Mastering Spring's DeferredResult: A Guide to Callables
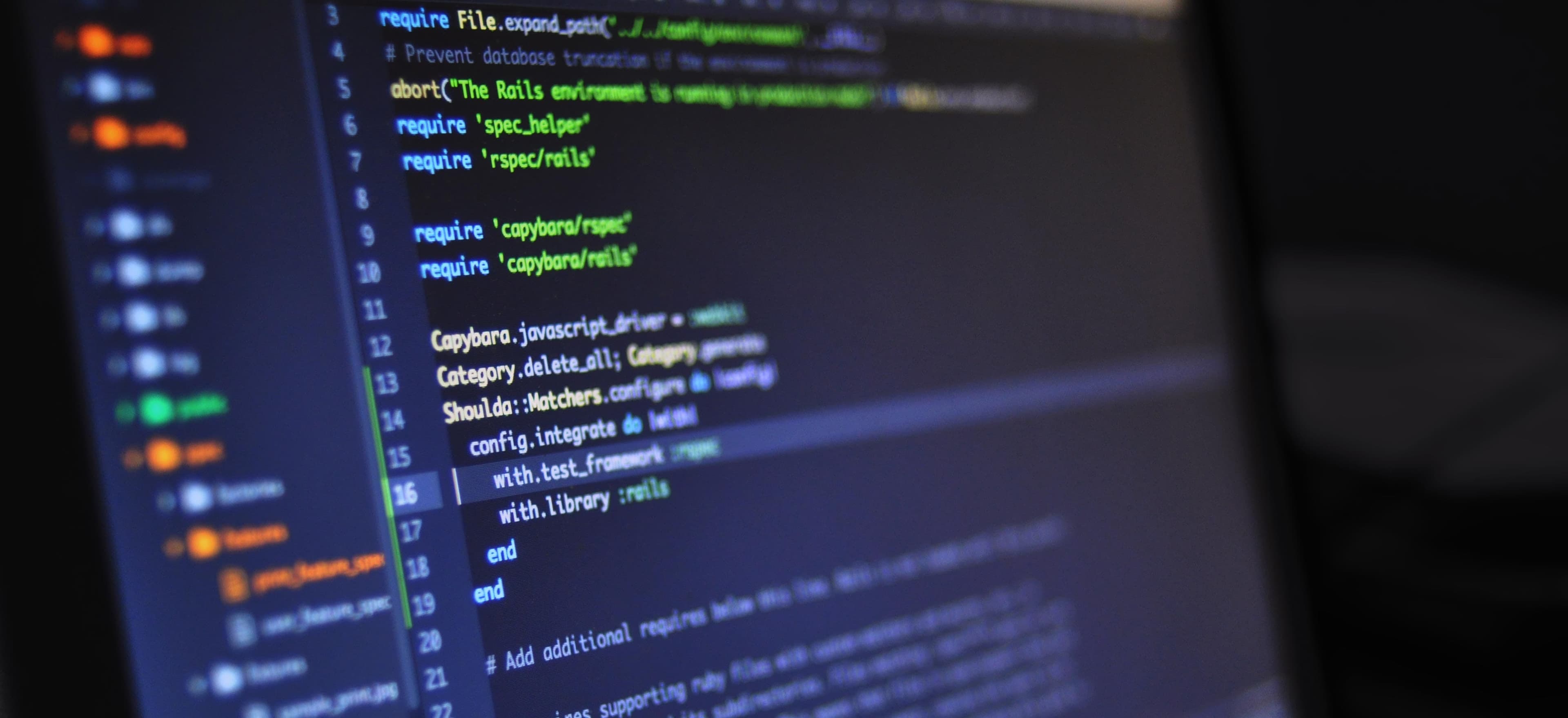
- Published on
Mastering Spring's DeferredResult: A Guide to Callables
In today's fast-paced web application environments, responsiveness is key. Users demand instant feedback, and delivering this while maintaining server efficiency can pose a significant challenge. Luckily, the Spring Framework provides powerful tools to tackle this problem, particularly through features such as DeferredResult
and Callable
. In this blog post, we will dive deep into DeferredResult
, understand its significance, and how it can be utilized for building responsive applications effectively.
Getting Started to Spring's Asynchronous Processing
Asynchronous processing in Spring helps improve the performance of web applications. The traditional synchronous model blocks a thread while waiting for an operation to complete, which can be inefficient. This is where Callable
and DeferredResult
come into play.
-
Callable: A
Callable
is a specialization ofRunnable
that can return a value or throw an exception, making it suitable for executing tasks asynchronously. -
DeferredResult:
DeferredResult
takes asynchronous processing a step further by allowing you to return a response to the client even after an operation has completed.
Understanding these two concepts will empower you to build more robust web services that can efficiently handle multiple requests without sacrificing server performance.
Getting Started with DeferredResult
To use DeferredResult
, you need to create a Spring MVC controller method that returns a DeferredResult
object. Below is a simplified example to illustrate the basic setup.
Simple Example of DeferredResult
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.context.request.async.DeferredResult;
import java.util.concurrent.Callable;
@RestController
public class DeferredResultController {
private final TaskService taskService;
public DeferredResultController(TaskService taskService) {
this.taskService = taskService;
}
@GetMapping("/deferred")
public DeferredResult<String> getDeferredResponse() {
DeferredResult<String> deferredResult = new DeferredResult<>(5000L, "Request timeout");
// This is where we start processing the task asynchronously
new Thread(() -> {
try {
// Simulate a long-running task
String result = taskService.performTask();
deferredResult.setResult(result);
} catch (Exception e) {
deferredResult.setErrorResult("Error processing request");
}
}).start();
return deferredResult;
}
}
Explanation of the Code
-
Creating the Controller: We define a REST controller named
DeferredResultController
. -
Injecting Task Service: A service class,
TaskService
, is injected to handle the business logic. -
Setting Up DeferredResult: A
DeferredResult
object is created with a timeout value of 5000 milliseconds. If the operation does not complete within this timeframe, a timeout message will be returned. -
Running Asynchronously: A new thread is started to perform a lengthy task asynchronously. Upon completion of the task,
setResult
is called to send data back to the client. -
Error Handling: If there’s an exception, we set an error result to handle failures gracefully.
Why Use DeferredResult?
- Non-Blocking: It allows the server to handle other requests while waiting for the task to complete.
- Customizable Timeout: The ability to define a timeout improves user experience by preventing long wait times.
- Better Resource Utilization: Fewer blocked threads lead to more responsive applications.
Advanced Usage of DeferredResult
The basic implementation of DeferredResult
demonstrates its crucial role in asynchronous processing. But how do you handle complex scenarios? Let's explore some advanced concepts.
Using Callable with DeferredResult
Sometimes, you need a more structured approach. Callable
provides a simple way to handle requests without dealing with threads directly.
@GetMapping("/callable")
public Callable<String> getCallableResponse() {
return () -> {
Thread.sleep(2000); // Simulating a long task execution
return "Callable response completed!";
};
}
Explanation of Callable
- Simplicity: With
Callable
, you can avoid thread management, making your code cleaner. - Automatic Handling: Spring handles the threading, allowing you to focus on your business logic.
Chaining DeferredResult
You can also create more complex flows by chaining multiple DeferredResult
instances.
@GetMapping("/chained")
public DeferredResult<String> getChainedResponse() {
DeferredResult<String> result = new DeferredResult<>();
// Call the first task
DeferredResult<String> firstTask = performFirstTask();
firstTask.onCompletion(() -> {
// Once the first task is done, you can trigger the second task
DeferredResult<String> secondTask = performSecondTask();
secondTask.onCompletion(() -> result.setResult("Both tasks completed successfully!"));
secondTask.onError(() -> result.setErrorResult("Error in second task"));
});
result.onCompletion(() -> {
// Final clean-up logic
});
result.onTimeout(() -> result.setErrorResult("Request timed out"));
return result;
}
Explanation of Chaining
- Sequential Processing: Each
DeferredResult
represents a separate operation, and you can chain them to create workflows. - Callbacks: Callbacks are registered to handle successful or error completion, allowing for flexible flow control.
Error Handling in Deferred Results
Handling errors gracefully is critical to maintaining exceptional user experiences. Spring allows you to set error results on DeferredResult
, as seen in our previous examples.
Customizing Error Responses
deferredResult.setErrorResult(new ErrorResponse("Custom Error", "Detailed error message"));
Best Practices
- Timeout Handling: Always be wary of the possible timeout scenario and provide meaningful feedback when this occurs.
- Logging: Log errors for debugging purposes.
- Graceful Degradation: Always plan for failures by implementing fallback mechanisms or returning default values.
Final Considerations
Spring's DeferredResult
and Callable
are incredibly powerful tools for building asynchronous Spring MVC applications. They provide the means to evolve your applications to be more responsive and efficient while maintaining code simplicity and clarity.
By understanding when and how to use these features, you can enhance user experience significantly and make better use of your server resources.
For further exploration, consider the official Spring documentation on Spring Web MVC - Asynchronous Processing or learn more about Spring's Task Execution and Scheduling.
By mastering DeferredResult
and its interplay with asynchronous processing, you are well on your way to creating efficient, modern web applications.
Stay tuned for more in-depth articles on the Spring Framework!
Checkout our other articles