Optimizing JavaFX for Mobile: Common Pitfalls to Avoid
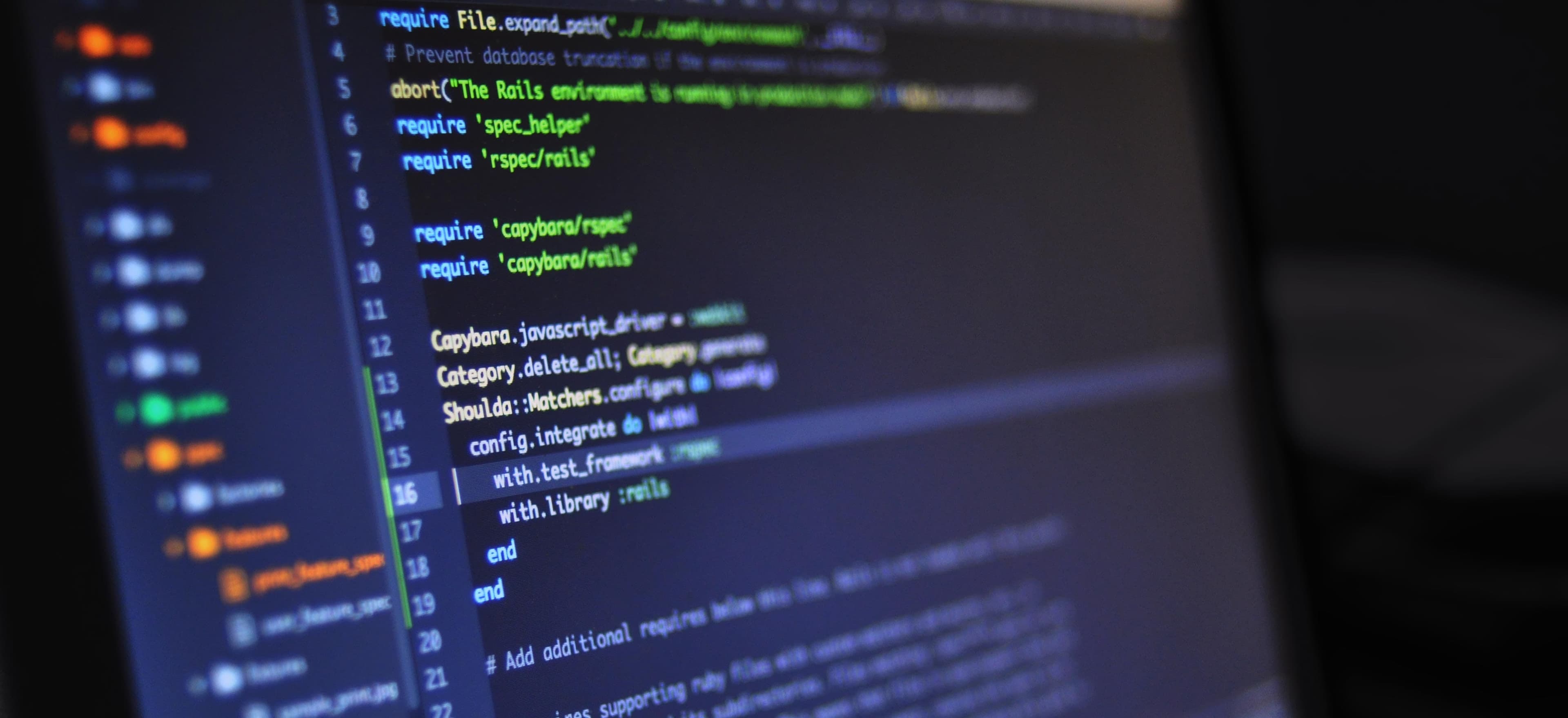
- Published on
Optimizing JavaFX for Mobile: Common Pitfalls to Avoid
JavaFX is a powerful framework that facilitates the creation of rich client applications. While the possibilities it affords desktop developers are vast, extending those capabilities to mobile devices requires careful consideration. In this blog post, we will explore common pitfalls encountered when optimizing JavaFX for mobile, and how you can effectively navigate around them for a smoother mobile experience.
Understanding JavaFX on Mobile
JavaFX caters to the needs of developers building feature-rich applications with multimedia and graphics capabilities. When it comes to mobile app development, however, its behavior and performance can differ from traditional desktop applications. Recognizing these differences is the first step in avoiding potential pitfalls.
Pitfall #1: Overcomplicating the UI
One of the most glaring issues developers face is overcomplicating the user interface (UI). Mobile screens are significantly smaller than desktop displays, which means more intricate UIs can result in cluttered, confusing applications.
Solution
Keep your UI simple and intuitive. Prioritize essential components and avoid overwhelming users with too many options at once. Use layout managers effectively to ensure that your UI is adaptive to various screen sizes.
VBox root = new VBox();
root.setSpacing(10); // Space between elements
root.setAlignment(Pos.CENTER); // Center align items
Button button = new Button("Submit");
root.getChildren().add(button);
In the example above, we’ve used a VBox
layout to stack elements vertically with proper spacing. This keeps the UI organized, allowing users to focus on one task at a time.
Pitfall #2: Ignoring Touchscreen Interactions
Desktop applications commonly rely on mouse interactions. Mobile apps, however, depend heavily on touchscreen gestures. Ignoring these interactions can lead to poor user experiences.
Solution
Implement touch-optimized controls. JavaFX provides various nodes that can handle touch events, such as onTouchPressed
, onTouchReleased
, and onTouchMoved
.
button.setOnTouchPressed(event -> {
System.out.println("Button pressed!");
button.setStyle("-fx-background-color: lightblue;"); // Change color on press
});
Using touch events allows for a more engaging experience. With responsive feedback on touch gestures, users are more likely to enjoy your app.
Pitfall #3: Excessive Use of Graphics and Complex Animations
While JavaFX is known for its robust graphics capabilities, excessive use of graphics or complex animations can hinder performance on mobile devices. Many developers underplay the performance cost associated with these features.
Solution
Optimize your graphics and animations. Use lightweight graphics when possible and limit the complexity of animations. You can also reduce frame rates for animations specifically designed for mobile.
RotateTransition rotateTransition = new RotateTransition(Duration.seconds(2), circle);
rotateTransition.setByAngle(360);
rotateTransition.setCycleCount(Timeline.INDEFINITE);
rotateTransition.setInterpolator(Interpolator.LINEAR);
In the code snippet above, we're creating a smooth, continuous rotation animation for a circle. By ensuring animations utilize efficient interpolators and durations, you can keep the experience fluid without draining resources.
Pitfall #4: Inadequate Screen Orientation Handling
Mobile devices offer various orientations (portrait and landscape). A common error is failing to adapt the application when the device's orientation changes. Ignoring this fact can badly hinder usability.
Solution
Listen for orientation changes and dynamically adjust your layout. You can use the Scene
object to refresh the layout based on orientation.
scene.widthProperty().addListener((observable, oldValue, newValue) -> {
if (newValue.doubleValue() > scene.getHeight()) {
System.out.println("Landscape mode");
// Adjust your layout for landscape
} else {
System.out.println("Portrait mode");
// Adjust your layout for portrait
}
});
This approach allows you to respond proactively to changes in orientation, ensuring that your app’s layout remains user-friendly regardless of how the device is held.
Pitfall #5: Neglecting Performance Profiling
Performance profiling is an essential aspect often overlooked by developers. Failing to measure your application's performance can lead to sluggish apps that frustrate users.
Solution
Use profiling tools to monitor performance metrics. Java Mission Control and VisualVM are great tools for analyzing your JavaFX app's memory consumption and CPU usage. By identifying bottlenecks, you can optimize relevant areas.
Profiling Example
Suppose you find that certain methods are taking longer to execute than expected. Here’s a simple way to analyze performance:
long startTime = System.currentTimeMillis();
// execute method
long endTime = System.currentTimeMillis();
System.out.println("Execution time: " + (endTime - startTime) + " ms");
By leveraging profiling, you can ensure that your application runs smoothly even under mobile constraints.
Final Thoughts
Optimizing JavaFX for mobile is not a trivial task. However, by avoiding these common pitfalls, establishing good practices, and maintaining a user-centered design philosophy, you can create fluid, engaging applications suitable for mobile platforms.
For more in-depth discussions on developing Java applications, check out JavaFX Documentation, and consider diving into community forums for real-world tips from fellow developers.
By keeping your designs well-structured, intuitive, and optimized for performance from the ground up, you can deliver a seamless mobile experience that will delight users and stand the test of time.
Remember, users judge applications not just by their functionality but also by the quality of their interaction experience. Happy coding!