Overcoming Resource Leakage in Command Pattern Implementations
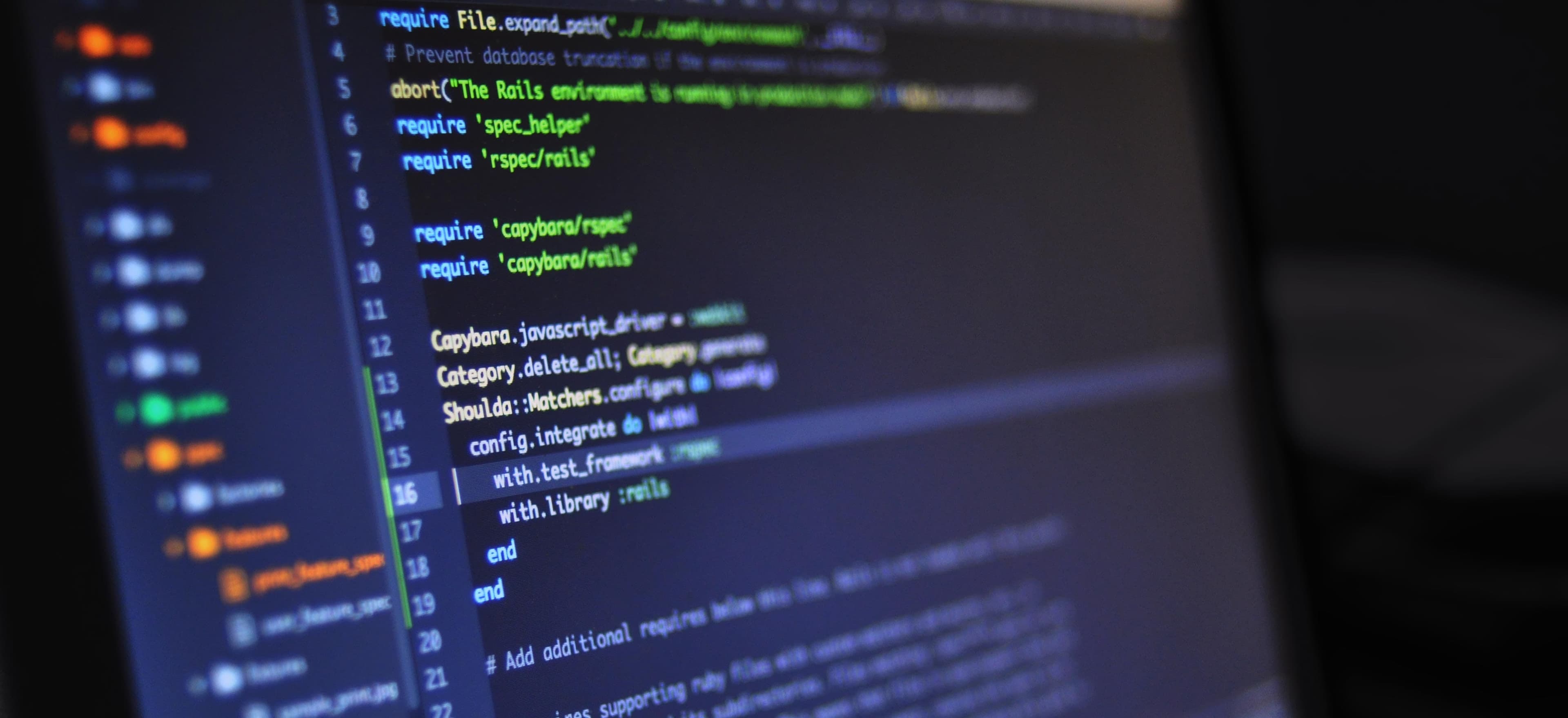
- Published on
Overcoming Resource Leakage in Command Pattern Implementations
Essentials at a Glance
In software design, the Command Pattern is a behavioral design pattern that encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. This pattern is useful for implementing undoable operations, queuing tasks, or even logging. However, like many patterns, improper implementation can lead to issues such as resource leakage.
In this blog post, we will discuss what resource leakage is, why it is a concern, how it can occur in Command Pattern implementations, and strategies to overcome it. We will emphasize clear code examples to illustrate our points and provide practical insights to mitigate these risks.
What is Resource Leakage?
Resource leakage occurs when a program holds onto system resources like memory, file handles, database connections, or network sockets longer than necessary, resulting in depletion of those resources. This often leads to performance issues or even application crashes.
For example, if a file handle is opened and not closed properly, the system may run out of available file handles, preventing other parts of the application (or other applications) from performing necessary file operations.
Understanding the Command Pattern
Before diving deeper into resource leakage, let's clarify the Command Pattern.
Implementation Overview
The Command Pattern involves three main components:
- Command Interface: Defines a method for executing a command.
- Concrete Command: Implements the
Command
interface and carries out the action. - Invoker: Holds a command and invokes it when needed.
- Receiver: Contains the business logic to execute the command.
Here’s a simple implementation:
// Command Interface
public interface Command {
void execute();
}
// Receiver
public class Light {
public void turnOn() {
System.out.println("The light is on");
}
public void turnOff() {
System.out.println("The light is off");
}
}
// Concrete Command
public class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOn();
}
}
public class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.turnOff();
}
}
// Invoker
public class RemoteControl {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
if (command != null) command.execute();
}
}
How Resource Leakage Manifests in Command Pattern Implementations
The Command Pattern, if not implemented correctly, can lead to several issues regarding resource management. Here are a few situations where resource leakage commonly occurs:
- Unclosed Resources: Commands holding onto resources may not properly release them after execution.
- Long-lived Commands: Keeping commands around longer than needed can result in unintended retention of resources.
- Circular References: Commands holding references to objects which in turn hold references back to the commands can create memory leaks.
Example of Resource Leakage
Let’s consider an example where we open a database connection in the command implementation but forget to close it after use.
public class DatabaseCommand implements Command {
private Connection connection;
public DatabaseCommand() {
try {
// Open a connection
this.connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void execute() {
// Perform database operations here...
}
public void closeConnection() {
// Close the connection - critical step that can be overlooked
try {
if (connection != null && !connection.isClosed()) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this case, the DatabaseCommand
doesn't guarantee that closeConnection
will be called, leading to resource leakage.
Strategies to Overcome Resource Leakage
1. Implementing Resource Management with Try-with-Resources
Java provides an elegant way to handle resources using the try-with-resources statement. This allows resources to be automatically closed at the end of the statement.
Here's how we can modify the DatabaseCommand
:
public class DatabaseCommand implements Command {
private String query;
public DatabaseCommand(String query) {
this.query = query;
}
@Override
public void execute() {
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement statement = connection.createStatement()) {
// Perform database operations here using the statement
statement.executeUpdate(query);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
By using try-with-resources, we ensure that the connection and statement are closed automatically, eliminating the risk of resource leakage.
2. Using Weak References
If your command objects are relatively long-lived, controlled use of weak references can help prevent memory leaks:
import java.lang.ref.WeakReference;
public class MyCommand implements Command {
private WeakReference<Receiver> receiver;
public MyCommand(Receiver receiver) {
this.receiver = new WeakReference<>(receiver);
}
public void execute() {
Receiver r = receiver.get();
if (r != null) {
r.performAction();
} else {
System.out.println("Receiver has been garbage collected");
}
}
}
Using WeakReference
allows the referenced Receiver
object to be garbage collected when there are no strong references to it.
3. Lifecycle Management
Manage your Command’s lifecycle explicitly. If a command is designed to hold resources, ensure you implement a cleanup mechanism (e.g., a dispose
or cleanup
method) that kinds of like a destructor to release resources.
public class HeavyCommand implements Command {
private Resource resource;
public HeavyCommand() {
this.resource = new Resource(); // Acquire Resource
}
@Override
public void execute() {
// Perform operation
}
public void cleanup() {
// Release resource
resource.release();
}
}
// Somewhere in your code:
HeavyCommand command = new HeavyCommand();
command.execute();
command.cleanup(); // Explicit cleanup
4. Monitor Resources
Using profilers or monitoring tools to check for memory leaks can help detect potential problems early in the development process. Regular profiling helps catch issues before they impact performance.
Key Takeaways
Overcoming resource leakage in Command Pattern implementations is crucial for robust and resilient software design. By understanding where these leaks can occur and using the aforementioned strategies—like try-with-resources, weak references, meticulous lifecycle management, and resource monitoring—you can significantly improve your applications' stability and performance.
The Command Pattern can be immensely powerful, enabling you to create flexible and reusable code. However, as with all powerful tools, it requires careful management to avoid pitfalls such as resource leakage. By following best practices, you can leverage this pattern effectively while ensuring your applications remain responsive and reliable.
For further reading on design patterns in Java, consider checking out the Design Patterns book by Gamma et al. and the Java Documentation for design patterns that are widely recognized in the industry.
Happy coding!