Mastering Generic Types in Java for Robust API Handling
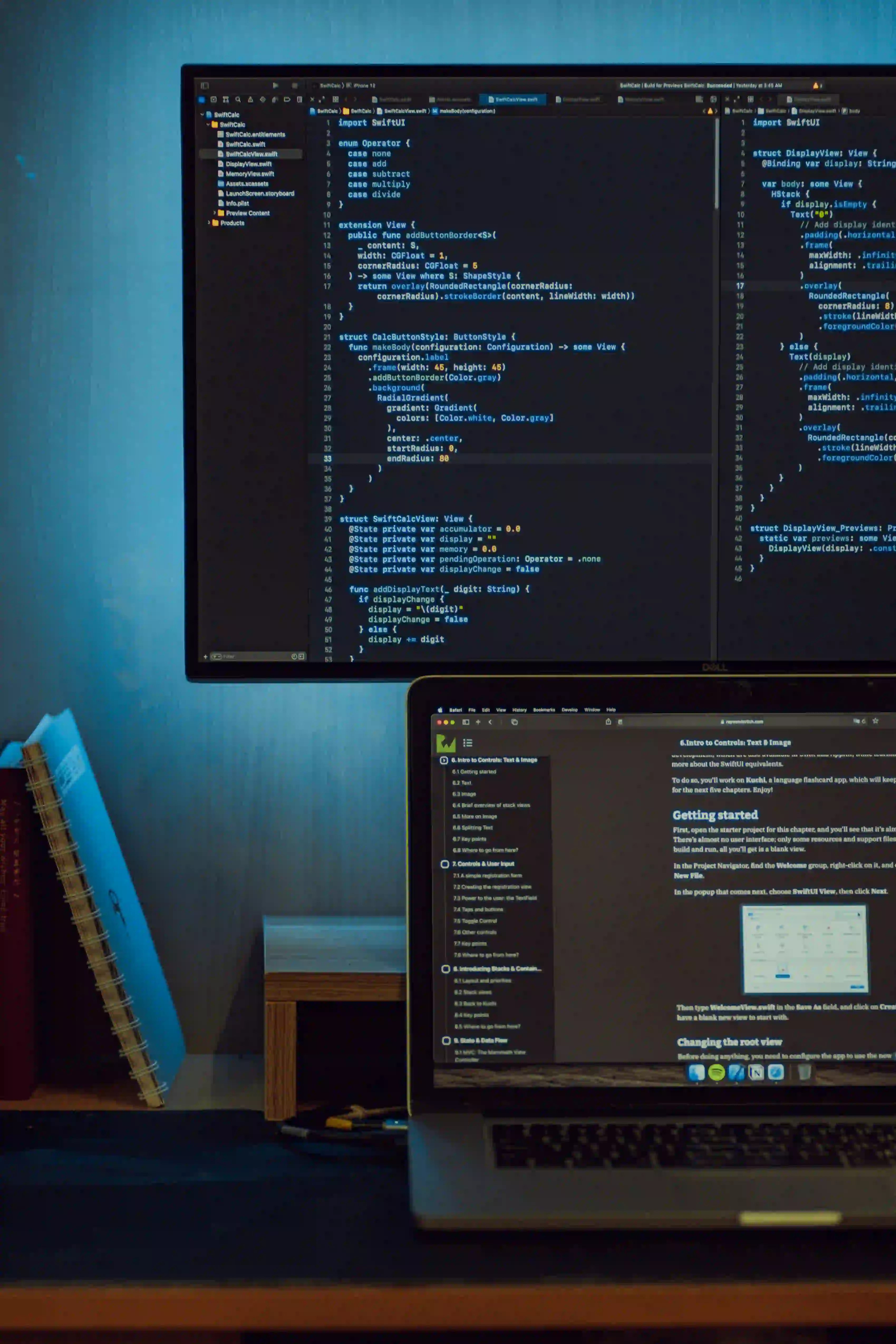
Mastering Generic Types in Java for Robust API Handling
Java is a powerful, versatile programming language widely used in enterprise applications, especially for creating robust APIs. One of the fundamental features that enhance the effectiveness of Java APIs is generic types. Understanding and mastering these characteristics can significantly improve code readability, reusability, and type safety.
In this article, we'll delve into the intricacies of generic types in Java, focusing on how they facilitate robust API handling. If you're already feeling challenged by generic types, you may find the article "Struggling with Generic Types in API Responses? Here's Help!" on infinitejs.com/posts/generic-types-api-responses-help beneficial as it covers essential tips and common pitfalls.
What Are Generic Types?
Simply put, generics enable types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. This powerful feature promotes type safety by allowing you to specify what type of object a collection can hold, eliminating the need for typecasting.
Why Use Generics?
- Type Safety: Generics offer compile-time checking, reducing the likelihood of
ClassCastException
at runtime. - Code Reusability: You can write a single method or class that operates on various data types.
- Cleaner Code: It often leads to less ambiguous code, enhancing both readability and maintainability.
Basic Syntax of Generics
Here's an example of a simple generic class:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Why Use This Code?
- The type parameter
T
makesBox
a generic class, meaning that it can hold an item of any specified type when instantiated. - The
setItem
method allows adding items to the box, whilegetItem
retrieves the item without casting.
Instantiating Generic Classes
You can use the Box
class as follows:
Box<String> stringBox = new Box<>();
stringBox.setItem("Hello Generics");
System.out.println(stringBox.getItem());
In this scenario, you specify String
as the type when creating a Box
. This specificity avoids type safety issues.
Generic Methods
Generics can also be applied to methods, allowing method definitions to work with various types. Here’s how to implement a generic method:
public class Utility {
public static <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
}
Why Use This Code?
- The
<T>
before the return typevoid
indicates that this method can operate with any object type. - This utility method prints elements of any array type passed to it.
Using Generic Methods
Here’s how to call the printArray
method:
Integer[] numbers = {1, 2, 3, 4, 5};
String[] names = {"Alice", "Bob", "Charlie"};
Utility.printArray(numbers);
Utility.printArray(names);
In both cases, printArray
works seamlessly with the specified types, showcasing efficient usage of generics.
Bounded Type Parameters
Sometimes, you may want to restrict the types that can be passed to a generic class or method. This is accomplished through bounded type parameters. Here’s how to do it:
public class NumericBox<T extends Number> {
private T number;
public void setNumber(T number) {
this.number = number;
}
public double doubleValue() {
return number.doubleValue();
}
}
Why Use This Code?
T extends Number
restrictsT
to Number subclasses such as Integer, Float, and Double, ensuring that only numeric values can be utilized.- The
doubleValue
method casts the number to a double type.
Using Bounded Type Parameters
Now, you can use the NumericBox
as follows:
NumericBox<Integer> intBox = new NumericBox<>();
intBox.setNumber(10);
System.out.println(intBox.doubleValue());
This ensures that you can enforce type checks at compile time, avoiding the potential pitfalls of working with incompatible types.
Wildcards in Generics
Wildcards provide flexibility in specifying a type. They are denoted by ?
and can be categorized into three main types:
- Unbounded Wildcards:
?
allows for any type. - Bounded Wildcards:
? extends T
allows forT
or its subtypes. - Lower Bounded Wildcards:
? super T
allows forT
or its supertypes.
Using Wildcards
Here’s an example demonstrating a method that utilizes wildcards:
public static void printNumbers(List<? extends Number> list) {
for (Number number : list) {
System.out.println(number);
}
}
Why Use This Code?
- The method can accept any list of
Number
or its subclasses, likeInteger
orDouble
, providing flexibility in working with multiple types. - This is particularly useful when you want to ensure that a method can operate with various related types without restricting the type argument.
Generics in API Responses
When designing APIs, handling responses with generic types can greatly streamline the process. For instance, by using generics in your API responses, you can ensure consistent data types and enhance readability.
Consider a simple API response using generics:
public class ApiResponse<T> {
private T data;
private String message;
private int statusCode;
// Getters and Setters
public T getData() { return data; }
public void setData(T data) { this.data = data; }
public String getMessage() { return message; }
public void setMessage(String message) { this.message = message; }
public int getStatusCode() { return statusCode; }
public void setStatusCode(int statusCode) { this.statusCode = statusCode; }
}
// Example Usage
ApiResponse<User> userResponse = new ApiResponse<>();
userResponse.setData(new User("John Doe"));
userResponse.setMessage("Success");
userResponse.setStatusCode(200);
Why Use This Code?
- The
ApiResponse
class allows you to encapsulate any response type (such as User, Product, etc.), promoting cleaner API design. - Using generics helps ensure that the client code can handle the data type flexibly without extensive type-checking or casting.
Lessons Learned
Mastering generic types in Java is not just about learning syntax; it's about enhancing your programming skills to create more robust, maintainable code. The power of generics is particularly evident in API handling, where type safety, reusability, and cleaner code lead to better application architecture.
If you find yourself grappling with generic types in your API responses, consider reviewing the detailed guidance in the article "Struggling with Generic Types in API Responses? Here's Help!" available at infinitejs.com/posts/generic-types-api-responses-help.
By leveraging generics, you can build APIs that are not only functional but also elegant and efficient. Happy coding!