Common Pitfalls in Implementing Annotation Interfaces
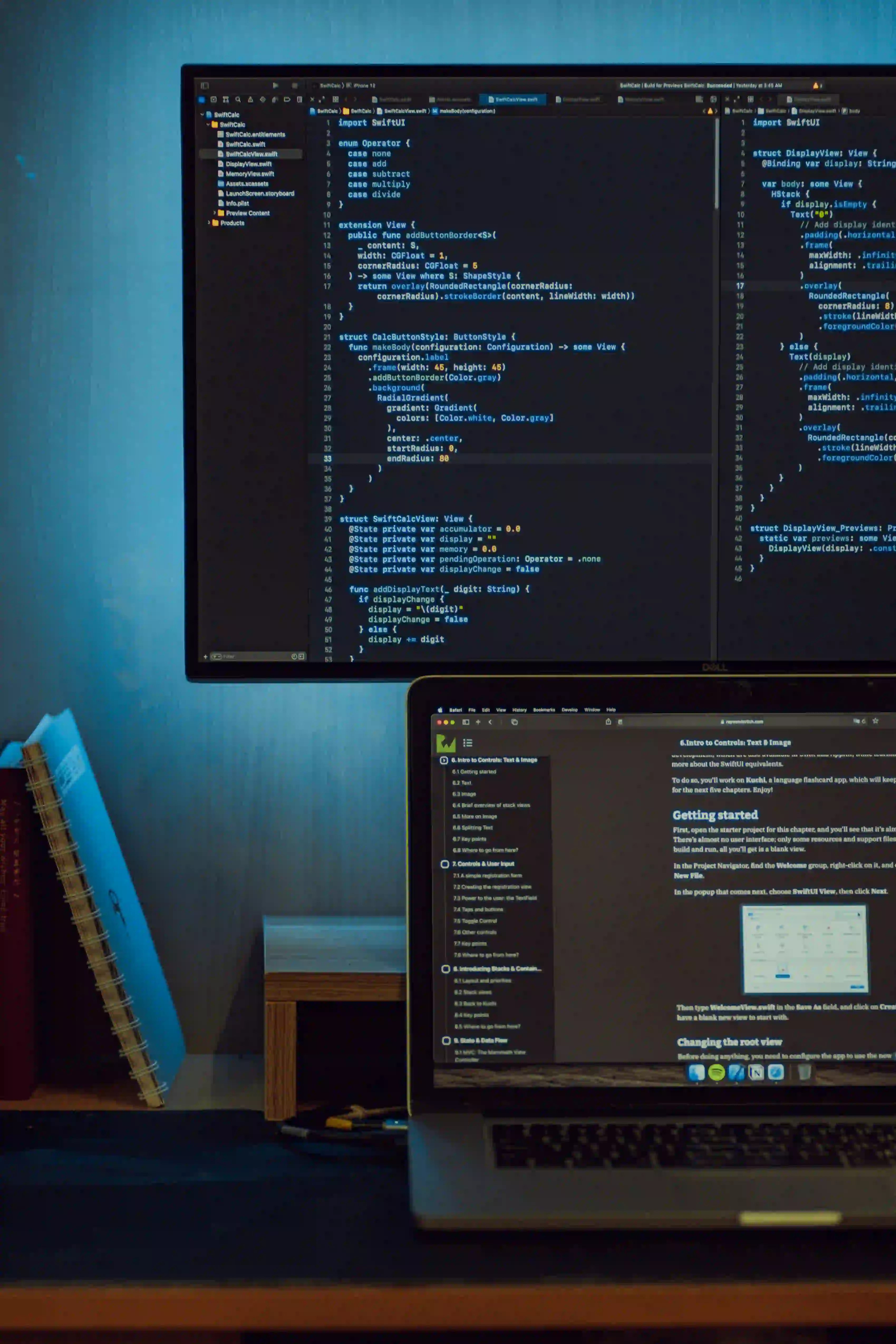
Common Pitfalls in Implementing Annotation Interfaces in Java
Java annotations are a powerful feature that allow developers to add metadata to code elements such as classes, methods, fields, and parameters. They can provide information for the compiler, enable special processing at runtime, and enhance code readability. However, implementing annotation interfaces can also lead to several common pitfalls that developers must be wary of.
In this blog post, we will explore these pitfalls, offering insights and best practices to help you navigate the complexities of annotation interfaces in Java.
What Are Annotation Interfaces?
An annotation in Java is defined using the @interface
keyword. It acts as a marker to provide information about the program without affecting its semantics. Annotations can be applied to various Java elements, and they can contain elements (which serve as attributes). Here is a basic example of an annotation interface:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyCustomAnnotation {
String value() default "default value";
int count() default 1;
}
In this example, the @Retention
annotation specifies that MyCustomAnnotation
will be available at runtime, while @Target
specifies that it can only be applied to methods.
Common Pitfalls in Implementing Annotation Interfaces
1. Misunderstanding Retention Policies
One of the most common pitfalls is misunderstanding the retention policy of an annotation. There are three retention policies defined in the java.lang.annotation.RetentionPolicy
enumeration:
SOURCE
: Annotations are discarded by the compiler.CLASS
: Annotations are recorded in the class file but are not available at runtime.RUNTIME
: Annotations are available at runtime through reflection.
Using the wrong retention policy can lead to misleading behavior and functionality. For instance, if you mark an annotation with SOURCE
, it won't be accessible during runtime. Thus, you won’t be able to process it using reflection.
Example:
@Retention(RetentionPolicy.SOURCE)
@interface SourceLevelAnnotation { }
In this case, SourceLevelAnnotation
won't be available for runtime processing, which is a common mistake made by developers unaware of to which lifecycle phase their annotations apply.
2. Annotation Element Defaults
Another common mistake is neglecting to define default values for annotation elements when appropriate. If the elements of an annotation require parameters, the users of your annotation will be forced to provide these parameters every time they use it.
Best Practice:
Always provide sensible defaults for annotation elements to simplify usage:
@interface MyAnnotation {
String name() default "Unknown";
int age() default 0;
}
By providing a default value, it allows developers to use the annotation without needing to fill in unnecessary information.
3. Excessive Use of Annotations
Annotations are a powerful tool but using them excessively can lead to clutter and confusion. Over-relying on annotations can make code less readable, making it challenging to identify logic at a glance.
A good rule of thumb is to use annotations for cross-cutting concerns like logging, security, or transaction management.
4. Not Using the Correct Target for Annotations
Java annotations are defined with specific targets such as ElementType.TYPE
, ElementType.METHOD
, ElementType.FIELD
, etc. Misusing these targets can lead to errors or code that doesn't behave as expected.
For example, if you create an annotation intended to be used on methods but mistakenly allow it on fields or parameters:
@Target({ElementType.METHOD, ElementType.FIELD})
@interface MyMethodOnlyAnnotation { }
In this case, MyMethodOnlyAnnotation
can be used on both methods and fields when it was likely intended for methods only. This can confuse users and lead to unintended behaviors.
5. Forgetting Documentation
Annotations serve not only as metadata but also as guidance or specifications for code behavior. When creating custom annotations, it is essential to provide proper JavaDoc comments. Documentation helps other developers understand how to use your annotation correctly.
Example:
/**
* This annotation marks a method as needing documentation review.
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface NeedsDocumentation { }
By documenting your annotations, not only do you make it easier for others to use them correctly, but you also improve maintainability in the long run.
6. Assume Default Behavior With Java Annotations
Many Java developers assume that annotations create a standard behavior without explicitly defining it. However, unless accompanied by processing code (like an Aspect or a Processor), the annotation itself will not alter code execution.
For instance, defining an annotation won't magically implement logging; you must implement the logic to invoke the correct actions according to the annotation.
Example Implementation:
Using reflection to process the annotation:
public void processAnnotations() {
for (Method method : MyClass.class.getDeclaredMethods()) {
if (method.isAnnotationPresent(NeedsDocumentation.class)) {
System.out.println("Documentation needed for method: " + method.getName());
}
}
}
This processing method iterates through the declared methods in MyClass
, providing functionality to the NeedsDocumentation
annotation.
7. Not Considering Annotations in Frameworks
Many Java frameworks make heavy use of annotations (like Spring, Hibernate, etc.). When creating custom annotations intended for use with these frameworks, you must familiarize yourself with how these frameworks handle annotations.
Ignoring the conventions or life cycle of these frameworks can result in your annotations being ignored or functioning incorrectly.
Closing Remarks
Java annotations are a valuable tool in the developers' toolbox, enabling powerful annotations-driven functionality. However, the implementation of annotation interfaces is fraught with potential pitfalls, as discussed in this blog post.
By keeping these common pitfalls in mind—such as understanding retention policies, providing default values, avoiding excessive use, using correct targets, documenting annotations, clarifying behavior, and being aware of framework integration—developers can leverage annotations' potential without falling into common traps.
Further Reading:
To learn more about Java annotations or delve deeper into the nuances of this powerful feature, consider the following resources:
Incorporating the best practices and being aware of common pitfalls when implementing annotation interfaces will undoubtedly enhance the robustness of your Java applications. Happy coding!