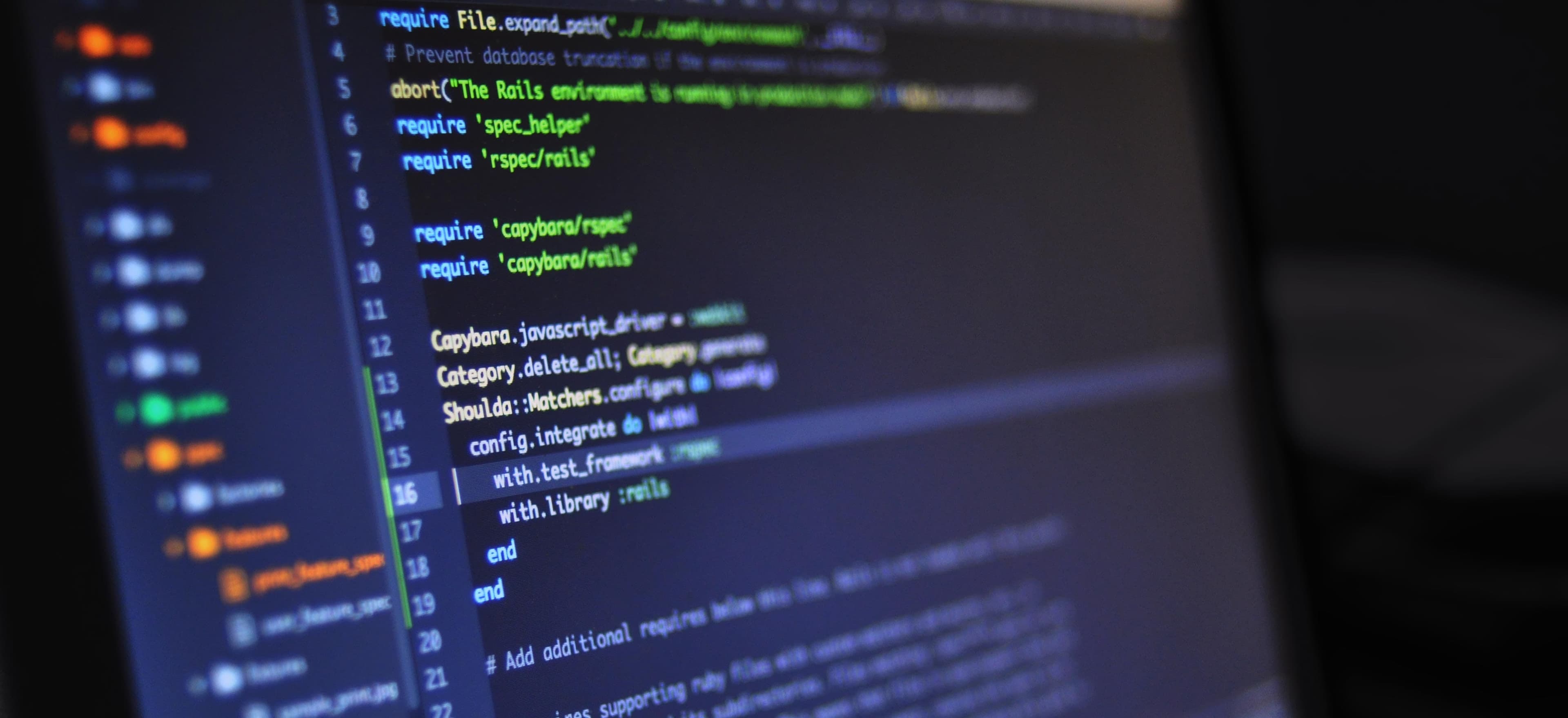
- Published on
Enhancing Java Web Apps with Dynamic Expand/Collapse Menus
In the world of web applications, user experience is paramount. As developers, we constantly strive to create interfaces that are intuitive, responsive, and most importantly, enjoyable to use. One of the UI elements that can significantly enhance the usability of Java web applications is the dynamic expand/collapse menu. In this post, we will explore how to implement these menus in your Java applications, along with some best practices and examples, all while maintaining a clear and concise format.
Understanding Expand/Collapse Menus
Before diving into the implementation, let’s understand what an expand/collapse menu is. It is a UI component that allows users to hide or show content, providing a cleaner interface while maximizing the available information display area. Such menus are especially useful in applications that deal with large sets of hierarchical data.
Benefits of Dynamic Expand/Collapse Menus
- Space Management: Dynamic expand/collapse menus help you save space by hiding content that may not need immediate attention.
- Improved Navigation: They enhance the navigation experience by allowing users to drill down into specifics without overwhelming them with all the data at once.
- User Engagement: A well-implemented expand/collapse menu can encourage users to interact with your content more, potentially increasing their engagement.
Setting Up the Project
To get started, we need to set up a simple Java web application, which can be done using Servlets, JSP, and CSS for styling. Below is a brief description of the prerequisites:
- Java SDK: Make sure you have Java installed. You can download it from the official Java website.
- Servlet Container: Apache Tomcat is a popular choice. Download it from the official Tomcat website.
- IDE: Any Java IDE like Eclipse or IntelliJ IDEA.
Project Structure
Your project structure should look somewhat like this:
YourWebApp
│
├── src
│ └── com.example
│ └── MyServlet.java
│
├── WebContent
│ ├── index.jsp
│ └── styles.css
│
└── WEB-INF
└── web.xml
Sample Code Implementation
1. The HTML Structure (index.jsp)
Let's create a simple JSP file that includes a menu structure. You can add this code to your index.jsp
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Dynamic Expand/Collapse Menu</title>
<link rel="stylesheet" type="text/css" href="styles.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function(){
$('.menu-item').click(function(){
$(this).siblings('.submenu').slideToggle();
});
});
</script>
</head>
<body>
<h1>Dynamic Expand/Collapse Menu</h1>
<div class="menu">
<div class="menu-item">Item 1</div>
<div class="submenu">
<div>Subitem 1-1</div>
<div>Subitem 1-2</div>
</div>
<div class="menu-item">Item 2</div>
<div class="submenu">
<div>Subitem 2-1</div>
<div>Subitem 2-2</div>
</div>
</div>
</body>
</html>
Explanation:
- The HTML structure sets up a list of menu items, each of which contains a submenu.
- JQuery is used to toggle visibility with a smooth sliding effect.
2. CSS Styles (styles.css)
Include the following styles to make your menu visually appealing:
body {
font-family: Arial, sans-serif;
}
.menu {
width: 200px;
border: 1px solid #ccc;
}
.menu-item {
background-color: #f2f2f2;
padding: 10px;
cursor: pointer;
border-bottom: 1px solid #ccc;
}
.submenu {
display: none; /* Initially hide submenus */
padding-left: 20px;
background-color: #e9e9e9;
}
Explanation:
- The menu items receive basic styles including padding and background colors.
- Submenus are initially hidden but will be displayed when the corresponding menu item is clicked.
3. Java Servlet (MyServlet.java)
Though our current implementation is mostly front-end, we'll use a Servlet to eventually serve dynamic content if needed.
package com.example;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/myServlet")
public class MyServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Here you can handle dynamic data retrieval if necessary.
}
}
Explanation:
- This simple Servlet is mapped to
/myServlet
and can be expanded later to handle dynamic data if your menu items require data fetched from a database or an API.
Best Practices
- Keep It Simple: Avoid overwhelming your users with too many options. Group related items together.
- Test Across Devices: Ensure your expand/collapse menu works seamlessly across different devices and browsers.
- Accessibility: Consider screen reader users by implementing ARIA roles to describe the state of expandable items.
- Performance Consideration: If you have a large dataset, consider fetching menu items through an AJAX call to avoid slow initial load times.
Key Takeaways
Implementing dynamic expand/collapse menus in your Java web applications can significantly improve user experience by allowing for better navigation and content management. Using jQuery for interactivity and basic styling with CSS creates a simple yet effective solution.
For further enhancement of your UI, you can integrate learnings from Mastering CSS: Create the Perfect Expand/Collapse Menu. This article provides in-depth CSS smart solutions that pair perfectly with our Java solution.
Incorporate this design pattern, and enhance the usability of your Java applications today! Whether you are creating a dashboard, admin panel, or any interactive site, a well-structured menu will always keep users engaged and informed. Happy coding!
Checkout our other articles