Hydration Challenges in Java: Managing Memory with Dried Data
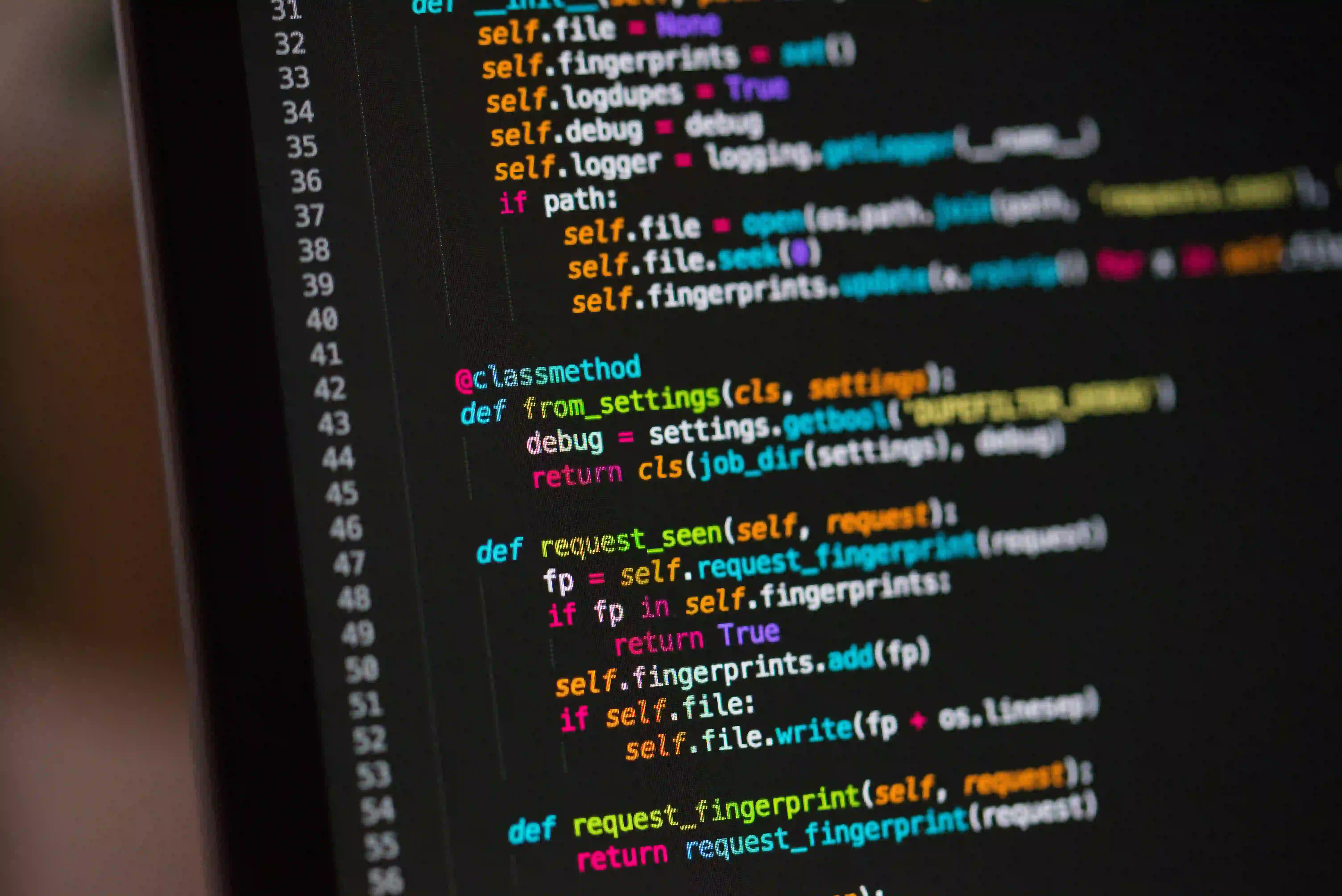
Hydration Challenges in Java: Managing Memory with Dried Data
In the realm of programming, resource management is paramount. Just as we need to hydrate ourselves with water to function optimally, Java developers must hydrate their applications with data, allocating memory efficiently and managing resources adeptly. This article delves into the concept of "hydration" in Java, particularly in relation to handling dried data—data that may not be readily available, such as information stored in a database or serialized objects.
If you've ever come across the article titled "Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods", you’ll appreciate the careful balance needed between resource allocation and consumption. Understanding how to manage memory effectively can help prevent performance bottlenecks and application crashes.
What is Data Hydration?
In Java programming, hydration typically refers to the process of converting raw data into usable objects. Often, this raw data comes in the form of JSON, XML, or response data from a database. The hydration process is crucial, as it converts this dried data into a form that your program can efficiently manipulate.
Here’s a succinct breakdown of how hydration works:
- Data Retrieval: Pulling data from a data source.
- Data Mapping: Mapping raw data to a specific object.
- Object Instantiation: Creating objects from the mapped data for use in the application.
Understanding this process is critical for efficient Java application design.
The Importance of Efficient Memory Management
Just like the hydration needs defined in "Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods," managing memory correctly can make the difference between a well-performing application and one that falters under load. Java employs garbage collection, which helps manage memory by automatically reclaiming space from objects that are no longer in use. However, reliant solely on this system can lead to performance issues such as memory leaks or excessive garbage collection cycles.
Memory-Leak Risks
Memory leaks occur when your application retains references to objects that are no longer needed, preventing garbage collection from freeing that memory. This is particularly relevant when you are dealing with large datasets or complex object structures.
Implementing a Simple Hydration Strategy
To illustrate the concept of data hydration in a Java context, let’s look at an example where we retrieve user data from a JSON API and convert it into Java objects.
Step 1: Dependency Management
First, ensure that you have the necessary library to handle JSON, such as Gson
from Google. If you are using Maven, include this in your pom.xml
:
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
Step 2: Create the User Class
Next, we will create a User
class that represents the data structure we expect to retrieve.
public class User {
private String name;
private String email;
// Constructor
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Getters
public String getName() {
return name;
}
public String getEmail() {
return email;
}
// toString for easy debugging
@Override
public String toString() {
return "User{name='" + name + "', email='" + email + "'}";
}
}
This code encapsulates user data effectively. Including a toString()
method assists in debugging, allowing you to print instances conveniently.
Step 3: Hydration from JSON
Next, we will write a method to fetch user data from an API and hydrate the User
objects:
import com.google.gson.Gson;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
public class UserFetcher {
private static final String USER_API_URL = "https://api.example.com/users"; // Replace with an actual API URL
public User fetchUser() throws Exception {
URL url = new URL(USER_API_URL);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setRequestMethod("GET");
// Check for a successful response
if (httpURLConnection.getResponseCode() == 200) {
Scanner scanner = new Scanner(url.openStream());
StringBuilder responseBuilder = new StringBuilder();
while (scanner.hasNext()) {
responseBuilder.append(scanner.nextLine());
}
scanner.close();
// Use GSON to convert the JSON response to User object
Gson gson = new Gson();
User user = gson.fromJson(responseBuilder.toString(), User.class);
return user;
} else {
throw new RuntimeException("Failed to fetch user data: " + httpURLConnection.getResponseCode());
}
}
}
Code Explanation
- URL Connection: We establish a connection to an API endpoint that returns user data in JSON format.
- Response Handling: The response code is checked to ensure the request was successful.
- Data Reading: Data from the connection is read via a scanner and compiled into a
StringBuilder
. - JSON Deserialization: Gson's
fromJson
method is invoked to hydrate ourUser
object from the JSON string.
Closing Remarks
The above implementation outlines a framework for fetching and hydrating data effectively in Java. However, as with any system, challenges may arise, particularly when dealing with large datasets or fluctuating API responses.
Consider caching frequent requests or implementing a retry mechanism to ensure reliability. This mirrors the lessons learned in managing hydration for food; just as the right amount of water ensures proper nourishment, the right amount of memory management can keep your Java applications running smoothly.
In conclusion, successful data hydration extends beyond technical implementation; it requires a thoughtful approach to memory management, coding practices, and application design. For further insights on resource management, you can familiarize yourself with the concepts discussed in Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods.
By understanding the hydration challenges in Java, you will not only improve your program's performance but also forge a path towards becoming a more proficient developer. Happy coding!