JavaScript Techniques for Dynamic Expand/Collapse Menus
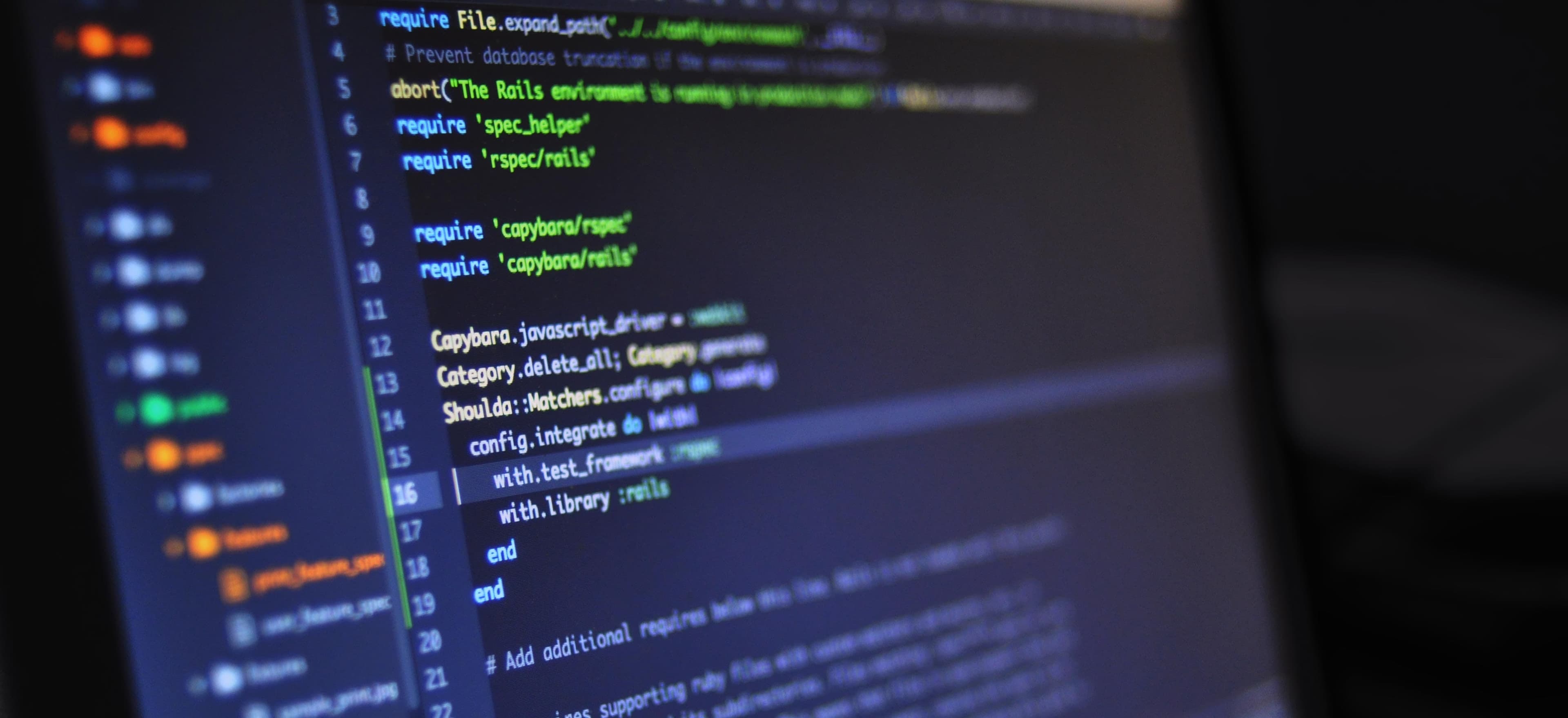
- Published on
JavaScript Techniques for Dynamic Expand/Collapse Menus
When it comes to creating interactive user interfaces, expand/collapse menus are essential. They offer a clean and organized way to present information, allowing users to focus on what matters most to them. In this blog post, we'll discuss various JavaScript techniques to implement dynamic expand/collapse menus that can enhance your web applications.
Expand/collapse menus not only improve user experience but also optimize space in your UI. Implementing them correctly requires a good understanding of both JavaScript and CSS. For optimal CSS styling, you may find our referenced article, Mastering CSS: Create the Perfect Expand/Collapse Menu, quite helpful.
Basic Structure of Expand/Collapse Menus
Before diving into any JavaScript code, it's essential to create a suitable HTML structure for the menu. A typical expand/collapse menu consists of a header that will act as the clickable area and a content area that will expand or collapse. Here's a simple example:
<div class="menu">
<div class="header">Menu Item 1</div>
<div class="content">
<p>This is the content for Menu Item 1.</p>
</div>
</div>
Explanation of Structure
- .menu: A wrapper that contains the entire menu item.
- .header: Clickable area that triggers the expand/collapse behavior.
- .content: Area that holds the content to be shown or hidden.
CSS For Basic Styling
Before we jump into JavaScript, let’s also write some CSS to style our menu:
.menu {
border: 1px solid #ccc;
margin: 10px 0;
}
.header {
background-color: #f1f1f1;
padding: 10px;
cursor: pointer;
}
.content {
display: none;
padding: 10px;
}
Motivation Behind the CSS
- .menu: Adds a border and spacing to each menu item.
- .header: Provides a clear, clickable area and changes the cursor to indicate it's interactive.
- .content: Hides the content by default using
display: none;
.
Using JavaScript to Implement Expand/Collapse Functionality
Now, let's enhance our menu with JavaScript functionality. We will add an event listener to the header that toggles the visibility of the content when clicked.
document.querySelectorAll('.header').forEach(header => {
header.onclick = function() {
const content = this.nextElementSibling;
if (content.style.display === 'block') {
content.style.display = 'none'; // Hide content
} else {
content.style.display = 'block'; // Show content
}
};
});
Why This JavaScript Code?
document.querySelectorAll('.header')
: This line collects all elements with the class "header" so we can add event listeners to each.header.onclick
: This sets a click event on each header. It's efficient and provides immediate feedback.this.nextElementSibling
: This retrieves the content area directly following the header. UsingnextElementSibling
is crucial as it ensures the JavaScript dynamically accesses the relevant content.
Advanced Techniques: Smooth Transitions
For a more visually appealing user experience, you might want to include smooth transitions when the content is shown or hidden. Instead of toggling display
, we can use max-height
, which allows for CSS transitions.
Updated CSS
Add the following to your CSS:
.content {
max-height: 0;
overflow: hidden;
transition: max-height 0.2s ease-out;
}
.content.expanded {
max-height: 200px; /* Adjust the max height as necessary */
}
Updated JavaScript
Now, let's adjust our JavaScript accordingly:
document.querySelectorAll('.header').forEach(header => {
header.onclick = function() {
const content = this.nextElementSibling;
content.classList.toggle('expanded'); // Toggle expanded class
if (content.classList.contains('expanded')) {
content.style.maxHeight = content.scrollHeight + "px"; // Set max height
} else {
content.style.maxHeight = null; // Reset max height
}
};
});
Explanation of the Enhancements
- CSS Transition: The transition creates a smooth effect when collapsing or expanding.
- Toggle Class: By adding or removing the 'expanded' class, it keeps the code clean and improves readability.
- Dynamic max-height: By setting the
max-height
dynamically toscrollHeight
, we ensure each content block is displayed fully.
Best Practices for Expand/Collapse Menus
- Accessibility: Include keyboard navigation options and ARIA attributes to make your menus usable for everyone.
- Semantic HTML: Consider using
<details>
and<summary>
tags for native support of expandable content. - Performance: Optimize performance by using event delegation if you expect a large number of headers.
- Visual Indicators: Indicate if a section is expanded or collapsed. This can be a simple icon change.
The Closing Argument
Implementing dynamic expand/collapse menus with JavaScript opens up a realm of possibilities for improving user interaction on your site. From basic features to advanced transitions, the techniques discussed here cater to various use cases.
Don't forget to refer to our handy styling guide in Mastering CSS: Create the Perfect Expand/Collapse Menu to perfect the look and feel of your menus.
By utilizing these techniques and best practices, you can create dynamic and user-friendly web applications that stand out. Happy coding!
Checkout our other articles