Uncovering Java's Secrets: Animal Data Processing Demystified
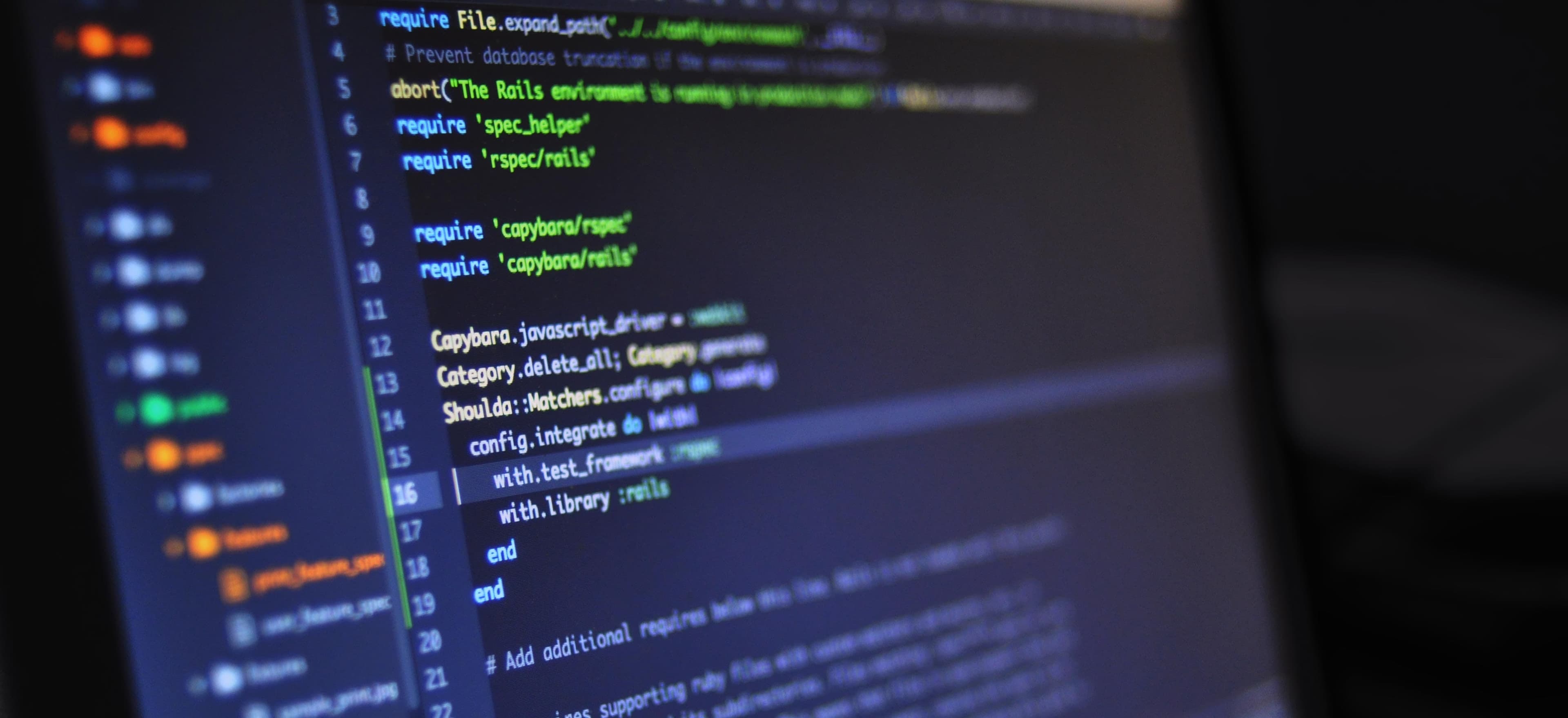
- Published on
Uncovering Java's Secrets: Animal Data Processing Demystified
Java is one of the most versatile programming languages, widely used for building applications across various domains, including data processing. The power of Java lies not only in its syntax but also in its robust libraries and frameworks that facilitate complex data manipulation. This blog post aims to explore Java's capabilities in processing animal-related data, drawing inspiration from the insightful article "Tierische Botschaften: Wie Tiere uns Geheimnisse verraten" (available at auraglyphs.com/post/tierische-botschaften-wie-tiere-uns-geheimnisse-verraten). By employing engaging methodologies, we will delve into how Java empowers developers in the realm of animal data analysis.
Understanding Data Structures
When processing animal data, selecting the right data structures is crucial. Java provides several collection frameworks: ArrayList, HashMap, and LinkedList, among others.
Example of ArrayList
import java.util.ArrayList;
public class AnimalData {
public static void main(String[] args) {
ArrayList<String> animals = new ArrayList<>();
animals.add("Lion");
animals.add("Elephant");
animals.add("Giraffe");
for (String animal : animals) {
System.out.println(animal);
}
}
}
Why Use ArrayList?
ArrayLists are dynamic arrays that can grow as needed. The choice of an ArrayList here allows for easy addition and modification of animal names, which is essential when dealing with unbounded datasets. The enhanced flexibility makes it an excellent choice for applications that require frequent updates.
A Closer Look at HashMap
Next, let’s discuss the potential of a HashMap in storing more complex data.
import java.util.HashMap;
public class AnimalTraits {
public static void main(String[] args) {
HashMap<String, String> animalTraits = new HashMap<>();
animalTraits.put("Lion", "Brave");
animalTraits.put("Elephant", "Intelligent");
animalTraits.put("Giraffe", "Tall");
for (String animal : animalTraits.keySet()) {
System.out.println(animal + ": " + animalTraits.get(animal));
}
}
}
Why Use HashMap?
HashMaps allow key-value pairs, providing an efficient way to associate animals with their traits. This structure enhances lookup efficiency, as retrieving traits for each animal is done in constant time on average.
Data Processing Techniques
Java's data processing capabilities extend far beyond the basics. Utilizing Java Streams can offer a powerful way to handle large datasets in a more functional style.
Using Java Streams
import java.util.ArrayList;
import java.util.List;
public class AnimalStreamDemo {
public static void main(String[] args) {
List<String> animals = new ArrayList<>();
animals.add("Lion");
animals.add("Elephant");
animals.add("Giraffe");
animals.add("Hippo");
animals.stream()
.filter(animal -> animal.startsWith("E"))
.forEach(System.out::println);
}
}
Why Use Streams?
Java Streams provide a concise way to express complex data processing operations, such as filtering, mapping, and reducing. In this example, we leverage the filter
method to retrieve animals starting with the letter "E." This approach enhances readability and reduces boilerplate code.
Real-World Applications: Analyzing Animal Behavior Data
Building on the earlier sections, let's look at how of all these Java features can be utilized to analyze animal behavior data. Imagine you are collecting data on various species and their captured behaviors.
Designing a Simple Data Model
class Animal {
private String name;
private String species;
private int age;
public Animal(String name, String species, int age) {
this.name = name;
this.species = species;
this.age = age;
}
public String getName() {
return name;
}
public String getSpecies() {
return species;
}
public int getAge() {
return age;
}
}
Why Use a Class?
Creating a dedicated class ensures that each Animal
object encapsulates related properties. This encapsulation is fundamental for organizing complex data and managing future enhancements or features in data processing.
Analyzing Data with Filter and Collect
Now, let’s retrieve animals older than a specific age and group them by species.
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class AnimalDataProcessing {
public static void main(String[] args) {
List<Animal> animals = new ArrayList<>();
animals.add(new Animal("Leo", "Lion", 5));
animals.add(new Animal("Ella", "Elephant", 10));
animals.add(new Animal("Gigi", "Giraffe", 2));
animals.add(new Animal("Hank", "Hippo", 8));
Map<String, List<Animal>> animalsOlderThan5 = animals.stream()
.filter(animal -> animal.getAge() > 5)
.collect(Collectors.groupingBy(Animal::getSpecies));
animalsOlderThan5.forEach((species, animalList) -> {
System.out.println("Species: " + species);
animalList.forEach(animal -> System.out.println(" - " + animal.getName()));
});
}
}
Why Collecting Data This Way?
The filter and collect operations allow us to smoothly sift through the data without manually iterating over the collections. By grouping animals by species, we can easily analyze how many older animals exist across different species, which can be vital in fields such as wildlife conservation and behavioral studies.
To Wrap Things Up
As highlighted throughout this blog post, Java offers numerous tools for processing and analyzing animal data. From using appropriate data structures like ArrayList and HashMap to the more sophisticated Java Streams and object-oriented design, developers can harness these features to gain insights into animal behavior and ecological studies.
If you find the theme of animal data analysis as fascinating as the secrets animals unveil to us in their behavior, I encourage further reading of Tierische Botschaften: Wie Tiere uns Geheimnisse verraten. This article can further illuminate the elaborate relationship between animal behavior and our understanding of nature.
By mastering these techniques in Java, you will be equipped to tackle a myriad of data-driven challenges in the field of animal study, ensuring that you contribute valuable insights towards conservation and animal welfare. Happy coding!
Checkout our other articles