Mastering JUnit's ExpectedException Rule for Advanced Testing
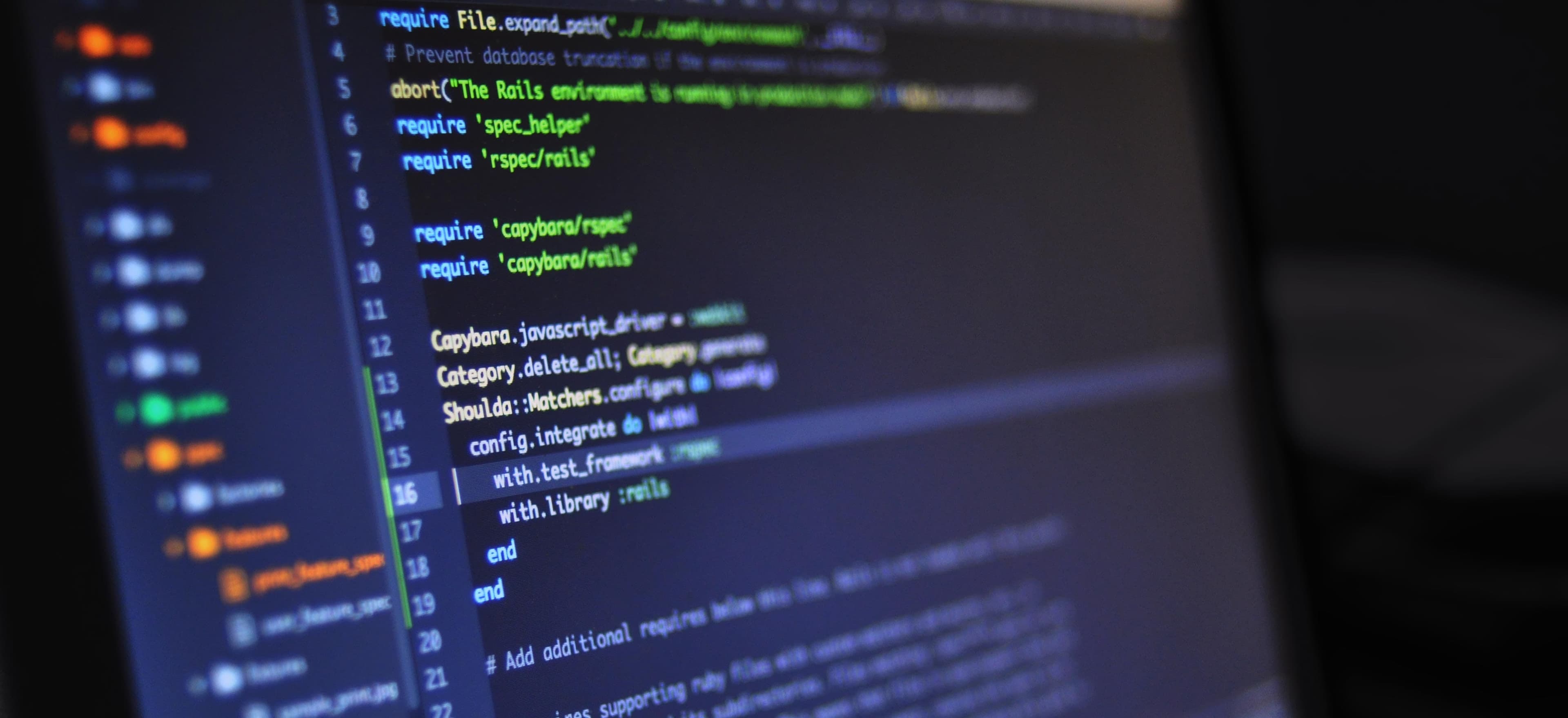
- Published on
Mastering JUnit's ExpectedException Rule for Advanced Testing
The Starting Line
In the world of software development, writing tests is as crucial as writing the actual code. As you venture into testing frameworks in Java, JUnit stands out because of its robustness and flexibility. One of the features of JUnit that is often overlooked but immensely powerful is the ExpectedException
rule. In this blog post, we will dive deep into understanding the ExpectedException
rule, its benefits, and how to implement it in your test cases effectively.
Why Use JUnit?
JUnit is a popular unit testing framework for Java. It provides annotations to identify test methods, assertions to verify the expected results, and test runners that determine how tests are executed. Using JUnit helps in:
- Automated Testing: Run your tests automatically, ensuring your code behaves as expected.
- Regression Testing: Confirm that new changes do not introduce bugs into existing code.
- Design Validation: Validate the design through tests, improving code quality.
Understanding the ExpectedException Rule
The ExpectedException
rule was introduced to provide a cleaner way to assert that exceptions are thrown during testing. It allows you to specify an expected exception type, along with the message you expect that exception to contain.
Setting Up JUnit with the ExpectedException Rule
To use the ExpectedException
rule, you first need to add JUnit to your project. In a Maven project, you would include the following dependency in your pom.xml
:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
A Basic Example
Let’s write a simple Java class that throws an exception:
public class Division {
public static double divide(int numerator, int denominator) {
if (denominator == 0) {
throw new IllegalArgumentException("Denominator cannot be zero");
}
return (double) numerator / denominator;
}
}
In this example, attempting to divide by zero will result in an IllegalArgumentException
. Now, let’s write a test to verify that this exception is thrown correctly using the ExpectedException
rule.
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
public class DivisionTest {
@Rule
public ExpectedException thrown = ExpectedException.none();
@Test
public void testDivideByZero() {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("Denominator cannot be zero");
Division.divide(10, 0);
}
}
Code Explanation
- The
@Rule
annotation registers theExpectedException
rule with the test runner. thrown.expect(IllegalArgumentException.class)
specifies that we expect anIllegalArgumentException
to be thrown.thrown.expectMessage("Denominator cannot be zero")
checks if the exception message matches our expectation.- The test method
testDivideByZero()
calls thedivide
method with a denominator of zero, triggering the exception.
Advantages of ExpectedException Rule
-
Readability: The
ExpectedException
rule makes your test cases easier to read and understand. Instead of wrapping your code in try-catch blocks, you can clearly express what exceptions you expect. -
Granularity: You can specify exact messages you expect from exceptions, helping in pinpointing issues more effectively during testing.
-
Separation of Concerns: By handling expected exceptions separately, you maintain focus on what is being tested without noisy assertions cluttering your logic.
Handling Multiple Exceptions
You might encounter situations where a method can throw multiple types of exceptions. Here’s how you can handle such scenarios with the ExpectedException
rule:
@Test
public void testMultipleExceptions() {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("Denominator cannot be zero");
Division.divide(10, 0);
thrown.expect(ArithmeticException.class);
thrown.expectMessage("Numerator cannot be negative");
Division.divide(-10, 2);
}
In this example, we’ve structured our test to check for multiple exceptions. This method triggers expected exceptions defined in a linear fashion.
Best Practices for Using ExpectedException Rule
-
Keep Tests Clear: Use descriptive names for your test methods, and keep the structure tidy. This aids in understanding the purpose of the test at a glance.
-
Limit Your Expectations: Test one exception per method when possible. While you can catch multiple exceptions, focusing on a single responsibility per test method leads to better testing practices.
-
Documentation: Comment on the purpose of the exception expectations, especially if they're complex. Clear documentation is beneficial for code reviews and future maintenance.
-
Preferred Over Try-Catch: Avoid using try-catch blocks in your test methods unless absolutely necessary. The
ExpectedException
rule provides a cleaner alternative.
Transitioning to JUnit 5
As of JUnit 5, the ExpectedException
rule has been replaced with a more streamlined approach using Assertions.assertThrows()
. Although our focus has been on JUnit 4, it is good to know you can upgrade to:
import static org.junit.jupiter.api.Assertions.assertThrows;
@Test
public void testDivideByZero() {
IllegalArgumentException thrown = assertThrows(
IllegalArgumentException.class,
() -> Division.divide(10, 0),
"Expected divide() to throw, but it didn't"
);
assertEquals("Denominator cannot be zero", thrown.getMessage());
}
This way, you can utilize lambda expressions for better readability and performance. Transitioning to newer frameworks keeps your codebase modern and leverages improvements made by the community.
Final Thoughts
JUnit's ExpectedException
rule is an essential tool for writing unit tests in Java. It enhances readability, maintains clarity, and promotes effective testing practices. While it may require a mindset shift for those used to traditional methods, the benefits become apparent quickly.
Whether you're still using JUnit 4 or considering a transition to JUnit 5, understanding how to effectively handle exceptions in your tests is crucial to maintaining high-quality code. For more on JUnit best practices, refer to the official JUnit documentation.
By mastering unit testing and exceptions, you not only create a solid foundation for your code but also build confidence in its reliability. Happy coding!
Checkout our other articles