Mastering JDK 9 Compact Strings: Key Challenges Explained
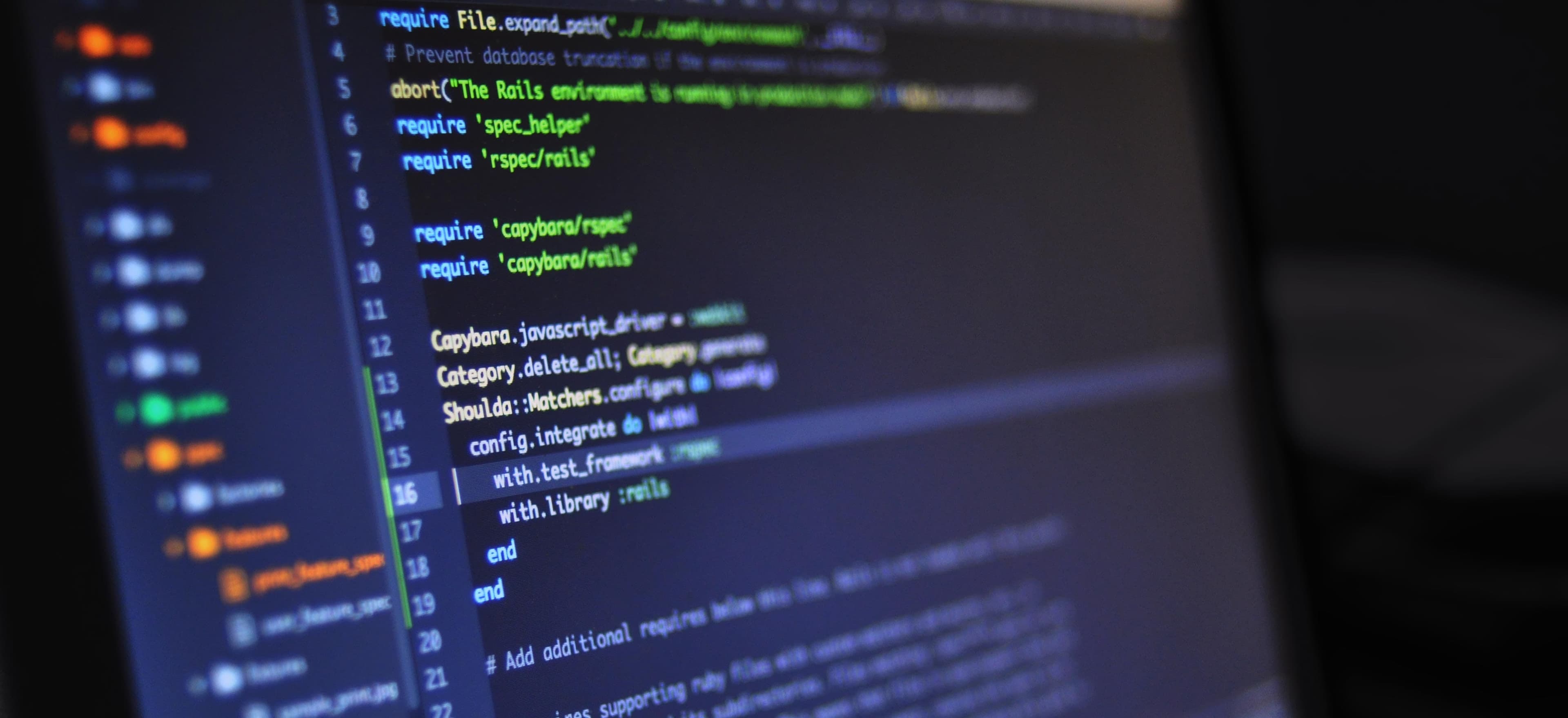
- Published on
Mastering JDK 9 Compact Strings: Key Challenges Explained
Java continues to evolve, providing developers with new features and enhancements that make programming more efficient and effective. One of the notable changes introduced in JDK 9 is the concept of Compact Strings. This modification fundamentally alters how Java handles String
storage in memory, aiming to optimize space consumption and overall performance. However, transitioning to this new system comes with its own set of challenges. In this post, we will delve into these challenges, the underlying mechanisms of Compact Strings, and what developers need to consider when working with Java after the introduction of JDK 9.
What Are Compact Strings?
In earlier versions of Java, the String
class stored character data as an array of char
elements. Each char
was 16 bits (2 bytes), resulting in potentially high memory usage, especially for strings containing only ASCII characters. JDK 9 introduced Compact Strings as a way to optimize this model.
With Compact Strings, Java uses a byte array to store the character data when possible. If a string contains only ASCII characters, it can be stored in 1 byte per character instead of 2. For strings containing larger Unicode characters, the traditional char[]
representation is still utilized.
Benefits of Compact Strings
- Memory Efficiency: Compact Strings reduce the memory footprint for strings made up of mostly ASCII characters.
- Performance Improvements: With a reduced memory footprint, cache efficiency improves, leading to better performance in string manipulation operations.
- Simplified Internal Handling: A unified internal representation streamlines string processing, making it easier for the Java Virtual Machine (JVM) to handle string data.
Key Challenges
While Compact Strings promise advantages, several challenges accompany their implementation. Let's explore these intricacies:
1. Transitioning Legacy Code
Challenge
One of the most significant challenges with the introduction of Compact Strings is updating and maintaining legacy codebases. Many older applications were developed with the assumption of the traditional String storage model.
Solution
To ease this transition, developers should perform code reviews and test existing functionalities thoroughly. Here’s a simple snippet that shows how you can identify if a string is using the compact representation:
String str = "Example";
System.out.println("Is string compact? " + isStringCompact(str));
private static boolean isStringCompact(String s) {
// Check if the string uses the byte array instead of char array.
return s.getClass().getDeclaredFields()[0].getName().equals("value");
}
Why This Matters: As the workings of a string change under the hood, understanding how your string is stored can help you optimize your code further and avoid unforeseen issues arising from implicit assumptions.
2. Performance Comparisons
Challenge
One of the concerns with the conversion to Compact Strings is understanding when performance could actually degrade. Operations that involve changing the character set or frequently modifying strings could incur additional overhead.
Solution
Performance testing is essential. Profiling existing applications with tools such as Java Mission Control or VisualVM will help pinpoint bottlenecks. Here’s a quick example to illustrate the performance comparison between typical string operations before and after adapting to compact strings:
public class StringPerformance {
public static void main(String[] args) {
long start = System.nanoTime();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1_000_000; i++) {
sb.append("Sample");
}
long end = System.nanoTime();
System.out.println("Elapsed time: " + (end - start) + " ns");
}
}
Why This Matters: Benchmarking allows developers to understand the performance impacts of Compact Strings under different workloads and choose the most efficient approach.
3. Memory Consumption Variability
Challenge
Developers must also recognize that not all strings will benefit equally from the compact representation. When working with strings that frequently change size or those containing mixed character sets, the memory benefits may be negligible.
Solution
Analyze your string usage patterns. If you regularly handle international characters, be aware that performance improvements might not be realized. Here’s how you can determine the storage format of strings programmatically:
public static void showStringDetails(String str) {
if (str.value.length == str.representation()) {
System.out.println("String is stored compactly.");
} else {
System.out.println("String is stored as char array.");
}
}
Why This Matters: Understanding your application's memory usage can help inform decisions to further optimize and maintain consistency.
4. Documentation and Development Training
Challenge
The introduction of Compact Strings also requires adequate documentation and training for developers. With changes in internal representations, new developers may not be familiar with the implications, leading to confusion and potential performance pitfalls.
Solution
Regularly update documentation and provide training sessions for developers. Educating your team about the benefits and challenges of Compact Strings helps foster a more prepared and informed development environment.
For comprehensive documentation and updates on Java features, visit Oracle's official documentation.
My Closing Thoughts on the Matter
The advent of Compact Strings in JDK 9 represents a significant improvement for Java developers, offering increased memory efficiency and performance potentials. However, the transition comes with challenges that can impact existing applications and the development process. It is crucial for developers to navigate these changes diligently, employing necessary analyses, testing, and training.
As you adapt to JDK 9 and its features, remember the foundational principles of Java development, focusing on efficiency, readability, and maintainability. By mastering the nuances of Compact Strings, you can maximize your development efforts and improve application performance in the competitive world of software development.
Remember, understanding the tools and systems behind Java advances helps you become a more proficient and effective developer. Happy coding!
Checkout our other articles