Optimizing Java Bean Indexing for Effective Elasticsearch Search
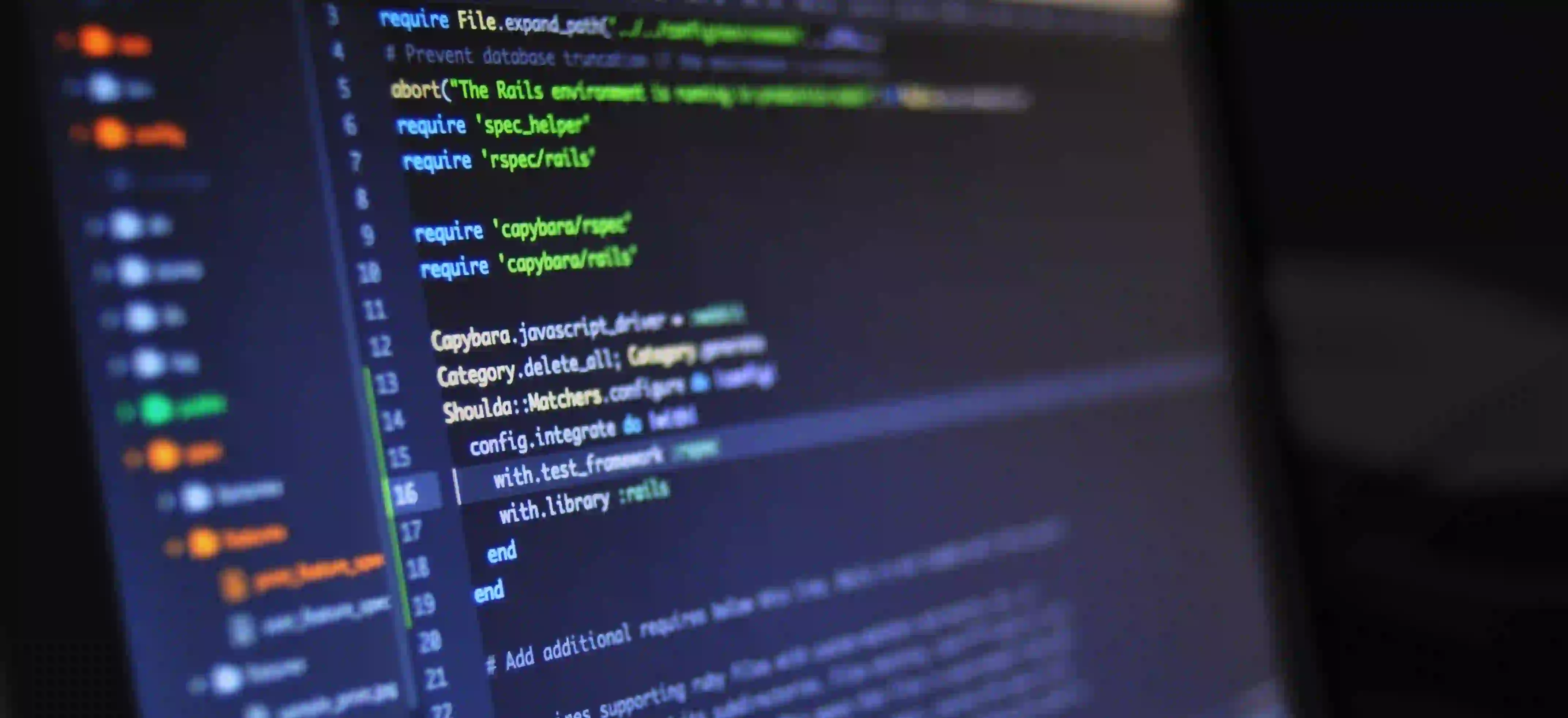
Optimizing Java Bean Indexing for Effective Elasticsearch Search
In a world where data is paramount, leveraging efficient search mechanisms becomes essential. Elasticsearch, an open-source search engine, offers powerful full-text search capabilities and scalable querying functionalities. When paired with Java, it allows developers to build robust applications that need to store and retrieve data effectively. In this post, we will explore how to optimize Java Bean indexing for effective searches in Elasticsearch.
Understanding Elasticsearch Concepts
Before diving into optimization techniques, let’s recap some fundamental concepts of Elasticsearch:
-
Index: An index is a collection of documents that share similar characteristics. In the context of Java Beans, each bean can represent a document.
-
Document: This term refers to the basic unit of information that can be indexed. For our purposes, it would be the serialized form of a Java Bean.
-
Field: Each document consists of fields which can contain data in the form of strings, numbers, dates, etc.
-
Mapping: Defines how a document and its fields are stored and indexed.
Elasticsearch is designed for fast searching. Therefore, how you structure and index your Java Beans significantly impacts performance and retrieval accuracy.
Structuring Your Java Beans
To facilitate effective mapping and transformation into documents, your Java Beans should follow a clean and organized structure. Consider the following example of a simple Java Bean:
public class Product {
private String id;
private String name;
private String description;
private double price;
private String category;
// Constructors, getters, and setters
}
Why This Structure?
- Clarity: Each property is self-explanatory, making it easier for developers to understand.
- Encapsulation: Using private fields and public getters/setters ensures that your data is well-protected and manageable.
Creating Elasticsearch Mappings
Mapping is crucial in Elasticsearch, as it determines how the fields of the Java Bean will be indexed and searchable. Once you define a mapping, Elasticsearch uses it to correctly parse the fields when indexing and querying.
Here’s how you can define a mapping for the Product
bean in Elasticsearch:
PUT /products
{
"mappings": {
"properties": {
"id": {
"type": "keyword"
},
"name": {
"type": "text"
},
"description": {
"type": "text"
},
"price": {
"type": "double"
},
"category": {
"type": "keyword"
}
}
}
}
Explanation of Mapping
- keyword: Suitable for fields that should not be analyzed, e.g., IDs and categories.
- text: Used for fields that need to be full-text searched, enabling Elasticsearch to tokenize and analyze these fields.
For a more in-depth understanding, refer to the Elasticsearch Mapping Documentation.
Indexing Java Beans
To optimize how Java Beans are indexed on Elasticsearch, we need to consider the data that gets sent for indexing. Using a REST client library such as Elasticsearch RestHighLevelClient, you can easily perform indexing.
Here is an example code snippet that demonstrates indexing a Product
bean.
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import com.fasterxml.jackson.databind.ObjectMapper;
public void indexProduct(Product product, RestHighLevelClient client) throws IOException {
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(product); // Convert Product to JSON
IndexRequest indexRequest = new IndexRequest("products")
.id(product.getId())
.source(jsonString, XContentType.JSON);
client.index(indexRequest, RequestOptions.DEFAULT);
}
Why This Code?
- JSON Serialization: The
ObjectMapper
converts the Java Bean into a JSON string, which is the format Elasticsearch accepts for indexing. - IndexRequest: This constructs the request to index the document under the
products
index.
Optimizing Indexing Performance
- Bulk Indexing: Instead of indexing documents one by one, using the Bulk API allows you to send multiple indexing requests in a single API call. This can significantly improve indexing throughput.
BulkRequest bulkRequest = new BulkRequest();
for (Product product : productList) {
String jsonString = mapper.writeValueAsString(product);
bulkRequest.add(new IndexRequest("products").id(product.getId()).source(jsonString, XContentType.JSON));
}
client.bulk(bulkRequest, RequestOptions.DEFAULT);
- Refresh Interval: Adjusting the refresh interval of your index can help optimize write-heavy operations. By default, Elasticsearch refreshes the index every second. This can be modified based on your data ingestion rate.
PUT /products/_settings
{
"index": {
"refresh_interval": "30s" // Refresh every 30 seconds
}
}
- Mapping Updates: Frequent changes to mappings can be expensive. Structure your mapping in such a way that you anticipate future changes enough to minimize mapping updates after indexing.
For more details, visit Optimizing Elasticsearch Indexing.
Querying Indexed Data
Once your data is indexed effectively, you will want to retrieve it efficiently. An effective query in Elasticsearch can enhance performance significantly. Here's an example of a simple search query:
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.index.query.QueryBuilders;
public void searchProductsByName(String productName, RestHighLevelClient client) throws IOException {
SearchRequest searchRequest = new SearchRequest("products");
searchRequest.source().query(QueryBuilders.matchQuery("name", productName));
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
// Process searchResponse here
}
Why This Approach?
- Efficient Querying: The
matchQuery
is perfect for full-text search and relies on tokenization. - Scalable: Elasticsearch is built for scalability, so your search can cater to a large dataset efficiently.
Lessons Learned
Optimizing Java Bean indexing in Elasticsearch is vital for building effective data retrieval systems. By carefully structuring your Java Beans, defining appropriate mappings, managing the indexing process, and querying efficiently, you can leverage the powerful capabilities of Elasticsearch to deliver robust performance.
Are you ready to optimize your data indexing strategy? To dive deeper into Elasticsearch indexing, check out the Elasticsearch Guide.
For support or discussions, feel free to connect or share your thoughts in the comments below!