Common Pitfalls When Creating Executable JAR Files
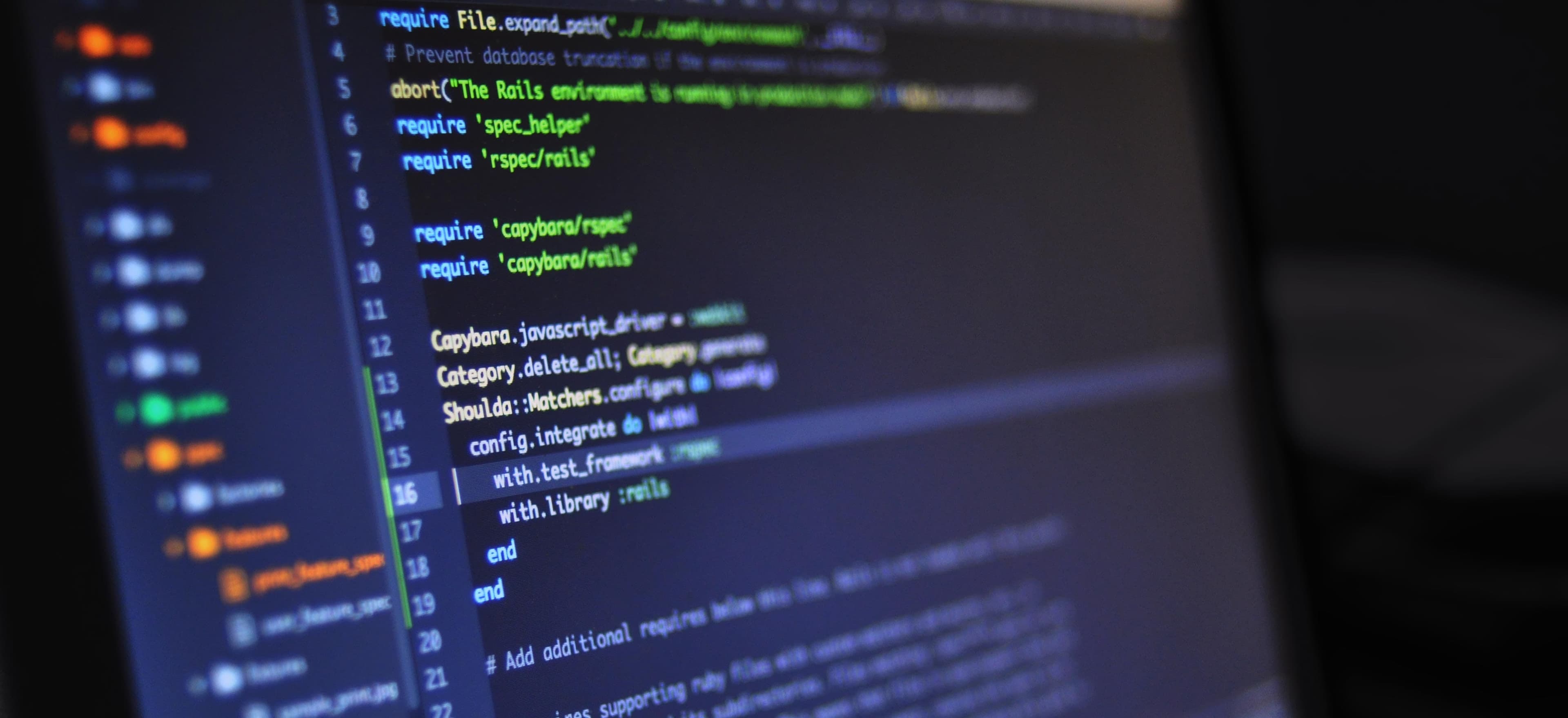
- Published on
Common Pitfalls When Creating Executable JAR Files in Java
Java has gained immense popularity over the years for its portability, which largely stems from its ability to run the same bytecode on any platform with a Java Virtual Machine (JVM). However, when it comes to packaging your application into an executable JAR file, new challenges may arise. In this blog post, we will delve into common pitfalls developers encounter when creating executable JAR files, provide solutions, and include code snippets to illustrate our points.
What is an Executable JAR File?
Before we dive into the pitfalls, let's clarify what an executable JAR file is. A JAR (Java Archive) file is essentially a .zip file that contains the compiled Java class files, and it may also include resources such as images, configuration files, and property files. When we refer to an "executable JAR," we’re talking about a specific JAR file that can be run directly from the command line or a double-click, thanks to a specified Main-Class
manifest entry.
Creating a Basic Executable JAR
To create an executable JAR file, you can use the command line. Below is a simple way to package your Java application into a JAR.
-
Compile your classes:
javac MyApp.java
-
Create a manifest file (manifest.txt):
Main-Class: MyApp
-
Package the JAR:
jar cfm MyApp.jar manifest.txt MyApp.class
Now you can run your JAR file via:
java -jar MyApp.jar
However, as you venture into packaging complex applications, the following common pitfalls may emerge.
Pitfall 1: Missing Class Files
The Issue
One of the most common pitfalls is not including all the necessary class files in the JAR. Forgetting to specify a class file in the JAR packaging process means that you'll receive a ClassNotFoundException
when you execute your program.
The Fix
Always ensure that all relevant class files and resources are included in your JAR. An easy way to do this is to use the command *.class
, which includes all compiled classes in the current directory.
jar cvf MyApp.jar *.class
Notes
Create a structured directory for your package. For instance, if your main class is com.example.MyApp
, ensure your class files are organized accordingly.
Pitfall 2: Incorrect Manifest File
The Issue
The manifest file is critical in defining entry points, dependencies, and versioning for JAR files. If the Main-Class
entry is improperly formatted or missing a new line at the end, your JAR will not execute properly.
The Fix
Ensure the manifest file looks like this:
Main-Class: com.example.MyApp
Note the blank line at the end, which separates the manifest entries from any possible additional entries.
Code Snippet
Here's how to create your JAR and include the manifest correctly:
echo "Main-Class: com.example.MyApp" > manifest.txt
jar cfm MyApp.jar manifest.txt -C bin/ .
This command ensures you're using -C
to change to the target directory, which includes the compiled classes (bin
in this example).
Pitfall 3: Dependencies Are Not Included
The Issue
If your application depends on external libraries (JAR files), forgetting to include these in your executable JAR means that a NoClassDefFoundError
will likely occur at runtime.
The Fix
You have a couple of options:
-
Fat JAR (Uber JAR): Package all your dependencies into one JAR file. Tools like Maven or Gradle can handle this for you.
-
Class-Path Manifest Entry: Alternatively, you can specify the JARs in a
Class-Path
entry inside the manifest file:Class-Path: lib/dependency1.jar lib/dependency2.jar
Example Using Maven
If you're using Maven, include the following plugin configuration in your pom.xml
to create a fat JAR:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-assembly-plugin</artifactId>
<version>3.3.0</version>
<configuration>
<archive>
<manifest>
<mainClass>com.example.MyApp</mainClass>
</manifest>
</archive>
<descriptorRefs>
<descriptorRef>jar-with-dependencies</descriptorRef>
</descriptorRefs>
</configuration>
<executions>
<execution>
<id>make-assembly</id>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
Pitfall 4: File Encoding Issues
The Issue
Running into character encoding problems is commonplace, especially when your application deals with file input/output. An executable JAR might read files as ISO-8859-1
instead of UTF-8
unless specified.
The Fix
You can enforce UTF-8 encoding by setting the encoding during compilation and execution:
javac -encoding UTF-8 MyApp.java
java -Dfile.encoding=UTF-8 -jar MyApp.jar
This ensures compatibility across different environments.
Pitfall 5: Permissions Issues
The Issue
Another pitfall lies in file permissions, especially on Unix-based systems. If your executable JAR needs to access specific files or directories, failing to assign the right permissions could cause it to crash.
The Fix
Make sure the application has the necessary permissions by using chmod
. For example:
chmod +x MyApp.jar
Also, use appropriate paths in your code to refer to resources. They may differ based on the environment you are in.
Final Thoughts
Creating executable JAR files can be a straightforward task if you’re mindful of these common pitfalls. Ensuring all class files are included, the manifest is correctly formatted, dependencies are managed, character encodings are set properly, and permissions are honored rises to the top of essential tasks for a smooth deployment.
For a more comprehensive look into JAR files, check out Oracle's official documentation. Keep exploring and best of luck on your Java journey!
Checkout our other articles