Unlocking Java Secrets: What Animals Can Teach Us About Code
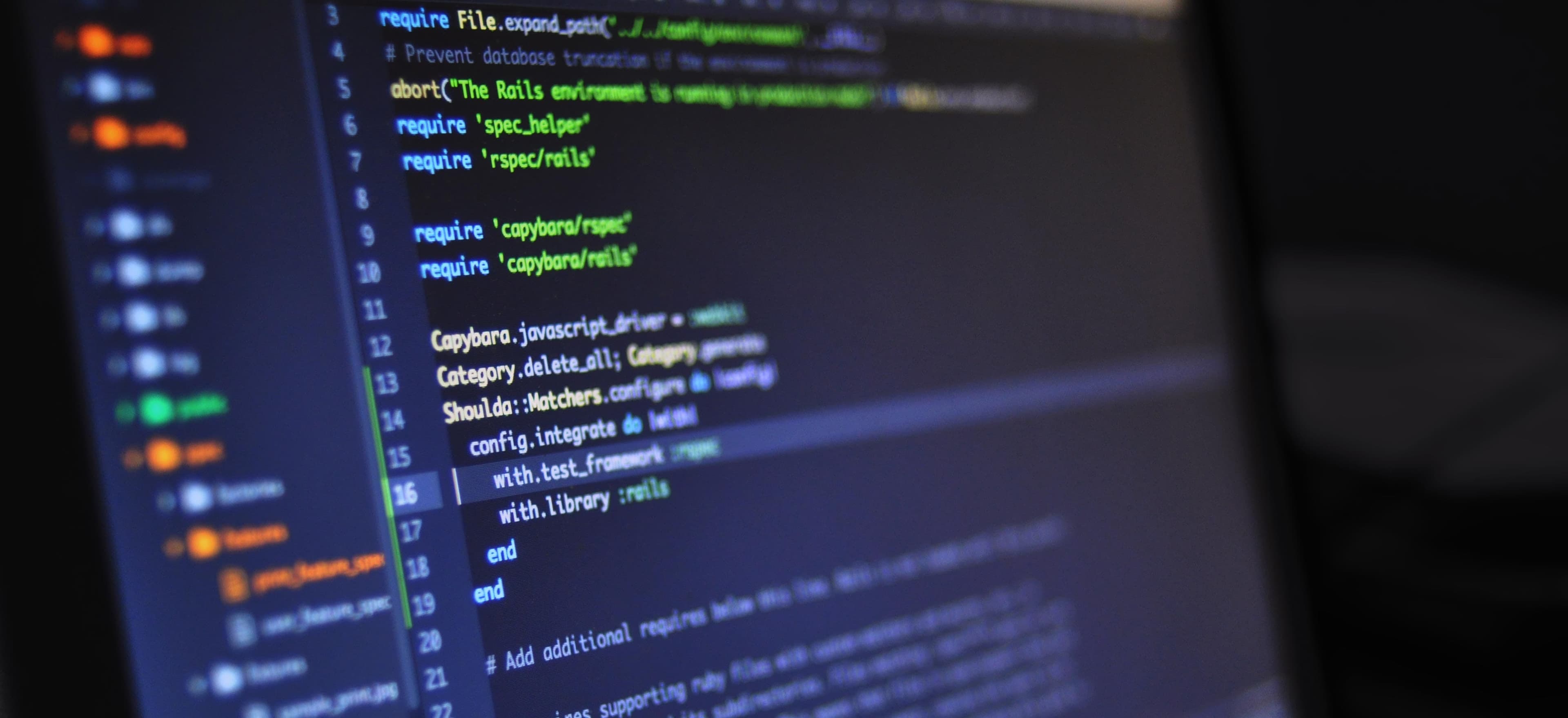
- Published on
Unlocking Java Secrets: What Animals Can Teach Us About Code
In the intricate world of programming, developers often find themselves navigating through complex tasks. Much like animals in the wild, we, as coders, can learn valuable lessons from nature that can enhance our Java coding practices. Drawing inspiration from an exploration of animal behaviors, as discussed in the article Tierische Botschaften: Wie Tiere uns Geheimnisse verraten, we will uncover how these principles can help us write cleaner, more efficient code.
The Art of Adaptation: Learning from Chameleons
Chameleons are renowned for their impressive adaptability. While it's not possible for Java developers to change their coding style on a whim, we can adjust our methodologies based on the project's requirements.
Code Snippet Example: Strategy Pattern
One way to implement adaptability in Java is through design patterns. The Strategy Pattern allows a class to choose behavior at runtime. This is akin to a chameleon changing colors based on its environment.
// Strategy Interface
public interface PaymentStrategy {
void pay(int amount);
}
// Concrete Strategy A
public class CreditCardPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
// Concrete Strategy B
public class PayPalPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using PayPal.");
}
}
// Context
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
paymentStrategy.pay(amount);
}
}
Commentary:
In this example, the ShoppingCart
class can adapt to different payment strategies. By implementing this pattern, the code remains flexible and can evolve based on its functionality, similar to how chameleons thrive in varying environments.
Collaboration: Lessons from Pack Animals
Pack animals, such as wolves, demonstrate the importance of teamwork. In coding, collaboration can lead to better software design and project delivery.
Code Snippet Example: Singleton Pattern
One approach to fostering collaboration in Java is through the Singleton Pattern, ensuring a class has only one instance while providing a global point of access. This is particularly useful in coordinating shared resources.
// Singleton Class
public class ConfigurationManager {
private static ConfigurationManager instance;
private ConfigurationManager() {
// Private constructor to prevent instantiation
}
public static ConfigurationManager getInstance() {
if (instance == null) {
instance = new ConfigurationManager();
}
return instance;
}
// Configuration methods
public String getConfigValue(String key) {
return "SomeConfigValue"; // Placeholder, simulate fetching config
}
}
Commentary:
In this code snippet, ConfigurationManager
guarantees that any part of the application accessing the configuration data will use the same instance. This reduces conflicts and fosters efficient communication among team members working on different components of a system.
Persistence and Tenacity: The Ant’s Approach
Ants symbolize persistence, working tirelessly toward a common goal. As developers, we face challenges requiring determination and strong problem-solving skills.
Code Snippet Example: Error Handling
When coding, the ability to handle errors gracefully is essential. Like ants finding alternate routes when obstacles arise, effective error handling leads to resilient applications.
public class FileHandler {
public void readFile(String filePath) {
try {
// Simulating file reading operation
System.out.println("Reading from file: " + filePath);
// Code that may throw IOException
} catch (IOException e) {
System.err.println("File not found: " + filePath);
// Implement a fallback mechanism, perhaps retry or log
}
}
}
Commentary:
In the FileHandler
class, we handle potential errors when attempting to read a file. This approach showcases the importance of being prepared for unexpected scenarios, just like an ant persists despite obstacles.
Efficiency: Swift Moves Inspired by Cheetahs
Cheetahs are often recognized for their speed and efficiency. Writing efficient code is a priority for any developer. Java offers various features that facilitate optimization.
Code Snippet Example: Stream API
The Java Stream API enables powerful data manipulation with concise, readable code, echoing the swift maneuvers of a cheetah.
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum: " + sum);
}
}
Commentary:
In the above example, the Stream API allows us to compute the sum of integers efficiently. This not only improves performance but also keeps the code concise and readable, avoiding verbose iterations.
The Closing Argument: Nature’s Lessons for Code Craftsmanship
The exploration of animal behaviors provides profound insights into improving our coding practices. From the adaptability of chameleons through design patterns to the collaboration inspired by pack animals, we can enhance our programming skills.
Final Thoughts
Emulating the qualities of persistence seen in ants and the efficiency of cheetahs allows us to create resilient, swift, and adaptable Java applications. As we continue our journey in programming, let us look to nature for inspiration and wisdom.
For those interested in additional insights into how animals share their secrets, check out this article, reinforcing the concept that learning can come from unexpected sources.
By integrating these concepts and patterns into our Java projects, we will become not only better coders but also effective collaborators and problem solvers, embodying the best traits of the natural world.
By leveraging both solid coding practices and lessons from nature, Java developers can can cultivate a robust skill set transforming challenges into opportunities for growth. Happy coding!