Mastering Javadoc Extraction: Common Pitfalls to Avoid
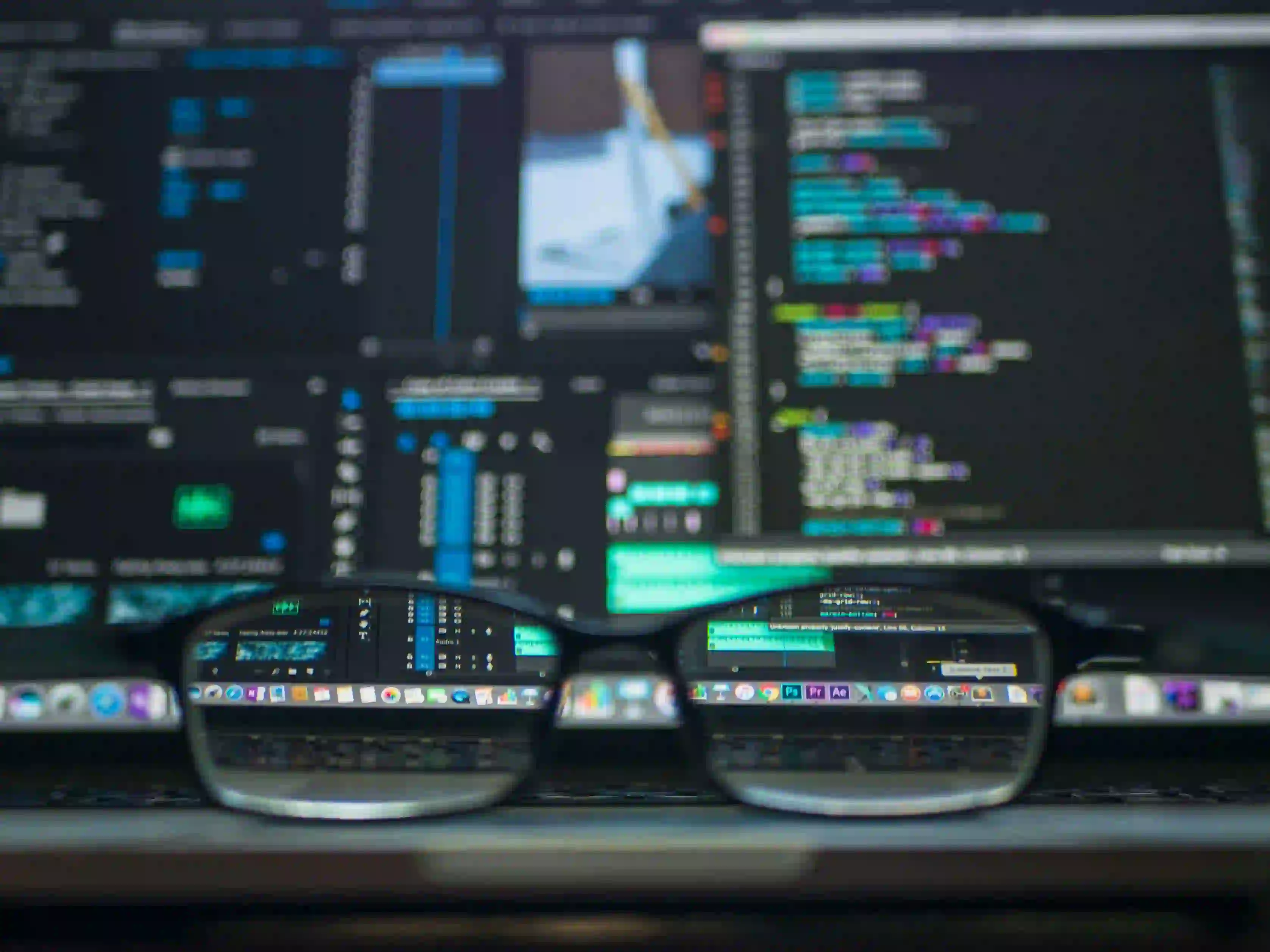
Mastering Javadoc Extraction: Common Pitfalls to Avoid
When developing Java applications, documenting your code effectively is crucial for maintainability and collaboration. Javadoc, the tool that generates API documentation from Java source code, is a powerful ally in this endeavor. However, improper usage can lead to common pitfalls that might undermine your efforts. This blog post will explore effective Javadoc extraction techniques, pitfalls to avoid, and best practices to ensure your documentation is as beneficial as your code.
What is Javadoc?
Javadoc is a documentation tool included in the JDK (Java Development Kit) that converts comments in your Java code into HTML documentation. By adding structured comments, you enable APIs to be self-explanatory and easier to use by anyone who interacts with them.
Basic Syntax of Javadoc
Before diving into common pitfalls, let’s quickly review the basic syntax of Javadoc comments:
/**
* This is a Javadoc comment.
* It can span multiple lines.
*
* @param paramName Description of the parameter.
* @return Description of the return value.
* @throws ExceptionType Description of the exception.
*/
public ReturnType methodName(ParameterType paramName) throws ExceptionType {
// Method logic
}
Common Pitfalls in Javadoc Extraction
1. Missing Javadoc Comments for Public APIs
One of the biggest mistakes developers make is neglecting to write Javadoc comments for public classes and methods. Javadoc is essential for public APIs since those are used by other developers.
Example of a missing Javadoc comment:
public class User {
public String getName() {
return name;
}
}
Solution: Always document your public API.
/**
* Represents a user in the application.
*/
public class User {
/**
* Gets the name of the user.
*
* @return the name of the user.
*/
public String getName() {
return name;
}
}
2. Inconsistent Javadoc Tags
Using inconsistent or nonexistent Javadoc tags can confuse users of the API. For example, failing to include @param
, @return
, or @throws
tags can leave your API users guessing.
Incorrect Usage:
/**
* Calculates the age.
*/
public int calculateAge(int birthYear) {
return currentYear - birthYear;
}
Correct Usage:
/**
* Calculates a person's age based on the provided birth year.
*
* @param birthYear The year of birth.
* @return The calculated age.
*/
public int calculateAge(int birthYear) {
return currentYear - birthYear;
}
3. Overly Verbose or Vague Documentation
Being overly descriptive can lead to ambiguity in your documentation. On the other hand, being too vague can make your documentation useless. Striking a balance is crucial.
Overly Verbose Example:
/**
* This method takes an integer parameter and performs a calculation
* that is intended to determine the sum of numbers which could lead
* to another integer and hence the resulting value is what we provide.
*
* @param number The integer parameter for calculation.
* @return The final calculated integer value post the summation outcome.
*/
public int complicatedMethod(int number) {
// implementation
}
Concise Example:
/**
* Calculates the sum of the provided number and a predefined constant.
*
* @param number The integer to sum with.
* @return The resulting sum.
*/
public int calculateSum(int number) {
return number + PREDEFINED_CONSTANT;
}
4. Ignoring Exception Documentation
Documentation for exceptions thrown by methods is essential, especially for methods that involve multiple risk factors. Ignoring this can lead to run-time issues and confusion.
Lacking Exception Documentation:
/**
* Divides two numbers.
*/
public double divide(int numerator, int denominator) {
return numerator / denominator;
}
Including Exception Documentation:
/**
* Divides two numbers.
*
* @param numerator The number to be divided.
* @param denominator The number to divide by.
* @return The result of the division.
* @throws IllegalArgumentException If the denominator is zero.
*/
public double divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero.");
}
return numerator / denominator;
}
5. Failing to Update Javadoc
Code evolves, and often, what was true when you wrote your Javadoc is no longer valid. Failing to update Javadoc can lead to more confusion than clarity.
Outdated Example:
/**
* Sets the user's age. Age must be a positive integer.
*
* @param age The user's age.
*/
public void setAge(int age) {
this.age = age;
}
Updated version (If the method now allows for negative values):
/**
* Sets the user's age. Can now accept negative integers for future
* changes in the application, but verification is still important.
*
* @param age The user's age, including the option to pass in negative values.
*/
public void setAge(int age) {
this.age = age;
}
Best Practices for Writing Javadoc
- Be Clear and Concise: Always aim for clarity. Brevity is essential; avoid unnecessary jargon.
- Use Proper Tags: Always use
@param
,@return
, and@throws
where applicable to facilitate understanding. - Update Regularly: Regularly review and keep documentation updated as the code base evolves.
- Focus on the User: Write your documentation from the perspective of the user. Consider what information they need to use your API effectively.
- Add Examples: If applicable, add usage examples in your documentation. This enhances understanding significantly.
Final Considerations
Mastering Javadoc extraction means being conscious of common pitfalls and actively working to avoid them. Well-documented code can save time, reduce collaboration friction, and improve overall code quality. By following best practices and regularly updating documentation, you can ensure that your code remains understandable and maintainable for years to come.
For further reading, consider the official Javadoc documentation, which provides deeper insights into advanced features and formatting options available in Javadoc.
By integrating these practices into your workflow, you will greatly enhance the usability and longevity of your Java applications. Happy coding!