Troubleshooting JAX-RS and JSON-P Integration Errors
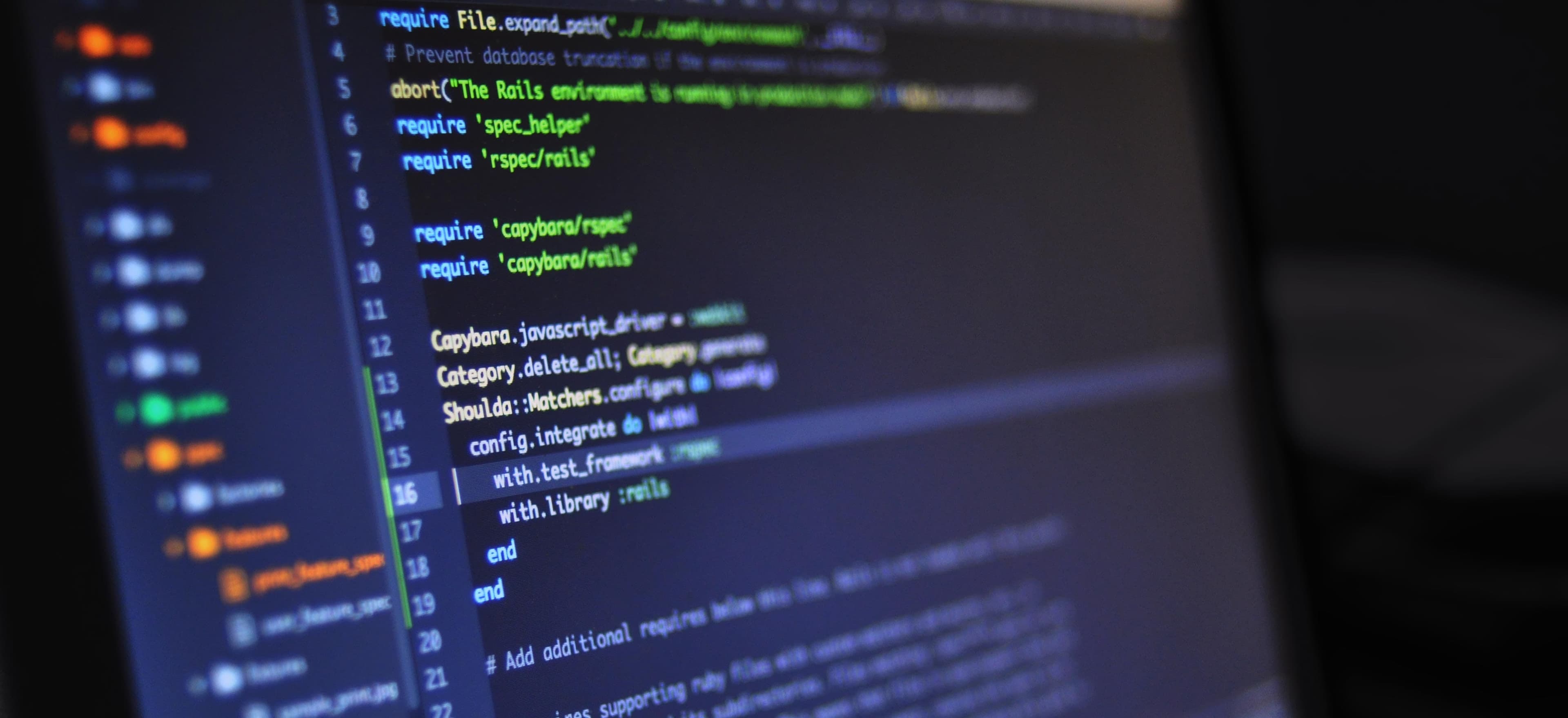
- Published on
Troubleshooting JAX-RS and JSON-P Integration Errors
In today's development landscape, RESTful services are an essential part of software architecture. Java API for RESTful Web Services (JAX-RS) provides a powerful and flexible framework for building these services, while JSON Processing (JSON-P) allows us to manipulate JavaScript Object Notation (JSON) data. However, integrating these two technologies can sometimes lead to complex issues that need troubleshooting. This blog post aims to help you identify and resolve common integration errors that occur between JAX-RS and JSON-P.
Understanding JAX-RS and JSON-P
Before diving into troubleshooting, it's critical to understand what JAX-RS and JSON-P are and why they are often used together.
-
JAX-RS: A set of APIs to create RESTful Web Services in Java. It simplifies the development of web services by providing annotations for defining resources.
-
JSON-P: A Java API for processing JSON. It allows for both reading and writing JSON data and is widely used for interacting with RESTful services.
The combination of JAX-RS and JSON-P offers developers an efficient way to build and consume RESTful APIs that require JSON serialization and deserialization.
Common Integration Errors
Building applications with JAX-RS and JSON-P can sometimes lead to errors. Below, we will discuss common issues and how to resolve them.
1. Missing JSON-P Dependencies
One of the most common mistakes is the lack of necessary dependencies in the project's build tool (such as Maven or Gradle).
Solution: Ensure that your pom.xml
or build.gradle
file includes the required dependencies. Here’s how you can add them:
Maven:
<dependency>
<groupId>javax.json</groupId>
<artifactId>javax.json-api</artifactId>
<version>1.1.4</version>
</dependency>
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.json</artifactId>
<version>1.1.4</version>
</dependency>
Gradle:
implementation 'javax.json:javax.json-api:1.1.4'
implementation 'org.glassfish:javax.json:1.1.4'
After ensuring these dependencies are in place, conduct a clean build of your project to verify everything is configured correctly.
2. Incorrect Media Type Configurations
When sending or receiving JSON data, it's crucial to specify the correct media type for the requests and responses. Omitting this can lead to errors.
Solution: Clearly define the media types using JAX-RS annotations in your resource classes. For instance:
@Path("/users")
public class UserResource {
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createUser(User user) {
// Logic for creating a user
return Response.status(Response.Status.CREATED).entity(user).build();
}
}
In this example, @Consumes
specifies that the endpoint will accept JSON data, while @Produces
indicates that it will return JSON. This helps avoid media type mismatches.
3. Incorrect JSON Structure
Another frequent issue arises from expecting a certain JSON structure that does not match the representation in your Java classes.
Solution: Use JSON-P to handle JSON data carefully. Here's an example demonstrating how you can use JSON-P to parse incoming JSON:
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response createUser(JsonObject jsonObject) {
String username = jsonObject.getString("username");
String email = jsonObject.getString("email");
// Validation and user creation logic here
User user = new User(username, email);
return Response.status(Response.Status.CREATED).entity(user).build();
}
This approach helps validate the incoming JSON structure. You can also throw exceptions or return error responses when the expected data is not present.
4. Deserialization Errors
A failure to convert JSON into an object can occur if the expected fields in the class do not match the incoming JSON.
Solution: Ensure that your object fields exactly align with the JSON structure. For example:
public class User {
private String username;
private String email;
// Constructors, getters, and setters
}
If the JSON keys are "user_name" and "email_address", you could handle it with custom deserialization logic or by adapting the JSON structure sent to the server. Using a mapping library such as Jackson or Gson can simplify this process.
5. Exceptions and Error Handling
Whenever an error occurs, it’s essential to return appropriate responses to inform clients of what went wrong.
Solution: Implement proper exception handling in JAX-RS using ExceptionMapper
. Here’s an example:
@Provider
public class CustomExceptionMapper implements ExceptionMapper<Exception> {
@Override
public Response toResponse(Exception exception) {
String errorMessage = exception.getMessage();
JsonObject jsonMessage = Json.createObjectBuilder()
.add("error", errorMessage)
.build();
return Response.status(Response.Status.INTERNAL_SERVER_ERROR)
.entity(jsonMessage)
.type(MediaType.APPLICATION_JSON)
.build();
}
}
This approach helps ensure that clients receive structured error messages in a JSON format.
6. Cross-Origin Resource Sharing (CORS) Issues
When making requests from different origins, CORS issues can arise, blocking access to your REST API.
Solution: Configure CORS in your application by adding the appropriate filters. Here’s how you can implement a simple CORS filter:
@Provider
public class CORSFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext,
ContainerResponseContext responseContext) {
responseContext.getHeaders().add("Access-Control-Allow-Origin", "*");
responseContext.getHeaders().add("Access-Control-Allow-Headers",
"origin, content-type, accept, authorization");
responseContext.getHeaders().add("Access-Control-Allow-Credentials", "true");
responseContext.getHeaders().add("Access-Control-Allow-Methods",
"GET, POST, PUT, DELETE, OPTIONS, HEAD");
}
}
This filter permits all domains to access your REST API, but you can restrict it to specific domains as per your needs.
Closing the Chapter
Integrating JAX-RS with JSON-P to build robust RESTful web services is a powerful combination. However, like any software solution, it can come with its challenges. By understanding the common errors outlined in this post and following the suggested solutions, you can effectively troubleshoot and enhance your application.
For further reading about JAX-RS and JSON-P concepts, consider exploring the official documentation:
- JAX-RS Documentation
- JSON-P Specification
If you encounter other issues not mentioned here, feel free to share your experience in the comments! Happy coding!