Top Java Code Quality Tools: Boost Your Development Today!
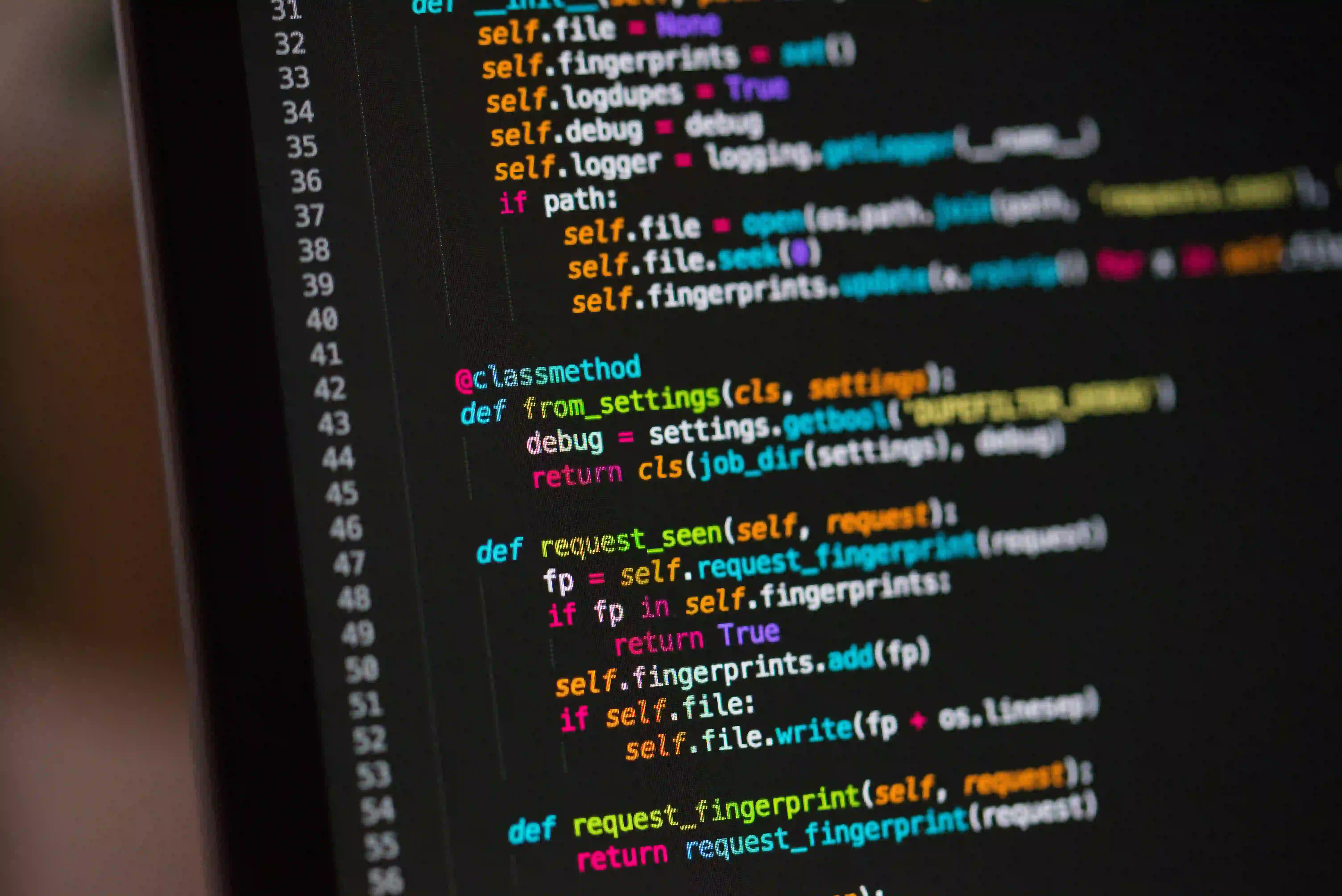
Top Java Code Quality Tools: Boost Your Development Today!
In the world of software development, code quality is paramount. The cleaner and more efficient your code, the easier it becomes to maintain and extend. Java, being one of the most popular programming languages, has a rich ecosystem of tools dedicated to enhancing code quality. In this post, we will explore some of the best Java code quality tools available. We will discuss how these tools can improve your development process and provide code snippets that demonstrate their usage.
Why Code Quality Matters
Before diving into the tools, it’s essential to understand why code quality matters. High-quality code results in:
- Reduced Bugs: Clean code is often more accessible to read, which translates to fewer mistakes.
- Improved Maintenance: Code that adheres to best practices is easier to debug and modify.
- Enhanced Collaboration: When multiple developers work on the same codebase, consistency is key.
- Better Performance: Well-optimized code runs faster and is generally more efficient.
Feeling intrigued? Let’s explore the tools that can help you achieve better code quality in your Java projects.
1. SonarQube
SonarQube is one of the most popular tools for continuous inspection of code quality. It helps developers manage code quality by tracking various metrics such as bugs, code smells, vulnerabilities, and coverage.
Key Features:
- Multi-language analysis: Supports Java and many other languages.
- Reporting: Provides actionable insights and prioritizes which issues to fix.
- Integration: Works well with CI/CD systems like Jenkins, GitLab, and others.
Example Integration
Here’s how you can set up SonarQube for your Java project:
-
Install SonarQube: Follow the installation guide to get SonarQube running locally or on a server.
-
Create a Project: Navigate to the dashboard and create a new project.
-
Add SonarQube Properties:
# sonar-project.properties
sonar.projectKey=my-java-project
sonar.sources=src/main/java
sonar.java.binaries=target/classes
Why Use SonarQube?
SonarQube provides you with a comprehensive overview of your code's health. The actionable insights make it easier to prioritize fixes, helping teams maintain high code quality without excessive overhead.
2. Checkstyle
Checkstyle is a development tool that helps programmers write Java code that adheres to a coding standard. It can help reduce the number of style-related bugs in your code.
Key Features:
- Extensible Configuration: You can define your coding standards.
- Code Formatting: Ensures consistency across the codebase.
- Integration: Easily integrates with build tools like Maven and Gradle.
Example Usage
To integrate Checkstyle into a Maven project, simply add the plugin configuration in your pom.xml
:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.2.0</version>
<configuration>
<configLocation>checkstyle.xml</configLocation>
<charset>UTF-8</charset>
<includeTestSourceDirectory>true</includeTestSourceDirectory>
</configuration>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
Why Use Checkstyle?
Checkstyle consistently enforces coding standards, ensuring that all developers follow agreed-upon practices. This leads to cleaner code and improved readability.
3. PMD
PMD is a static code analysis tool that scans Java source code and identifies potential issues such as unused variables, empty catch blocks, and more.
Key Features:
- Rule Sets: Comes with default rules but allows you to create custom rules.
- Reports: Generates easy-to-read reports highlighting code issues.
- Integrations: Works with various IDEs and build tools.
Example of a PMD Rule Configuration
You can customize PMD using a rule set XML, as shown below:
<ruleset xmlns="http://pmd.sourceforge.net/ruleset_2_0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://pmd.sourceforge.net/ruleset_2_0 http://pmd.sourceforge.net/ruleset_2_0.xsd">
<description>
My custom PMD ruleset
</description>
<rule ref="rulesets/java/basic.xml/UnusedImports" />
<rule ref="rulesets/java/braces.xml/EmptyBlock" />
</ruleset>
Why Use PMD?
By identifying potential issues before code goes into production, PMD can significantly reduce the time spent on debugging. Its ability to customize rules makes it adaptable to various projects.
4. SpotBugs
SpotBugs is a tool that finds bugs in Java programs by inspecting bytecode. It is the successor to the popular FindBugs and offers many additional features.
Key Features:
- User-Friendly: Provides a clean GUI for understanding bugs in your code.
- Comprehensive Analysis: Includes a wide array of bug detectors.
- Integration: Compatible with build tools and CI/CD setups.
Example Usage
For Maven integration, include the SpotBugs plugin in your pom.xml
:
<plugin>
<groupId>com.github.spotbugs</groupId>
<artifactId>spotbugs-maven-plugin</artifactId>
<version>4.2.0</version>
<configuration>
<outputDir>${project.build.directory}/spotbugs</outputDir>
<failOnError>true</failOnError>
</configuration>
</plugin>
Why Use SpotBugs?
SpotBugs identifies bugs that might have escaped attention during development. By diagnosing issues early, it allows for a more efficient development cycle.
5. JUnit and Mockito
JUnit and Mockito are essential for unit testing in Java. They ensure that your code behaves as expected and encourages better design.
Key Features:
- JUnit: Provides a simple way to create and run tests, with support for annotations.
- Mockito: Lets you create mock objects to facilitate isolated testing.
Example of Unit Testing with JUnit and Mockito
Here's how you can test a simple Java method:
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
@Test
public void testAddWithMock() {
MathService mathService = mock(MathService.class);
when(mathService.add(2, 3)).thenReturn(5);
assertEquals(5, mathService.add(2, 3));
}
}
Why Use JUnit and Mockito?
Automated testing prevents regressions and helps you refactor with confidence. JUnit's simplicity combined with Mockito's capabilities for creating mocks provides a robust testing framework.
In Conclusion, Here is What Matters
In Java development, quality tools are essential. They not only help in maintaining high code quality but also improve collaboration and reduce bugs. By leveraging tools like SonarQube, Checkstyle, PMD, SpotBugs, and JUnit with Mockito, you're setting yourself up for success.
Invest in your coding environment today, and watch your Java projects thrive. For additional enhancements, consider exploring continuous integration platforms such as Jenkins or Travis CI. The synergy of these tools can further elevate your code quality to new heights. Happy coding!