Fixing JDK 14 InstanceOf EA Issues: A Quick Guide
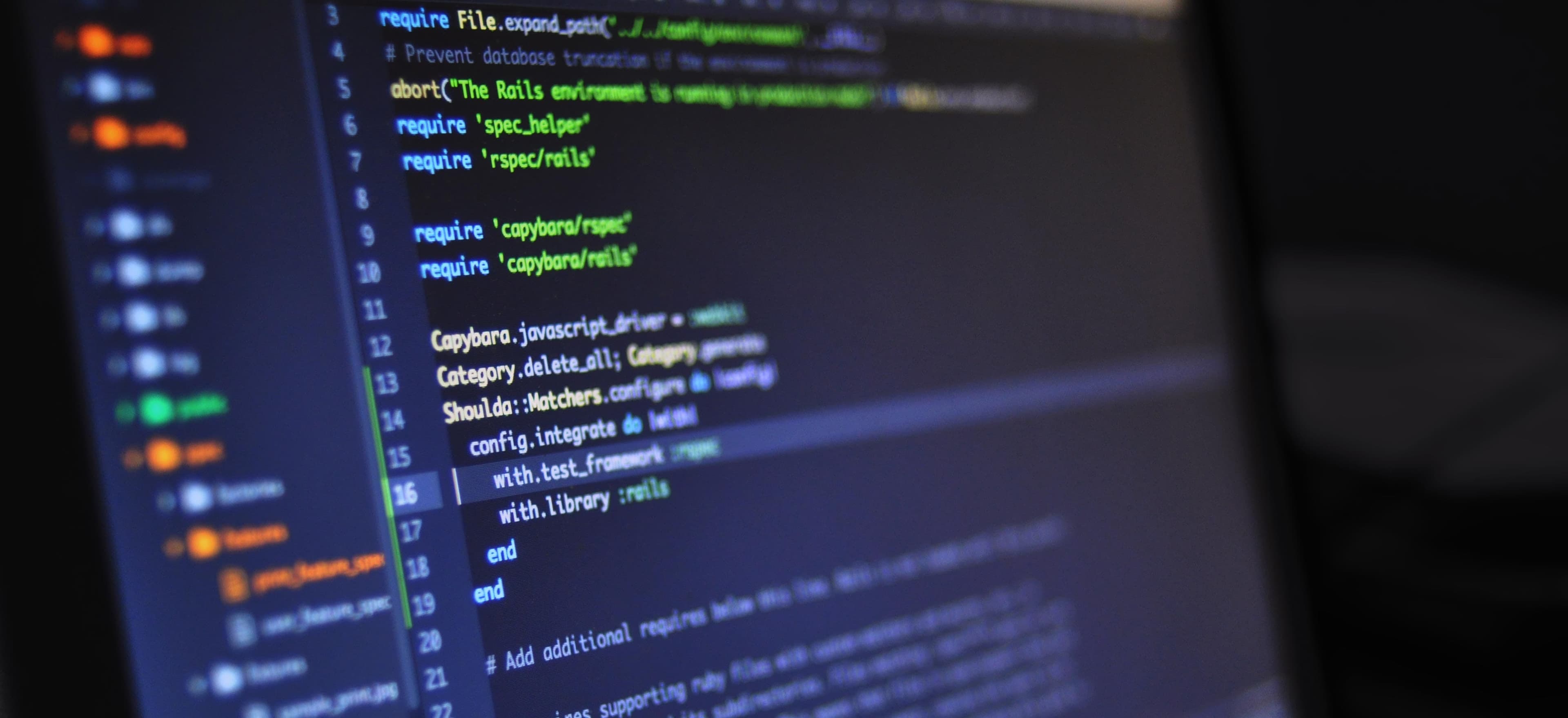
- Published on
Fixing JDK 14 InstanceOf EA Issues: A Quick Guide
Improving performance and enhancing readability, the instanceof
operator has seen several updates and improvements through various Java Development Kit (JDK) versions. Specifically, JDK 14 introduced an enhancement called JEP 305: Pattern Matching for instanceof. This enhancement allows you to combine type checking and type casting into a single operation.
However, with these updates come potential issues, especially concerning the effectiveness of escape analysis (EA). In this article, we will delve into the challenges faced with InstanceOf EA in JDK 14 and how to address them effectively.
What is Escape Analysis?
Escape Analysis is a technique used by the Java Virtual Machine (JVM) to determine the scope of object references. When the compiler can determine that an object does not escape the method in which it is created, it can optimize memory allocation, potentially placing objects on the stack instead of the heap, leading to performance improvements.
Why is This Important?
Improved memory management enhances application's performance, reduces garbage collection overhead, and might result in fewer memory leaks.
For more information on how Escape Analysis works, you can refer to Oracle's Documentation on Java Garbage Collection.
The Problem with JDK 14 InstanceOf EA
In JDK 14, changes to the instanceof
operator introduce complexities in how the compiler performs EA. Specifically, if the instanceof
check is not optimized correctly by the compiler, it can lead to unnecessary heap allocations instead of stack allocations.
Common Issues You Might Encounter
- Performance Degradation: Increased overhead in memory allocation due to ineffective EA management.
- Type Safety Issues: Potential casting errors that may arise from incorrect assumptions about object types.
Steps To Resolve InstanceOf EA Issues
1. Review Your Code
Start by reviewing instances of the instanceof
operator in your code. Pay attention to pattern matching and potential areas where escape analysis may fail.
Example Code Snippet:
public static void processShape(Shape shape) {
if (shape instanceof Circle circle) {
// Working with circle here
System.out.println("This is a circle with radius: " + circle.getRadius());
} else if (shape instanceof Rectangle rectangle) {
// Working with rectangle here
System.out.println("This is a rectangle with area: " + rectangle.getArea());
}
}
Why This Matters:
The above code elegantly combines type-checking and casting. However, depending on the context, this may lead to escape analysis issues, especially when the method processes a large number of shape objects.
2. Optimize Object Creation
Consider rethinking your object creation logic. If possible, create your objects only when necessary. For example, instead of creating new shape instances every time, consider reusing existing ones.
3. Use Local Variables Wisely
Local variables can help mitigate issues with escape analysis. By reducing the visibility of objects, the compiler can more confidently optimize memory management.
Example Code Snippet:
public static double calculateArea(Shape shape) {
double area = 0.0;
if (shape instanceof Circle c) {
area = Math.PI * Math.pow(c.getRadius(), 2);
} else if (shape instanceof Rectangle r) {
area = r.getLength() * r.getWidth();
}
return area;
}
Why Local Variables are Key:
This structure keeps references local, enhancing the chance for EA optimizations.
4. Profile and Test
Even after refactoring, it’s crucial to profile your application. Tools like Java VisualVM can provide insights into memory usage, helping you to recognize if your changes positively affect performance.
For more diagnostic tips, check out How to Profile Java Applications.
5. Update JVM Options
You can also tweak JVM options that may help the EA process. For example:
-XX:+DoEscapeAnalysis
-XX:MaxHeapSize=512m
These options can help enhance the compiler's efficiency in managing memory based on escape analysis.
6. Understand the Performance Characteristics
Be aware that while pattern matching is convenient and can improve readability, it may introduce a slight overhead compared to traditional casting. Always measure and know when to use these features.
Lessons Learned
While the JDK 14 instanceof
pattern matching feature introduces significant improvements, it can also create challenges with escape analysis. By understanding the intricacies of the instanceof
operator, optimizing object creation, utilizing local variables, and testing comprehensively, we can mitigate these EA issues and maintain high performance in our Java applications.
For further reading on escape analysis and how it impacts performance, see Escape Analysis Techniques.
In practice, applying these techniques will not only help in fixing existing issues but also contribute to cleaner and more efficient Java code going forward. Always remain vigilant about performance optimizations as you embrace new Java features!