Overcoming Common Spring Boot Service Development Pitfalls
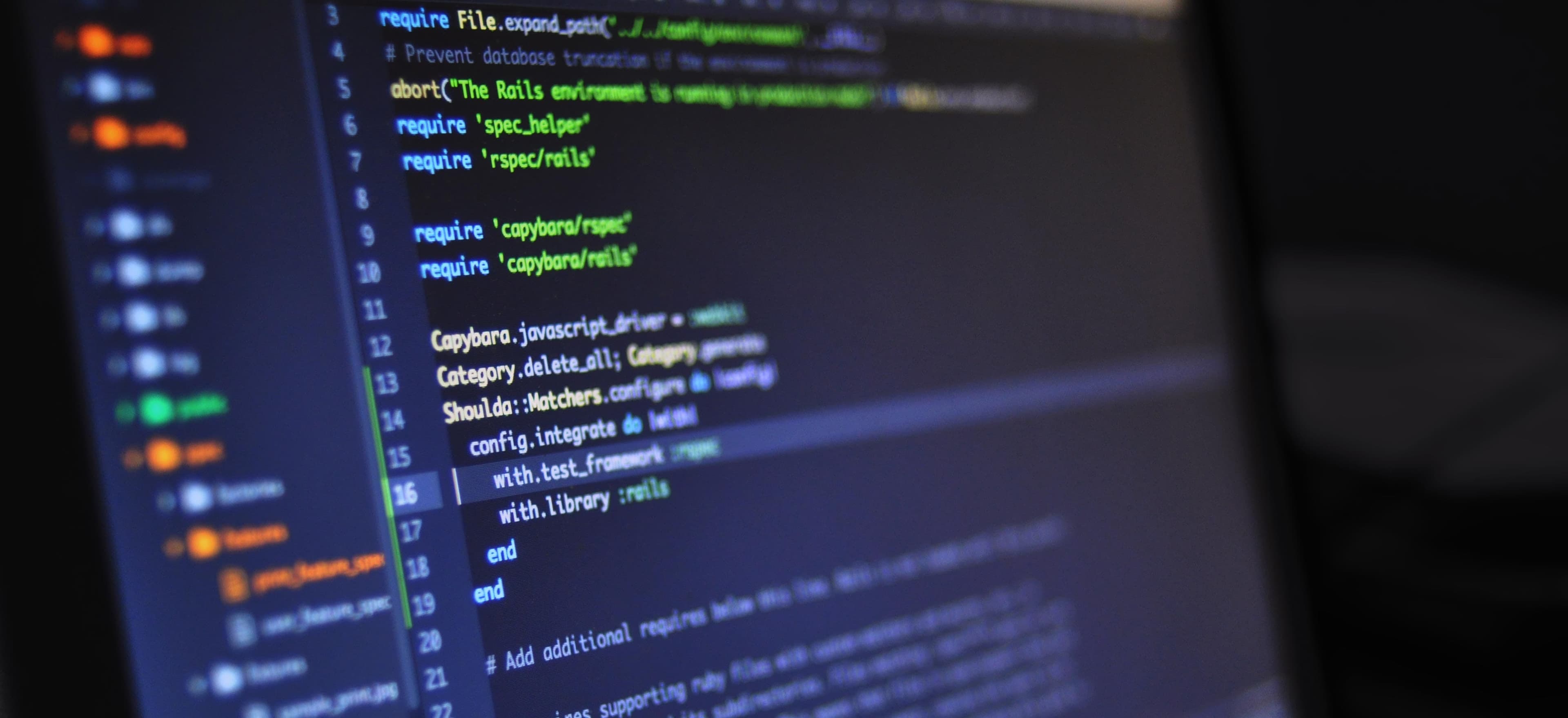
- Published on
Overcoming Common Spring Boot Service Development Pitfalls
Spring Boot has revolutionized the way developers approach Java-based application development. With its array of features, it simplifies the setup and configuration process, allowing developers to focus on writing application logic rather than boilerplate code. Nonetheless, there are common pitfalls that developers often encounter during the service development cycle. This blog post will address these challenges and provide practical solutions to overcome them.
Understanding Spring Boot
Spring Boot is an extension of the Spring framework, designed to ease the bootstrapping and development of new Spring applications. It is opinionated, meaning it comes with a set of defaults that minimize the need for configuration. This design aspect significantly lowers the barriers to entry for developers, making it an excellent choice for microservices architecture.
However, even seasoned developers can trip over some common issues. Let's explore these pitfalls in detail.
1. Improper Use of @Autowired
Problem
The @Autowired
annotation is a powerful feature that allows Spring to automatically inject dependencies. However, overusing it or using it incorrectly can lead to hidden dependencies and make your code less maintainable.
Solution
Limit the use of @Autowired
, especially for constructors and method parameters. Constructor injection is generally preferred over field injection because it promotes immutability and makes your beans easier to test.
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final UserRepository userRepository;
// Constructor injection
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void registerUser(User user) {
userRepository.save(user);
}
}
Why Use Constructor Injection?
Constructor injection allows for easier testing by enabling the use of mocking frameworks. It also makes your dependencies explicit, leading to better design practices.
2. Ignoring Exception Handling
Problem
When developing services, a common oversight is to neglect proper exception handling. This can lead to unhandled exceptions, causing your application to fail without proper feedback to the end user.
Solution
Implement a global exception handler using @ControllerAdvice
. This allows you to centralize exception handling.
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestController;
@ControllerAdvice
@RestController
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGenericException(Exception ex) {
return new ResponseEntity<>("An error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why Centralize Exception Handling?
Centralizing exception handling helps maintain a clean codebase. It allows you to modify the behavior for exceptions globally without having to repeat error-handling code in each controller.
3. Forgetting to Use Profiles
Problem
Configuration management can become cumbersome as services grow. Many developers forget to utilize Spring's profile feature to manage environment-specific configurations.
Solution
Utilize Spring Profiles to separate your application settings based on environments like development, testing, and production. You can create application properties files for each profile.
# application-dev.properties
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driver-class-name=org.h2.Driver
# application-prod.properties
spring.datasource.url=jdbc:mysql://prod-db:3306/mydb
spring.datasource.username=prodUser
spring.datasource.password=prodPass
How to Activate a Profile
You can activate a profile using environment variables or command-line arguments:
java -jar myapp.jar --spring.profiles.active=prod
Why Use Profiles?
Profiles enable you to keep your configurations organized and relevant to their respective environments, reducing the risk of accidental misconfigurations that could lead to downtime.
4. Hardcoding Values
Problem
Another persistent issue is the practice of hardcoding values within your application. This is not only bad practice but also creates maintenance nightmares.
Solution
Utilize configuration files and the @Value
annotation for configurable values.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class MailService {
@Value("${mail.host}")
private String mailHost;
@Value("${mail.port}")
private int mailPort;
public void sendMail(String recipient, String message) {
// Use mailHost and mailPort to send the email
}
}
Why Avoid Hardcoding?
Using configuration allows changes to be made without modifying the source code. This makes deployments more flexible and reduces dependencies on a specific runtime environment.
5. Not Leveraging Spring Boot's Actuator
Problem
Many developers overlook Spring Boot's built-in Actuator, which provides valuable tools for monitoring and managing your application. Not utilizing it means missing out on insights into application health, metrics, and environment details.
Solution
Add the Actuator dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Then, expose the necessary endpoints in your application.properties
:
management.endpoints.web.exposure.include=*
The Bottom Line
Spring Boot's Actuator can expose endpoints such as /actuator/health
, which allows you to check the health status of your application.
Why Use Actuator?
Actuator endpoints provide critical runtime information about your application. This can be a game-changer when diagnosing issues in production environments.
The Bottom Line
Embarking on a journey of developing services in Spring Boot can be incredibly rewarding, yet it is laden with pitfalls that can hinder productivity and application performance. By keeping best practices in mind—such as using constructor injection, implementing centralized exception handling, utilizing profiles, avoiding hardcoding, and leveraging Spring Boot's Actuator—you can bolster both your code quality and application reliability.
The wisdom behind overcoming these common pitfalls lies not just in understanding them but in actively developing habits that help prevent them. Spring Boot has so much to offer, and with these insights, you're equipped to harness its full potential.
For further reading on Spring Boot best practices, you might find these links helpful:
With these practices and guidelines, you should now be better prepared to tackle the complexities of Spring Boot service development. Happy coding!
Checkout our other articles