Conquering Dynamic IDs: Selenium Testing Made Simple
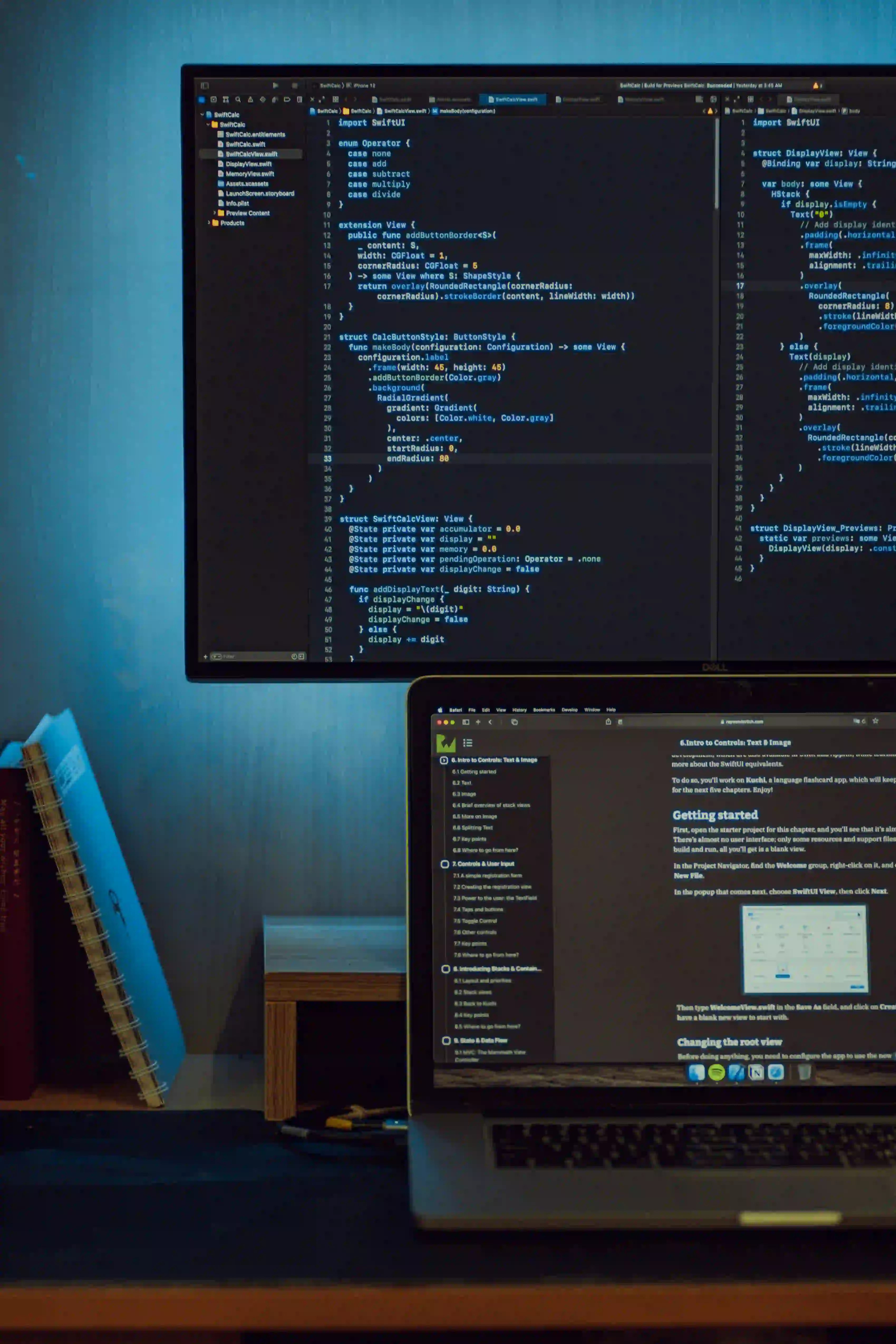
Conquering Dynamic IDs: Selenium Testing Made Simple
In the world of web automation and testing, Selenium has emerged as a powerful tool that allows developers and testers to ensure that web applications function flawlessly. One of the challenges testers often face while using Selenium is dealing with dynamic IDs. In this post, we will explore what dynamic IDs are, why they can be problematic, and how to effectively use Selenium to handle them.
What Are Dynamic IDs?
Dynamic IDs are unique identifiers that change with each page load or user session. While they serve a purpose in web development—like enhancing security and preventing caching—they can complicate automated testing. For instance, if a Selenium test script references an element by its ID that changes every time the page loads, the script will fail, leading to unreliable tests.
Example of Dynamic IDs
Consider the following HTML snippet. Notice the ID changes with each page load:
<div id="userProfile_12345">John Doe</div>
<div id="userProfile_67890">Jane Smith</div>
Here, userProfile_12345
and userProfile_67890
are dynamic IDs that will change every time the page is refreshed.
Why Are Dynamic IDs Problematic?
- Test Reliability: Scripts that rely on these dynamic elements can unpredictably fail, leading to false negatives in testing.
- Maintenance Costs: Tests may require frequent updates, increasing overhead in test automation.
- Increased Time: Writing workarounds to locate these elements effectively can consume valuable time.
Strategies to Handle Dynamic IDs
1. Utilizing XPath
XPath is a powerful querying language that can be used to navigate through elements and attributes in an XML document. It allows you to search for elements based on their relationship and attributes, rather than relying solely on their ID.
Example: Using XPath
Let's say you want to locate the userProfile
div. You can select it using its text:
WebElement userProfile = driver.findElement(By.xpath("//div[contains(text(), 'John Doe')]"));
Why XPath?
Using XPath prioritizes the content of the element over its ID. This makes your tests more robust since the location won’t change if the ID does.
2. CSS Selectors
CSS selectors offer another way to identify elements without relying on IDs. They can target classes, types, or even positional elements.
Example: Using CSS Selectors
WebElement userProfile = driver.findElement(By.cssSelector("div[id^='userProfile_']"));
Why CSS Selectors?
This example selects any div
element whose ID starts with userProfile_
. This method is efficient because it allows you to match multiple elements without worrying about their exact dynamic ID.
3. Using Parent or Sibling Elements
If elements share a common pattern in their structure, you can use their parent or sibling elements to locate them.
Example: Navigating the DOM
WebElement userProfile = driver.findElement(By.xpath("//div[@class='user-container']/div[1]"));
Why Parent/Sibling Navigation?
This method keeps your tests focused on the structure rather than the ID. Even if the ID changes, as long as the hierarchy remains intact, your tests will still pass.
4. Data-Attributes
If you have control over the HTML, adding data-attributes
can be incredibly helpful. For instance:
<div data-user="12345">John Doe</div>
This way, you can select elements via their data attributes:
WebElement userProfile = driver.findElement(By.cssSelector("div[data-user='12345']"));
Why Data-Attributes?
Data-attributes are static and can be safe references within tests. This method promotes stability and maintainability.
5. Using the Selenium Page Object Model (POM)
When dealing with a large number of dynamic elements, the Page Object Model can help keep your code organized. It allows you to abstract element locators into a separate object.
Example: Page Object Model Structure
public class UserProfilePage {
private WebDriver driver;
@FindBy(xpath = "//div[contains(text(), 'John Doe')]")
private WebElement userProfile;
public UserProfilePage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public String getUserName() {
return userProfile.getText();
}
}
Why POM?
The Page Object Model promotes reusability and makes the code easier to maintain. Once you locate elements effectively, any script that uses this object will benefit.
Best Practices for Handling Dynamic IDs
- Favor Attributes: Use stable attributes (like class names, text, or data attributes) whenever possible.
- Keep Tests Maintainable: Write tests in a way that makes them easy to update when the application changes.
- Use Implicit/Explicit Waits: Ensure your tests wait for elements to load, reducing flaky tests due to timing issues.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement userProfile = wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//div[contains(text(), 'John Doe')]")));
Closing the Chapter
Dynamic IDs present a real challenge in automated testing with Selenium, but they do not have to derail your efforts. By leveraging strategies such as XPath, CSS selectors, and the Page Object Model, you can construct robust, reliable test scripts.
For more insights on Selenium testing, you can check out the official Selenium documentation here. Remember, when tests are well-crafted, they become an asset rather than a liability.
Additional Resources
With these tools and practices in your toolkit, you're well-equipped to conquer the challenges of dynamic IDs in Selenium testing!