Common Issues in Spring Boot 2 SSO with OAuth 2 and OpenID
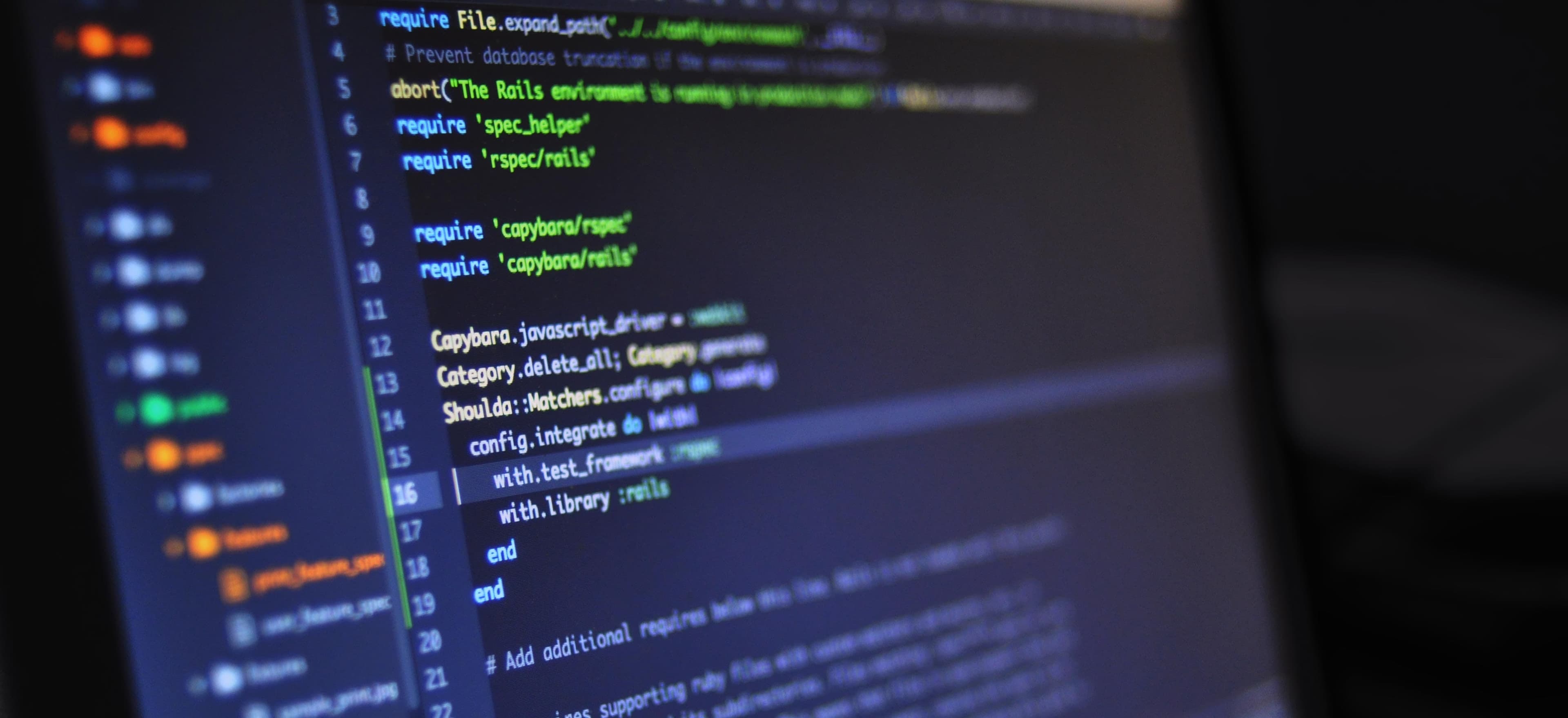
- Published on
Common Issues in Spring Boot 2 SSO with OAuth 2 and OpenID: A Comprehensive Overview
Single Sign-On (SSO) is one of the most sought-after functionalities in modern applications. The Spring Boot framework, alongside OAuth 2.0 and OpenID Connect, simplifies the SSO implementation. However, integrating these technologies can lead to common challenges. In this blog post, we will explore those typical issues, how to identify and troubleshoot them, and effective solutions.
Understanding the Basics
Before diving into the issues, let's clarify how OAuth 2.0 and OpenID Connect work. OAuth 2.0 is a protocol that allows applications to obtain limited access to user accounts while OpenID Connect is built on top of OAuth 2.0, providing authentication as a service.
The Benefits of Using Spring Boot with SSO
- Easy dependency management
- Automatic configuration
- Microservices support
- Flexibility in security customizations
Common Issues in Spring Boot 2 SSO with OAuth 2 and OpenID
Identifying common problems is the first step in an effective SSO implementation. Below are the most encountered issues along with code snippets and solutions.
1. Configuration Failures
Issue Description
Configuration is key in any SSO setup. A minor misconfiguration can lead to a plethora of issues—permissions denied, callbacks misdirected, or even complete authentication failures.
Solution
Begin by reviewing the application properties or YAML file. Ensure you have the correct entries for your OAuth 2.0 provider. The following code snippet illustrates the essential settings:
spring:
security:
oauth2:
client:
registration:
google:
client-id: YOUR_CLIENT_ID
client-secret: YOUR_CLIENT_SECRET
scope: profile, email
redirect-uri: "{baseUrl}/oauth2/callback/google"
provider:
google:
authorization-uri: https://accounts.google.com/o/oauth2/auth
token-uri: https://oauth2.googleapis.com/token
user-info-uri: https://www.googleapis.com/userinfo/me
Why This Matters? Correct configuration ensures that your application can interact with the OAuth provider seamlessly. Misconfigured URIs can lead to redirection issues or failed authorization requests.
2. Redirect URI Mismatches
Issue Description
A common issue arises when the redirect URI used in the application does not match the URI registered with the OAuth provider. This results in the application being unable to receive the access token.
Solution
Ensure that the redirect URI specified in your application properties matches exactly what is set in the provider’s configuration. For example:
@GetMapping("/login/oauth2/code/google")
public String getGoogleCallback(@RequestParam String code) {
// Code to handle the callback
}
Tip: Always use HTTPS in production environments for secure redirection.
3. Scopes Issues
Issue Description
Another frequent problem stems from improperly defined scopes. If you request access to resources that the OAuth provider does not permit, authentication will fail.
Solution
Map the required scopes in your application configuration. For instance, if you want to access user profile and email information, ensure the relevant scopes are specified:
scope: profile, email
Why It’s Important? Each OAuth provider has different permissions. Always refer to the provider’s documentation to request the correct scopes.
4. User Authentication Failures
Issue Description
Users may experience authentication failures due to expired tokens or incorrect user information being presented during the SSO process.
Solution
Implement a token refresh mechanism in your application. This can look something like this:
public void refreshToken() {
OAuth2AuthorizedClient authorizedClient =
this.authorizedClientService.loadAuthorizedClient(CLIENT_REGISTRATION_ID, principal.getName());
OAuth2AccessToken accessToken = authorizedClient.getAccessToken();
if (accessToken.isExpired()) {
// Refresh the token
OAuth2AccessToken newAccessToken = refreshAuthToken(authorizedClient);
}
}
Why This Matters? Refreshing the token will keep the user session active without requiring them to sign in again, enhancing user experience.
5. Lack of Proper Exception Handling
Issue Description
Failing to implement proper exception handling leads to unhelpful error messages for end users and developers alike.
Solution
Create custom error handlers and messages. The following code provides an example of handling authentication failures effectively:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(AuthenticationException.class)
public ResponseEntity<String> handleAuthError(Exception e) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("Authentication failed: " + e.getMessage());
}
}
Key Point: Integrated exception handling improves application reliability and enhances the development process.
6. Debugging Common Security Annotations
Issue Description
Spring Security annotations, such as @PreAuthorize and @Secured, may not behave as expected if misconfigured. Ensuring that these annotations correctly reflect method security requirements is crucial.
Solution
Start with a review of the security rules and ensure that roles and permissions are properly assigned. Here's an example:
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin")
public String adminPanel() {
return "Welcome to the Admin panel!";
}
Why is This Important? Properly utilized security annotations help maintain secure access control across your application.
Summary
Implementing SSO with Spring Boot, OAuth 2.0, and OpenID Connect can be a complex endeavor. However, by understanding common issues and applying the right fixes, developers can create robust and seamless authentication experiences.
Key Takeaways:
- Pay close attention to configuration settings.
- Ensure URIs and scopes are accurately defined.
- Incorporate token management strategies.
- Develop strong exception handling mechanisms.
- Review method security annotations carefully.
For additional reading, check out the official Spring Security Reference Documentation and OAuth 2.0 Specification for a deeper insight into these technologies.
Implementing these best practices will not only enhance the security and performance of your application but also streamline the user authentication experience significantly.