Unlocking Java Secrets: What Nature Can Teach Us About Code
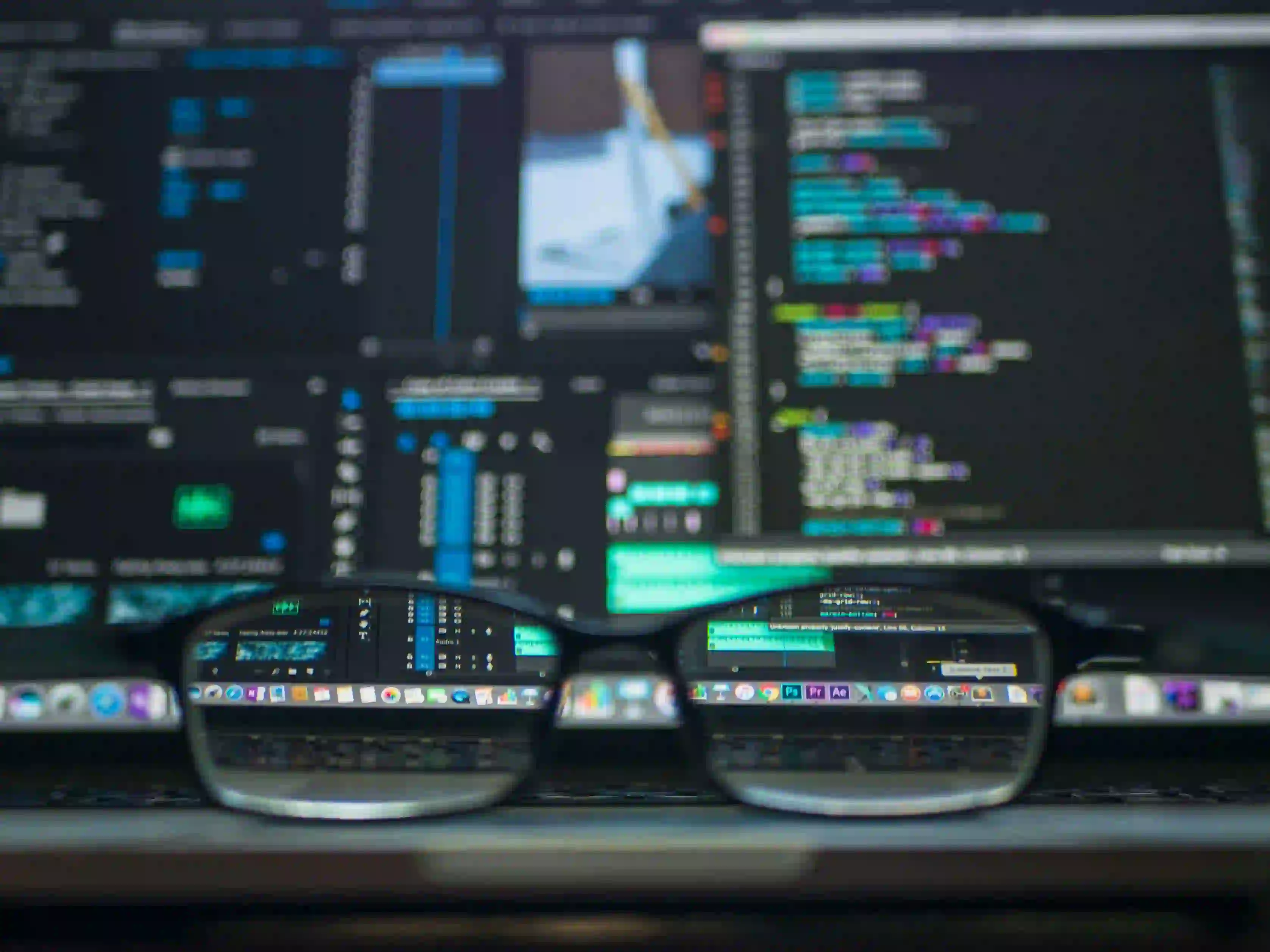
Unlocking Java Secrets: What Nature Can Teach Us About Code
Nature has always served as a rich source of inspiration for innovation, especially in the realms of technology and programming. Just as animals convey secrets through their behaviors, Java offers a unique set of features and best practices that can reveal effective programming techniques. Much like the concepts discussed in the article "Tierische Botschaften: Wie Tiere uns Geheimnisse verraten" (auraglyphs.com/post/tierische-botschaften-wie-tiere-uns-geheimnisse-verraten), we can decode the “messages” in Java to enhance our coding proficiency.
In this blog post, we will explore various Java programming concepts and features, illustrated with code snippets that clarify their purpose and importance. By connecting these principles to lessons we can learn from nature, we will unlock the secrets of effective Java programming.
The Importance of Structure: Learning from Beehives
When we observe how bees construct their hives, we notice a remarkable structure that allows them to work collaboratively. This is akin to how Java applications are built upon solid architectural principles.
Understanding Classes and Objects
In Java, everything revolves around the concept of classes and objects. A class serves as a blueprint for creating objects, while an object is an instance of a class. This structure is pivotal for organizing code clearly.
public class Bee {
private String type;
public Bee(String type) {
this.type = type;
}
public void displayType() {
System.out.println("The bee type is: " + type);
}
}
Why This Matters:
- Encapsulation: By using access modifiers (like private here), we protect the internal state of the object.
- Reusability: The class can be reused to create multiple instances of different bee types without duplicating code.
Instantiation
Now, let’s create an object of type Bee
.
public class Hive {
public static void main(String[] args) {
Bee workerBee = new Bee("Worker");
workerBee.displayType();
}
}
Explanation:
- Object Creation: This instance of
Bee
represents a worker bee. It showcases how we can create objects that contain specific behaviors and properties. - Method Invocation: Calling the method
displayType()
on ourBee
instance prints its type, demonstrating a concrete output from our object's properties.
The Power of Inheritance: Nature's Family Tree
Inheritance in Java is analogous to genetic traits passed down through generations. It allows one class to inherit the attributes and methods of another class, promoting code reusability and organization.
Parent and Child Classes
Let’s explore the concept of inheritance with an example featuring bees.
public class QueenBee extends Bee {
public QueenBee() {
super("Queen");
}
public void layEggs() {
System.out.println("The queen is laying eggs.");
}
}
Explanation:
- Extending Classes:
QueenBee
extends theBee
class, inheriting its properties while adding its own unique behavior. - Super Constructor: The
super("Queen")
call initializes thetype
for theQueenBee
.
Using the Subclass
Now, let’s create an instance of QueenBee
.
public class Hive {
public static void main(String[] args) {
QueenBee queen = new QueenBee();
queen.displayType(); // The bee type is: Queen
queen.layEggs(); // The queen is laying eggs.
}
}
Why This Matters:
- Reusability and Maintenance: By following inheritance principles, Java promotes DRY (Don't Repeat Yourself) code practices. You can build complex structures that are easier to maintain over time.
Polymorphism: Adaptation in Nature
Nature is replete with examples of adaptation — creatures that can change forms or behavior according to their surroundings. This is mirrored in Java through polymorphism, which allows methods to do different things based on the object that it is acting upon.
Method Overriding
Let’s create an example where we override a method in the parent class.
public class WorkerBee extends Bee {
public WorkerBee() {
super("Worker");
}
@Override
public void displayType() {
super.displayType();
System.out.println("This bee collects nectar.");
}
}
Demonstrating Polymorphism
Now, let's see polymorphism in action:
public class Hive {
public static void main(String[] args) {
Bee myBee = new WorkerBee(); // Reference of type Bee points to a WorkerBee object
myBee.displayType();
}
}
Explanation:
- Dynamic Method Dispatch: The method
displayType()
of theWorkerBee
class gets called. This happens because the actual object is of typeWorkerBee
, demonstrating polymorphism in action. - Flexibility: This concept adds flexibility to your code and enhances its scalability, allowing for future expansions.
Interfaces: Collaboration in Ecosystems
In nature, many species rely on symbiotic relationships for survival. Similarly, interfaces in Java allow different classes to collaborate, sharing a common contract while ensuring their specific implementations.
Creating an Interface
Let's define an interface for flying behavior:
public interface Flyable {
void fly();
}
Implementing the Interface
Now, let’s create a FlyingBee
class that can implement this interface:
public class FlyingBee extends Bee implements Flyable {
public FlyingBee() {
super("Flying Bee");
}
@Override
public void fly() {
System.out.println("The bee is flying!");
}
@Override
public void displayType() {
super.displayType();
System.out.println("And it's flying!");
}
}
Using the Flyable Interface
public class Hive {
public static void main(String[] args) {
FlyingBee flyingBee = new FlyingBee();
flyingBee.displayType();
flyingBee.fly();
}
}
Explanation:
- Contract Implementation: Classes that implement the
Flyable
interface are contractually obliged to provide thefly()
method, ensuring standardized behavior with room for individual class specifics. - Collaboration: The use of interfaces promotes a collaborative approach, allowing different classes to seamlessly work together while adhering to a standardized protocol.
To Wrap Things Up: Harmonizing Code with Nature's Wisdom
As demonstrated throughout this blog post, Java programming offers us valuable tools akin to the wisdom found in nature. Whether it is through structural organization, inheritance, polymorphism, or collaborative interfaces, we can unleash our programming potential by decoding the “messages” that Java reveals to us.
The seamless interplay between the principles of nature and the constructs of Java serves as a powerful reminder that effective programming requires not only technical skill but also an appreciation for the structure and relationships inherent in our code.
As you continue your journey with Java, let the lessons learned from nature guide your coding practices. For further inspiration, be sure to check out the article "Tierische Botschaften: Wie Tiere uns Geheimnisse verraten," which delves into the compelling connections between the natural world and our understanding of communication.
Whether you are starting or elevating your Java expertise, embracing these fundamentals can help you navigate the complexities of software development successfully. So, the next time you're writing Java code, think of the intricacies of beehives, the adaptation strategies of animals, and let nature inspire your programming journey. Happy coding!