Unbelievable Java Facts That Will Change Your Coding Perspective
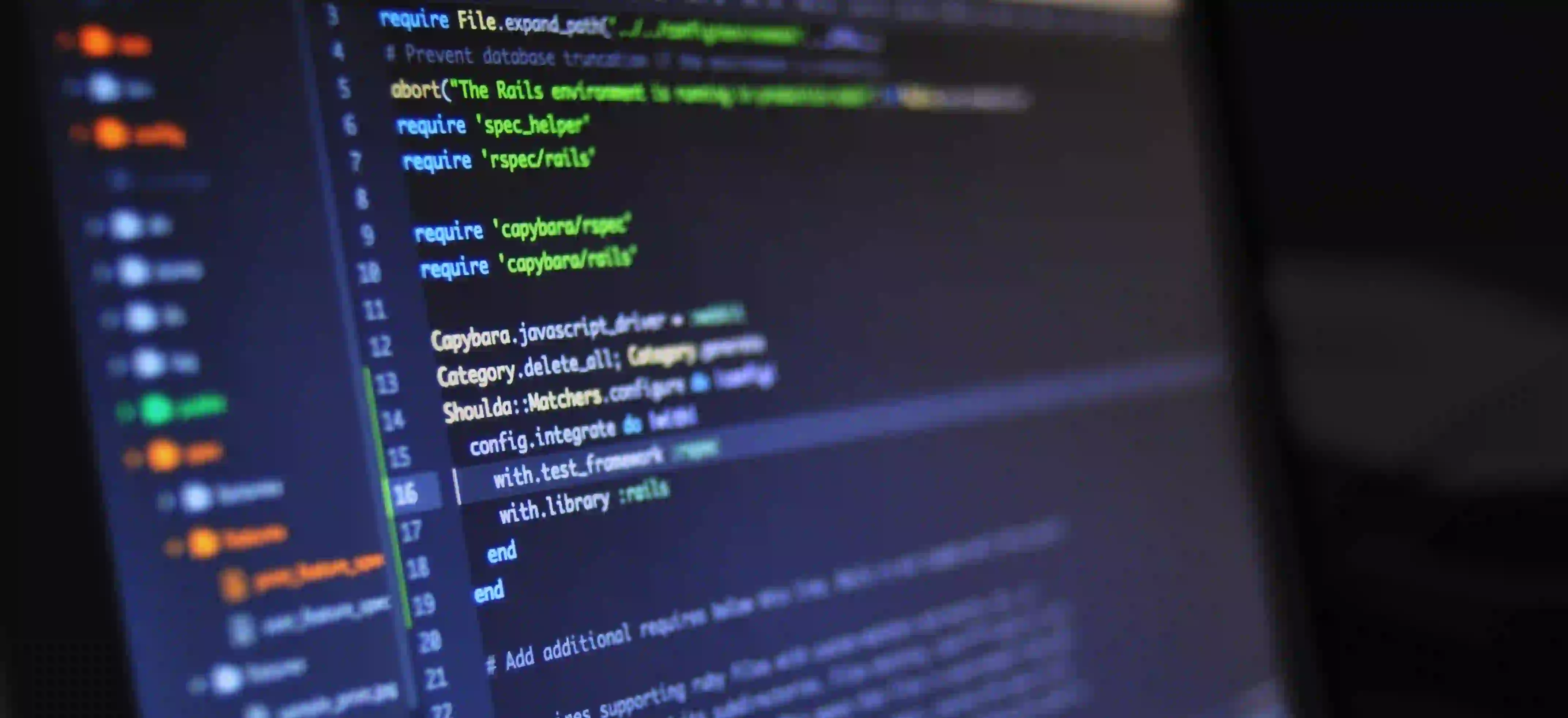
Unbelievable Java Facts That Will Change Your Coding Perspective
Java is one of the most popular programming languages in the world. It has powered everything from corporate applications to mobile apps, games, and even large-scale web services. Despite its widespread use, many developers still have misconceptions about Java or are unaware of some incredible features it offers. In this blog post, we will explore some unbelievable Java facts that can change your coding perspective and possibly improve your programming practices.
1. Java Was Developed in Just 18 Months
Yes, you read that right! The first version of Java was developed in just 18 months by a small team at Sun Microsystems, led by James Gosling. Introduced in 1995, what started as a project for interactive television has now become a staple in software development.
Why is this noteworthy? Understanding that a language can be thoughtfully designed and developed in a short time span underscores the importance of team dynamics and focused objectives in software creation. It also signifies the potential that can be unlocked with the right vision.
2. Write Once, Run Anywhere (WORA)
One of Java's most famous slogans is "Write Once, Run Anywhere" (WORA). This means that Java code can be executed on any device that has a Java Virtual Machine (JVM), regardless of the underlying architecture.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this simple code snippet, you can see how one piece of Java code can run on any machine with a JVM. The JVM interprets the compiled Java bytecode (which is platform-independent) and converts it into machine code specific to the OS and underlying architecture. This abstraction layer is a game-changer for developers building cross-platform applications.
3. Java is Everywhere
Java plays a critical role in various fields:
- Web Development: Frameworks like Spring MVC and JavaServer Faces (JSF) make web application development robust and scalable.
- Mobile Applications: Android development primarily uses Java, enabling developers to create feature-rich mobile applications.
- Enterprise Solutions: Java is the backbone for many enterprise-level applications due to its security features and scalability.
- Big Data and Analytics: Technologies like Apache Hadoop, Apache Kafka, and Apache Spark are Java-based, widely used in processing large data sets.
This versatility means that choosing Java often opens many career opportunities and facilitates seamless integration across varied systems.
4. Rich Ecosystem of Libraries and Frameworks
Java has a rich ecosystem of libraries and frameworks, which gives developers the tools they need to build more robust applications without reinventing the wheel.
For example:
- Spring Framework: Simplifies Java development by providing comprehensive infrastructure support.
- Hibernate: Handles database operations flexibly and powerfully, reducing the boilerplate code significantly.
- Apache Commons: A collection of reusable Java components that can simplify common programming tasks.
Using frameworks not only speeds up development time but also promotes best practices, allowing developers to focus more on business logic rather than basic coding tasks.
5. Garbage Collection is a Boon to Developers
Java uses an automatic garbage collection (GC) system, which means that developers do not have to manually manage memory allocation and deallocation.
Consider this simple program:
public class GarbageCollectionExample {
public static void main(String[] args) {
String str = new String("Hello");
str = null; // The string object can now be garbage collected.
}
}
When the str
variable is set to null
, the associated object becomes eligible for garbage collection. The JVM automatically reclaims this memory, preventing memory leaks and making Java applications more stable. Nevertheless, it's essential to understand its workings and limitations, as poor memory management can still degrade performance.
6. Java is Backward Compatible
One of Java's strong suits is its backward compatibility. This means that newer versions of the Java language can run code written in older versions without any issues.
// Old code from Java 1.0
class LegacyCode {
void showMessage() {
System.out.println("This is legacy code.");
}
}
// New code in Java 17
class ModernCode extends LegacyCode {
void showMessage() {
System.out.println("This is modern code.");
}
}
In this example, the older LegacyCode
class can be utilized in codebases built with newer versions of Java. This characteristic allows organizations to upgrade their applications incrementally, reducing risks and ensuring longevity.
7. Asynchronous Programming with CompletableFuture
With the adoption of the CompletableFuture
class introduced in Java 8, asynchronous programming has become more manageable and flexible.
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a long-running computation
return "Result calculated";
});
// This will run after the computation is complete
future.thenAccept(result -> System.out.println(result));
}
}
This code illustrates how CompletableFuture
runs asynchronously, allowing your application to perform other tasks without waiting for the computation to finish. Asynchronous programming enhances responsiveness and performance, especially in applications requiring real-time processing.
8. Design Patterns Are Native to Java
Java heavily utilizes design patterns, which are proven solutions to common problems in software design.
Examples of Popular Design Patterns in Java:
- Singleton: Ensures a class has only one instance and provides a global point of access.
- Observer: Defines a one-to-many dependency between objects to allow for automatic updates.
- Factory Method: Defines an interface for creating an object, allowing subclasses to alter the type of created objects.
Implementing these patterns appropriately can lead to more maintainable code and facilitate collaboration within a team by providing a common framework for developers.
9. Strong Typing Improves Code Quality
Java is a statically typed language, meaning that variable types are explicitly declared and checked at compile-time.
int number = 10; // Declaration with type explicitly defined
String message = "Welcome to Java!";
This strong typing approach helps catch errors early in the development process, resulting in fewer runtime errors. Furthermore, it improves code readability, making it easier for developers to understand codebases, thus enhancing collaboration.
10. Java Community is Thriving
Last but definitely not least, the Java community is vibrant and expansive. From forums and user groups to conferences like Oracle Code One, a wealth of resources can help developers at all skill levels.
Getting involved with the community not only provides learning opportunities but also opens up professional networks.
My Closing Thoughts on the Matter
These unbelievable Java facts highlight the language's robust and versatile nature, showcasing why Java continues to thrive in the world of programming. Understanding these aspects can provide deeper insights into Java's architecture and benefits, ultimately enhancing your coding practices.
Whether you're a seasoned Java developer or just starting your coding journey, embracing these core features will broaden your perspective and improve your coding agility. So, keep coding, keep learning, and embrace the possibilities that Java presents!
For a deeper dive into Java and its advanced features, consider exploring Oracle's official Java documentation or joining the Java community on platforms like Stack Overflow and GitHub.
Happy coding!