Overcoming Challenges in Geospatial Distance Faceting with Lucene
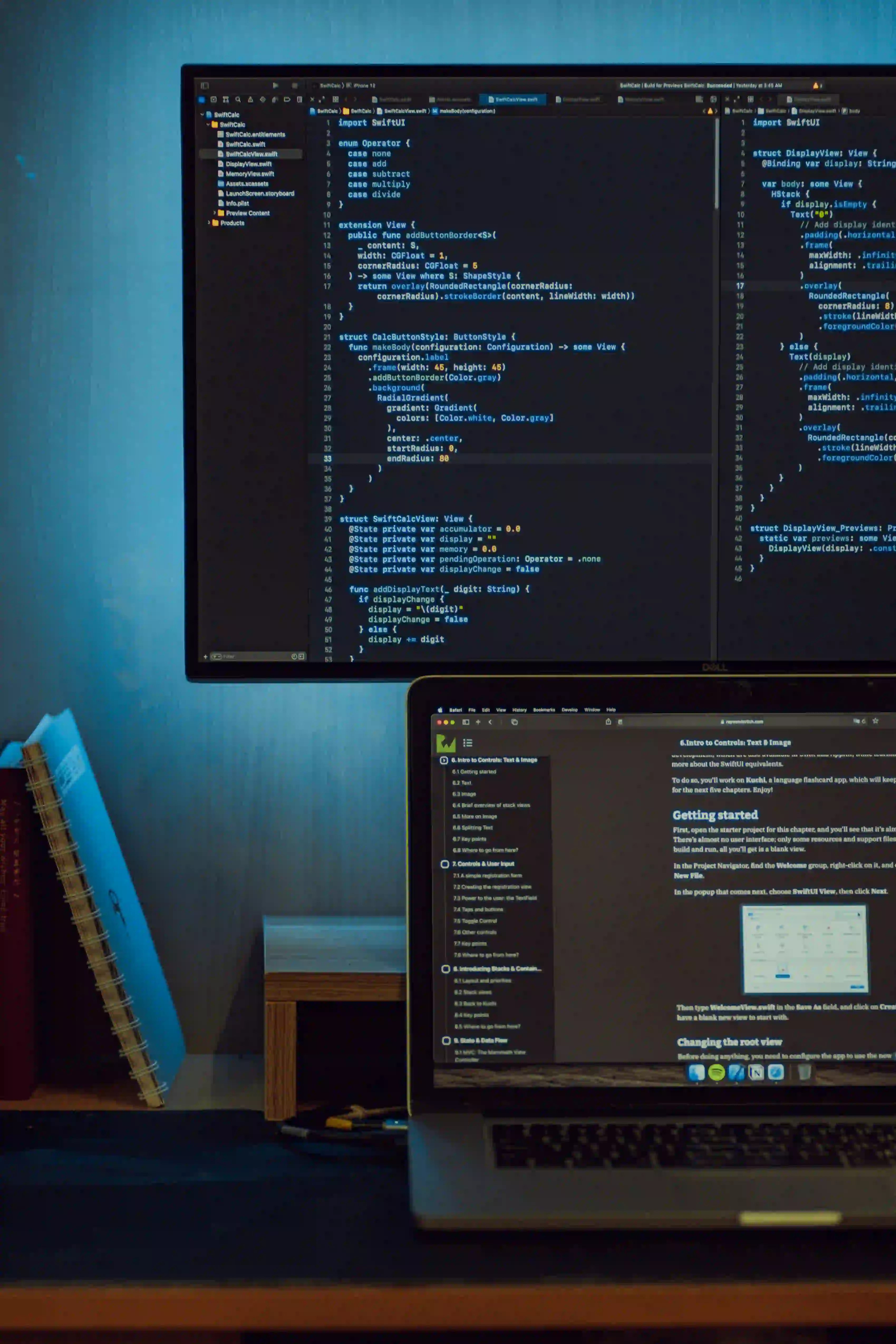
Overcoming Challenges in Geospatial Distance Faceting with Lucene
Geospatial data is increasingly vital in today's world. From mapping software to location-based services, the ability to process and analyze spatial data can provide significant advantages. Apache Lucene, a powerful search library, offers dynamic faceting capabilities, including geospatial distance faceting. However, even while leveraging Lucene's advantages, developers will encounter challenges. In this post, we will explore these challenges and practical ways to overcome them effectively.
Understanding Geospatial Distance Faceting
Before diving into the challenges, let's clarify what geospatial distance faceting involves. At its core, geospatial distance faceting allows you to categorize search results based on their spatial proximity to a specific point. This feature is crucial for applications requiring filtering of results based on user location or proximity to landmarks.
When querying large datasets, efficient spatial search is a necessity. Lucene provides a robust framework for indexing and searching. However, implementing distance faceting involves considerations like indexing strategies, query performance, and data accuracy.
Challenge 1: Efficient Indexing of Geospatial Data
The Importance of Efficient Indexing
Efficient indexing is critical for maximizing the performance of any search engine, and geospatial data can be particularly complex due to its multidimensional nature.
Indexing Strategies
To properly index geospatial data, you can utilize point data types and spatial filtering provided by Lucene:
import org.apache.lucene.document.Document;
import org.apache.lucene.document.DoublePoint;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.store.Directory;
// A method to create a Lucene Index Writer
public void indexGeospatialData(IndexWriter writer, double latitude, double longitude) throws Exception {
Document document = new Document();
// Indexing latitude and longitude as DoublePoints
document.add(new DoublePoint("location", latitude, longitude));
writer.addDocument(document);
}
Commentary
- The use of
DoublePoint
is advantageous since it allows for spatial queries on numeric values. By indexing latitude and longitude as points, searches will become more efficient. - Make sure to batch your indexing operations. Lucene's performance can degrade significantly if documents are indexed one at a time.
Challenge 2: Complex Query Handling
Understanding Spatial Queries
Sending complex queries to the Lucene index is often necessary. The challenge here lies in appropriately constructing spatial queries that can handle geospatial distance faceting.
Query Construction
You can use Query
classes to facilitate spatial queries:
import org.apache.lucene.spatial3d.geo.GeoML;
import org.apache.lucene.spatial3d.geom.*;
public void performGeospatialQuery(IndexSearcher searcher, double latitude, double longitude, double distance) throws Exception {
// Creating a new Geographic Point
GeoPoint point = new GeoPoint(latitude, longitude);
// Creating a Distance Query
Circle circle = new Circle(point, distance);
Query geoQuery = GeoQuery.newDistanceQuery("location", circle);
TopDocs results = searcher.search(geoQuery, 10); // Limit results to top 10.
// Process results...
}
Commentary
- This example shows how to establish a
GeoPoint
and query for a specific radius. - Carefully consider how many results to limit your query to; too many can negate performance advantages.
Challenge 3: Performance Optimization
Understanding Performance Bottlenecks
Performance can become an issue when dealing with large datasets. Inefficient queries, high document counts, and insufficient resources can contribute to slow response times.
Techniques for Optimization
To mitigate performance issues, you can utilize several techniques:
-
Caching: Leverage Lucene's caching capabilities for frequent queries to improve performance.
-
Parallelization: Use multithreading when processing multiple queries or indexing.
-
Index Sharding: Split large indexes into smaller, more manageable shards.
import org.apache.lucene.index.MultiReader;
import org.apache.lucene.index.IndexReader;
import org.apache.lucene.index.DirectoryReader;
public IndexReader createMultiReader(Directory[] directories) throws IOException {
return new MultiReader(directories);
}
// Example usage
IndexReader reader = createMultiReader(new Directory[]{indexDir1, indexDir2});
Commentary
- Parallelization can significantly reduce query response time. By breaking down tasks among multiple threads, you maximize CPU utilization.
- Using
MultiReader
allows you to merge different indices, which can help when sharding your data.
Challenge 4: Precision and Accuracy of Geospatial Data
Importance of Precision
Geospatial data requires a high degree of precision, as small inaccuracies can lead to large deviations in distance.
Techniques for Ensuring Accuracy
When dealing with accuracy, consider the following:
- Normalization: Normalize your geographic coordinates to ensure consistency.
- Validation: Regularly check the integrity of your data to identify and correct any inaccuracies.
public double normalizeLatitude(double latitude) {
if (latitude < -90 || latitude > 90) {
throw new IllegalArgumentException("Latitude must be between -90 and 90 degrees.");
}
return latitude;
}
Commentary
- This method ensures that any latitude value being indexed must fall within the acceptable range. Data validation is crucial to maintain high-quality indices.
Closing the Chapter
In conclusion, implementing geospatial distance faceting with Lucene can indeed pose challenges involving efficient indexing, complex query handling, performance optimization, and data accuracy. However, by understanding these challenges and employing the right techniques, developers can harness Lucene’s capabilities and create powerful geospatial applications.
Whether you are developing a location-based app or running an analysis of spatial data, Lucene's geospatial features can significantly enhance your application’s functionality. For more comprehensive insights, consider examining the official Apache Lucene documentation for deep dives into specific APIs and functionalities.
As you tackle these challenges, remember to iterate on your solutions. The field of geospatial analysis is vast, and staying current with best practices and new advancements will empower you to create even more effective spatial applications.
Happy coding!