Java Solutions for Seamless Integration with jQuery GalleryView
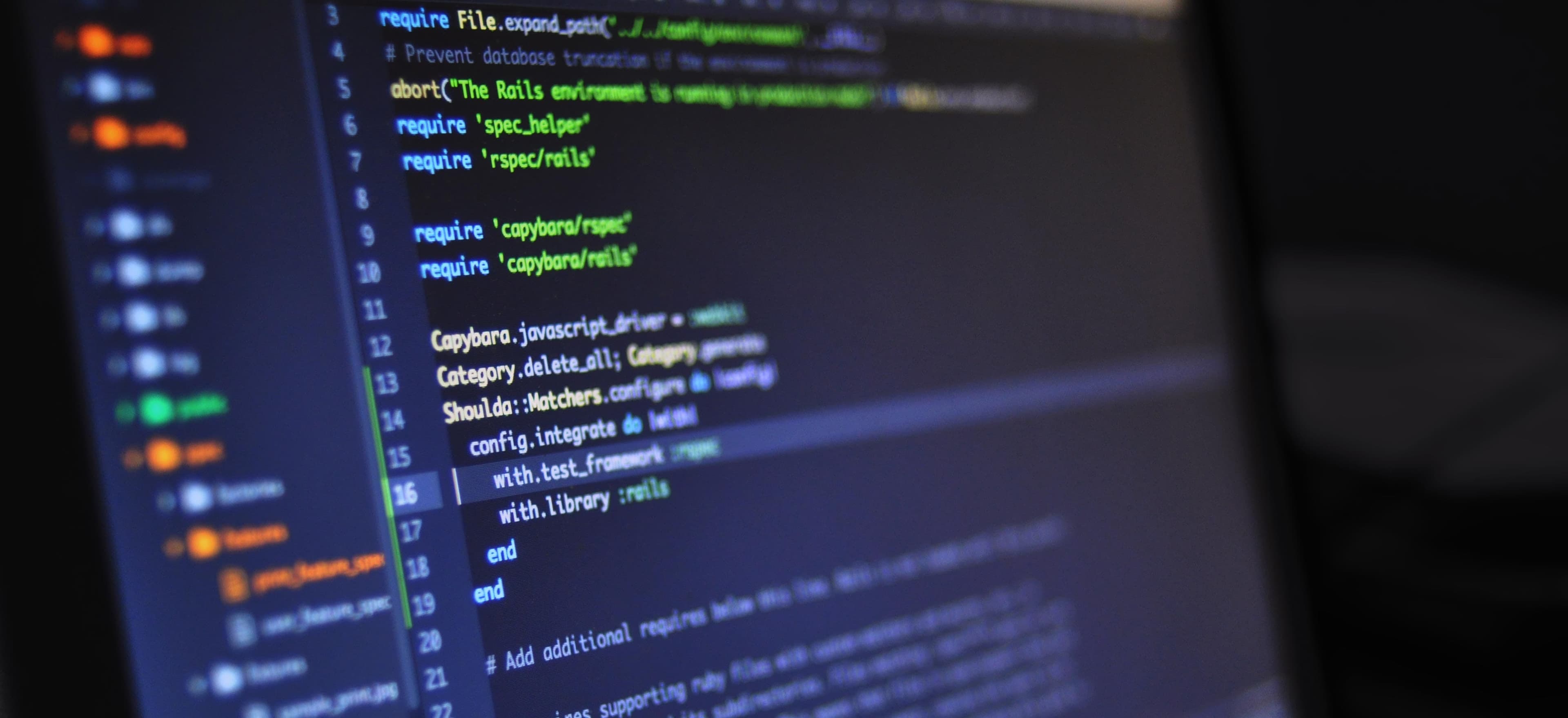
- Published on
Java Solutions for Seamless Integration with jQuery GalleryView
In today's web applications, the synergy between front-end technologies like jQuery and back-end solutions such as Java can be transformative. Front-end libraries streamline user experiences, while robust back-end frameworks ensure data management and business logic functionality. One of the most common integrations involves the jQuery GalleryView plugin, which enhances image display and interaction. In this blog post, we will explore how to seamlessly integrate Java with jQuery GalleryView, tackling common challenges and providing valuable Java solutions.
Understanding the Java and jQuery Dynamic
Java is a powerful, object-oriented programming language that is widely used for building enterprise-level applications. On the other hand, jQuery is a fast and concise JavaScript library that simplifies HTML document traversing, event handling, and animations for interactive designs.
Integrating Java with jQuery involves creating a smooth connection between server-side programming (Java) and client-side scripting (jQuery). This integration often requires AJAX (Asynchronous JavaScript and XML) to facilitate communication without disrupting the user experience.
Overview of jQuery GalleryView
Before diving into integration techniques, let's briefly discuss what jQuery GalleryView is all about. The GalleryView plugin allows developers to create an expandable and user-friendly image gallery. Using this plugin, users can smoothly scroll through images, with enhanced functionalities such as thumbnail previews and descriptions.
For a complete guide on solving common jQuery GalleryView issues, check out the existing article "Revive Your Site: Solving jQuery GalleryView Issues" at tech-snags.com/articles/revive-site-solving-jquery-galleryview-issues.
Setting Up the Java Back-End
To build a seamless experience, we need to create a Java-based RESTful API that serves image data to the jQuery GalleryView plugin. Here is a simple example of how to do that.
Step 1: Create a RESTful Endpoint
Start with creating a REST controller. This endpoint will serve image data in JSON format:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("/gallery")
public class GalleryController {
@GetMapping("/images")
public List<Image> getImages() {
List<Image> images = new ArrayList<>();
images.add(new Image("image1.jpg", "Description of image 1"));
images.add(new Image("image2.jpg", "Description of image 2"));
images.add(new Image("image3.jpg", "Description of image 3"));
return images;
}
}
class Image {
private String url;
private String description;
public Image(String url, String description) {
this.url = url;
this.description = description;
}
public String getUrl() {
return url;
}
public String getDescription() {
return description;
}
}
Code Commentary
- @RestController: This annotation is used to define a controller that returns JSON data instead of views.
- @RequestMapping: It specifies the base URL for the API. In this case, it is "/gallery".
- getImages(): This method retrieves a list of images encapsulated in a simple data structure. It constructs a list of Image objects that contain the URL and description of each image.
The method returns this list, which will be serialized to JSON and sent to the client.
Step 2: Configure CORS
To enable your Java application to communicate with the jQuery GalleryView, you need to configure Cross-Origin Resource Sharing (CORS).
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/gallery/**").allowedOrigins("http://localhost:3000");
}
}
Code Commentary
- WebMvcConfigurer: This is an interface to customize the Spring MVC configuration.
- addCorsMappings(): This method specifies which endpoints are accessible from the frontend and which domains are allowed to access them.
Integrating jQuery with the RESTful API
Now that we have set up our Java back-end, let’s integrate it with jQuery. The idea is to fetch images dynamically from the API and display them in the GalleryView plugin.
HTML Setup
Create a basic HTML structure that will house the gallery:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="path-to-galleryview.css">
<title>GalleryView Integration</title>
</head>
<body>
<div id="gallery" class="gallery"></div>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="https://path-to-galleryview.js"></script>
<script src="script.js"></script>
</body>
</html>
JavaScript to Fetch Data
Now, we will use jQuery to fetch the image data from our Java RESTful API and initialize the GalleryView.
$(document).ready(function() {
$.ajax({
url: 'http://localhost:8080/gallery/images',
method: 'GET',
success: function(images) {
images.forEach(image => {
$('#gallery').append(`
<div class="thumbnail">
<img src="${image.url}" alt="${image.description}">
<p>${image.description}</p>
</div>
`);
});
$('#gallery').galleryView(); // Initialize GalleryView
},
error: function(err) {
console.log('Error fetching images:', err);
}
});
});
Code Commentary
- $.ajax(): The core jQuery function for making AJAX requests.
- success: This callback function processes the image data returned from the server, dynamically appending each image to the HTML gallery.
- galleryView(): This function initializes the GalleryView plugin on the populated div.
Common Challenges and Solutions
Managing CORS Issues
As mentioned earlier, CORS can lead to frustrating issues when developing across different hosts. Ensure that your Java application is configured to accept requests from the origin of your frontend host.
Handling Asynchronous Data
AJAX introduces asynchronicity, meaning the user may try to interact with the UI before data is loaded. Consider implementing loading indicators to inform users when data is fetching.
Error Handling
While implementing the AJAX call, be sure to include error handling to capture any issues that arise during the request, such as network interruptions or server errors.
My Closing Thoughts on the Matter
Integrating Java with jQuery GalleryView can elevate the user experience of your web application dramatically. By following the steps outlined in this blog post, you can create a seamless interaction between your Java back-end and your jQuery front-end.
For deeper insights, remember to refer to the existing article titled "Revive Your Site: Solving jQuery GalleryView Issues" at tech-snags.com/articles/revive-site-solving-jquery-galleryview-issues. This resource will assist in troubleshooting any issues you may encounter with the GalleryView implementation.
Feel free to explore additional features of the GalleryView plugin and expand the functionality of your Java application as your project evolves. Happy coding!