Mastering Java Integration: Resolving GitLab Webhook Errors
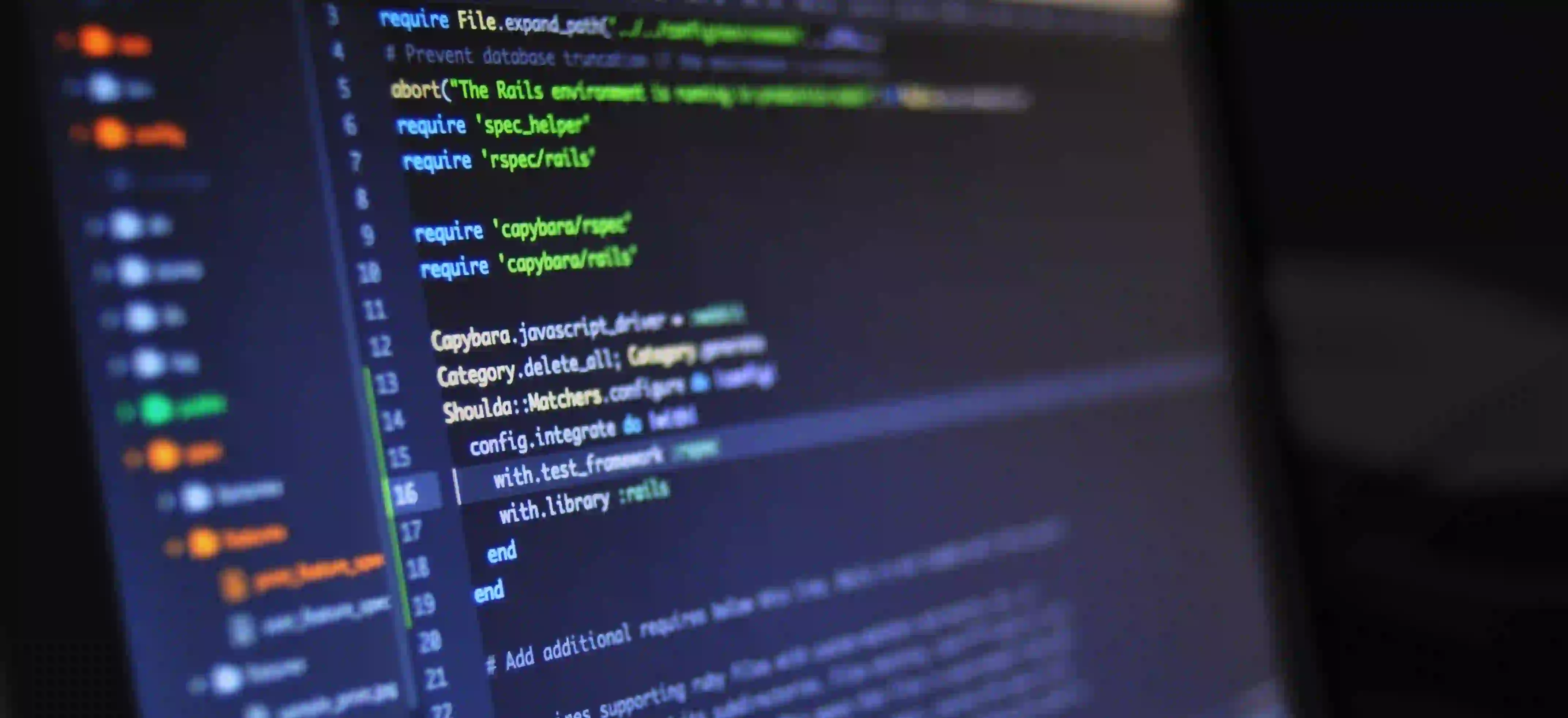
Mastering Java Integration: Resolving GitLab Webhook Errors
In the fast-paced world of software development, tools like GitLab play a crucial role in streamlining workflows through version control. One powerful feature of GitLab is the webhook, which enables real-time notifications about project events. However, developers sometimes encounter issues with these integrations. In this blog post, we will explore how to resolve common GitLab webhook errors using Java, guiding you step-by-step for a smoother continuous integration/continuous deployment (CI/CD) experience.
What Are Webhooks?
Webhooks are automated messages sent from apps when certain events occur. They allow for real-time interaction between different services. In the case of GitLab, webhooks can notify external services when actions like pushes, merge requests, or tag events happen in a repository. This is particularly useful for triggering builds, deployments, or other automated processes.
Common GitLab Webhook Issues
Webhooks can be prone to errors due to various reasons, such as configuration issues, network problems, or incorrect parameters. For more context on troubleshooting webhook issues, refer to the existing article titled Troubleshooting GitLab Webhooks: Fixing Connection Issues.
Here are some typical problems you might encounter along with solutions to resolve them using Java.
Issue 1: Incorrect URL Configuration
One of the most common issues when setting up a webhook is providing an incorrect URL. If GitLab cannot reach your application's endpoint, the webhook will fail to trigger.
Solution
Ensure your application is running and the endpoint is explicitly defined. Let’s look at a simple Java Spring Boot application that listens for webhook events.
@RestController
@RequestMapping("/webhook")
public class GitlabWebhookController {
@PostMapping
public ResponseEntity<String> handleWebhook(@RequestBody String payload) {
System.out.println("Received webhook: " + payload);
return ResponseEntity.status(HttpStatus.OK).body("Webhook received");
}
}
Why This Code Works:
- Here, we have defined a
POST
endpoint at/webhook
. - The
@RequestBody
annotation allows us to capture the JSON payload sent by GitLab, giving us access to the event data. - Returning a 200 OK response confirms to GitLab that the webhook was received successfully.
Issue 2: Network Configuration
Even with a correct URL, network configurations like firewall rules or private networks may block incoming requests from GitLab.
Solution
Validate your network settings, including firewall rules, to ensure traffic can flow through. Additionally, testing the endpoint with tools like Postman can help determine if it is accessible.
Example of a Test
curl -X POST http://yourserver.com/webhook -d '{"action": "push", "repository": {"name": "test-repo"}}'
Why This Test Is Valuable:
- By simulating a POST request to your endpoint, you can confirm whether the server is responding as expected and investigate any potential issues on the application side.
Issue 3: Parsing the Payload
Sometimes, the webhook might trigger, but the data does not parse correctly. This might be due to changes in the payload structure that your application is not accommodating.
Solution
Make sure to update your Java code to handle the expected payload structure. Here’s an example of how you might parse a JSON payload.
@RestController
@RequestMapping("/webhook")
public class GitlabWebhookController {
@PostMapping
public ResponseEntity<String> handleWebhook(@RequestBody WebhookPayload payload) {
// Use the payload object to operate on the event data
System.out.println("Event: " + payload.getEventType());
return ResponseEntity.status(HttpStatus.OK).body("Webhook received");
}
static class WebhookPayload {
private String eventType;
public String getEventType() {
return eventType;
}
public void setEventType(String eventType) {
this.eventType = eventType;
}
// Additional fields based on the payload structure...
}
}
Why This Payload Parsing Works:
- The
WebhookPayload
class represents the structure of the incoming JSON, allowing us to access specific fields programmatically. - This object mapping simplifies extracting information, which is critical for executing subsequent actions, such as triggering CI/CD jobs.
Issue 4: Missing Headers
Webhooks sent from GitLab typically contain essential headers, such as the X-Gitlab-Token
for authentication. If these headers are missing or incorrect, GitLab may ignore the request.
Solution
Check that your webhook configuration in GitLab includes the necessary headers and that your Java application verifies them. Here is a simple example:
@PostMapping
public ResponseEntity<String> handleWebhook(
@RequestHeader("X-Gitlab-Token") String token,
@RequestBody String payload) {
if (!"yourSecretToken".equals(token)) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("Invalid token");
}
System.out.println("Received webhook: " + payload);
return ResponseEntity.status(HttpStatus.OK).body("Webhook received");
}
Why This Security Check Is Necessary:
- Always verify incoming request tokens to prevent unauthorized access. This verification step is essential for maintaining the security of your application.
Issue 5: Timeouts and Retries
GitLab will retry the webhook if it doesn’t receive a successful response (2xx status code) within a specific timeout. To mitigate this, ensure that your webhook endpoint processes requests quickly.
Solution
Implementing asynchronous processing might be the key to ensuring your application can respond quickly while still performing necessary actions.
@PostMapping
public ResponseEntity<String> handleWebhook(@RequestBody String payload) {
CompletableFuture.runAsync(() -> {
// Process the webhook payload
processWebhook(payload);
});
return ResponseEntity.status(HttpStatus.ACCEPTED).body("Webhook will be processed");
}
private void processWebhook(String payload) {
// Long-running tasks can go here
System.out.println("Processing webhook: " + payload);
}
Why Asynchronous Processing Works:
- By returning an ACCEPTED (202) status code, you inform GitLab that the request has been received and will be processed later, thus minimizing timeout issues.
Bringing It All Together
Mastering Java integration with GitLab webhooks involves understanding how to handle potential errors effectively. From ensuring correct URL configurations, addressing network issues, parsing payloads correctly, verifying headers, and managing timeouts, each aspect plays a vital role in creating a seamless experience.
To deepen your understanding and for additional context on resolving webhook-related issues, check out the article on Troubleshooting GitLab Webhooks: Fixing Connection Issues. By implementing the solutions discussed here, you can enhance your CI/CD pipeline, making it more robust and responsive to changes in your development environment.
Feel free to share your experiences or additional tips in the comments below. Happy coding!