Optimizing REST API for Efficient Collection Sorting
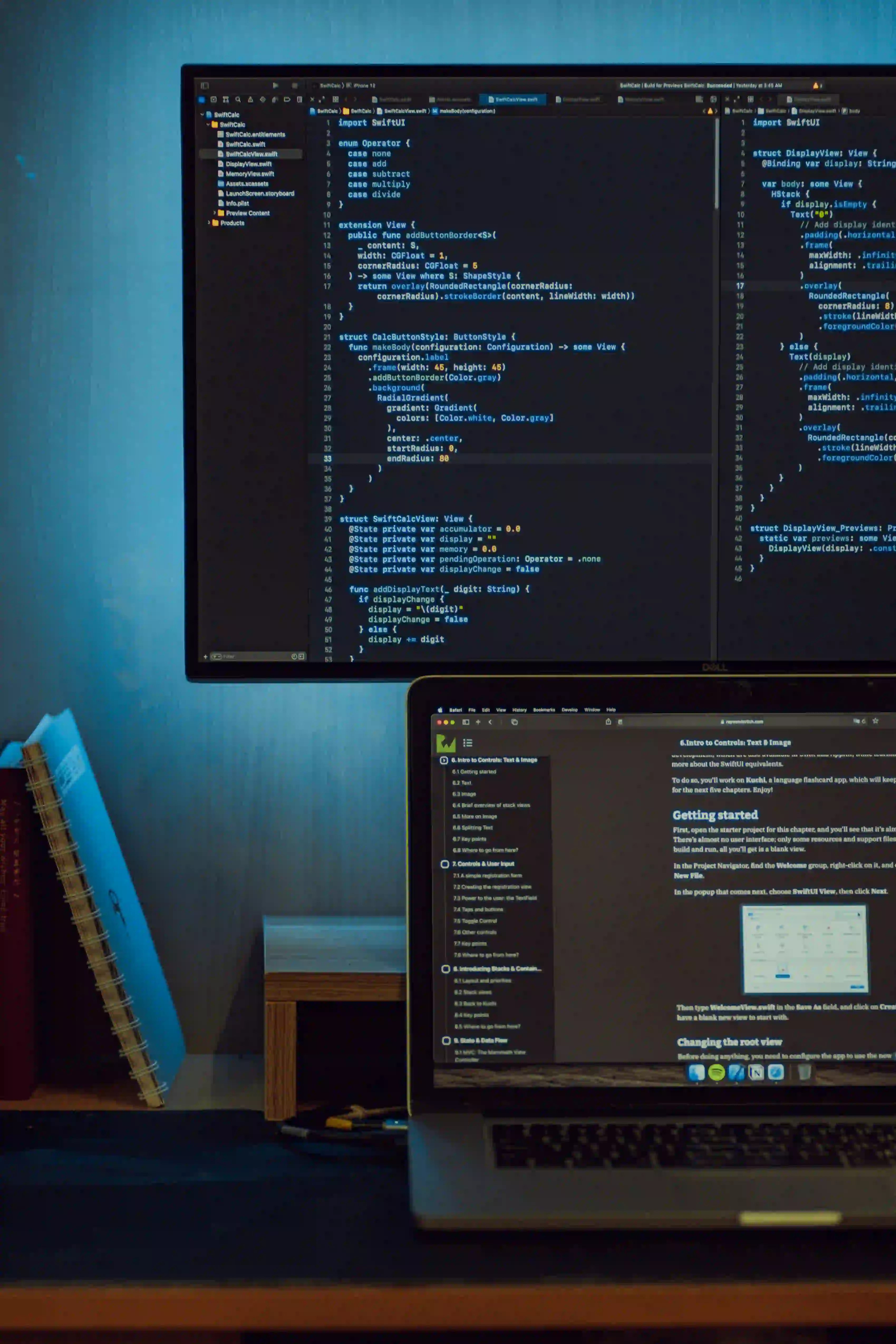
Optimizing REST API for Efficient Collection Sorting
In the world of web development, REST APIs are the backbone that connects client applications to server functionality. With a growing trend towards cloud-based solutions and an emphasis on performance, it is paramount for developers to implement efficient methodologies for data retrieval and manipulation. One essential aspect of this is sorting—especially when dealing with large datasets. In this post, we’ll explore the best practices for optimizing REST APIs for efficient collection sorting, supplemented with code snippets and solid reasoning.
Understanding REST and Sorting
REST (Representational State Transfer) APIs allow clients to interact with resources using standard HTTP methods. Sorting serves as a fundamental operation that enhances the accessibility of data. But it is crucial to note that inefficient sorting can severely degrade performance, particularly for large datasets.
Why Optimize Sorting?
- Performance: Slow response times lead to a poor user experience. Users today expect quick data retrieval.
- Bandwidth: Transmitting large datasets without sorting can waste bandwidth.
- Scalability: A well-optimized sorting mechanism ensures that your application can handle increased load gracefully.
Website performance for APIs can be assessed using tools such as Apache Bench or Postman. These tools help evaluate the impact of your sorting operations.
Best Practices for Sorting in REST APIs
1. Use Query Parameters for Sorting
The most common approach for sorting in REST APIs is passing sorting parameters in the query string. For instance:
GET /api/items?sort=price&order=asc
Why this works: Using query parameters isolates the sorting functionality, making it flexible and easily adjustable.
Example in Java with Spring Boot
Here is how you can implement this in a Spring Boot application:
@GetMapping("/items")
public ResponseEntity<List<Item>> getItems(
@RequestParam(defaultValue = "id") String sort,
@RequestParam(defaultValue = "asc") String order) {
List<Item> items = itemService.getAllItems();
Comparator<Item> comparator;
if (sort.equals("price")) {
comparator = Comparator.comparing(Item::getPrice);
} else {
comparator = Comparator.comparing(Item::getId);
}
if (order.equals("desc")) {
comparator = comparator.reversed();
}
items.sort(comparator);
return new ResponseEntity<>(items, HttpStatus.OK);
}
Commentary:
In this code, the getItems
method retrieves a list of items and sorts them based on the query parameters provided. The use of comparators allows for dynamic sorting based on various fields, fostering reusability.
2. Implement Pagination alongside Sorting
Sorting large datasets without pagination can lead to performance bottlenecks. Implementing pagination ensures that clients can retrieve data in manageable chunks:
GET /api/items?sort=price&order=asc&page=1&size=10
Why pagination matters: It reduces the load on both server and client, enabling efficient data transfers.
Example with Pagination in Spring Boot
You can enhance our previous example to include pagination:
@GetMapping("/items")
public ResponseEntity<Page<Item>> getItems(
@RequestParam(defaultValue = "id") String sort,
@RequestParam(defaultValue = "asc") String order,
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Pageable pageable = PageRequest.of(page, size, Sort.by(order.equals("asc") ? Sort.Direction.ASC : Sort.Direction.DESC, sort));
Page<Item> itemsPage = itemService.getItems(pageable);
return new ResponseEntity<>(itemsPage, HttpStatus.OK);
}
Commentary: The use of the PageRequest
class from Spring Data simplifies the implementation of pagination and sorting simultaneously. A Page<Item>
returns additional metadata like the total number of pages, which further enhances the client’s experience.
3. Leverage Database Capabilities
When dealing with massive datasets, it may be more efficient to let the database handle sorting rather than retrieving all data and sorting in applications. For example, using SQL queries can significantly accelerate the process.
SELECT * FROM items ORDER BY price ASC LIMIT 10 OFFSET 0;
Why use the database?: Databases are optimized for such operations and can often leverage indexes to speed up sorting.
Integrating with JPA in Spring Boot
You can use Spring Data JPA to take full advantage of the above query:
public interface ItemRepository extends JpaRepository<Item, Long> {
Page<Item> findAll(Sort sort, Pageable pageable);
}
Commentary: By defining your repository correctly, you can tailor your queries to be efficient by taking advantage of database indices for the attributes being sorted.
4. Caching Sorted Results
Implementing a caching mechanism helps speed up data retrieval times. Caching commonly accessed sorted collections allows you to serve faster responses.
@Cacheable(value = "sortedItems", key = "#sort + #order + #page")
public List<Item> getSortedItems(String sort, String order, int page) {
// method implementation
}
Why caching is essential: Caching reduces unnecessary database hits, thus saving on latency and resources.
The Last Word
Optimizing REST APIs for efficient collection sorting is crucial to maintain performance standards as your application grows. By utilizing query parameters for sorting, implementing pagination, leveraging database capabilities, and caching results, you can effectively improve user experience and resource management.
Consider the techniques discussed in this blog not just as standalone solutions, but as components of a comprehensive approach to API design. Always profile your application and adopt improvements iteratively.
Now you have the tools and understanding to make your REST API not only efficient but also responsive to the needs of your users. For more in-depth discussions on REST API principles and best practices, check out RESTful API Design.
With these insights, you should be well-equipped to tackle the challenge of sorting in REST APIs head-on. Happy coding!