Common Pitfalls in Java EE 7 Batch Property Passing
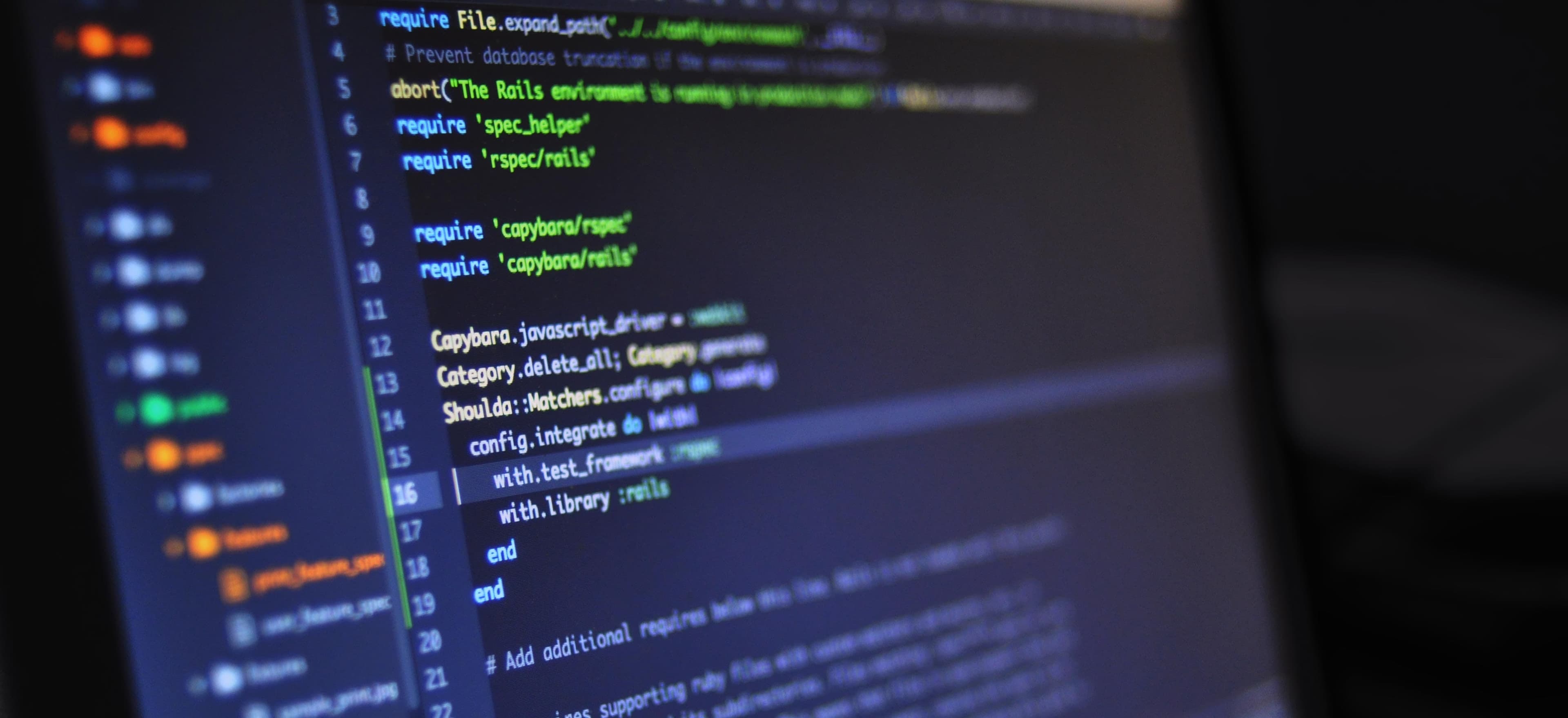
- Published on
Common Pitfalls in Java EE 7 Batch Property Passing
Java EE (now known as Jakarta EE) is a popular platform for building enterprise-grade applications. Among its many features, the Batch Processing specification allows developers to handle large volumes of data efficiently. However, the implementation can come with its own set of challenges. In this blog post, we will explore common pitfalls with batch property passing in Java EE 7 and how to avoid them.
Understanding Batch Processing in Java EE 7
Before we delve into the pitfalls, it is essential to grasp the basics of batch processing in Java EE. The Java EE 7 Batch specification provides a mechanism for processing large sets of data through a well-defined framework, which includes defining jobs, partitions, and chunk processing.
Key components of the batch processing architecture include:
- Jobs: Defined execution paths that process a specific set of data.
- Steps: Individual processing steps that define the actual functions performed on the data.
- Operators: Used to manage job execution and control flow.
For a deeper understanding, refer to Java EE Batch Processing Docs.
Pitfall 1: Misunderstanding Batch Job Execution Context
When executing batch jobs, a common mistake is misunderstanding the execution context. Each job has its own context, but properties can be passed in several ways—via job parameters, job execution context, or step context.
Consider the following code snippet:
@BatchProperty
private String inputFile;
public void processJob() {
// Job execution context can be used to retrieve the input filename, if set correctly.
String inputFile = (String) jobExecution.getExecutionContext().get("inputFile");
// Process input file...
}
Why This Matters
If you forget to set the property at the job execution context, you may end up with null values or defaults. Always ensure you're correctly assigning properties before job execution.
Pitfall 2: Not Using the Right Data Type for Properties
Another common error is using the inappropriate data type for batch job properties. Properties can be passed as strings, but they may represent different data types (like integers or booleans).
For instance:
@BatchProperty
private String threshold;
public void executeStep() {
// Attempting to process threshold without conversion
int thresholdValue = Integer.parseInt(threshold); // Throws NumberFormatException if threshold is not correctly set
// Continue processing...
}
Why This Matters
By using the incorrect data type, you risk runtime exceptions that halt your job execution. To prevent this, always validate and convert incoming properties as needed.
Pitfall 3: Overlooking Property Scope in Jobs and Steps
In Java EE batch processing, properties have different scopes depending on whether they are set at the job, step, or partition level. A common oversight is expecting properties set at one scope to be available within another.
For example:
@BatchProperty
private String partitionSize;
@Step
public void processPartition() {
// This may result in null if partitionSize isn't set at the job level.
System.out.println("Processing partition of size: " + partitionSize);
}
Why This Matters
Properties scoped to jobs will not be found at the step level unless explicitly passed. To ensure proper scope management, always review how you're defining and accessing properties in your batch jobs.
Pitfall 4: Not Using the Correct Java EE Annotations
Java EE provides several annotations for configuring batch jobs and steps, but it is crucial to use these annotations correctly. Mislabeling or misusing annotations can lead to jobs not being executed as expected.
Here’s an example of common misconfiguration:
@Job
@BatchJob
public class DataProcessingJob {
// Incorrectly annotated Job class
}
Why This Matters
Using the wrong annotations leads to runtime errors and incomplete job execution. Always consult the official Java EE specifications to confirm you're using the appropriate annotations.
Pitfall 5: Failing to Handle Exceptions Properly
Exception handling is a vital part of any batch processing job. Ignoring this aspect can result in partial data processing or corrupt output.
Consider this approach:
public void process() {
try {
// Data processing logic
} catch (Exception e) {
// Failing silently can lead to undetected issues
}
}
Why This Matters
By catching exceptions without adequate logging or recovery, you put your job integrity at risk. Always log exceptions adequately and consider using a retry logic or compensation pattern for failed executions.
Best Practices to Avoid Common Pitfalls
To circumvent the pitfalls discussed, adhere to these best practices:
-
Understand Context Scopes: Make sure you know where properties are set and how they can be accessed.
-
Always Validate Data Types: Use utility methods to convert and validate property types before utilizing them.
-
Properly Annotate Your Classes: Use the correct annotations to ensure job definitions and executions are aligned with Java EE specifications.
-
Implement Comprehensive Error Handling: Utilize logging frameworks like SLF4J or Log4J to capture and analyze specific error contexts.
-
Test Thoroughly: Implement unit tests and integration tests to cover various execution scenarios and verify property passing under different conditions.
A Final Look
Batch processing in Java EE 7 can greatly enhance data handling capabilities but comes with its pitfalls. By understanding the potential challenges associated with batch property passing and adhering to best practices, you can build more robust and efficient applications.
For more insights into Java EE batch processing, consider checking out some resources such as DZone and Baeldung.
Stay informed, keep coding, and avoid those common pitfalls!