Solving GitLab Webhook Failures in Your Java Application
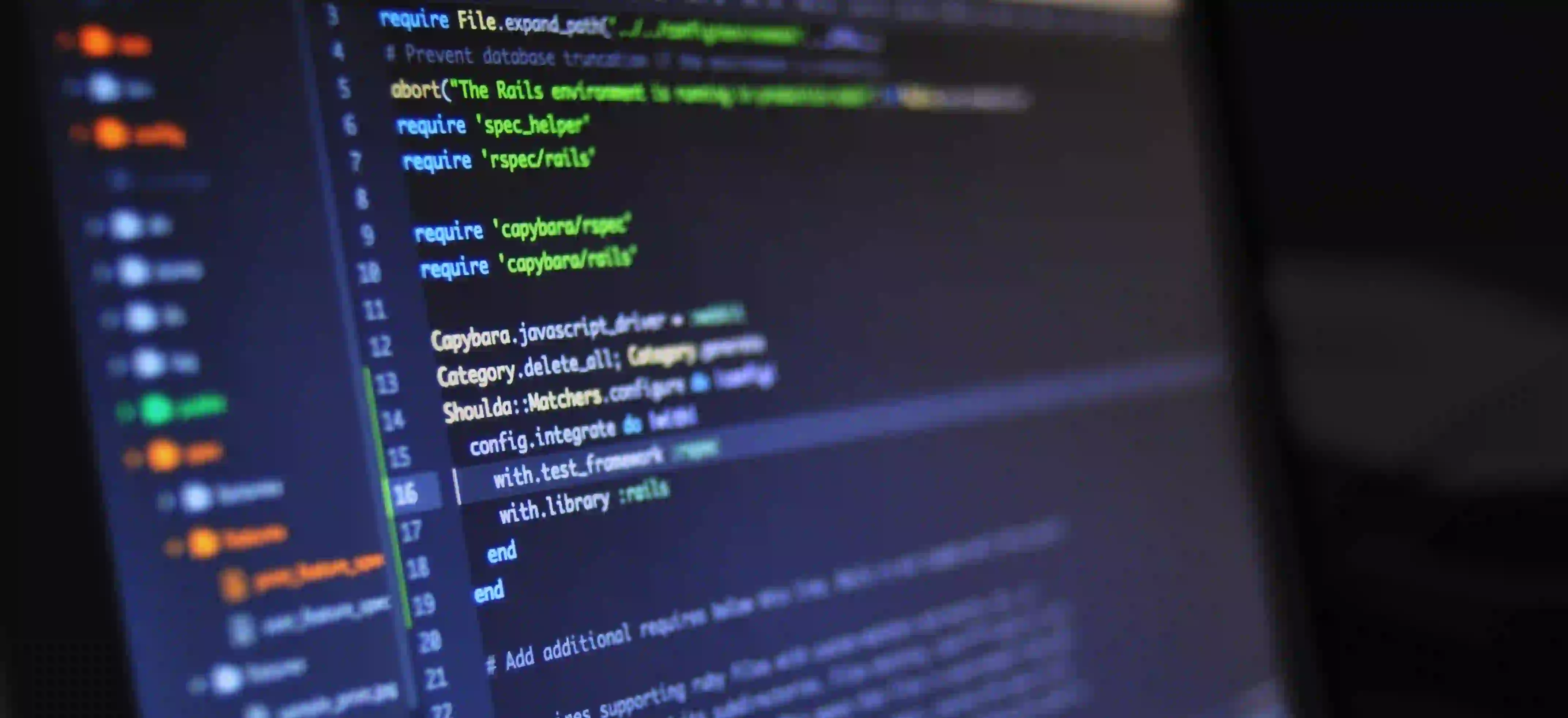
Solving GitLab Webhook Failures in Your Java Application
If you are developing applications that integrate with GitLab, you might have encountered the challenge of webhook failures. Webhooks are a powerful feature that allows GitLab to notify your application about various events, such as pushes, merges, and issues. However, when these notifications fail to reach your application, it can disrupt workflow and cause significant delays. In this post, we will explore how to diagnose and resolve these webhook issues within a Java application.
Understanding Webhooks
Webhooks are essentially HTTP callbacks; they allow GitLab to send real-time data to your server. When a specified event occurs, GitLab makes an HTTP POST request to your configured webhook URL with payload data about the event.
Example Structure of a Webhook Request:
{
"object_kind": "commit",
"event_type": "push",
"repository": {
"name": "my-repo",
"url": "https://gitlab.com/my-repo.git"
},
"commits": [
{
"id": "a1b2c3",
"message": "Sample commit message",
"timestamp": "2023-05-01T00:00:00Z"
}
]
}
In this structure, you can see vital information regarding the repository and the commit that triggered the notification. The challenge lies in receiving and processing this data accurately in your Java application.
Setting Up a Java Application to Handle GitLab Webhooks
Before we dive into troubleshooting, let’s set up a basic Java Spring Boot application that can receive webhooks.
Step 1: Create a Spring Boot Application
First, ensure you have your development environment ready. Create a new Spring Boot application using Spring Initializr. Choose dependencies such as Spring Web
and Spring Boot DevTools
.
Step 2: Implement the Webhook Endpoint
Here is a sample implementation of a simple webhook endpoint:
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/webhooks")
public class GitLabWebhookController {
@PostMapping("/gitlab")
public ResponseEntity<String> handleGitLabWebhook(@RequestBody String payload) {
// Log the payload for debugging purposes
System.out.println("Received GitLab webhook: " + payload);
// Handle the payload (parse, save to database, etc.)
// This is where you would add your processing logic.
return ResponseEntity.ok("Webhook received successfully");
}
}
Why This Code Matters
- Parsing the Payload: The
@RequestBody
annotation allows us to directly receive the POST payload data as a String. You can later parse this JSON string into a structured object, which is essential for handling significant payloads effectively. - Response Handling: We are returning an
HTTP 200
status to acknowledge receipt of the webhook, which helps GitLab understand that the request was successful.
Step 3: Testing Your Webhook Receiver
You can test your webhook endpoint by sending a POST request using tools like Postman or curl. For example:
curl -X POST -H "Content-Type: application/json" \
-d '{"object_kind": "push", "event_type": "push", "repository": {"name": "my-repo"}}' \
http://localhost:8080/webhooks/gitlab
Ensure your application is running and can handle the request. Verify if the logs show the received payload; if not, we will need to troubleshoot.
Troubleshooting Webhook Failures
Webhook failures often arise from multiple issues. Here are common problems and their solutions:
1. Incorrect Webhook URL
Ensure that the webhook URL is correctly configured in your GitLab repository settings.
- Solution: Visit your GitLab project Settings > Integrations. Verify that the URL matches your endpoint exactly.
2. Firewall and Network Issues
If your application is hosted behind a firewall or in a cloud environment, the incoming requests might be blocked.
- Solution: Ensure your server settings and firewall allow incoming requests on the port your application is listening to. This might involve adjusting cloud security settings if you're using services like AWS.
3. SSL Issues
When using HTTPS, GitLab requires valid SSL certificates. Self-signed certificates will likely lead to connection issues.
- Solution: Use a certificate from a recognized Certificate Authority (CA). You can also temporarily disable SSL verification in testing environments but note that this is not advisable for production.
4. Debugging Connection Problems
Leverage logging to gain insight into connection attempts and failures. You can use tools like Wireshark or telnet for deeper connection analysis. The information logged often helps identify connection issues promptly.
Example Logging Configuration with Log4j
To set up logging in your Java application, you can use Log4j:
<configuration>
<appender name="Console" class="org.apache.log4j.ConsoleAppender">
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="%d{yyyy-MM-dd HH:mm:ss} %p %c{1} - %m%n"/>
</layout>
</appender>
<root>
<priority value="debug"/>
<appender-ref ref="Console"/>
</root>
</configuration>
This configuration will help you track incoming requests and potentially identify failure points.
5. Reviewing Server Logs for Errors
When GitLab sends a webhook, it logs the response time and status. If your server responds with an error (4xx or 5xx series), investigate those logs.
Example of Checking Logs
tail -f /path/to/your/application/logs/application.log
Review the logs for response codes and errors related to the incoming requests.
Additional Resources
For deeper insights and best practices when troubleshooting webhooks, consider reading the Troubleshooting GitLab Webhooks: Fixing Connection Issues. This resource provides detailed guidelines on common issues and best practices for configuration.
Closing Remarks
Successfully integrating GitLab webhooks into your Java application requires a good understanding of both the concepts behind webhooks and the infrastructure needed to support them. With the steps outlined above, you should be well on your way to receiving and processing GitLab webhook notifications.
By following proper configurations and logging practices, you can ensure that your application reliably processes incoming events. Should you encounter issues, remember that detailed logs and well-defined error handling can significantly speed up troubleshooting.
If you have any questions or additional tips to share about managing GitLab webhooks in Java, feel free to leave a comment below!