Mastering Java Integration: Solving GitLab Webhook Challenges
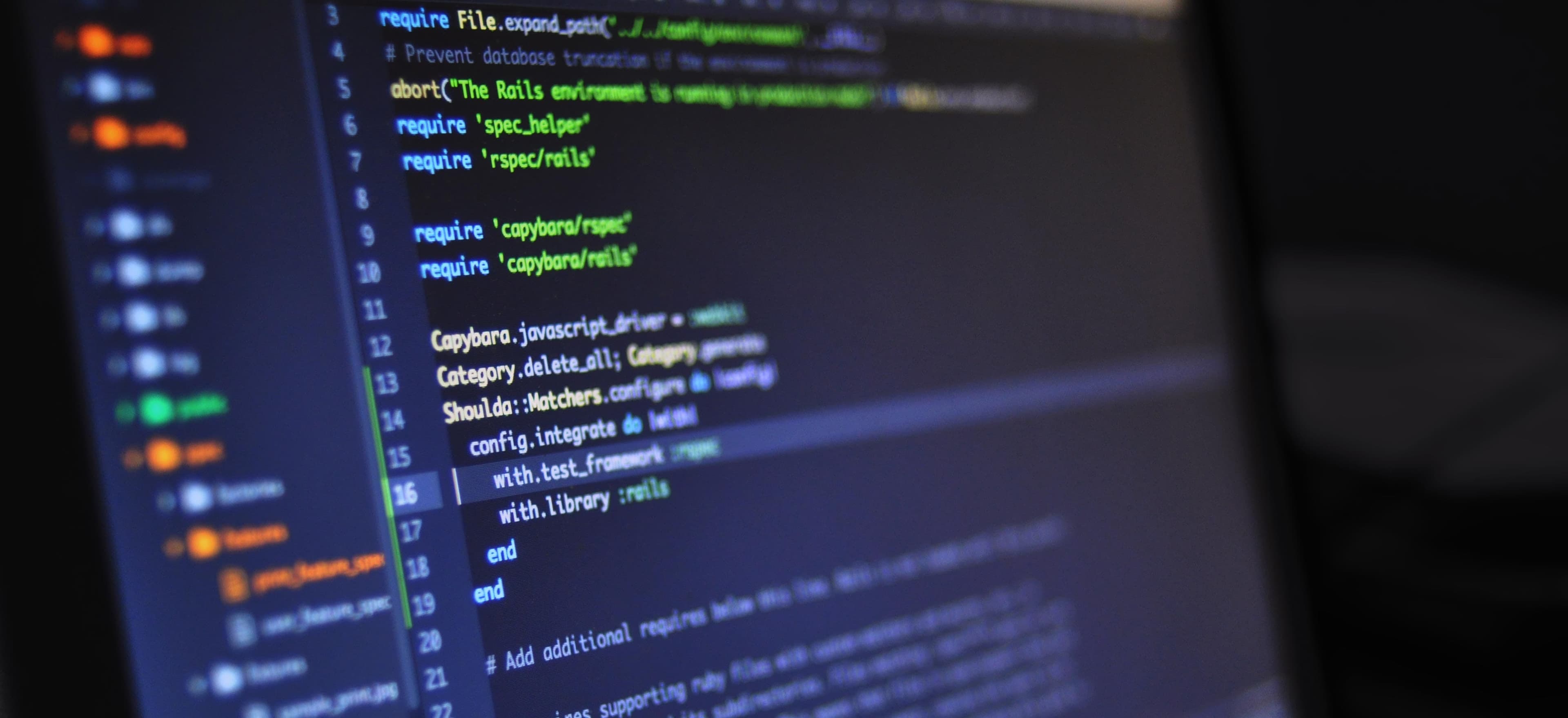
- Published on
Mastering Java Integration: Solving GitLab Webhook Challenges
In the modern software landscape, integrating various platforms poses both opportunities and challenges. One common integration technique is leveraging webhooks, especially in CI/CD environments like GitLab. Understanding how to effectively manage GitLab webhooks using Java is essential for seamless software deployments and updates.
This article will dive into the mechanics of GitLab webhooks, explore Java code snippets that facilitate webhook handling, and suggest best practices to troubleshoot connection issues. For an in-depth analysis of troubleshooting GitLab webhooks, check out Troubleshooting GitLab Webhooks: Fixing Connection Issues.
What are GitLab Webhooks?
GitLab webhooks are HTTP callbacks that are triggered by specific events occurring in a GitLab repository. When an event happens, GitLab sends a POST request to the specified URL, allowing you to automate processes or trigger actions in external systems.
Why Use Webhooks?
Webhooks enable a responsive integration model that eliminates the need for constant polling, saving both resources and time. For instance, when a new commit is made to your repository, a webhook can notify your CI server to start a new build automatically. This real-time feedback loop is crucial for achieving continuous integration and deployment.
Setting Up GitLab Webhooks
To set up a webhook in GitLab:
- Navigate to your repository.
- Go to Settings > Webhooks.
- Enter the URL of your Java application that will handle the incoming requests.
- Select which events you want the webhook to trigger on (Push events, Merge requests, etc.).
- Click Add webhook.
Your Java application must be prepared to handle the incoming JSON payloads that GitLab sends.
Creating a Simple Java Webhook Listener
Let's create a simple Java application to listen for GitLab webhooks. We’ll use Spring Boot for its ease of use and rapid development capabilities.
Step 1: Set Up a Spring Boot Application
Add the necessary dependencies to your pom.xml
:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Step 2: Create a Webhook Listener Class
This class will handle incoming webhook POST requests.
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GitLabWebhookListener {
@PostMapping("/gitlab/webhook")
public void handleWebhook(@RequestBody String payload) {
// Process the incoming payload
System.out.println("Received webhook payload: " + payload);
// Here you can convert the payload into a structured object as needed
}
}
Why Use @RestController
?
The @RestController
annotation combines @Controller
and @ResponseBody
, making it easy to build RESTful web services. This simplifies handling JSON data sent by GitLab.
Step 3: Running the Application
You can run your Spring Boot application by executing:
mvn spring-boot:run
Validating Incoming Requests
It's critical to ensure that the incoming requests are indeed from GitLab. This can be done using the webhook secret token.
Adding a Secret Token
- Set a secret token in your GitLab webhook settings.
- Append it to your listener to validate incoming requests.
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestHeader;
@RestController
public class GitLabWebhookListener {
private final String secretToken = "your_secret_token";
@PostMapping("/gitlab/webhook")
public void handleWebhook(@RequestBody String payload,
@RequestHeader(value = "X-Gitlab-Token") String token) {
if (!token.equals(secretToken)) {
System.out.println("Invalid token received.");
return;
}
System.out.println("Received valid webhook payload: " + payload);
}
}
Why Validate the Token?
Validating the token ensures that your application only processes requests from trusted sources, enhancing your webhook's security.
Logging and Exception Handling
Effective logging and exception handling are imperative for maintaining your webhook listener, as webhooks may fail for various reasons (network issues, malformed payloads, etc.).
Adding Logging
You can use SLF4J for logging purposes:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
// Inside the GitLabWebhookListener class
private static final Logger logger = LoggerFactory.getLogger(GitLabWebhookListener.class);
@PostMapping("/gitlab/webhook")
public void handleWebhook(@RequestBody String payload,
@RequestHeader(value = "X-Gitlab-Token") String token) {
if (!token.equals(secretToken)) {
logger.warn("Invalid token received.");
return;
}
logger.info("Received valid webhook payload: {}", payload);
}
Why Use SLF4J?
SLF4J (Simple Logging Facade for Java) provides a simple interface for various logging frameworks and is widely regarded for its flexibility and ease of use.
Troubleshooting Connection Issues
Even with a correctly set up listener, you may encounter issues with GitLab webhooks not firing. Here are some common troubleshooting tips:
- Check Firewall Settings: Ensure that your server allows incoming requests on the specified port (e.g., port 8080).
- Inspect Logs: Look into the logs for any errors or warnings to diagnose issues.
- Validate Endpoint: Test the webhook endpoint using tools like Postman or curl to ensure it's reachable.
- Review GitLab's Settings: Make sure the webhook URL and secret token are correctly configured.
For further guidance on fixing specific connection issues, refer to the article Troubleshooting GitLab Webhooks: Fixing Connection Issues.
To Wrap Things Up
Integrating GitLab with Java applications through webhooks provides a powerful mechanism for automating responses to changes in your repository. By crafting a robust webhook listener, validating incoming requests, and employing effective logging, you can create a seamless integration that enhances your CI/CD workflows.
As you delve deeper into Java and webhooks, remember that hands-on practice and troubleshooting are key to mastering integration techniques. Happy coding!
Checkout our other articles