used to store the cache, e.g., using query parameters or headers.
Selecting the right TTL is crucial. A longer TTL can speed up the response time but may lead to stale data. Conversely, a shorter TTL ensures freshness but may lead to overhead from repeated requests.
Example of Setting Cache TTL
// Example retrieval and setting cache TTL
public void configureCacheSettings(String apiId, String stageName, int ttl) {
// Assuming 'apiGateway' is already defined like the previous example
MethodSetting methodSetting = new MethodSetting()
.withHttpMethod("GET")
.withCachingEnabled(true)
.withCacheTtl(ttl) // Set the TTL as specified
.withResourceId("resourceId");
UpdateStageRequest updateStageRequest = new UpdateStageRequest()
.withRestApiId(apiId)
.withStageName(stageName)
.withMethodSettings(methodSetting);
apiGateway.updateStage(updateStageRequest);
}
Commentary: Here, we define a method to set the cache TTL for the configured API. The management of cache settings ensures that your application remains both fast and responsive.
Step 4: Test Caching
Testing is crucial. Validate whether caching works effectively:
- Simulate Load: Use tools like Apache JMeter to simulate repeated API calls and gauge the performance.
- Analyze Response Times: Monitor how caching impacts response times before and after implementation.
Step 5: Monitor and Optimize
After implementation, continuously monitor your application performance. Pay attention to:
- Cache Hit Ratio: This indicates how often requests are served from the cache versus the backend. Aim for a higher ratio.
- Response Time Trends: Observe changes in response times to ensure the caching strategy is yielding desired results.
Common Pitfalls to Avoid
-
Over-Caching: Caching too much data can lead to stale responses. Use appropriate cache invalidation strategies.
-
Ignoring TTL Misconfigurations: Incorrect TTL configurations can lead to either stale data or excessive backend calls. Monitor and adjust as necessary.
-
Lack of Analytics: Without tracking performance metrics, optimizing your caching strategy becomes a matter of guesswork. Implement logging and analytics to foster data-driven decisions.
Wrapping Up
API Gateway Caching is a powerful optimization technique for Java applications. By storing frequent responses temporarily, you can significantly enhance performance, reduce server load, and save costs.
For additional insights and tips on effectively managing API Gateway Caching, check out the article titled Struggling with API Gateway Caching? Here's Your Fix!.
By following the steps outlined in this post, you will be well on your way to optimizing your Java applications dramatically. As APIs continue to evolve, adopting effective caching strategies will be imperative for maintaining high application performance in a competitive landscape.
Finally, remember that optimization is an ongoing process. Stay current with best practices, monitor your metrics, and iterate on your solutions for the best results.
Happy coding!