Mastering Configuration Management in Spring Boot Applications
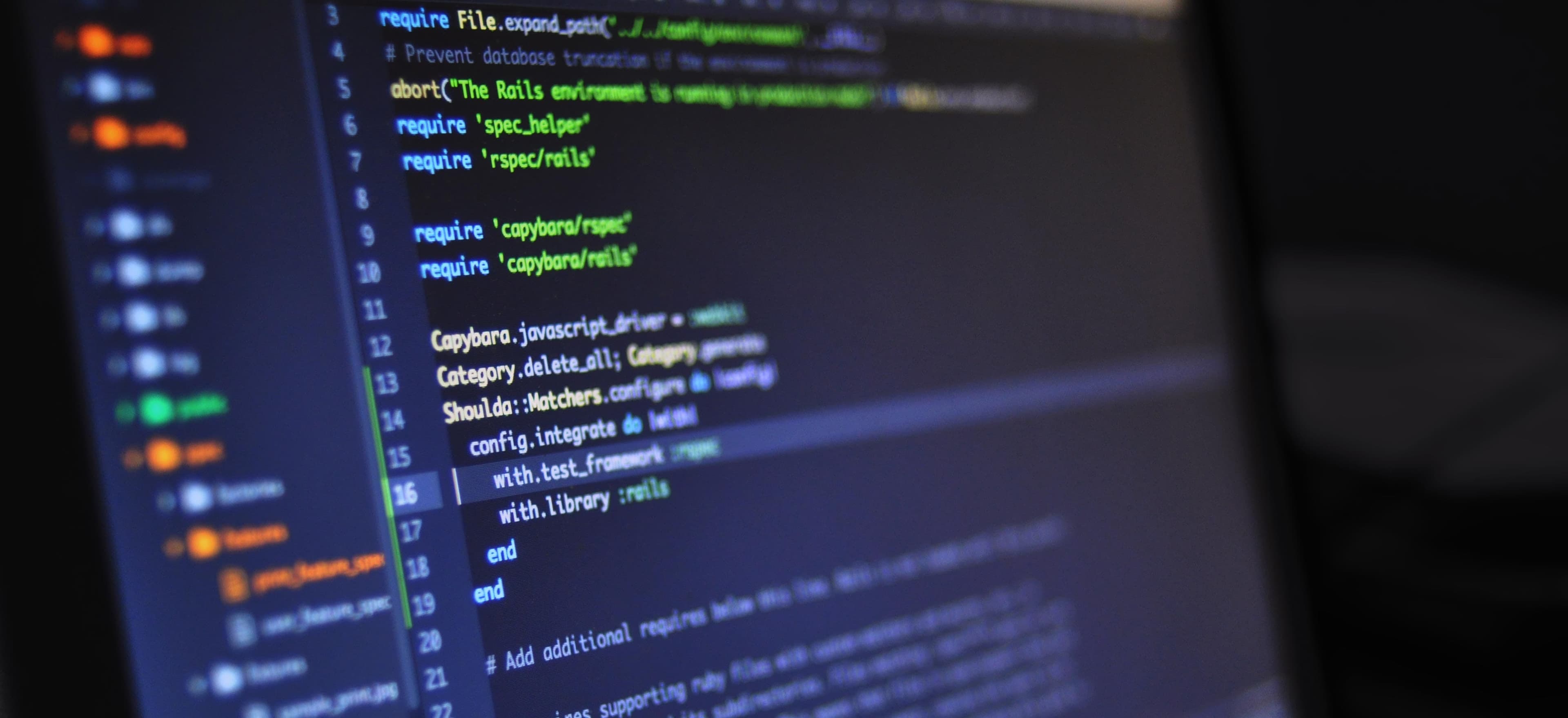
- Published on
Mastering Configuration Management in Spring Boot Applications
In modern software development, configuration management plays a pivotal role, especially in Spring Boot applications. A robust configuration management strategy allows developers to manage application settings effectively, ensuring that the right configurations are deployed in different environments - be it development, testing, or production.
Why Configuration Management is Crucial
Configuration management in Spring Boot ensures that your application behaves consistently across all environments. Proper configuration strategies help in:
- Separation of Concerns: Keep configuration separate from code.
- Environment-Specific Settings: Manage different settings for development, testing, and production seamlessly.
- Ease of Deployment: Simplify deployment processes by avoiding hardcoded values.
- Security: Protect sensitive information like API keys, database passwords, and more.
Let’s explore how to master configuration management in Spring Boot, utilizing Spring’s features effectively.
Spring Boot Configuration Files
Spring Boot supports various configuration files, primarily application.properties
or application.yml
files. These files define properties that your application uses.
Example of application.properties
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=secret
- Why is this important?: Centralizing your application settings makes them manageable and searchable. You can easily change ports or database connections without altering the core code.
Example of application.yml
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: root
password: secret
- YAML vs Properties: YAML is often preferred for its readability, especially when dealing with hierarchical data structures.
Profiles in Spring Boot
One of the standout features of Spring Boot is the ability to use profiles. This allows developers to define separate configurations for different environments.
Defining Profiles
You can define profiles in the same application.properties
or application.yml
file. For example:
# application.properties
# Default settings
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=secret
# Development settings
spring.profiles.active=dev
---
spring.datasource.url=jdbc:mysql://localhost:3306/devdb
spring.datasource.username=devuser
spring.datasource.password=devsecret
# Test settings
spring.profiles.active=test
---
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
spring.datasource.username=testuser
spring.datasource.password=testsecret
Why Use Profiles?
Using profiles simplifies the process of using multiple configurations based on the environment. This prevents code from being riddled with conditional statements checking for the current environment.
Activating Profiles
You can activate a profile via command line, environment variable, or within your code. To run your application with a specific profile, you can use:
spring.profiles.active=dev
Or set an environment variable in your terminal session:
export SPRING_PROFILES_ACTIVE=dev
External Configuration
Spring Boot allows you to keep your configuration outside of the packaged JAR or WAR file, which is a great practice for different environments. External configurations can be achieved using command-line arguments, environment variables, and files.
Command-Line Arguments
You can pass configurations directly from the command line when starting your application.
java -jar myapp.jar --spring.datasource.url=jdbc:mysql://localhost:3306/proddb --spring.datasource.username=produser --spring.datasource.password=prodsecret
- Why Use Command-Line Arguments?: It enables dynamic changes without modifying the code or redeploying the application.
Environment Variables
Spring Boot supports environment variables directly. You can define configurations as environment variables that override the properties defined in your application.
export SPRING_DATASOURCE_URL=jdbc:mysql://localhost:3306/proddb
Application Configurations in Different Locations
You can also specify an external configuration file location. For example, if your configuration file is in a specific directory:
java -jar myapp.jar --spring.config.location=/path/to/config/
Using @Value Annotation for Configuration Properties
In Spring Boot, the @Value
annotation is used to inject property values into your components. Here’s how you can utilize it:
Example Component
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Value("${spring.datasource.url}")
private String datasourceUrl;
public void printDatasourceUrl() {
System.out.println("Datasource URL: " + datasourceUrl);
}
}
- Why Use @Value?: It enables you to inject property values directly into your classes, simplifying access to configuration properties.
Configuration Properties Classes
For complex configurations, you can create a properties class annotated with @ConfigurationProperties
. This allows you to bind the properties to a strongly typed POJO.
Example of Configuration Properties
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "app")
public class AppProperties {
private String name;
private String version;
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
}
Loading from application.properties
app.name=My Application
app.version=1.0.0
- Why Use Configuration Properties Classes?: It enhances the clarity of your code and allows for a robust way of managing related configuration properties in one place.
Security Considerations
While configuration management is crucial, so is security. Avoid hardcoding sensitive data directly into the configuration files. Instead, consider using properties on the Spring Cloud Config server or secrets management tools such as HashiCorp Vault.
Example of Using External Secrets
Spring Cloud Config can load configurations from external sources. Here’s a typical application properties setup:
spring:
cloud:
config:
server:
git:
uri: https://github.com/myorg/config-repo
This way, you separate the configuration completely, enhancing security.
Closing Remarks
Mastering configuration management in Spring Boot applications is pivotal for creating scalable, maintainable, and secure applications. By adopting best practices around configuration files, profiles, external configurations, and ensuring the separation of sensitive data, you set your application up for success.
For more insights on Spring Boot and Configuration Management, consider checking the Spring Boot Documentation and delve into Spring Cloud Config for advanced configuration management solutions.
By implementing these strategies, you will not only enhance your Spring Boot applications but also simplify the complexities associated with managing configurations across various environments. Happy coding!
Checkout our other articles