Java Solutions for Managing Food Hydration Data Efficiently
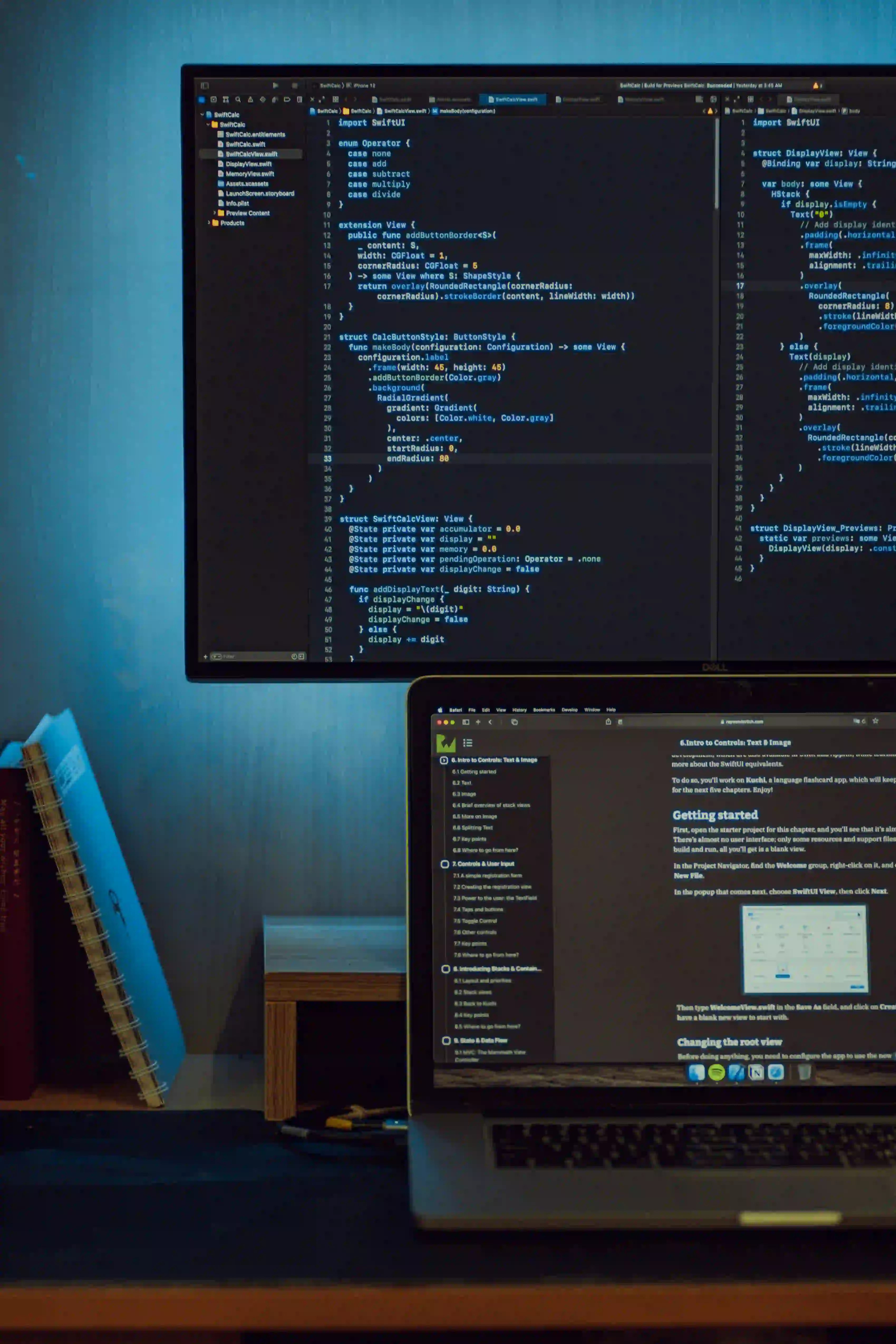
Java Solutions for Managing Food Hydration Data Efficiently
In the world of food preparation and survivalist habits, understanding hydration needs is paramount. Especially when dealing with freeze-dried versus dehydrated foods, the nuances can make a significant difference in health and nutrition. In a relevant article titled Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods, the importance of water needs is thoroughly discussed. This blog post will not only summarize essential hydration data but also explore how Java can be employed to manage and analyze this information effectively.
Why Manage Hydration Data?
Hydration data is crucial because it affects how we consume dehydrated foods. It influences factors like preparation time, nutritional values, and storage longevity. Without a systematic approach to managing this data, users may struggle with determining the proper hydration level, leading to inefficiencies or even health issues.
Setting Up Our Java Project
To begin, let’s set up a simple Java project that will help users maintain hydration data for different food types.
Dependencies
For this project, we will use basic Java libraries. Ensure that your Java environment is correctly set up. You can utilize an IDE like IntelliJ IDEA or Eclipse for convenience.
// Main class
public class HydrationManager {
public static void main(String[] args) {
// Your code will go here.
}
}
Using a Class to Represent Foods
It is essential to represent various food items with appropriate attributes, like water needs, preparation methods, and types. By leveraging object-oriented principles, we can create a class called Food
. This class will encapsulate all necessary hydration properties.
class Food {
private String name;
private double hydrationFactor; // Liters of water needed per kg food
private boolean isFreezeDried;
// Constructor
public Food(String name, double hydrationFactor, boolean isFreezeDried) {
this.name = name;
this.hydrationFactor = hydrationFactor;
this.isFreezeDried = isFreezeDried;
}
// Getters
public String getName() {
return name;
}
public double getHydrationFactor() {
return hydrationFactor;
}
public boolean isFreezeDried() {
return isFreezeDried;
}
}
Code Commentary
- Attributes: We have three attributes:
name
,hydrationFactor
, andisFreezeDried
. ThehydrationFactor
indicates how much water is required for each kilogram of food. - Constructor: The constructor initializes these values, allowing us to create instances of food items effortlessly.
- Getters: These methods enable the retrieval of values without exposing the internal state directly.
Storing Food Data
To store multiple food items, we can use a List
. This allows us to easily manage and query our hydration data.
import java.util.ArrayList;
import java.util.List;
public class HydrationManager {
private List<Food> foodList;
public HydrationManager() {
foodList = new ArrayList<>();
}
// Method to add food to the list
public void addFood(Food food) {
foodList.add(food);
}
// Method to print all food items
public void printFoodItems() {
for (Food food : foodList) {
System.out.println(food.getName() + ": " + food.getHydrationFactor() + "L/kg, Freeze-Dried: " + food.isFreezeDried());
}
}
public static void main(String[] args) {
HydrationManager manager = new HydrationManager();
// Adding some food items
manager.addFood(new Food("Apple", 1.2, false));
manager.addFood(new Food("Corn", 2.0, true));
manager.addFood(new Food("Chicken", 2.5, true));
// Print food items
manager.printFoodItems();
}
}
Explanation of the Enhancement
- List: We utilized an
ArrayList
to store our food objects, making it easy to add, retrieve or manage food data. - Methods:
addFood
: This method takes aFood
object and adds it to the list.printFoodItems
: This outputs all stored food items along with their hydration requirements.
Advanced Features: Calculating Total Hydration Needs
Let’s add functionality that calculates the total hydration needs based on the number of kilograms of food. This is where our hydration management can become more dynamic.
public double calculateTotalHydration(double kg) {
double totalHydration = 0;
for (Food food : foodList) {
totalHydration += food.getHydrationFactor() * kg;
}
return totalHydration;
}
// Using it in the main method
public static void main(String[] args) {
//... (previous code)
double totalHydrationNeeded = manager.calculateTotalHydration(5);
System.out.println("Total hydration needed for 5 kg of food: " + totalHydrationNeeded + " liters.");
}
Why This Feature is Important
Calculating total hydration needs makes it easier for users to understand the total amount of water they need to prepare all food items they will be consuming. This feature aids in meal planning, ensuring hydration requirements are met systematically.
To Wrap Things Up
In managing the hydration needs for freeze-dried and dehydrated foods, an organized approach using Java can significantly streamline the process. By storing food data in a structured format, users can efficiently access and compute hydration requirements.
Whether you're a survival enthusiast or just someone interested in nutrition and hydration, utilizing Java as a tool to manage this data can make a difference.
For further insights on hydration needs of various food types, don’t forget to check out the article titled Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods.
The journey into this realm of nutritional programming is just beginning. With Java, not only can we Google search hydration requirements, but we can also integrate this data smartly into manageable applications for daily life. Happy coding!