Common Pitfalls When Using Spring Data JPA with Spring Boot
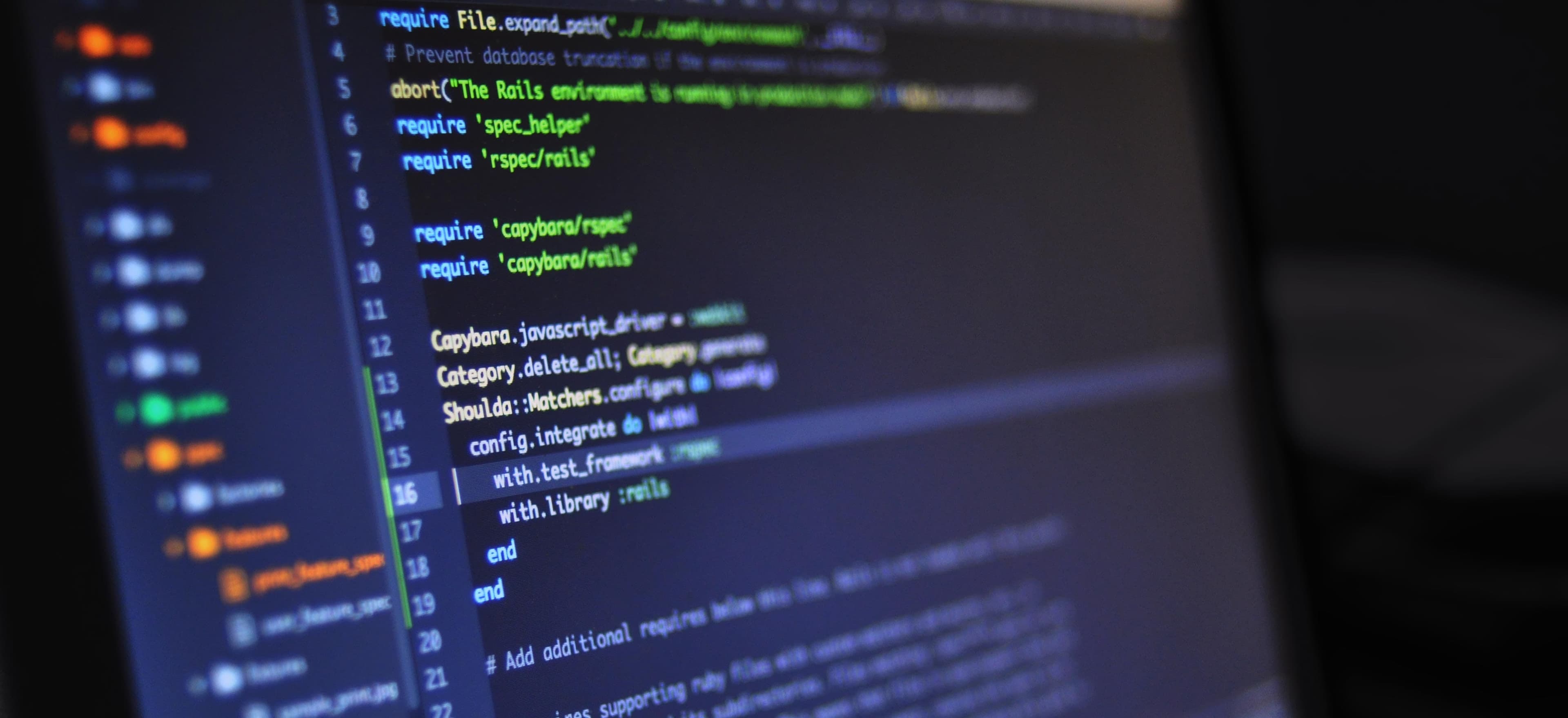
- Published on
Common Pitfalls When Using Spring Data JPA with Spring Boot
Spring Data JPA is a powerful framework that simplifies data persistence in Java applications. It integrates seamlessly with Spring Boot, allowing developers to build robust applications without much boilerplate code. However, while working with Spring Data JPA, developers may encounter pitfalls that can lead to performance issues, data inconsistency, or even application crashes. This blog post discusses common pitfalls when using Spring Data JPA with Spring Boot and offers practical tips to avoid them.
1. Not Configuring the Fetch Type Correctly
One of the most common mistakes developers make is not properly configuring the fetch type for their entity relationships. JPA provides two fetch types: EAGER
and LAZY
.
Eager vs Lazy Loading
- Eager Loading: This retrieves the associated entities immediately when the parent entity is fetched. It can lead to performance issues, especially with large data sets.
- Lazy Loading: This retrieves associated entities only when they are accessed. It's generally more efficient but requires care to prevent
LazyInitializationException
.
Example Code Snippet:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@OneToMany(fetch = FetchType.LAZY, mappedBy = "user")
private List<Order> orders;
}
Commentary: Using FetchType.LAZY
prevents loading all orders immediately when a user is fetched. This is crucial in scenarios with a large number of associated orders, improving performance and reducing memory consumption.
Solution
Always evaluate the relationships in your domain model. Use LAZY
loading for collections or large associations and only use EAGER
when absolutely necessary.
2. Ignoring Transactions
Transactions ensure data integrity and consistency when performing multiple related operations. Failing to use transactions in your repository methods can lead to data corruption or partial updates.
Example Code Snippet:
@Service
@Transactional
public class UserService {
@Autowired
private UserRepository userRepository;
public void createUserAndOrder(User user, Order order) {
userRepository.save(user);
order.setUser(user);
orderRepository.save(order);
}
}
Commentary: The @Transactional
annotation ensures that both user and order are saved in the same transaction. If one fails, neither will be committed, maintaining data integrity.
Solution
Use the @Transactional
annotation at the service layer where multiple repository calls are made. This ensures that all operations succeed or fail as one unit.
3. Overusing @Query
for Simple Operations
While Spring Data JPA provides powerful querying capabilities with the @Query
annotation, overusing it can lead to unnecessary complexity, especially for simple operations. For straightforward CRUD operations, rely on method naming conventions provided by Spring Data JPA.
Example Code Snippet:
public interface UserRepository extends JpaRepository<User, Long> {
User findByEmail(String email);
// Avoid
@Query("SELECT u FROM User u WHERE u.email = :email")
User findUserByEmail(@Param("email") String email);
}
Commentary: The first method is simpler and easier to maintain than the annotated version. Spring Data JPA efficiently translates the method name to the underlying query.
Solution
Use method naming conventions whenever possible. Reserve @Query
for complex queries or when dealing with specific custom logic.
4. Forgetting to Handle Optional
Returns
When dealing with optional results from repository methods, failing to handle potential null
values can lead to NullPointerExceptions
at runtime.
Example Code Snippet:
Optional<User> userOptional = userRepository.findById(1L);
User user = userOptional.orElseThrow(() -> new UserNotFoundException("User not found"));
Commentary: This approach makes the method fail gracefully by throwing a custom exception if the user is not found. It also maintains clean and readable code.
Solution
Always check for Optional
results and provide meaningful fallbacks or exceptions. This improves code safety and clarity.
5. Not Leveraging Spring Data JPA Projections
Spring Data JPA supports projections, which allow you to retrieve only the needed fields from an entity. Not using projections can lead to loading more data than necessary, which impacts performance.
Example Code Snippet:
public interface UserProjection {
String getUsername();
String getEmail();
}
public interface UserRepository extends JpaRepository<User, Long> {
List<UserProjection> findAllBy();
}
Commentary: Using projections fetches only necessary fields like username
and email
, reducing the payload size and improving application performance.
Solution
Evaluate your queries and, where applicable, define interfaces for projections to load only the necessary attributes of your entities, especially in complex queries.
6. Ignoring Entity Lifecycle Events
JPA entities have lifecycle events (like pre-persist, post-load, etc.) that can help manage tasks such as setting default values or validating attributes. Neglecting these can lead to inconsistencies in your application's state.
Example Code Snippet:
@Entity
public class User {
@PrePersist
public void prePersist() {
this.createdAt = LocalDateTime.now();
}
private LocalDateTime createdAt;
}
Commentary: By using @PrePersist
, every time a User
entity is created, the createdAt
field is automatically set, ensuring every record has this essential timestamp.
Solution
Utilize lifecycle callback annotations (@PrePersist
, @PostLoad
, etc.) to manage entity states consistently.
7. Skipping Caching for Read-heavy Applications
If your application performs many read operations, caching the results can dramatically improve performance. Spring Data JPA supports caching, but many developers overlook it.
Example Code Snippet:
@EnableCaching
@Configuration
public class CacheConfig {
// Cache configuration here
}
@Service
public class UserService {
@Cacheable("users")
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
Commentary: The @Cacheable
annotation indicates that the result of the method should be cached, and subsequent calls will retrieve the data from the cache rather than hitting the database.
Solution
For applications with significant read-heavy operations, implement caching strategies using Spring's caching abstraction to enhance performance.
My Closing Thoughts on the Matter
Spring Data JPA is a powerful ally for managing data in Spring Boot applications, but it comes with its share of common pitfalls. By understanding and addressing these issues, you can build robust, maintainable applications while avoiding performance bottlenecks and data integrity problems.
This list is not exhaustive, but by being aware of these common pitfalls and adopting best practices, you will set yourself on a sound path toward leveraging the full potential of Spring Data JPA.
For further learning, consider reading through the official Spring Data JPA documentation and exploring integrated transactions with Spring Boot. Happy coding!