Mastering SOLID Principles for Future-Proof Code
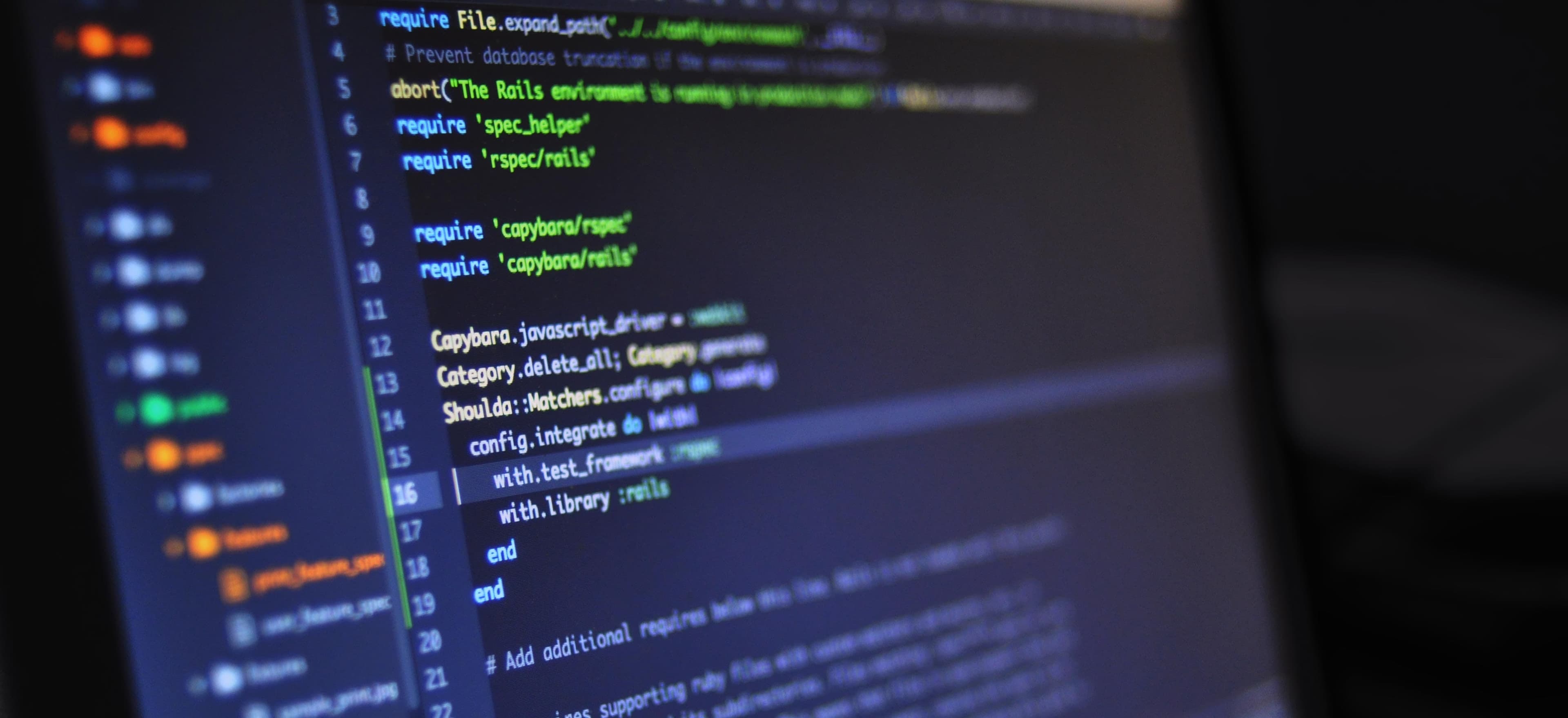
- Published on
Mastering SOLID Principles for Future-Proof Code
In the world of software development, writing maintainable, scalable, and robust code is paramount. Since we tread deeper into the realm of complexity, our coding practices should evolve as well. One such framework that has gained prominence is the SOLID principles. This acronym stands for five design principles to improve software development and maintainability. By adhering to these principles, you can write future-proof code that stands the test of time.
Let’s dive into each of the SOLID principles in detail, supplemented with real-world Java examples.
Understanding SOLID Principles
S - Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have one, and only one, reason to change. This means that a class should encapsulate only one functionality or behavior.
Why SRP?
By adhering to SRP, your classes become more manageable, ensuring that changes in one area don’t inadvertently affect others. This lays the groundwork for easier testing and debugging.
Example:
Let's demonstrate this principle with a simple Java example:
class Report {
private String title;
private String body;
public Report(String title, String body) {
this.title = title;
this.body = body;
}
public void generateReport() {
// logic to generate report
}
public void sendReport(String email) {
// logic to send the report via email
}
}
In this example, the Report
class has two responsibilities: generating the report and sending it. To adhere to SRP, we should separate these responsibilities into distinct classes.
class Report {
private String title;
private String body;
public Report(String title, String body) {
this.title = title;
this.body = body;
}
public void generateReport() {
// logic to generate report
}
}
class ReportSender {
public void sendReport(String email, Report report) {
// logic to send the report via email
}
}
Now, each class has a clear purpose and reason to change, adhering to SRP.
O - Open/Closed Principle (OCP)
The Open/Closed Principle asserts that software entities (like classes, modules, functions) should be open for extension but closed for modification. This means you should be able to add new functionality without altering existing code.
Why OCP?
By following OCP, you can reduce the risk of introducing bugs while expanding your system's capabilities. It promotes a more stable and predictable codebase.
Example:
Consider an interface for a payment system:
interface Payment {
void pay(int amount);
}
class PaypalPayment implements Payment {
public void pay(int amount) {
// implementation for PayPal
}
}
class CreditCardPayment implements Payment {
public void pay(int amount) {
// implementation for Credit Card
}
}
Here, both PaypalPayment
and CreditCardPayment
classes conform to the Payment
interface. If we want to introduce a new payment method, like a Bitcoin payment, we can create a new class without modifying existing classes.
class BitcoinPayment implements Payment {
public void pay(int amount) {
// implementation for Bitcoin
}
}
This way, we have extended functionality while keeping existing code intact.
L - Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
Why LSP?
This principle ensures that a subclass can stand in for its parent class without unexpected behavior, tightening up code reliability and supporting polymorphism.
Example:
Suppose we have a class Bird
and a subclass Penguin
.
class Bird {
public void fly() {
System.out.println("I can fly!");
}
}
class Sparrow extends Bird {
}
class Penguin extends Bird {
// Penguins don't fly!
@Override
public void fly() {
throw new UnsupportedOperationException("I cannot fly!");
}
}
In this scenario, Penguin
violates the LSP because substituting a Bird
with a Penguin
can lead to unexpected exceptions. A better approach is to restructure the inheritance hierarchy.
abstract class Bird {
public abstract void move();
}
class Sparrow extends Bird {
public void move() {
System.out.println("I can fly!");
}
}
class Penguin extends Bird {
public void move() {
System.out.println("I can swim!");
}
}
Now, both Sparrow
and Penguin
comply with the LSP as both can substitute Bird
without issues.
I - Interface Segregation Principle (ISP)
The Interface Segregation Principle suggests that no client should be forced to depend on methods it does not use. This principle advocates for smaller, more specific interfaces instead of one large, monolithic one.
Why ISP?
Embracing ISP leads to cleaner interfaces and code that is easier to maintain and understand.
Example:
Consider a large interface Animal
:
interface Animal {
void walk();
void swim();
}
class Dog implements Animal {
public void walk() {
// Dog walks
}
public void swim() {
// Dog can swim
}
}
class Fish implements Animal {
public void walk() {
// Not applicable for Fish
throw new UnsupportedOperationException("Fish can't walk!");
}
public void swim() {
// Fish swims
}
}
Here, Fish
is forced to implement the walk()
method, violating ISP. A better approach would be to create separate interfaces.
interface Walkable {
void walk();
}
interface Swimmable {
void swim();
}
class Dog implements Walkable, Swimmable {
public void walk() {
// Dog walks
}
public void swim() {
// Dog swims
}
}
class Fish implements Swimmable {
public void swim() {
// Fish swims
}
}
Now Dog
and Fish
only implement interfaces relevant to them, adhering to ISP.
D - Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Moreover, abstractions should not depend on details; details should depend on abstractions.
Why DIP?
This principle promotes loose coupling and increases the flexibility of your code.
Example:
Here’s a simplified approach that violates DIP:
class EmailService {
public void sendEmail(String message) {
// logic to send an email
}
}
class Notification {
private EmailService emailService;
public Notification() {
emailService = new EmailService(); // High-level module depends directly on low-level module
}
public void notify(String message) {
emailService.sendEmail(message);
}
}
In this scenario, the Notification
class is tightly coupled with EmailService
. To adhere to DIP, we can use an interface.
interface MessagingService {
void sendMessage(String message);
}
class EmailService implements MessagingService {
public void sendMessage(String message) {
// logic to send an email
}
}
class Notification {
private MessagingService messagingService;
public Notification(MessagingService messagingService) {
this.messagingService = messagingService; // Dependency Injection
}
public void notify(String message) {
messagingService.sendMessage(message);
}
}
Now, Notification
depends on the MessagingService
abstraction rather than a concrete implementation, resulting in more flexible and maintainable code.
Closing Remarks
Mastering the SOLID principles is key to writing high-quality, future-proof code. These principles simplify understanding, testing, and refactoring, leading to a more maintainable codebase.
By systematically applying these principles, you can create systems that are resilient to change and extendability.
If you’re eager to explore more about software design principles, consider checking out Martin Fowler's blog, which offers in-depth insights and guidance on Agile software development and design patterns.
By incorporating SOLID principles into your Java development practices, you not only enhance your productivity but also ensure that your applications stand strong in an ever-evolving tech landscape. Happy coding!